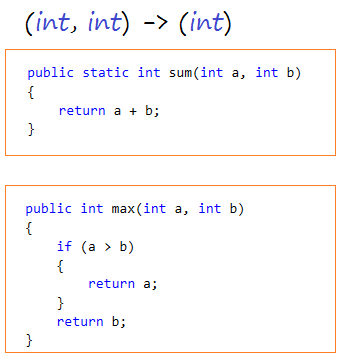
You can specify an event in an interface, but you can't declare a delegate (or any other type) - at least not in C#. For instance:
Full Answer
What is the difference between interface and delegate?
Delegates in C# are similar to the function pointer in C/C++. It provides a way which tells which method is to be called when an event is triggered. Like a class, Interface can have methods, properties, events, and indexers as its members. But interfaces will contain only the declaration of the members.
What are events and delegates in C #?
Events and Delegates in C# are undoubtedly among the most confusing topics. Let us explore more about these. The code used in this article can be downloaded from GitHub. Delegates are function pointers. Let’s write some code using delegates to understand delegates.
What is the use of delegate?
Delegates are ideally suited for use as events - notifications from one component to "listeners" about changes in that component. For more information on the use of delegates for events, Delegates and interfaces are similar in that they enable the separation of specification and implementation.
How to declare a delegate variable and event at the same time?
C# provides a simple way of declaring both a delegate variable and an event at the same time. This is called a field-like event, and is declared very simply - it's the same as the "longhand" event declaration, but without the "body" part: This creates a delegate variable and an event, both with the same type.
What is an interface in programming?
What does the interface inherited within a derived class do?
What happens if you forget to add delegates?
How to enforce coding standards?
Can you hook event args in any handler?
Do delegate types belong in an interface?
Can you customize event handlers?
See 4 more
About this website

Can interface contain delegates?
Interface can be inherited and implemented by any class and a Delegate can be created for a method on any class, as long as the method suits the method signature of the delegate. An Interface or Delegate is being used by an object. Both have no knowledge of the class that implement.
CAN interface have events?
An interface can declare an event. The following example shows how to implement interface events in a class. Basically the rules are the same as when you implement any interface method or property.
Can we declare delegate inside interface and why?
A Delegate is just another type, so you don't gain anything by putting it inside the interface. You shouldn't need to create your own delegates. Most of the time you should just use EventHandler, Func, Predicate, or Action.
CAN interface have events in C#?
An interface can be created to define a contract containing members that classes that implement it must provide. Interfaces can define events, sometimes leading to classes that implement several interfaces being required to declare an event name twice.
What is the difference between event and delegate in C#?
An event is declared using the event keyword. Delegate is a function pointer. It holds the reference of one or more methods at runtime. Delegate is independent and not dependent on events.
Is event an object?
An event, like a button click, is represented as an object. When the user generates an event, the system creates an event object which is then sent to the listener that has been registered for the GUI component. Then, a method in the listener object is invoked.
What is difference between interface and delegate?
Delegates and Interfaces are two distinct concepts in C#. Interfaces allow to extend some object's functionality, it's a contract between the interface and the object that implements it, while delegates are just safe callbacks, they are a sort of function pointers.
Are delegates static?
Static delegates are not without limitations. They can only refer to static functions; member methods on objects are not permitted because there is no place to store the pointer to the object. Furthermore, static delegates cannot be chained to other delegates.
Why delegates why not call methods directly?
If you think of delegates as being similar to interface definitions for a specific type of method, you can start to see why delegates exist. They allow clients of our delegates to ignore all the details of their implementations - even their names!
CAN interface have private methods in C#?
Interface cannot include private, protected, or internal members. All the members are public by default. Interface cannot contain fields, and auto-implemented properties. A class or a struct can implement one or more interfaces implicitly or explicitly.
What is callback delegate in C#?
Callback by Delegate Delegate provides a way to pass a method as argument to other method. To create a Callback in C#, function address will be passed inside a variable. So, this can be achieved by using Delegate. The following is an example of Callback by Delegate.
When would you use delegates instead of interfaces?
If you only needed one implementation of a method... use a method (perhaps a virtual method). As with interfaces, part of the point of delegates is that you can substitute multiple different implementations.
What does an interface Do C#?
An interface defines a contract. Any class or struct that implements that contract must provide an implementation of the members defined in the interface. Beginning with C# 8.0, an interface may define a default implementation for members.
What are events in C# with example?
Events are user actions such as key press, clicks, mouse movements, etc., or some occurrence such as system generated notifications. Applications need to respond to events when they occur. For example, interrupts.
