
Full Answer
How do you add items to a dictionary?
Adding an item to the dictionary is done by using a new index key and assigning a value to it: Example thisdict = { "brand": "Ford", "model": "Mustang", "year": 1964 thisdict["color"] = "red" print(thisdict) Try it Yourself » Related Pages
How can I add new keys to a dictionary?
you create a new keyvalue pair on a dictionary by assigning a value to that key. If the key doesn’t exist, it’s added and points to that value. If it exists, the current value it points to is overwritten. — R. Navega To add multiple keys simultaneously, use dict.update ():
How to append a list item in Python?
In Python, we can append an item to a list by using the append() method. This method takes an item as an argument and adds it to the end of the list. It’s important to note that the append() method only adds a single item to the list. If you want to add multiple items, then either use the extend() method or perform several calls of append().
How to append elements into a dictionary in Swift?
Swift: Append an element to an array in a dictionary value, creating the array/value if needed - Dictionary.Value+RangeReplaceableCollection.swift

How do you add an item to a dictionary in Python?
There is no add() , append() , or insert() method you can use to add an item to a dictionary in Python. Instead, you add an item to a dictionary by inserting a new index key into the dictionary, then assigning it a particular value.
How do I add an item to a dictionary in dictionary?
Appending element(s) to a dictionary To append an element to an existing dictionary, you have to use the dictionary name followed by square brackets with the key name and assign a value to it.
Can we add elements to dictionary?
We add a new element to the dictionary by using a new key as a subscript and assigning it a value.
Can you append to a dictionary value?
We can add / append new key-value pairs to a dictionary using update() function and [] operator. We can also append new values to existing values for a key or replace the values of existing keys using the same subscript operator and update() function.
How do I update a dictionary in Python?
Python Dictionary update() method updates the dictionary with the elements from another dictionary object or from an iterable of key/value pairs.Syntax: dict.update([other])Parameters: This method takes either a dictionary or an iterable object of key/value pairs (generally tuples) as parameters.More items...•
Can you put a list in a dictionary Python?
You can convert a Python list to a dictionary using the dict. fromkeys() method, a dictionary comprehension, or the zip() method. The zip() method is useful if you want to merge two lists into a dictionary.
How do I add items to a list in python?
How to add Elements to a List in Pythonappend() : append the element to the end of the list.insert() : inserts the element before the given index.extend() : extends the list by appending elements from the iterable.List Concatenation: We can use the + operator to concatenate multiple lists and create a new list.
How do you add a value to a key in python?
Add Values to Dictionary in PythonAssigning a value to a new key.Using dict. update() method to add multiple key-values.For Python 3.9+, use the merge operator ( | ).For Python 3.9+, use the update operator ( |= ).Creating a custom function.Using __setitem__() method (It is not recommended).
How do I add an item to an empty dictionary in python?
How to Add New Items to A Dictionary in Python. To add a key-value pair to a dictionary, use square bracket notation. First, specify the name of the dictionary. Then, in square brackets, create a key and assign it a value.
How do you add a key-value pair to a dictionary?
In Python, we can add multiple key-value pairs to an existing dictionary. This is achieved by using the update() method. This method takes an argument of type dict or any iterable that has the length of two - like ((key1, value1),) , and updates the dictionary with new key-value pairs.
How do you change a value in a dictionary?
Assign a new value to an existing key to change the value Use the format dict[key] = value to assign a new value to an existing key.
How do you access a dictionary value in Python?
To access a dictionary value in Python you have three options:Use the dictionary. values() method to get all the values.Use the square brackets [] to get a single value (unsafely).Use the dictionary. get() method to safely get a single value from a dictionary.
How do you add elements to an empty dictionary?
To create an empty dictionary, first create a variable name which will be the name of the dictionary. Then, assign the variable to an empty set of curly braces, {} . Another way of creating an empty dictionary is to use the dict() function without passing any arguments.
How do I merge two dictionaries in Python?
Python 3.9 has introduced the merge operator (|) in the dict class. Using the merge operator, we can combine dictionaries in a single line of code. We can also merge the dictionaries in-place by using the update operator (|=).
How do you add items to a dictionary quizlet?
We can add an item by simply using the key and re-assigning it. Also in a dict we can insert a key-value pair by simply creating the key and assigning the value.
Can we merge two dictionaries in Python?
In the latest update of python now we can use “|” operator to merge two dictionaries. It is a very convenient method to merge dictionaries.
How to add items to a dictionary?
We can add an item to a dictionary using the update () method. The update () method when invoked on a dictionary, takes a dictionary or an iterable object having key-value pairs as input and adds the items to the dictionary.
What is a dictionary in Python?
