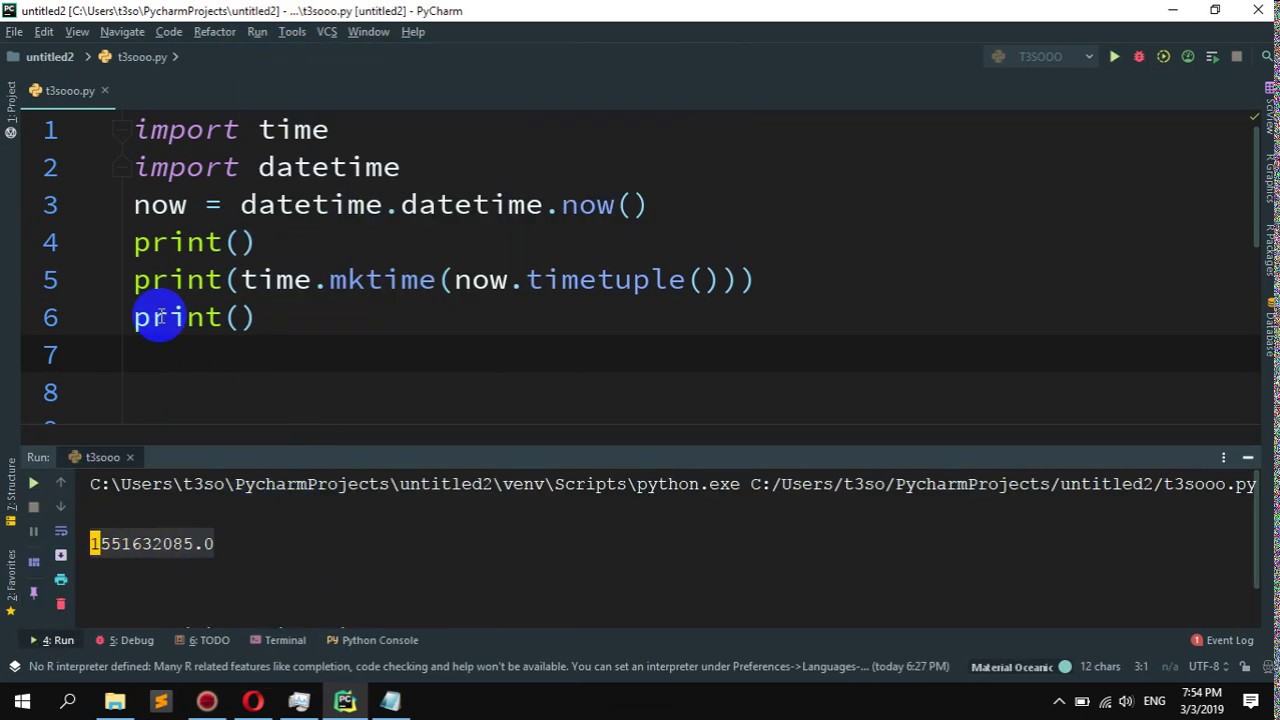
How to convert datetime to Seconds in Python
- Import datetime module Python datetime module provides various functions to create and manipulate the date and time. Use the from datetime import datetime statement to import a datetime class from a datetime module.
- Subtract the input datetime from the epoch time To convert a datetime to seconds, subtracts the input datetime from the epoch time. ...
- Use the timestamp () method. ...
What is the return value of datetime?
What is the value returned in a timedelta?
Is the starting date in UTC?
Does timezone affect results?
Do you need tzinfo to start datetime?
See 2 more
About this website

How do I convert time to seconds in Python?
Python 3 provides datetime. timestamp() method to easily convert the datetime object to seconds. This method will only be useful if you need the number of seconds from 1970-01-01 UTC. It returns a float value representing even fractions of a second.
How do I convert datetime to minutes in Python?
“convert datetime to minutes” Code Answerseconds = duration. total_seconds()hours = seconds // 3600.minutes = (seconds % 3600) // 60.seconds = seconds % 60.
How do I convert a datetime to a number in Python?
