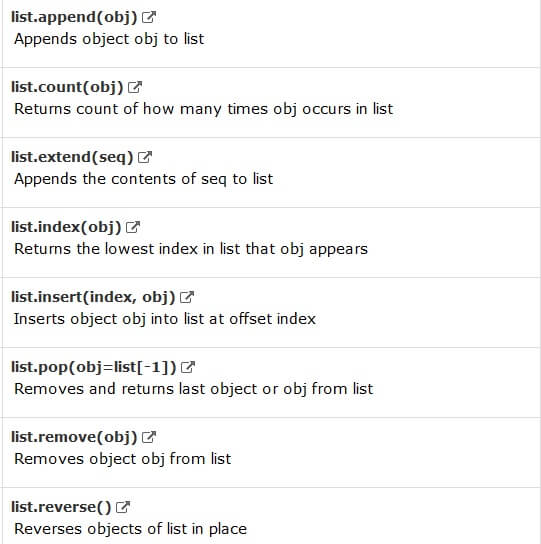
- List. Lists are used to store multiple items in a single variable. ...
- List Items. List items are ordered, changeable, and allow duplicate values. List items are indexed, the first item has index [0] , the second item has index [1] etc.
- Ordered. When we say that lists are ordered, it means that the items have a defined order, and that order will not change.
- Changeable. The list is changeable, meaning that we can change, add, and remove items in a list after it has been created.
- Allow Duplicates
- List Length
- List Items - Data Types
- type () What is the data type of a list?
- The list () Constructor. It is also possible to use the list () constructor when creating a new list.
- Python Collections (Arrays) List is a collection which is ordered and changeable. Allows duplicate members. Tuple is a collection which is ordered and unchangeable.
How to create and initialize list of lists in Python?
Initialize a List Using list() Python list() function creates the list from the iterable object. An iterable may be a container, a sequence that supports iteration, or an iterator object. If no parameter is specified, then a new empty list is created. Another way to create and initialize the list with no values is to use the list() method.
How do you make a list in Python?
Python apply function to a list
- The apply function to a list, we will use a map ().
- Call map (func, *iterables) with a list as iterables for applying a function to each element in the list and it returns a map object.
- Use list () to convert the map object to list.
How to get item or element from list in Python?
Specifically, here is what we will cover in depth:
- An overview of lists in Python How indexing works
- Use the index () method to find the index of an item 1. Use optional parameters with the index () method
- Get the indices of all occurrences of an item in a list Use a for-loop to get indices of all occurrences of an item in a list Use list comprehension ...
How to remove an item from the list in Python?
Syntax
- To remove an element from the list, you need to pass the index of the element. The index starts at 0. ...
- The index argument is optional. If not passed, the default value is considered -1, and the last element from the list is returned.
- If the index given is not present, or out of range, the pop () method throws an error saying IndexError: pop index.

How do Python lists work internally?
Python lists are internally represented as arrays. The idea used is similar to implementation of vectors in C++ or ArrayList in Java. The costly operations are inserting and deleting items near the beginning (as everything has to be moved). Insert at the end also becomes costly if preallocated space becomes full.
What are 3 types of list in Python?
Python Collections (Arrays)List is a collection which is ordered and changeable. Allows duplicate members.Tuple is a collection which is ordered and unchangeable. ... Set is a collection which is unordered, unchangeable*, and unindexed. ... Dictionary is a collection which is ordered** and changeable.
Are list () and [] the same Python?
Technically speaking, one is a function that returns an object casted to a list, and the other is the literal list object itself. Kinda like int(0) vs 0 . In practical terms there's no difference. I'd expect [] to be faster, because it does not involve a global lookup followed by a function call.
What does list [:] mean in Python?
What Does [:] Mean In Python? Code Examples. When using the slice operator [start:stop:step] to capture only a subset of data from an original list or string, what does [:] do? The slice operator containing no values for the start and stop positions returns a complete copy of the original string or list.
What's a tuple in Python?
Tuple. Tuples are used to store multiple items in a single variable. Tuple is one of 4 built-in data types in Python used to store collections of data, the other 3 are List, Set, and Dictionary, all with different qualities and usage.
What is the difference between list and tuple?
