Can we write private static void main String args?
It has to be public so that java runtime can execute this method. Remember that if you make any method non-public then it's not allowed to be executed by any program, there are some access restrictions applied. So it means that the main method has to be public.
Do I need public static void main in Java?
Java :public static void main(String[] args) The main() method is a special method in Java Programming that serves as the externally exposed entrance point by which a Java program can be run. To compile a Java program, you doesn't really need a main() method in your program.
Does public static void main have to be static?
Need of static in main() method: Since main() method is the entry point of any Java application, hence making the main() method as static is mandatory due to following reasons: The static main() method makes it very clear for the JVM to call it for launching the Java Application.
What is private static void in Java?
static means that the method is associated with the class, not a specific instance (object) of that class. This means that you can call a static method without creating an object of the class. void means that the method has no return value. If the method returned an int you would write int instead of void .
Is private static void main correct?
private static void main(String args[]) : No. The correct statement is public static void main(string args[]).
Can we override public static void main?
You cannot override static methods and since the public static void main() method is static we cannot override it.
Can we overload main method?
Yes, We can overload the main method in java but JVM only calls the original main method, it will never call our overloaded main method.
Can we have multiple main methods in Java?
Yes. While starting the application we mention the class name to be run. The JVM will look for the main method only in the class whose name you have mentioned.
Can abstract class have public static void main?
Yes, you can use the main() method in abstract class. The main() method is a static method so it is associated with Class, not with object or instance. The abstract is applicable to the object so there is no problem if it contains the main method.
Can we have private static class in Java?
Static nested classes do not have access to other members of the enclosing class. As a member of the OuterClass , a nested class can be declared private , public , protected , or package private. (Recall that outer classes can only be declared public or package private.)
Why we use public static void main?
The main() method is static so that JVM can invoke it without instantiating the class.
Can we execute program without main?
Yes, you can compile and execute without main method by using a static block.
Why do we use public static void main?
The main() method is static so that JVM can invoke it without instantiating the class. This also saves the unnecessary wastage of memory which would have been used by the object declared only for calling the main() method by the JVM.
Why should main method be static public and void in Java?
The main() method in Java must be declared public, static and void. If any of these are missing, the Java program will compile but a runtime error will be thrown.
What happens if we don't use static in main method?
You can write the main method in your program without the static modifier, the program gets compiled without compilation errors. But, at the time of execution JVM does not consider this new method (without static) as the entry point of the program.
Why main () method is public static and void in Java?
The main method in Java is public so that it's visible to every other class, even which are not part of its package. if it's not public JVM classes might not able to access it. 2. The main method is static in Java so that it can be called without creating any instance.
1. Public
It is an Access modifier, which specifies from where and who can access the method. Making the main () method public makes it globally available. It is made public so that JVM can invoke it from outside the class as it is not present in the current class.
2. Static
It is a keyword that is when associated with a method, making it a class-related method. The main () method is static so that JVM can invoke it without instantiating the class. This also saves the unnecessary wastage of memory which would have been used by the object declared only for calling the main () method by the JVM.
3. Void
It is a keyword and is used to specify that a method doesn’t return anything. As the main () method doesn’t return anything, its return type is void. As soon as the main () method terminates, the java program terminates too. Hence, it doesn’t make any sense to return from the main () method as JVM can’t do anything with the return value of it.
4. main
It is the name of the Java main method. It is the identifier that the JVM looks for as the starting point of the java program. It’s not a keyword.
What is public access modifier?
The Public access modifier can be associated with class, method, constructor, interface, etc. public can be accessed from any other class. The protected access modifier can be associated with variables, methods, and constructors, which are declared protected in a superclass can be accessed only by the subclasses in other package or any class within ...
What is a private access modifier in Java?
Java provides various access specifiers namely private, public and protected etc... The Private modifier restricts the access of members from outside the class. A class and interface cannot be public. The Public access modifier can be associated with class, method, constructor, interface, etc. public can be accessed from any other class.
Can you declare a method private?
Declaring the main method private or, protected. You can define the main method in your program without private, protected or, default (none) modifier, the program gets compiled without compilation errors. But, at the time of execution JVM does not consider this as the entry point of the program.
Does JVM consider void as entry point?
But, at the time of execution JVM does not consider this as the entry point of the program. It searches for the main method which is public, static, with return type void, and a String array as an argument.
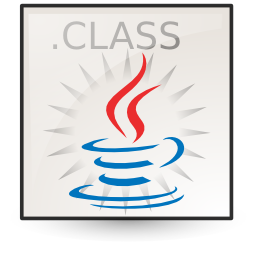