In this method, we are using strftime() function of datetime class which converts it into the string which can be converted to an integer using the int() function. Returns : It returns the string representation of the date or time object. Code: Python3.
How do you change time format in Python?
Use datetime. strftime(format) to convert a datetime object into a string as per the corresponding format . The format codes are standard directives for mentioning in which format you want to represent datetime. For example, the %d-%m-%Y %H:%M:%S codes convert date to dd-mm-yyyy hh:mm:ss format.
How do I convert datetime to seconds?
To convert a datetime to seconds, subtracts the input datetime from the epoch time. For Python, the epoch time starts at 00:00:00 UTC on 1 January 1970. Subtraction gives you the timedelta object. Use the total_seconds() method of a timedelta object to get the number of seconds since the epoch.
How do you convert timestamps to seconds?
First divide the number of seconds by 86400 (the number of seconds in a day). Once you have done this, you will get the time value as a number. To display the time value as a valid time (i.e. in minutes and seconds) format the cell with custom formatting.
What time time () returns in Python?
time() The time() function returns the number of seconds passed since epoch. For Unix system, January 1, 1970, 00:00:00 at UTC is epoch (the point where time begins).
How do you convert timestamps to milliseconds?
You can get the time in seconds using time. time function(as a floating point value). To convert it to milliseconds, you need to multiply it with 1000 and round it off.
How do you convert a number into a timestamp?
To convert a NUMBER to a TIMESTAMP you can use an expression like TO_TIMESTAMP(TO_CHAR(...)) . To compute the number of seconds between two timestamps, one solution is to cast both to dates, and substract them : you will get a (decimal) result in days, which can be then converted to seconds.
How do I change datetime in Python?
“how to change the year in datetime python” Code Answerfrom datetime import datetime.date = datetime. strptime('26 Sep 2012', '%d %b %Y')newdate = date. replace(hour=11, minute=59)
What is Python time format?
Python strftime() functionDirectiveMeaningOutput Format%HHour (24-hour clock) as a zero added decimal number.00, 01, …, 23%-HHour (24-hour clock) as a decimal number.0, 1, …, 23%IHour (12-hour clock) as a zero added decimal number.01, 02, …, 12%-IHour (12-hour clock) as a decimal number.1, 2, … 1224 more rows•Mar 15, 2021
What is the format of datetime?
For example, the "d" standard format string indicates that a date and time value is to be displayed using a short date pattern. For the invariant culture, this pattern is "MM/dd/yyyy". For the fr-FR culture, it is "dd/MM/yyyy". For the ja-JP culture, it is "yyyy/MM/dd".
How do I get only hours and minutes in Python?
To get the current time in particular, you can use the strftime() method and pass into it the string ”%H:%M:%S” representing hours, minutes, and seconds.
What time time () returns in Python?
time() The time() function returns the number of seconds passed since epoch. For Unix system, January 1, 1970, 00:00:00 at UTC is epoch (the point where time begins).
How do you count minutes in Python?
To calculate the value of minutes we need to first divide the total number of seconds by 3600 and take the remainder. Now to calculate the value of minutes from the above result we will again use the floor operator. A minute has sixty seconds hence we floor the seconds value with 60.
How do I convert a date to minutes in Excel?
Re: convert a custom Date Format to Minutes Option 1: change the number format to [m]. You'll see the number of minutes, but the underlying value will still be a date. Option 2: in another cell, enter the formula =E2*1440 and format the cell with the formula as Number with 0 decimals.