In Python, tuples are allocated large blocks of memory with lower overhead, since they are immutable; whereas for lists, small memory blocks are allocated. Between the two, tuples have smaller memory. This helps in making tuples faster than lists when there are a large number of elements.
Why use a tuple instead of a list?
Tuples are more memory efficient than the lists. When it comes to the time efficiency, again tuples have a slight advantage over the lists especially when lookup to a value is considered. If you have data which is not meant to be changed in the first place, you should choose tuple data type over lists.
Is a Python list an array?
While lists and arrays are superficially similar—they are both multi-element data structures—they behave quite differently in a number of circumstances. First of all, lists are part of the core Python programming language; arrays are a part of the numerical computing package NumPy.
Why is an array better than a list?
Arrays can store data very compactly and are more efficient for storing large amounts of data. Arrays are great for numerical operations; lists cannot directly handle math operations. For example, you can divide each element of an array by the same number with just one line of code.
What does this mean in Python [: 0?
It acts as an indicator in the format method that if you want it to be replaced by the first parameter(index zero) of format. Example : print(42+261={0}.format(303)) Here, {0} will be replaced by 303.
What is the difference between array and list in Python?
List is used to collect items that usually consist of elements of multiple data types. An array is also a vital component that collects several items of the same data type. List cannot manage arithmetic operations. Array can manage arithmetic operations.
What does :: mean in list?
So: [start::] will return the list starting from 'start' until the last element [:end:] will return all from the first element until the one preceding 'end' [0:-3] will return elements starting from the first one until (but not including) the third to last [::2] will return every second element of the list [::-3] will ...
How do you print 3 lists in Python?
3 Easy Methods to Print a Python ListUsing map() function to print a Python List. Python map() function can be clubbed with join() function to print a Python list easily. ... Using '*' symbol to print a Python list. Next, we will be using Python '*' symbol to print a list. ... Naïve Method- Using for loop.
What is list and its types?
There are three list types in HTML: unordered list — used to group a set of related items in no particular order. ordered list — used to group a set of related items in a specific order. description list — used to display name/value pairs such as terms and definitions.
What is list type of list?
There are three different types of HTML lists: Ordered List or Numbered List (ol) Unordered List or Bulleted List (ul) Description List or Definition List (dl)
What is list of list in Python?
A list of lists is a list where each element is a list by itself. The List is one of the 4 built-in data types in Python.
What is a list in Python?
The list is a most versatile datatype available in Python which can be written as a list of comma-separated values (items) between square brackets. Important thing about a list is that items in a list need not be of the same type.
What are the most common sequences in Python?
There are certain things you can do with all sequence types. These operations include indexing, slicing, adding, multiplying, and checking for membership.
How to access values in a list?
To access values in lists, use the square brackets for slicing along with the index or indices to obtain value available at that index. For example −
How to update multiple elements in a list?
You can update single or multiple elements of lists by giving the slice on the left-hand side of the assignment operator, and you can add to elements in a list with the append () method. For example −
How many types of sequences are there in Python?
Python has six built-in types of sequences, but the most common ones are lists and tuples, which we would see in this tutorial. There are certain things you can do with all sequence types. These operations include indexing, slicing, adding, multiplying, and checking for membership. In addition, Python has built-in functions for finding ...
Do lists respond to all sequence operations?
In fact, lists respond to all of the general sequence operations we used on strings in the prior chapter.
What is a list in Python?
Python has a great built-in list type named "list". List literals are written within square brackets [ ]. Lists work similarly to strings -- use the len () function and square brackets [ ] to access data, with the first element at index 0. (See the official python.org list docs .)
What does list.remove do?
list.remove (elem) -- searches for the first instance of the given element and removes it (throws ValueError if not present)
What is a variable name in a Python loop?
If you know what sort of thing is in the list, use a variable name in the loop that captures that information such as "num", or "name", or "url". Since Python code does not have other syntax to remind you of types, your variable names are a key way for you to keep straight what is going on.
What does list append do?
list.append (elem) -- adds a single element to the end of the list. Common error: does not return the new list, just modifies the original.
What is an empty list?
The "empty list" is just an empty pair of brackets [ ]. The '+' works to append two lists, so [1, 2] + [3, 4] yields [1, 2, 3, 4] (this is just like + with strings).
Can you use for/in in Python?