A dictionary in python is a data structure that stores data in the form of key-value pairs. The key-value pairs are also called items. The key-value pairs in each dictionary are separated by a colon “:” and each item in the dictionary is separated by a comma “,”. In this article, we will look at different ways to add an item to a dictionary in python.
How to add a key value to a dictionary?
If we have a dictionary named myDict and a key-value pair having values myKey and myValue, then we can add the key-value pair to the dictionary using the syntax myDict [ myKey ] = myValue. This can be done as follows.
What happens if the key of the item is added to the dictionary already exists in it?
Remember that if the key of the item which is being added to the dictionary already exists in it, the value for the key will be overwritten with a new value. This can be seen in the following example.
What happens if a key already exists in the dictionary?
If the key already exists in the dictionary, the value associated with it is overwritten with the new value. This can be seen in the following example.
Can we give a new dictionary as an input to the update method?
We can give a new dictionary as an input to the update () method and add the items to a given dictionary as follows.
Adding Items
Adding an item to the dictionary is done by using a new index key and assigning a value to it:
Update Dictionary
The update () method will update the dictionary with the items from a given argument. If the item does not exist, the item will be added.
How to add items to a dictionary in Python?
To add an item to a Python dictionary, you should assign a value to a new index key in your dictionary. Unlike lists and tuples, there is no add (), insert (), or append () method that you can use to add items to your data structure. Instead, you have to create a new index key, which will then be used to store the value you want to store in your ...
What is a dictionary in Python?
Python Dictionary: A Refresher. The dictionary data structure allows you to map keys to values. This is useful because keys act as a label for a value, which means that when you want to access a particular value, all you have to do is reference the key name for the value you want to retrieve.
Why isn't the dictionary ordered?
This is because data stored in a dictionary, unlike data stored in a list, does not have any particular order.
Can the same notation be used to update a value in a dictionary?
The same notation can be used to update a value in a dictionary. Suppose we have just baked a new batch of 10 cinnamon scones. We could update our dictionary to reflect this using the following line of code:
When adding new items to a dictionary, do you want to check?
Before adding new items to a dictionary, you may want to check whether a value already exists at the given key.
What is the in keyword?
The in keyword can be used to check whether a key already exists. This will return TRUE if the key exists and FALSE if it does not, so it can be used in an if statement:
Add an Item to a Python Dictionary
The easiest way to add an item to a Python dictionary is simply to assign a value to a new key. Python dictionaries don’t have a method by which to append a new key:value pair. Because of this, direct assignment is the primary way of adding new items to a dictionary.
Update an Item in a Python Dictionary
Python dictionaries require their keys to be unique. Because of this, when we try to add a key:value pair to a dictionary where the key already exists, Python updates the dictionary. This may not be immediately clear, especially since Python doesn’t throw an error.
Append Multiple Items to a Python Dictionary with a For Loop
There may be times that you want to turn two lists into a Python dictionary. This can be helpful when you get data from different sources and want to combine lists into a dictionary data structure.
Add Multiple Items to a Python Dictionary with Zip
The Python zip () function allows us to iterate over two iterables sequentially. This saves us having to use an awkward range () function to access the indices of items in the lists.
Conclusion
In this tutorial, you learned how to use Python to add items to a dictionary. You learned how to do this using direct assignment, which can be used to add new items or update existing items. You then also learned how to add multiple items to a dictionary using both for loops and the zip function.
What is dictionary in Python?
Dictionary in Python is an unordered collection of data values, used to store data values like a map, which unlike other Data Types that hold only single value as an element, Dictionary holds key:value pair. Key value is provided in the dictionary to make it more optimized. Each key-value pair in a Dictionary is separated by a colon : , ...
Why is key value provided in the dictionary?
Key value is provided in the dictionary to make it more optimized. Each key-value pair in a Dictionary is separated by a colon : , whereas each key is separated by a ‘comma’.
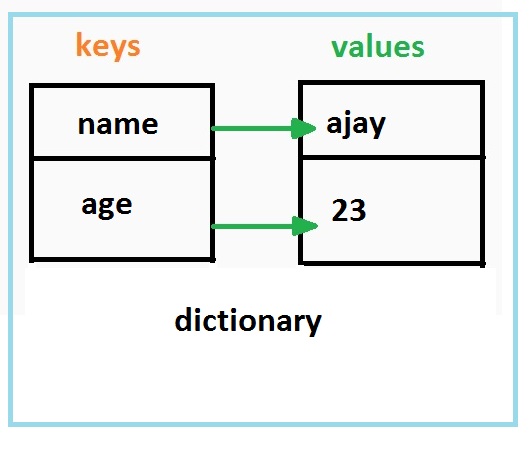