How to convert a datetime object to seconds? - Studytonight
In this article, we will learn to convert datetime object to seconds in Python.We will use some built-in modules available and some custom codes as well to see them working.
python - Convert datetime.time to seconds - Stack Overflow
You need to convert your datetime.time object into a datetime.timedelta to be able to use total_seconds() function.. It will return a float rather than an int as asked in the question but you can easily cast it. >>> from datetime import datetime, date, time, timedelta >>> timeobj = time(12, 45) >>> t = datetime.combine(date.min, timeobj) - datetime.min >>> isinstance(t, timedelta) # True >>> t ...
how to get the Seconds of the time using python - Stack Overflow
I believe the difference between two time objects returns a timedelta object. This object has a .total_seconds() method. You'll need to factor these into minutes+seconds yourself: minutes = total_secs % 60 seconds = total_secs - (minutes * 60)
Easily Convert Python Timedelta to Seconds - Python Pool
Output: Printing delta object 196 days, 18:50:50.000020 Number of days 196 Number of seconds 67850 Number of microseconds 20 Traceback (most recent call last): File "file4.py", line 13, in
Get Seconds from timestamp (date) in pandas python
dt.second is the inbuilt method to get seconds from timestamp (date) in Pandas Python. Let’s see how to. Get the seconds from timestamp (date) in pandas python; First lets create the dataframe. import pandas as pd import numpy as np import datetime date1 = pd.Series(pd.date_range('2012-1-1 11:20:00', periods=7, freq='s')) df = pd.DataFrame(dict(date_given=date1)) print(df)
Example 1: Convert datetime Object to Seconds
This example uses the strptime () function and the second attribute to extract the seconds from a datetime object:
Example 2: Convert datetime Object to Minutes
Example 2 uses the strptime () method to return minutes from a date and time object:
Example 3: Convert datetime Object to Hours
This example uses the strptime () function once again. This time we are converting our datetime object to hours.
Video, Further Resources & Summary
Do you need more explanations on the conversion of datetime objects to seconds, minutes, and hours in Python? In this case, you should definitely have a look at the YouTube video of the PyLenin YouTube channel below.
How to work with date in Python?
In Python, we can work on Date functions by importing a built-in module datetime available in Python. We have date objects to work with dates. This datetime module contains dates in the form of year, month, day, hour, minute, second, and microsecond. The datetime module has many methods to return information about the date object. It requires date, month, and year values to compute the function. Date and time functions are compared like mathematical expressions between various numbers.
What does datetime.total_seconds do?
3. datetime.total_seconds () returns the total number of seconds.
What is the Python 3 timegm?
Python 3 provides a standard library called calendar which has calendar.timegm () method to easily convert the datetime object to seconds. This method converts a datetime to seconds since Epoch i.e. 1970-01-01 UTC. As the above timestamp () method returns a float value, this method strips off the fractions of a second.
Is the starting date in UTC?
The starting date is usually specified in UTC, so for proper results the datetime you feed into this formula should be in UTC as well. If your datetime isn't in UTC already, you will need to convert it before you use it.
How to convert datetime to date in Python?
In this article, we are going to see how to convert DateTime to date in Python. For this, we will use the strptime () method. This method is used to create a DateTime object from a string. Then we will extract the date from the DateTime object using the date () function.
What is the format of datetime_str?
In this example, We have created a datetime_str which is “100201095407”, and its format is “%d%m%y%H%M%S”.
What is the default format for strftime?
format: This will be str, but the default is None. The strftime to parse time, eg “%d/%m/%Y”, note that “%f” will parse all the way up to nanoseconds.
What is the return value of datetime?
The return value will be a float representing even fractions of a second. If the datetime is timezone naive (as in the example above), it will be assumed that the datetime object represents the local time, i.e. It will be the number of seconds from current time at your location to 1970-01-01 UTC.
What is the value returned in a timedelta?
The value returned is a timedelta object from which you can use the member function total_seconds to get the value in seconds.
Is the starting date in UTC?
The starting date is usually specified in UTC, so for proper results the datetime you feed into this formula should be in UTC as well. If your datetime isn't in UTC already, you'll need to convert it before you use it, or attach a tzinfo class that has the proper offset.
Does timezone affect results?
Note that different timezones have impact on results, e.g. my current TZ/DST returns:
Do you need tzinfo to start datetime?
As noted in the comments, if you have a tzinfo attached to your datetime then you'll need one on the starting date as well or the subtraction will fail; for the example above I would add tzinfo=pytz.utc if using Python 2 or tzinfo=timezone.utc if using Python 3.
