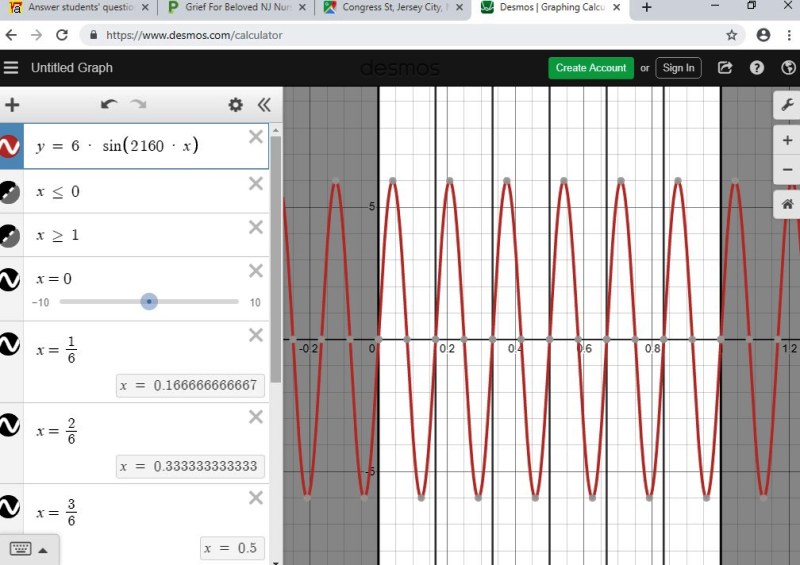
How to find all possible cycles in a directed graph?
Given below is the algorithm:
- Insert the edges into an adjacency list.
- Call the DFS function which uses the coloring method to mark the vertex.
- Whenever there is a partially visited vertex, backtrack till the current vertex is reached and mark all of them with cycle numbers. ...
How to detect a cycle in a directed graph?
Detect cycle in a directed graph. Given a Directed Graph with V vertices (Numbered from 0 to V-1) and E edges, check whether it contains any cycle or not. You dont need to read input or print anything. Your task is to complete the function isCyclic () which takes the integer V denoting the number of vertices and adjacency list as input parameters and returns a boolean value denoting if the given directed graph contains a cycle or not.
How to detect cycle in directed graph using DFS?
cycle detection for directed graph. union-find algorithm for cycle detection in undirected graphs. Approach: Run a DFS from every unvisited node. Depth First Traversal can be used to detect a cycle in a Graph. DFS for a connected graph produces a tree. There is a cycle in a graph only if there is a back edge present in the graph.
How to find a cycle in a graph?
Cycle in directed graphs can be detected easily using a depth-first search traversal. While doing a depth-first search traversal, we keep track of the nodes visited in the current traversal path in addition to the list of all the visited nodes. During the traversal of the current path, if we come to a node that was already marked visited then ...

Are directed graphs with no cycles?
Directed acyclic graphs (DAGs) are directed graphs with no directed cycles.
What is not allowed in a directed graph?
(You are not allowed to traverse edges in the wrong direction as part of a walk.) A path or cycle in a directed graph is said to be Hamiltonian if it visits every node in the graph. For example, a, b, d, c is the only Hamiltonian path for the graph in Figure 6.2.
How do you count the cycles on a directed graph?
To detect cycle, check for a cycle in individual trees by checking back edges. To detect a back edge, keep track of vertices currently in the recursion stack of function for DFS traversal. If a vertex is reached that is already in the recursion stack, then there is a cycle in the tree.
Does a directed graph allow loops?
Again, loops are allowed in directed graphs. In directed (multi)graphs, edges are drawn as arrows rather than lines.
Can a spanning tree have cycles?
A spanning tree is a subset of Graph G, which has all the vertices covered with minimum possible number of edges. Hence, a spanning tree does not have cycles and it cannot be disconnected..
How do you tell if a graph is directed?
If you are able to find edge of opposite direction for each edge in your list, you can treat your graph as undirected (or directed with 2 opposite directed edges per pair of connected nodes). Otherwise, it is directed. (considering example above, if for vertex b there is no vertex a in its adjacent vertices list).
How do you determine if an undirected graph has a cycle?
To detect if there is any cycle in the undirected graph or not, we will use the DFS traversal for the given graph. For every visited vertex v, when we have found any adjacent vertex u, such that u is already visited, and u is not the parent of vertex v. Then one cycle is detected.
Can a cycle have 2 vertices?
