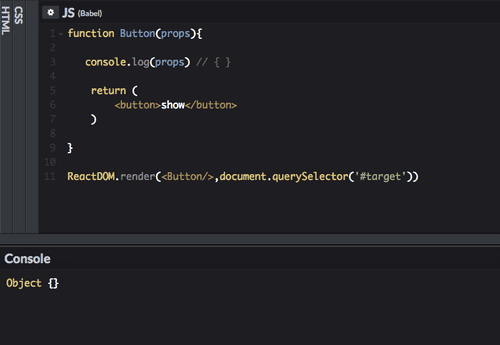
See more

Can we change the props value?
You can't change the value of props but you still have options to change values that you can use. You have two options: Either you change it from the parent component or you use state instead of props. Where ChildComponent has your code to use this.props.name .
How do you change props on a React element?
How to use props in ReactStep 1 - Pass in props as an argument. We did that in the first line of the code above: function Tool(props){} . ... Step 2 - Declare props variable(s) const name = props. ... Step 3 - Use variable(s) in JSX template. ... Step 4 - Pass data to props in the App component.
Can props be updated or read only?
Props are read-only components. It is an object which stores the value of attributes of a tag and work similar to the HTML attributes. It allows passing data from one component to other components.
Is props immutable in React?
Let's come to the main question “Are React props immutable?” No, it's not completely true. React props are shallow immutable. It will change the original propertyName in the Parent component also.
How do you change a prop?
0:001:47HOW TO: Change your propeller - YouTubeYouTubeStart of suggested clipEnd of suggested clipFirst you need the right tools needle nose pliers and a prop wrench step one remove the cotter pin.MoreFirst you need the right tools needle nose pliers and a prop wrench step one remove the cotter pin. Step two remove the retaining ring. Step 3 remove the prop nut. Step 4 remove the propeller.
Why we should not change props in React?
The React philosophy is that props should be immutable and top-down. This means that a parent can send whatever prop values it likes to a child, but the child cannot modify its own props. What you do is react to the incoming props and then, if you want to, modify your child's state based on incoming props.
Can child components change props?
Solution : As I had mentioned earlier — there are no direct ways to mutate a prop directly in child components. However when we pass the props from parent to child, we can also pass the function(with the logic) which changes the props.
Does changing props cause re-render React?
⛔️ Re-renders reason: props changes (the big myth) In order for props to change, they need to be updated by the parent component. This means the parent would have to re-render, which will trigger re-render of the child component regardless of its props.
Are props read only React?
Props are Read-Only Such functions are called “pure” because they do not attempt to change their inputs, and always return the same result for the same inputs. React is pretty flexible but it has a single strict rule: All React components must act like pure functions with respect to their props.
Are React props always objects?
React props can be accessed as an object or destructured Or they can be destructured, since props will always be an object, into separate variables. If you have a lot of props that you're passing down to your component, it may be best to include them on the entire props object and access them by saying props.
Can props be state?
It is Immutable (cannot be modified). It is Mutable ( can be modified). Props can be used with state and functional components. State can be used only with the state components/class component (Before 16.0).
Are props reactive?
Props and data are both reactive With Vue you don't need to think all that much about when the component will update itself and render new changes to the screen. This is because Vue is reactive. Instead of calling setState every time you want to change something, you just change the thing!
How do I change the props value in a React functional component?
Updating Props From Method Calls1render() { 2 return ( 3
6
How do you change a prop on a child component?
Solution : As I had mentioned earlier — there are no direct ways to mutate a prop directly in child components. However when we pass the props from parent to child, we can also pass the function(with the logic) which changes the props.
How do I edit props in React dev tools?
Editing Props and StateSelect the third ListItem in the Components tree.In the right side panel, click to expand the item prop.Click to toggle the isComplete attribute.The item should now be checked in the example app above.
How do you replace an object in React?
To replace an object in an array in React state: Use the map() method to iterate over the array. On each iteration, check if a certain condition is met. Replace the object that satisfies the condition and return all other objects as is.
When can props change?
Props can change when a component's parent renders the component again with different properties. I think this is mostly an optimization so that no new component needs to be instantiated.
What is the purpose of props in react?
PROPS. A React component should use props to store information that can be changed, but can only be changed by a different component. STATE. A React component should use state to store information that the component itself can change. A good example is already provided by Valéry.
What is the purpose of react state?
A React component should use state to store information that the component itself can change.
Is componentWillReceiveProps read only?
Much has changed with hooks, e.g. componentWillReceiveProps turned into useEffect + useRef ( as shown in this other SO answer ), but Props are still Read-Only, so only the caller method should update it.
Can a component update its own props?
A component cannot update its own props unless they are arrays or objects (having a component update its own props even if possible is an anti-pattern), but can update its state and the props of its children.
How many effect hooks are needed for a text prop?
This component will require two effect hooks. The first is to be called only when the text prop has been updated and will check if there is no highlight in progress and, if not, will call the setUpdate function defined in the previous section:
When does the effect hook run?
The effect hook can either run after every render, only on mount and unmount, or when specified values have been changed. It takes two parameters - the first is the function to execute and the second is an optional array of variables that, when changed, will trigger execution of the function.
What is the second effect hook?
