Converting a List of Dictionaries to a Single Dictionary
- dict.update () We can convert a list of dictionaries to a single dictionary using dict.update (). ...
- Dictionary comprehension A dictionary comprehension consists of brackets {} containing two expressions separated with a colon followed by a for clause, then zero or more for or if clauses. ...
- Collections.ChainMap By using collections.ChainMap (), we can convert a list of dictionaries to a single dictionary. ...
How to replace single quotes from a list in Python?
To remove quotes from a string using Python, the easiest way is to use the Python replace () function. You can remove single and double quotes using replace (). string_single_quotes = "This' is' a' string' with' quotes."
How to delete an item from a dictionary in Python?
Remove an item from a dictionary in Python (clear, pop, popitem, del)
- Remove all items: clear ()
- Remove an item by a key and return a value: pop ()
- Remove an item and return a key and value: popitem ()
- Remove an item by a key: del
- Remove items that meet the condition: Dictionary comprehensions
How can I add new keys to a dictionary?
you create a new keyvalue pair on a dictionary by assigning a value to that key. If the key doesn’t exist, it’s added and points to that value. If it exists, the current value it points to is overwritten. — R. Navega To add multiple keys simultaneously, use dict.update ():
How to convert string to list of words in Python?
Method 1: Convert String to list of strings in Python
- Syntax:
- Parameters:
- Output: The split method by default takes whitespace as delimiter and separates the words of the string from by the whitespace and converts them into a list.

Can you convert a list into a dictionary?
To convert a list to dictionary, we can use list comprehension and make a key:value pair of consecutive elements. Finally, typecase the list to dict type.
How do I convert a list to a dictionary in Python w3schools?
The zip() function is an in-built function that takes iterators (can be zero or more), aggregates and combines them, and returns them as an iterator of tuples and the dict() function creates a new dictionary.
Can I use a list as a dictionary key?
Second, a dictionary key must be of a type that is immutable. For example, you can use an integer, float, string, or Boolean as a dictionary key. However, neither a list nor another dictionary can serve as a dictionary key, because lists and dictionaries are mutable.
How do I extract a dictionary from a list in Python?
Here are 3 approaches to extract dictionary values as a list in Python:(1) Using a list() function: my_list = list(my_dict.values())(2) Using a List Comprehension: my_list = [i for i in my_dict.values()](3) Using For Loop: my_list = [] for i in my_dict.values(): my_list.append(i)
What is the dict () function in Python?
Python dict() Function The dict() function creates a dictionary. A dictionary is a collection which is unordered, changeable and indexed. Read more about dictionaries in the chapter: Python Dictionaries.
What does item () do in Python?
In Python Dictionary, items() method is used to return the list with all dictionary keys with values. Parameters: This method takes no parameters. Returns: A view object that displays a list of a given dictionary's (key, value) tuple pair.
Can you store a list in a dictionary Python?
Note that the restriction with keys in Python dictionary is only immutable data types can be used as keys, which means we cannot use a dictionary of list as a key .
Can we pass list as key in dictionary Python?
We can use integer, string, tuples as dictionary keys but cannot use list as a key of it .
Can a list be a value in a dictionary Python?
It definitely can have a list and any object as value but the dictionary cannot have a list as key because the list is mutable data structure and keys cannot be mutable else of what use are they.
How do you create a dictionary in Python?
How to Create An Empty Dictionary in Python. To create an empty dictionary, first create a variable name which will be the name of the dictionary. Then, assign the variable to an empty set of curly braces, {} . Another way of creating an empty dictionary is to use the dict() function without passing any arguments.
How do I extract a list?
6 Easy Ways to Extract Elements From Python ListsMethod 1: Use Slicing.Method 2: Use List Index.Method 3: Use List Comprehension.Method 4: Use List Comprehension with condition.Method 5: Use enumerate()Method 6: Use NumPy array()
How do you print an item from a list in Python?
Using the * symbol to print a list in Python. To print the contents of a list in a single line with space, * or splat operator is one way to go. It passes all of the contents of a list to a function. We can print all elements in new lines or separated by space and to do that, we use sep=”\n” or sep=”, ” respectively.
How do I convert a nested list to a dictionary?
Converting a Nested List to a Dictionary Using Dictionary Comprehension. We can convert a nested list to a dictionary by using dictionary comprehension. It will iterate through the list. It will take the item at index 0 as key and index 1 as value.
How do you create a dictionary from a list of tuples?
