
Java Remove Character from String
- replace (char oldChar, char newChar): This method returns a new string where oldChar is replaced with newChar. ...
- replace (CharSequence target, CharSequence replacement): This method replaces the target substring with the replacement substring. ...
- replaceFirst (String regex, String replacement): This method replaces the first match of the regex with the replacement string. ...
How to remove the last character from a string?
To remove the first and last character, we use the following steps:
- Split (break) the String based on the space.
- For each word, run a loop form the first to last letter.
- Identify the first and last character of each word.
- Now, delete the first and last character of each word.
How to extract characters from a string?
How to extract characters from a string. To extract a substring from a string in PHP, use the PHP substr() function. The substr() method does not change an original string. If we want to extract characters from the end of the string, use a negative start number. PHP substr. PHP substr() is a built-in string function that returns a part of a string.
How to remove character from string using JavaScript?
Using split () method. Another method JavaScript provides for removing characters is split () method which is used along with join () method. Firstly we use split () method to remove our desired character and it returns an array of strings. After that join () method is used to join the string, demonstrated below by an example:
How do you extract characters from a string?
- The startIndex of the first character of a PowerShell string will always be zero (0)
- To determine the startIndex of a substring, add 1 to the IndexOf command result
- Finally, the length of the PowerShell substring you want to extract is the total number (starting from 1) and counting from the first character of the substring to the last ...

How do I remove all letters from a string?
Example of removing special characters using replaceAll() methodpublic class RemoveSpecialCharacterExample1.{public static void main(String args[]){String str= "This#string%contains^special*characters&.";str = str.replaceAll("[^a-zA-Z0-9]", " ");System.out.println(str);}More items...
How do I remove multiple characters from a string in Java?
replaceAll() is used when we want to replace all the specified characters' occurrences. We can use regular expressions to specify the character that we want to be replaced. This method takes two arguments, the first is the regular expression pattern, and the second is the character we want to place.
How do I remove multiple characters from a string?
To remove multiple characters from a string we can easily use the function str. replace and pass a parameter multiple characters. The String class (Str) provides a method to replace(old_str, new_str) to replace the sub-strings in a string. It replaces all the elements of the old sub-string with the new sub-string.
How do you clear a string in Java?
delete() is an inbuilt method in Java which is used to remove or delete the characters in a substring of this sequence. The substring starts at a specified index start_point and extends to the character at the index end_point.
How do you replace multiple occurrences of a string in Java?
You can replace all occurrence of a single character, or a substring of a given String in Java using the replaceAll() method of java. lang. String class. This method also allows you to specify the target substring using the regular expression, which means you can use this to remove all white space from String.
What is difference between replace and replaceAll in Java?
The difference between replace() and replaceAll() method is that the replace() method replaces all the occurrences of old char with new char while replaceAll() method replaces all the occurrences of old string with the new string.
How do you replace a character in a string in Java without using replace method?
To replace a character in a String, without using the replace() method, try the below logic. Let's say the following is our string. int pos = 7; char rep = 'p'; String res = str. substring(0, pos) + rep + str.
How do I replace two characters in a string?
Python: Replace multiple characters in a string using the replace() In Python, the String class (Str) provides a method replace(old, new) to replace the sub-strings in a string. It replaces all the occurrences of the old sub-string with the new sub-string.
What does replaceAll do in Java?
Java replaceAll () method of String class replaces each substring of this string that matches the given regular expression with the replacement.
What is a character that is not an alphabet or numeric character called?
A character which is not an alphabet or numeric character is called a special character . We should remove all the special characters from the string so that we can read the string clearly and fluently. Special characters are not readable, so it would be good to remove them before reading.
What is the removeAll method?
In the following example, the removeAll () method removes all the special characters from the string and puts a space in place of them.
What does "removing from strings" mean in Java?
Also, strings in Java are immutable so when people talk about removing from strings or getting substrings of strings, they really mean “create a new string that has the result I want”.
What is the first argument in a string?
The first argument is the full string. The second is the character you want to remove. The third is the string you want to put in it’s place. This can be an empty string (not null) which has the effect of totally removing the offending character.
What is Google's technical interview process?
The process is designed to identify a candidate’s technical and non-technical skills, level of expertise, and potential to be a cultural fit. Candidates often have to wait between successive interview stages
Can you remove characters from a string in Java?
Also, Java by design has made String s immutable. Which means that a String after creation cannot be modified. Hence, you cannot remove characters from a String just like that or how you could do it with an array.
Can you use char array without reflection?
It's impossible. Without involving reflection, you'll end up using any of String.charAt (int), String.indexOf (char), or String.toCharArray () among others.
What Are Alphanumeric and Non-alphanumeric Characters?
Alpha stands for alphabets, and numeric stands for a number. So, alphabets and numbers are alphanumeric characters, and the rest are non-alphanumeric.
How to Remove Non-alphanumeric Characters in Java?
To remove non-alphanumeric characters in a given string in Java, we have three methods; let’s see them one by one.
Are You Ready to Nail Your Next Coding Interview?
Whether you’re a Coding Engineer gunning for Software Developer or Software Engineer roles, or you’re targeting management positions at top companies, IK offers courses specifically designed for your needs to help you with your technical interview preparation!
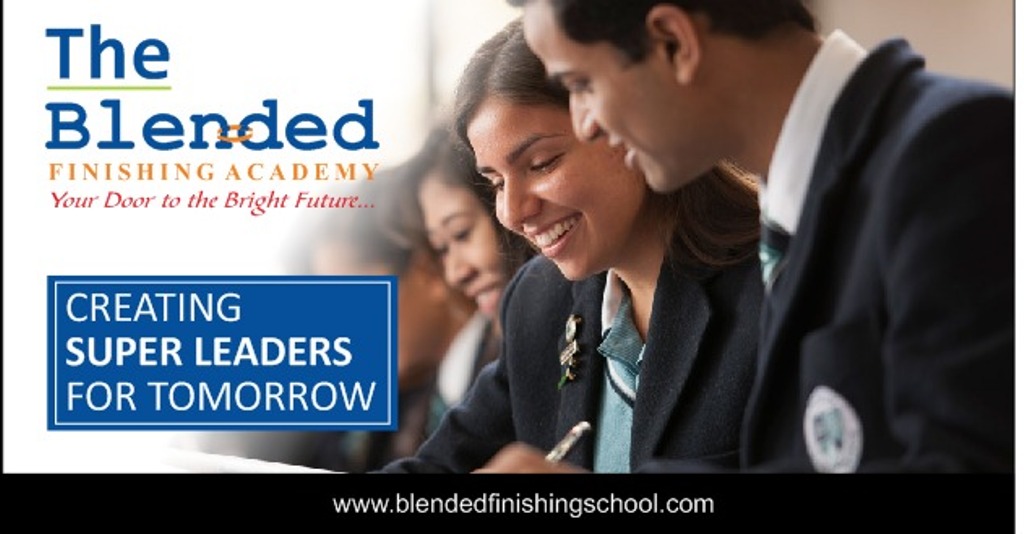