Cannot put delegate in interface - why not? - C# / C Sharp
An ***instance*** of a delegate is (essentially) a function pointer; however, starting with the word "delegate" (with a small d) here defines the
c# - Why can't I put a delegate in an interface? - Stack Overflow
As others have mentioned, you can only define delegates outside of the interface.. There is little to nothing wrong w/ using delegates. Personally I think that Func
c# - implementing delegate in interface - Stack Overflow
Moving the callback to the interface is required if you want to use the callback without casing to a concrete type. Note that your current implementation has callback as a field.To declare it in an interface, you must make it a property.. Because properties are really methods, you must implement the property in your concrete class. Using an auto-property is fine for the implementation here.
Create and Invoke C# Delegate - Dot Net For All
Create C# Delegate. As mentioned in one of my article delegate is a reference type and CLR converts delegate into a class while converting it into IL.. Action Func and Predicate were introduced in the C# 2.0. And it helped us not to create our own delegate every time we need one.
Delegates vs Interfaces in C# - GeeksforGeeks
A Delegate is an object which refers to a method or you can say it is a reference type variable that can hold a reference to the methods. Delegates in C# are similar to the function pointer in C/C++.It provides a way which tells which method is to be called when an event is triggered. Example:
What is an interface in programming?
Interfaces are means of abstraction, used in Object Oriented programming and design methods. Maybe you don't need an interface declaration at all, unless you want to see some concrete class instances as the interface elsewhere in your program (Abstraction).
What does the interface inherited within a derived class do?
The interface inherited within your derived class will remind you to define and link up the stuff you declared in it.
What happens if you forget to add delegates?
Using code analysis, if you "forget" to add the delegates (although I don't see the point of forgetting it, as if the delegate is not used, it's not needed) you'll get a warning / error.
How to enforce coding standards?
If you want to enforce some coding standards in your project, you may want to try using code analysis tools (like in Visual Studio) - They allow extensions, that you can incorporate to add you own code analysis rules.
Can you hook event args in any handler?
Now that the event args are returning your types, you can hook them in any handler you define.
Do delegate types belong in an interface?
They don't belong in an interface. The events using those delegate types are fine to be in the interface though: The implementation won't (and shouldn't) redeclare the delegate type, any more than it would redeclare any other type used in an interface.
Can you customize event handlers?
All you have to do is declare your event handlers as generic event handlers in both the interface and your implementation and you can customize the return results.
Why do we use events over delegates?
So, events are encapsulated delegates that provide an extra layer of security. This is the reason why we prefer using events over delegates.
How does a delegate help us?
Delegate help us reduce coupling. In the future, if we want an address verification, we simply have to add a new class for address verification and point a method of this class to the delegate instance. If we had not used delegates here, we would have simply called the email verification & SMS verification methods in RegisterAUser method. In this case, every time we add a new verification, RegisterUser class gets modified increasing coupling.
Do you have to declare a delegate in DotNet?
Every time you declare an event, you do not have to declare a delegate too. Dotnet provides an inbuilt delegate called EventHandler which can be used directly while calling an event as below.
How are delegate and interface similar?
Delegates and interfaces are similar in that they enable the separation of specification and implementation. Multiple independent authors can produce implementations that are compatible with an interface specification. Similarly, a delegate specifies the signature of a method, and authors can write methods that are compatible with the delegate specification. When should you use interfaces, and when should you use delegates?
What is a delegate in C#?
A delegate in C# is similar to a function pointer in C or C ++. Using a delegate allows the programmer to encapsulate a reference to a method inside a delegate object.
How to call a delegate object?
A delegate object is called by using the name of the delegate object, followed by the parenthesized arguments to be passed to the delegate.
What is a delegate declaration?
A delegate declaration defines a type that encapsulates a method with a particular set of arguments and return type. For static methods, a delegate object encapsulates the method to be called. For instance methods, a delegate object encapsulates both an instance and a method on the instance.
Why use delegate in a bookstore?
The use of delegates promotes good separation of functionality between the bookstore database and the client code. The client code has no knowledge of how the books are stored or how the bookstore code finds paperback books. The bookstore code has no knowledge of what processing is done on the paperback books after it finds them.
What is the property of a delegate?
An interesting and useful property of a delegate is that it does not know or care about the class of the object that it references. Any object will do; all that matters is that the method's argument types and return type match the delegate's. This makes delegates perfectly suited for "anonymous" invocation. Note.
What is the useful property of delegate objects?
A useful property of delegate objects is that they can be composed using the "+" operator. A composed delegate calls the two delegates it was composed from. Only delegates of the same type can be composed. The "-" operator can be used to remove a component delegate from a composed delegate. using System;
What is an event handler class?from codereview.stackexchange.com
class EventHandler is an abstract class which wraps the handler methods.