The cycle graph with n vertices is called Cn. The number of vertices in Cn equals the number of edges, and every vertex has degree 2; that is, every vertex has exactly two edges incident with it....Cycle graphNotationCnTable of graphs and parameters9 more rows
How many cycles are in a complete graph?
Actually a complete graph has exactly (n+1)! cycles which is O(nn).
Can undirected graphs have self loops?
No self loops are allowed in undirected graphs. That is, we cannot have (v, v), which would not make as much sense in the set notation {v, v}. We say that e = {u, v} is incident on u and v, and that the latter vertices are adjacent. The degree of a vertex is the number of edges incident on it.
Which of the following graphs has no loop?
A simple graph contains no loops.
Is a loop in a graph a cycle?
A loop is commonly defined as an edge (or directed edge in the case of a digraph) with both ends as the same vertex. (For example from a to itself). Although loops are cycles, not all cycles are loops.
Can a directed graph be complete?
A directed graph having no multiple edges or loops (corresponding to a binary adjacency matrix with 0s on the diagonal) is called a simple directed graph. A complete graph in which each edge is bidirected is called a complete directed graph.
Which of the following is not a type of graph?
Which of the following is not a type of graph in computer science? Explanation: According to the graph theory a graph is the collection of dots and lines. A bar graph is not a type of graph in computer science.
Can a directed graph be disconnected?
An edgeless graph with two or more vertices is disconnected. A directed graph is called weakly connected if replacing all of its directed edges with undirected edges produces a connected (undirected) graph.
Which of the following property is not true for a simple graph?
Which of the following properties does a simple graph not hold? Explanation: A simple graph maybe connected or disconnected. 10.
What does it mean when a graph has cycles?
And cycles in this kind of graph will mean deadlock — in other words, it means that to do the first task, we wait for the second task, and to do the second task, we wait for the first. So, detecting cycles is extremely important to avoid this situation in any application.
How to find cycles in a graph?
So, one famous method to find cycles is using Depth-First-Search (DFS). By traversing a graph using DFS, we get something called DFS Trees. The DFS Tree is mainly a reordering of graph vertices and edges. And, after building the DFS trees, we have the edges classified as tree edges, forward edges, back edges, and cross edges.
What does DAG mean in graphs?
Notice that with each back edge, we can identify cycles in our graph . So, if we remove the back edges in our graph, we can have a DAG (Directed Acyclic Graph).
What is the printing function?
Then, the function pops the elements from the stack and prints them, then pushes them back, using a temporary stack.
Can DFS be illustrated?
We can illustrate the main idea simply as a DFS problem with some modifications. The first thing is to make sure that we repeat the DFS from each unvisited vertex in the graph, as shown in the following flow-chart:
Should we mark all the back edges of a graph?
Instead, we should mark all the back edges found in our graph and remove them.
Can you print a cycle using a flow chart?
If our goal is to print the first cycle , we can use the illustrated flow-chart to print the cycle using the DFS stack and a temporary stack:
How many vertices can you find in a cycle?
For such a cycle, you can find two vertices on the path directly connected to each other (neighbors) that are both connected to t, therefore they form a cycle with length 3.
What is the shortest cycle?
The basic idea is that the shortest cycle has length 3 because (I assume that) t is connected to any other vertex through one and only one edge, and the other vertices do not form cycles without t.
Can a cycle be reduced to length 3?
Therefore, any cycle can be reduced to length 3.
Is there a shorter cycle than the one involving t, va, vb?
4a. if the edge is from t to vc, then there is a shorter cycle than the one involving [t, va, vb], because we can skip from t directly to vc, bypassing va; furthermore, if this new cycle is of length greater than 3, this process can then be repeated on the new cycle starting from step 1.
What is a directed graph?
In mathematics, and more specifically in graph theory, a directed graph (or digraph) is a graph that is made up of a set of vertices connected by directed edges often called arcs .
What is a symmetric directed graph?
Symmetric directed graphs are directed graphs where all edges are bidirected (that is, for every arrow that belongs to the digraph, the corresponding inversed arrow also belongs to it).
How many arcs are there in a semicomplete digraph?
Note that there can be one arc between x and y or two arcs in the opposite directions. Semicomplete digraphs are simple digraphs where there is an arc between each pair of vertices. Every semicomplete digraph is a semicomplete multipartite digraph, where the number of vertices equals the number of partite sets.
What is flow graph?
Flow graphs are digraphs associated with a set of linear algebraic or differential equations.
What is control flow graph?