The second effect hook this component requires is one to clean up the timer reference when the component unmounts. This can be achieved by passing an empty array as the second parameter and returning a function that will then be called when the component is being umounted. The code for this looks like:
What happens if a hook is called with no second parameter?
If the hook is called with no second parameter then the function will be called every time the component is updated like this:
Can you add side effects to a class in React?
It is relatively simple to add side-effects into both functional and class components using React. The code for the components in this guide can be found here.
What is a child component in React?
You are calling child component. While you are doing that, you are also passing the state of the parent ( parentName) to the child. In the child component, this state will be accessed as this.props.childName. Fair enough.
Does parent name change in react?
Now if there is any change of name required, parentName will be changed in the parent and that change will automatically be communicated to the child as is the case with the React mechanism. This setup works in most of the scenarios.
Can props be updated?
Props are never to be updated. We are to use them as is. Sounds rigid right? But React has its reasons behind this rule and I’m pretty convinced by their reasoning. The only caveat is, though, that there are situations where we might need to initiate the update of a prop. And we will soon know how.
Is react anti-pattern?
React argues against this and they are pretty right about it. This is anti-pattern. So whenever you run into a situation like this, check to see if you could lift your state up or if there is any way you could break your component down. It might sound a wee bit tedious, but know that that’s the way it is supposed to be in React!
Can you update a component's prop in ReactJS?
How to update a component’s prop in ReactJS — oh yes, it’s possible. If you have read the official React docs (and you should, as it is one great resource on React) you’d notice these lines: Whether you declare a component as a function or a class, it must never modify its own props.
Where do you name props?
We recommend naming props from the component’s own point of view rather than the context in which it is being used.
Where is the React app component?
Typically, new React apps have a single App component at the very top. However, if you integrate React into an existing app, you might start bottom-up with a small component like Button and gradually work your way to the top of the view hierarchy.
What is the name of the welcome component in React?
React calls the Welcome component with {name: 'Sara'} as the props.
When react sees an element representing a user-defined component, it passes JSX attributes and children to this?
When React sees an element representing a user-defined component, it passes JSX attributes and children to this component as a single object. We call this object “props”.
Is react flexible?
React is pretty flexible but it has a single strict rule:
Is a function a component of react?
This function is a valid React component because it accepts a single “props” (which stands for properties) object argument with data and returns a React element. We call such components “function components” because they are literally JavaScript functions.
React Props
React Props are like function arguments in JavaScript and attributes in HTML.
Pass Data
Props are also how you pass data from one component to another, as parameters.
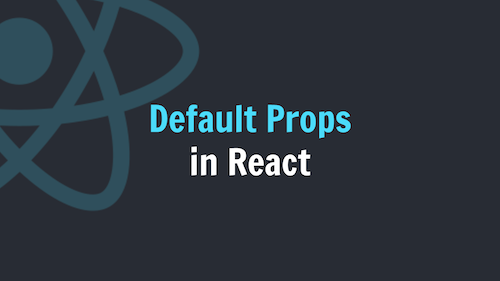
Popular Posts:
1.reactjs - How to Change Props value in React Js? - Stack …
Url:https://stackoverflow.com/questions/58486512/how-to-change-props-value-in-react-js
29 hours ago Web5. props should not be changed in react, they are readonly. update them in the parent component and then pass them down as the new value. the component receiving them …
2.Can I update a component's props in React.js? - Stack …
Url:https://stackoverflow.com/questions/24939623/can-i-update-a-components-props-in-react-js
10 hours ago WebPROPS. A React component should use props to store information that can be changed, but can only be changed by a different component. STATE. A React component should use …
3.Videos of Can Props Be Changed React
Url:/videos/search?q=can+props+be+changed+react&qpvt=can+props+be+changed+react&FORM=VDRE
18 hours ago WebThe first parameter for this method is the props before the update; so, testing whether a prop has changed can be done here. For this component the method will look like this: 1 …
4.Reacting to Prop Changes in a React Component
Url:https://www.pluralsight.com/guides/prop-changes-in-react-component/
13 hours ago WebA React aspect should use props to shop data that can be changed, but can merely be converted through an additional component. A React element should use state to store …
5.How to update a component’s prop in ReactJS — oh yes, …
Url:https://www.freecodecamp.org/news/how-to-update-a-components-prop-in-react-js-oh-yes-it-s-possible-f9d26f1c4c6d/
24 hours ago WebWhether you declare a component as a function or a class, it must never modify its own props. React is pretty flexible but it has a single strict rule: All React components must …
6.Components and Props – React
Url:https://reactjs.org/docs/components-and-props.html
26 hours ago Webprops should not be changed in react, they are readonly. update them in the parent component and then pass them down as the new value. the component receiving them …
7.How to Use Props in React.js
Url:https://www.freecodecamp.org/news/how-to-use-props-in-reactjs/
1 hours ago WebThis component can be tricky to change because of all the nesting, and it is also hard to reuse individual parts of it. Let’s extract a few components from it. First, we will extract …
8.React Props - W3Schools
Url:https://www.w3schools.com/react/react_props.asp
21 hours ago WebProps in React are inputs that you pass into components. The props enable the component to access customised data, values, and pieces of information that the inputs hold. The …