Use the dict class to convert a list of tuples to a dictionary, e.g. my_dict = dict(list_of_tuples) . The dict class can be passed a list of tuples and returns a new dictionary. Copied!
What is the use of all () any () CMP () and sorted () in dictionary?
Built-in functions like all() , any() , len() , cmp() , sorted() , etc. are commonly used with dictionaries to perform different tasks. Return True if all keys of the dictionary are True (or if the dictionary is empty). Return True if any key of the dictionary is true.
How do you create a dictionary in Python?
To create a Python dictionary, we pass a sequence of items (entries) inside curly braces {} and separate them using a comma ( , ). Each entry consists of a key and a value, also known as a key-value pair. Note: The values can belong to any data type and they can repeat, but the keys must remain unique.
How to convert a list to a dictionary in Python?
Mainly there are two methods for converting a list to a dictionary in Python one is dictionary comprehension and the other one is using the Python zip () method. We are going to discuss them one by one.
What is dictionary in Python?
What is a dictionary in Python? A dictionary in Python is a special type of data structure that is used to store the data in the key-value pair format. A Python dictionary has two main components: key & value where the key must be a single entity but value can be a multi-value entity like list, tuple, etc. The key and its value are separated by ...
What is a list in Python?
A list in Python is a linear data structure used to store a collection of values that may be of the same type or different types. Each item inside a list is separated by a comma (,).
What is the Python dict function?
And as the last step, we do the typecasting using the Python dict () function which creates and returns a Python dictionary from the zipped values returned by the zip () function.
How to separate key and value in Python?
The key and its value are separated by a colon (:) and each item (a key-value pair) is separated by a comma (,) just like the Python list. A dictionary is enclosed in the curly brackets {}. Like the Python list, the Python dictionary is also mutable.
What is a mutable list in Python?
A Python list is mutable, meaning we can change or modify its contents after its creation inside the Python program. A Python list stores the elements in an ordered manner and we can access any element in the list directly by its index.
What is dictionary in Python?
A dictionary, when invoked, makes items more callable in Python. In essence, it lets you call any item by its key. So you can access its matching value. That said, converting a list to a dictionary in Python is helpful in many real-life cases. Ultimately, a dictionary also confers speed on web requests.
What does dict (zip)) do?
Unlike the looping method that you used earlier, the dict (zip ()) function ignores any item without a pair.
What does zip_longest do in Python?
When you use the zip_longest module, Python assigns a null value to missing data. To use this module, first import it into your code:
Can you use Python without a for loop?
To achieve this without using the for loop, you can also use the built-in dict and zip functions of Python:
Is Python code complex?
The code may look complex at first, but it actually isn't. You're only telling Python to pick a pair each time it iterates through your list. Then it should make every item on the left of each pair the key to the one on its right side, which is its value.
Can you convert a list to a dictionary?
Provided that a list is symmetric (containing equal potential key-value pairs), you can convert it to a dictionary using the for loop . To do this, place your loop in a dictionary comprehension: The code may look complex at first, but it actually isn't.
Can you combine two lists into one dictionary?
Learn to convert lists to dictionaries. Converting a list to a dictionary is helpful if you want to create a key-value pair from that list. And if there are two lists , you can combine them into one dictionary as well. A dictionary, however, gives you more control over your data.
Method1-Using dictionary comprehension
This is one of the ways to convert a list to a dictionary in python. Let us understand what dictionary comprehension is. Dictionary comprehension is one of the easiest ways to create a dictionary. Using Dictionary comprehension we can make our dictionary by writing only a single line of code.
Method-2 Using zip () function
The zip () function is used to aggregate or zip or combine the two values together. zip () function returns a zip object so we can easily convert this zip object to the tuple, list, or dictionary.
First case: When both the list are of the same length
Explanation: Here we see that both the list are of the same length. We can see that the first zip () function returns a zip object that we print in the above example and then we typecast this object into the dictionary to get the desired result.
Second case: When both the list are of the different length
Explanation: Here we see that our first list is of length 5 while the second list is of length 4 and in the final result, we get only 4 key-value pairs. So it can be concluded that the list with the smallest length will decide the number of key-value pairs in output. While another part is the same i.e.
Method-3 Using dict () constructor
dict () constructor is also used to create a dictionary in python.This method comes into use when we have list of tuples. List of tuples means tuples inside the list.
How to make a list of lists in Python?