Control-flow graphs are rooted digraphs used in computer science as a representation of the paths that might be traversed through a program during its execution.
Is a complete digraph symmetric?
Complete directed graphs are simple directed graphs where each pair of vertices is joined by a symmetric pair of directed arcs (it is equivalent to an undirected complete graph with the edges replaced by pairs of inverse arcs). It follows that a complete digraph is symmetric.
Do isomorphic digraphs have the same degree sequence?
However, the degree sequence does not, in general, uniquely identify a directed graph; in some cases, non-isomorphic digraphs have the same degree sequence.
Why can't a graph have cycles?
A graph that has a topological ordering cannot have any cycles, because the edge into the earliest vertex of a cycle would have to be oriented the wrong way. Therefore, every graph with a topological ordering is acyclic. Conversely, every directed acyclic graph has at least one topological ordering.
What is a directed acyclic graph?
In mathematics, particularly graph theory, and computer science, a directed acyclic graph ( DAG or dag / ˈdæɡ / ( listen)) is a directed graph with no directed cycles. That is, it consists of vertices and edges (also called arcs ), with each edge directed from one vertex to another, ...
How to make an undirected graph into a DAG?
Any undirected graph may be made into a DAG by choosing a total order for its vertices and directing every edge from the earlier endpoint in the order to the later endpoint. The resulting orientation of the edges is called an acyclic orientation. Different total orders may lead to the same acyclic orientation, so an n -vertex graph can have fewer than n! acyclic orientations. The number of acyclic orientations is equal to |χ(−1)|, where χ is the chromatic polynomial of the given graph.
How to check if a graph is a DAG?
It is also possible to check whether a given directed graph is a DAG in linear time, either by attempting to find a topological ordering and then testing for each edge whether the resulting ordering is valid or alternatively, for some topological sorting algorithms, by verifying that the algorithm successfully orders all the vertices without meeting an error condition.
How are graphs formed?
A graph is formed by vertices and by edges connecting pairs of vertices, where the vertices can be any kind of object that is connected in pairs by edges. In the case of a directed graph, each edge has an orientation, from one vertex to another vertex. A path in a directed graph is a sequence of edges having the property that the ending vertex ...
What happens when you add red edges to a blue graph?
Adding the red edges to the blue directed acyclic graph produces another DAG, the transitive closure of the blue graph. For each red or blue edge u → v, v is reachable from u: there exists a blue path starting at u and ending at v.
Does every acyclic graph have a topological order?
Conversely, every directed acyclic graph has at least one topological ordering. The existence of a topological ordering can therefore be used as an equivalent definition of a directed acyclic graphs: they are exactly the graphs that have topological orderings.

Introduction
Problem Explanation
- In a directed graph, we’d like to find cycles. These cycles can be as simple as one vertex connected to itself or two vertices connected as shown: Or, we can have a bigger cycle like the one shown in the next example, where the cycle is B-C-D-E: Also, we can get graphs with multiple cycles intersecting with each other as shown (we have three cycles...
Algorithm Idea
- Finding cycles in a simple graph as in the first two examples in our article is simple. We can traverse all the vertices and check if any of them is connected to itself or connected to another vertex that is connected to it. But, of course, this isn’t going to work in more complex scenarios. So, one famous method to find cycles is using Depth-First-Search (DFS). By traversing a graph u…
Flow-Chart
- We can illustrate the main idea simply as a DFS problem with some modifications. The first thing is to make sure that we repeat the DFS from each unvisited vertex in the graph, as shown in the following flow-chart: The second part is the DFS processing itself. In this part, we need to make sure we have access to what is in the stack of the DFS to be able to check for the back edges. A…
Pseudocode
- Our next part of this tutorial is a simple pseudocode for detecting cycles in a directed graph. In this algorithm, the input is a directed graph. For simplicity, we can assume that it’s using an adjacency list. The first function is an iterative function that reads the graph and creates a list of flags for the graph vertices (called visited in this pseudocode) that are initially marked as NOT_V…
Complexity
- The time complexity of the given algorithm is mainly the DFS, which is . As for the space-complexity, we need to allocate space for marking the vertices and for the stack, so it’ll be in the order of .
Conclusion
- In this tutorial, we covered one of the algorithms to detect cycles in directed graphs. At first, we discussed one of the important applications for this algorithm. Then, we explained the idea and showed the general algorithm idea using examples, flow-charts, and pseudocode. Finally, we showed an average space and time complexity for the algorithm.