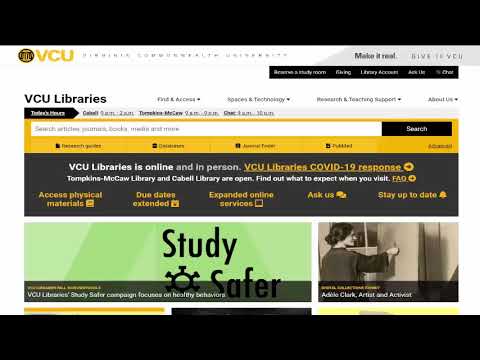
MySQL MySQLi Database To search exact word from string, use the below syntax − select *from yourTableName where yourColumnName regexp ' (^| [ [:space:]])yourWord ([ [:space:]]|$)';
How do I use MySQL_Query to search for a specific word?
mysql_query ("SELECT * FROM products WHERE product_name = '".$search."'"); If you are looking to match EXACT words don't use LIKE. EDIT: That clears things up a bit then. Just add a space after the search term. Or even add the hyphen (-) if that is always in the search term. mysql_query ("SELECT * FROM products WHERE product_name LIKE '".$search."
How to search for a string in all fields in MySQL?
How to search for a string in all fields of every table in a MySQL database 1) Select the desired database 2) Go to the Search form 3) Fill in your search criteria 4) Press “Go” and browse the results Summary
How to search text by user in mysql table?
The text by user will be searched in specified field of the MySql Table and the result containing all rows that match one or more keywords will be displayed. 1. Setup MySql Table To implement this tutorial you require a MySql Table. In this example I have created a very simple Table named 'table1' with only one Field named 'field1'. Task for You!
How do I search the contents of a MySQL database?
If you are using MySQL Workbench, right click on the database schema and select "Search Table Data..." then fill out the form. This will search the entire database. When (or if) the results come up, click on the arrow to expand. It will show the schema, table, primary key data, column name and the actual data that matches your search.

How do I find a specific word in MySQL?
LOCATE() function MySQL LOCATE() returns the position of the first occurrence of a string within a string. Both of these strings are passed as arguments. An optional argument may be used to specify from which position of the string (i.e. string to be searched) searching will start.
How do I search for a string in MySQL?
Steps: Select the database you need to search in from the left panel of GUI. Export > Export Database as SQL. In Table Tools window select "FIND TEXT" tab. Provide your string to search and click "FIND". It will list all the tables contains our string. Select the row with higher relevance %.More items...•
Does MySQL have full text search?
MySQL has support for full-text indexing and searching: A full-text index in MySQL is an index of type FULLTEXT . Full-text indexes can be used only with InnoDB or MyISAM tables, and can be created only for CHAR , VARCHAR , or TEXT columns.
How do I search a MySQL database?
Find data across a MySQL connection by using the text search feature on any number of tables and schemas. From the schema tree, select the tables, schemas, or both to search and then right-click the highlighted items and click Search Data Table from the context menu.
How do you check if a string contains a substring in MySQL?
MySQL query string contains using INSTR INSTR(str, substr) function returns the index of the first occurrence of the substring passed in as parameters. Here str is the string passed in as the first argument, and substr is the substring passed in as the second argument.
What is locate in MySQL?
LOCATE() function in MySQL is used for finding the location of a substring in a string. It will return the location of the first occurrence of the substring in the string. If the substring is not present in the string then it will return 0.
How do I do a full-text search?
Go to any cluster and select the “Search” tab to do so. From there, you can click on “Create Search Index” to launch the process. Once the index is created, you can use the $search operator to perform full-text searches.
What is SQL full-text search?
Full-text search refers to the functionality in SQL Server that supports full-text queries against character-based data. These types of queries can include words and phrases as well as multiple forms of a word or phrase.
How do I create a full-text search index?
Create FULLTEXT index using ALTER TABLE statementFirst, specify the name of the table that you want to create the index after the ALTER TABLE keywords.Second, use the ADD FULLTEXT clause to define the FULLTEXT index for one or more columns of the table.
How do I find a field in a database?
Select the Object search command:In the Search text field, enter the text that needs to be searched (e.g. a variable name)From the Database drop-down menu, select the database to search in.In the Objects drop-down list, select the object types to search in, or leave them all checked.More items...•
How do I search a SQL database?
SQL Server Management Studio Object Explorer browse to the database you want to search through. write the name (full or partial) of the database object in the Search text box. press Enter to start the search process.
How do I search for a string in a SQL Server database?
This will search every column of every table in a specific database. Create the stored procedure on the database that you want to search in....Different ways to search for SQL Server database objects.SQL Server Find Anything in Object Explorer in SSMS.Search text with wildcards.
How do you check if a column contains a value in MySQL?