How many Q&A communities are there on Stack Exchange?from codereview.stackexchange.com
Stack Exchange network consists of 178 Q&A communities including Stack Overflow, the largest, most trusted online community for developers to learn, share their knowledge, and build their careers.
Is there a C++ event system?from stackoverflow.com
There is a native Visual C++ event system. It's mostly for COM, but it has native C++ support too.
Using Visual Studio to add an event handler
A convenient way of adding an event handler to your project is by using the XAML Designer user interface (UI) in Visual Studio. With your XAML page open in the XAML Designer, select the control whose event you want to handle.
Register a delegate to handle an event
A simple example is handling a button's click event. It's typical to use XAML markup to register a member function to handle the event, like this.
Revoke a registered delegate
When you register a delegate, typically a token is returned to you. You can subsequently use that token to revoke your delegate; meaning that the delegate is unregistered from the event, and won't be called should the event be raised again.
Delegate types for asynchronous actions and operations
The examples above use the RoutedEventHandler delegate type, but there are of course many other delegate types. For example, asynchronous actions and operations (with and without progress) have completed and/or progress events that expect delegates of the corresponding type.
Delegate types that return a value
Some delegate types must themselves return a value. An example is ListViewItemToKeyHandler, which returns a string. Here's an example of authoring a delegate of that type (note that the lambda function returns a value).
Safely accessing the this pointer with an event-handling delegate
If you handle an event with an object's member function, or from within a lambda function inside an object's member function, then you need to think about the relative lifetimes of the event recipient (the object handling the event) and the event source (the object raising the event).
Introduction
This article introduces a very simple and comfortable event mechanism in C++. In many cases, a delegate in C++ is hard to understand because of its complexity. The attached code is a sample that uses the combination of the C# delegation style and Java's inner class callback style. It can show you an example of an easy event mechanism.
Points of Interest
You can easily find the reason why using the delegate is nice. It can break the cyclic dependency of each class - the 'Caller' depends on the 'Callee' but the 'Callee' does not have dependency with any classes.
License
This article, along with any associated source code and files, is licensed under The Code Project Open License (CPOL)
What is a delegate in C#?
A Delegate is an object which refers to a method or you can say it is a reference type variable that can hold a reference to the methods. Delegates in C# are similar to the function pointer in C/C++. It provides a way which tells which method is to be called when an event is triggered.
When you access a method using delegates, do you need to access the object of the class where the method is?
When you access the method you need the object of the class which implemented an interface. It does not support inheritance. It supports inheritance.
What is an interface's output?
Output: Like a class, Interface can have methods, properties, events, and indexers as its members. But interfaces will contain only the declaration of the members. The implementation of the interface’s members will be given by the class who implements the interface implicitly or explicitly.
Can an interface be used as a method?
Interface. It could be a method only. It contains both methods and properties. It can be applied to one method at a time. If a class implements an interface, then it will implement all the methods related to that interface. If a delegate available in your scope you can use it.
Can a class implement any number?
It can implement any method that provides the same signature with the given delegate. If the method of interface implemented, then the same name and signature method override. It can wrap any method whose signature is similar to the delegate and does not consider which from class it belongs. A class can implement any number ...
How to combine two delegate instances?
Combining two delegate instances is usually done using the addition operator, as if the delegate instances were strings or numbers. Subtracting one from another is usually done with the subtraction operator. Note that when you subtract one combined delegate from another, the subtraction works in terms of lists . If the list to subtract is not found in the original list, the result is just the original list. Otherwise, the last occurrence of the list is removed. This is best shown with some examples. Instead of actual code, the following uses lists of simple delegates d1, d2 etc. For instance, [d1, d2, d3] is a combined delegate which, when executed, would call d1 then d2 then d3. An empty list is represented by null rather than an actual delegate instance.
What are the key points of data in a delegate instance?
As mentioned earlier, the key points of data in any particular delegate instance are the method the delegate refers to, and a reference to call the method on ( the target ). For static methods, no target is required. The CLR itself supports other slightly different forms of delegate, where either the first argument passed to a static method is held within the delegate, or the target of an instance method is provided as an argument when the method is called. See the documentation for System.Delegate for more information on this if you're interested, but don't worry too much about it.
What are the methods of a delegate?
Any delegate type you create has the members inherited from its parent types, one constructor with parameters of object and IntPtr and three extra methods: Invoke, BeginInvoke and EndInvoke. We'll come back to the constructor in a minute. The methods can't be inherited from anything, because the signatures vary according to the signature the delegate is declared with. Using the sample code above, the first delegate has the following methods:
Why is field-like event locking used in add/remove?