How to Convert a List of List to a Dictionary in Python? 1 Databases: List of list is table where the inner lists are the database rows and you want to assign each row to a primary key in a new dictionary. 2 Spreadsheet: List of list is two-dimensional spreadsheet data and you want to assign each row to a key (=row name). 3 Data Analytics: You’ve got a two-dimensional matrix (= NumPy array) that’s initially represented as a list of list and you want to obtain a dictionary to ease data access.
What is a list of lists?
Databases: List of list is table where the inner lists are the database rows and you want to assign each row to a primary key in a new dictionary.
How much does a Python developer make an hour?
The average Python freelance developer earns $51 per hour in the US.
Can you use dictionary elements in a for loop?
Of course, there’s no need to get fancy here. You can also use a regular for loop and define the dictionary elements one by one within a simple for loop. Here’s the alternative code:
How to use dict.items in Python?
In this method, we will be using the dict.items () function of dictionary class to convert a dictionary to a list. The dict.items () function is used to iterate over the key: value pairs of the Python dictionary. By default, the key: value pairs are stored in the form of a Python tuple in the iterator returned by the dict.items () function. We can pass this returned iterator to the list () function which will finally give us a Python list of tuples containing the key: value pairs of the given Python dictionary. Let’s get into the actual Python code to implement this method.
How to initialize a list in Python?
The list comprehension method is the most widely used and compact way to initialize a Python list. We can also provide the list elements in the same single line using a for loop. Here we will fetch the key: value pairs of the given Python dictionary using a for loop and dict.items () function. Then we will initialize the Python list with the fetched key: value pairs of the given Python dictionary. Let’s see how we can use this method to convert a given Python dictionary to a Python list.
What is zip in Python?
The zip () function in Python is used to combine two iterator objects by zipping their values.
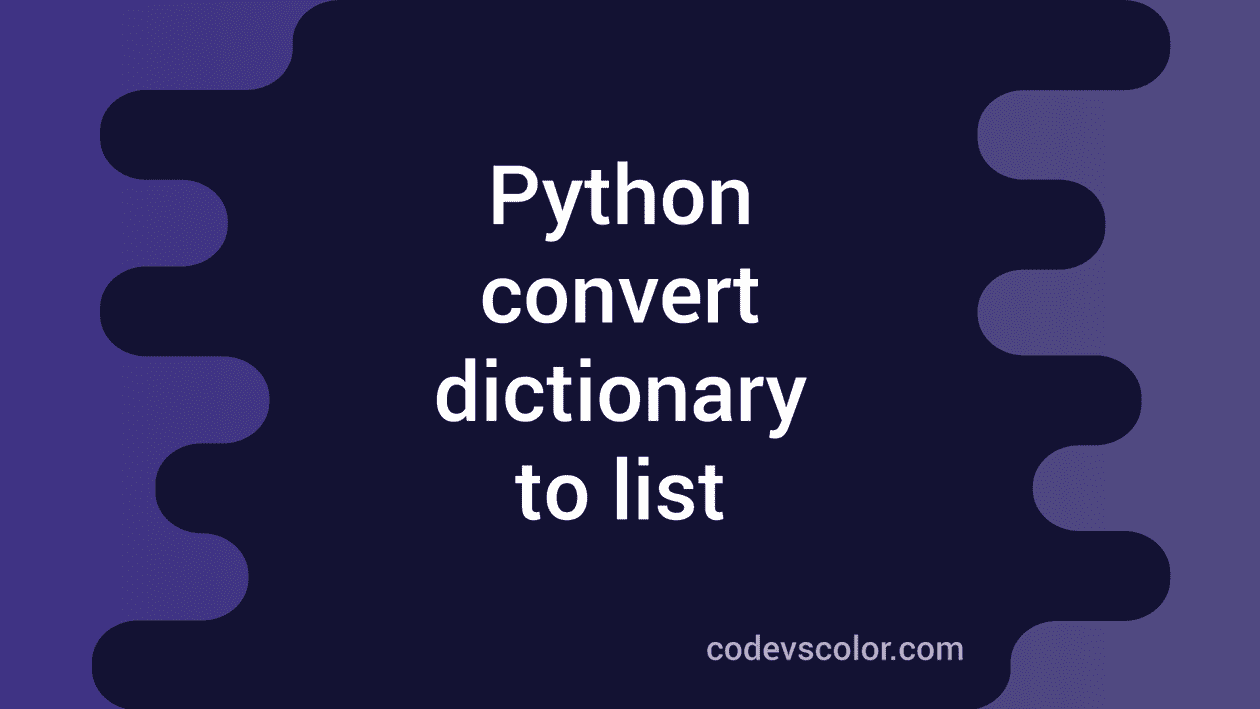