$query = "SELECT * FROM my_table WHERE categories LIKE '%2%'"; Instead you should consider the find_in_set mysql function which expects a comma separated list for the value. Show activity on this post. $query = "SELECT * FROM my_table WHERE categories LIKE '%2%'"; $rows = mysql_query($query);
Which operator in MySQL is used to check string contains specific characters?
Check if String Contains Certain Data in MySQL Using the LIKE Operator in MySQL. Another alternative to find the existence of a string in your data is to use LIKE . This operator is used along with the WHERE clause to look for a particular string.
How do I write a like query in MySQL?
The MySQL LIKE Operator The LIKE operator is used in a WHERE clause to search for a specified pattern in a column. There are two wildcards often used in conjunction with the LIKE operator: The percent sign (%) represents zero, one, or multiple characters. The underscore sign (_) represents one, single character.
What is full text search in MySQL?
Full-Text Search in MySQL server lets users run full-text queries against character-based data in MySQL tables. You must create a full-text index on the table before you run full-text queries on a table. The full-text index can include one or more character-based columns in the table.
What is stopwords in MySQL?
Certain common words (stopwords) are omitted from the search index and do not match if present in the search string. MySQL can perform boolean full-text searches using the IN BOOLEAN MODE modifier. With this modifier, certain characters have special meaning at the beginning or end of words in the search string.
How does boolean search work?
A boolean search interprets the search string using the rules of a special query language. The string contains the words to search for. It can also contain operators that specify requirements such that a word must be present or absent in matching rows, or that it should be weighted higher or lower than usual. Certain common words (stopwords) are omitted from the search index and do not match if present in the search string. MySQL can perform boolean full-text searches using the IN BOOLEAN MODE modifier. With this modifier, certain characters have special meaning at the beginning or end of words in the search string.
What is an asterisk in a word?
The asterisk serves as the truncation (or wildcard) operator. Unlike the other operators, it is appended to the word to be affected. Words match if they begin with the word preceding the * operator.
How many types of full text searches are there?
There are three types of full-text searches :
When is match used in a where clause?
When MATCH () is used in a WHERE clause, as in the example shown earlier, the rows returned are automatically sorted with the highest relevance first.
Can Boolean queries work without fulltext?
Boolean queries against a MyISAM search index can work even without a FULLTEXT index.
What this article will cover?
In this tutorial I’ll illustrate how to implement the multiple keyword search technique. The focus is on searching one or more keywords or even full sentence or long text specified by a user in search text box.
1. Setup MySql Table
To implement this tutorial you require a MySql Table. In this example I have created a very simple Table named 'table1' with only one Field named 'field1'.
2. Create HTML Form
The next step is to create HTML form. The HTML Form, in this tutorial, is very simple form. It has a label, a text box (also called INPUT box), and a search button. This form allows user to type one or more keywords in the text box and search those values by clicking search button. The form will look like following:
4. Build the Logic
This is the next step after creating user interface (UI) and specifying connection setting to MySql. The logic which I have implemented is given in code below:
5. Display the Result
The result is displayed as HTML table in which the first column shows the serial number for rows and second column displays the data fetched from the matching rows in the 'field1'.
How to do a search in PHP?
In the PHP search script, do a SELECT * FROM `TABLE` WHERE `FIELD` LIKE '%SEARCH%' SQL query and output the results in HTML.
What is the top half of a search form?
A – The top half is nothing but a simple HTML search form. Only has one text field and submits to itself.
Is AJAX search the same as HTML?
Yep, the HTML for AJAX searches remain the same. Just take note that we are using Javascript to manage the form submission – <form onsubmit="return fetch ();">
How many characters are short words?
Short words will be ignored, the default minimum length is 4 characters. You can change the min and max word length with the variables ft_min_word_len and ft_max_word_len
How to disable stopwords?
You can disable stopwords by setting the variable ft_stopword_file to an empty string.
Can MySQL use full text search?
Let’s talk about the basics for using MySQL Full-Text Search. It can’t be implemented on MySQL default In noDB storage engine tables. So if you are using InnoDB you need to convert them to MyISAM Engine.
Preparation
You should have Apache, MySQL and PHP installed and running of course (you can use XAMPP for different platforms or WAMP for windows, MAMP for mac) or a web server/hosting that supports PHP and MySQL databases.
Done!
Now it works. Try different words, variations, editing code, experiment. I'm adding full code of both files in case you think you've missed something. Feel free to ask questions or ask for tutorials.
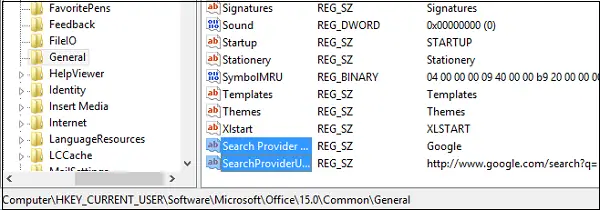