Earlier we touched on field-like events locking during the add/remove operations. This is to provide a certain amount of thread safety. Unfortunately, it's not terribly useful. Firstly, even with 2.0, the spec allows for the lock to be the reference to this object, or the type itself for static events. That goes against the principle of locking on privately held references to avoid accidental deadlocks.
How to understand events?
I find the easiest way to understand events is to think of them a bit like properties. While properties look like they're fields, they're definitely not - and you can write properties which don't use fields at all. Similarly, while events look like delegate instances in terms of the way you express the add and remove operations, they're not.
What is an event in IL?
Events are pairs of methods, appropriately decorated in IL to tie them together and let languages know that the methods represent events. The methods correspond to add and remove operations, each of which take a delegate instance parameter of the same type (the type of the event).
Why do you call end invoke?
Note that you must call EndInvoke when you use asynchronous execution in order to guarantee not to leak memory or handles. Some implementations may not leak, but you shouldn't rely on this. See my thread-pool article for some sample code to allow "fire and forget" style asynchronous behaviour if this is inconvenient.
What is an interface in programming?
Interfaces are means of abstraction, used in Object Oriented programming and design methods. Maybe you don't need an interface declaration at all, unless you want to see some concrete class instances as the interface elsewhere in your program (Abstraction).
What does the interface inherited within a derived class do?
The interface inherited within your derived class will remind you to define and link up the stuff you declared in it.
What happens if you forget to add delegates?
Using code analysis, if you "forget" to add the delegates (although I don't see the point of forgetting it, as if the delegate is not used, it's not needed) you'll get a warning / error.
How to enforce coding standards?
If you want to enforce some coding standards in your project, you may want to try using code analysis tools (like in Visual Studio) - They allow extensions, that you can incorporate to add you own code analysis rules.
Can you hook event args in any handler?
Now that the event args are returning your types, you can hook them in any handler you define.
Do delegate types belong in an interface?
They don't belong in an interface. The events using those delegate types are fine to be in the interface though: The implementation won't (and shouldn't) redeclare the delegate type, any more than it would redeclare any other type used in an interface.
Can you customize event handlers?
All you have to do is declare your event handlers as generic event handlers in both the interface and your implementation and you can customize the return results.
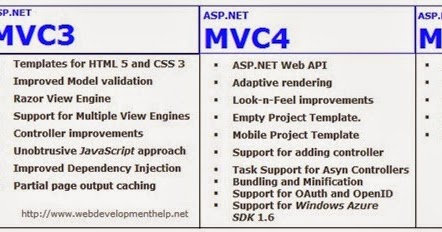
Delegates and Events
- A delegate in C# is similar to a function pointer in C or C++. Using a delegate allows the programmer to encapsulate a reference to a method inside a delegate object. The delegate object can then be passed to code which can call the referenced method, without having to know at compile time which method will be invoked. Unlike function pointers in C...
Example 1
- The following example illustrates declaring, instantiating, and using a delegate. The BookDB class encapsulates a bookstore database that maintains a database of books. It exposes a method ProcessPaperbackBooks, which finds all paperback books in the database and calls a delegate for each one. The delegate type used is called ProcessBookDelegate. The Test class uses this cl…
Declaring A Delegate
- The following statement, declares a new delegate type. Each delegate type describes the number and types of the arguments, and the type of the return value of methods that it can encapsulate. Whenever a new set of argument types or return value type is needed, a new delegate type must be declared.
Instantiating A Delegate
- Once a delegate type has been declared, a delegate object must be created and associated with a particular method. Like all other objects, a new delegate object is created with a new expression. When creating a delegate, however, the argument passed to the new expression is special - it is written like a method call, but without the arguments to the method. The following statement, cr…
Calling A Delegate
- Once a delegate object is created, the delegate object is typically passed to other code that will call the delegate. A delegate object is called by using the name of the delegate object, followed by the parenthesized arguments to be passed to the delegate. An example of a delegate call is, A delegate can either be called synchronously, as in this example, or asynchronously by using Begi…
Example 2
- This example demonstrates composing delegates. A useful property of delegate objects is that they can be composed using the "+" operator. A composed delegate calls the two delegates it was composed from. Only delegates of the same type can be composed. The "-" operator can be used to remove a component delegate from a composed delegate. Output Invoking delegate a: Hello, …
Delegates vs. Interfaces
- Delegates and interfaces are similar in that they enable the separation of specification and implementation. Multiple independent authors can produce implementations that are compatible with an interface specification. Similarly, a delegate specifies the signature of a method, and authors can write methods that are compatible with the delegate specification. When should yo…