You may have habits from other languages where you start manually iterating over a collection, where in Python you should just use for/in. You can also use for/in to work on a string. The string acts like a list of its chars, so for ch in s: print ch prints all the chars in a string.
Does Python have a while loop?
Python also has the standard while-loop, and the *break* and *continue* statements work as in C++ and Java, altering the course of the innermost loop. The above for/in loops solves the common case of iterating over every element in a list, but the while loop gives you total control over the index numbers. Here's a while loop which accesses every 3rd element in a list:
What can a Python list contain?
Just as our shopping list could contain items of any type such as fruits, vegetables, sweets and more, a Python list could also contain items of any type.
What are the functions that can be used to get the length of a list?
You can apply common built-in functions such as len (), min () , and max () on lists to get the length of the list, the minimum element, and the maximum element, respectively.
How to remove an item from an index?
If we would like to remove an item from a particular index, we can specify the index as an argument to pop ().
How to check how many times a specific item occurs in a list?
Another useful method is count which you can use to check how many times a specific item occurs in our list. list_name.count (elt) returns the number of times elt occurs in list list_name.
What if we had an other iterable that we wanted to add to an existing list?
What if we had an another list (or any other iterable) that we wanted to add to an existing list? Instead of adding the items from the new list one by one, we could use the extend () method to add the entire list to the first list as shown below.
What is it called when you read through a shopping list?
It’s quite common to read through our shopping_list to check if we’ve purchased all that we need. This is called traversing through the list.
Why use range in a list?
If we were to do some operations on the list instead, it's recommended to use range to get a set of indices that we can then loop through.
Where is the list stored after creating it?
After we created the list, we stored it in the computer’s memory by assigning it to a variable named row_1 .
How to retrieve a list of elements?
The syntax for retrieving individual list elements follows the model list_name [index_number]. For instance, the name of our list above is row_1 and the index number of the first element is 0 — following the list_name [index_number] model, we get row_1 [0], where the index number 0 is in square brackets after the variable name row_1 .
How many rows are in a dataset?
We can understand our entire table above as a collection of data points, so we call the entire table a dataset. We can see that our data set has five rows and five columns.
What is the index number of a list?
Each element (data point) in a list has a specific number associated with it, called an index number. The indexing always starts at 0, so the first element will have the index number 0, the second element the index number 1, and so on.
How to find the index of a list?
To quickly find the index of a list element, identify its position number in the list, and then subtract 1. For example, the string 'USD' is the third element of the list (position number 3), so its index number must be 2 since 3 – 1 = 2. The index numbers help us retrieve individual elements from a list.
What is the syntax for selecting the first n elements in a list?
When we select the first n elements ( n stands for a number) from a list named a_list, we can use the syntax shortcut a_list [0:n]. In the example above, we needed to select the first three elements from the list row_3, so we used row_3 [0:3] .
How many data points are in a Facebook list?
The ['Facebook', 0.0, 'USD', 2974676, 3.5] list has five data points. To find the length of a list, we can use the len () command:
Why do we use list in Python?
The biggest reason to use a list is to able to find objects by their position in the list. To do this, you use Python’s index notation: a number in brackets, starting at 0, that indicates the position of the item in the list.
How to define a list in Python?
Defining a list in Python is easy — just use the bracket syntax to indicate items in a list.
What does indexing mean in Python?
Python list indexing. If you use a positive integer for the index, the integer indicates the position of the item to look for. But if you use a negative integer, then the integer indicates the position starting from the end of the list. For example, using an index of -1 is a handy way to grab the last item from a list no matter the size of the list.
What happens if you omit slice index in Python?
If you omit a particular slice index, Python assumes a default. Leave off the start index, and Python assumes the start of the list:
What is a key parameter in sorted?
Both sorted () and .sort () also take a key parameter. The key parameter lets you provide a function that can be used to perform a custom sorting operation. When the list is sorted, each element is passed to the key function, and the resulting value is used for sorting. For instance, if we had a mix of integers and strings, and we wanted to sort them, we could use key like this:
What is the process of slicing in Python?
Python’s slice syntax lets you specify which part of a list to carve off, and how to manipulate the carved-off portion.
What is a slice of a list?
Also keep in mind that slices of lists are copies of the original list. The original list remains unchanged.
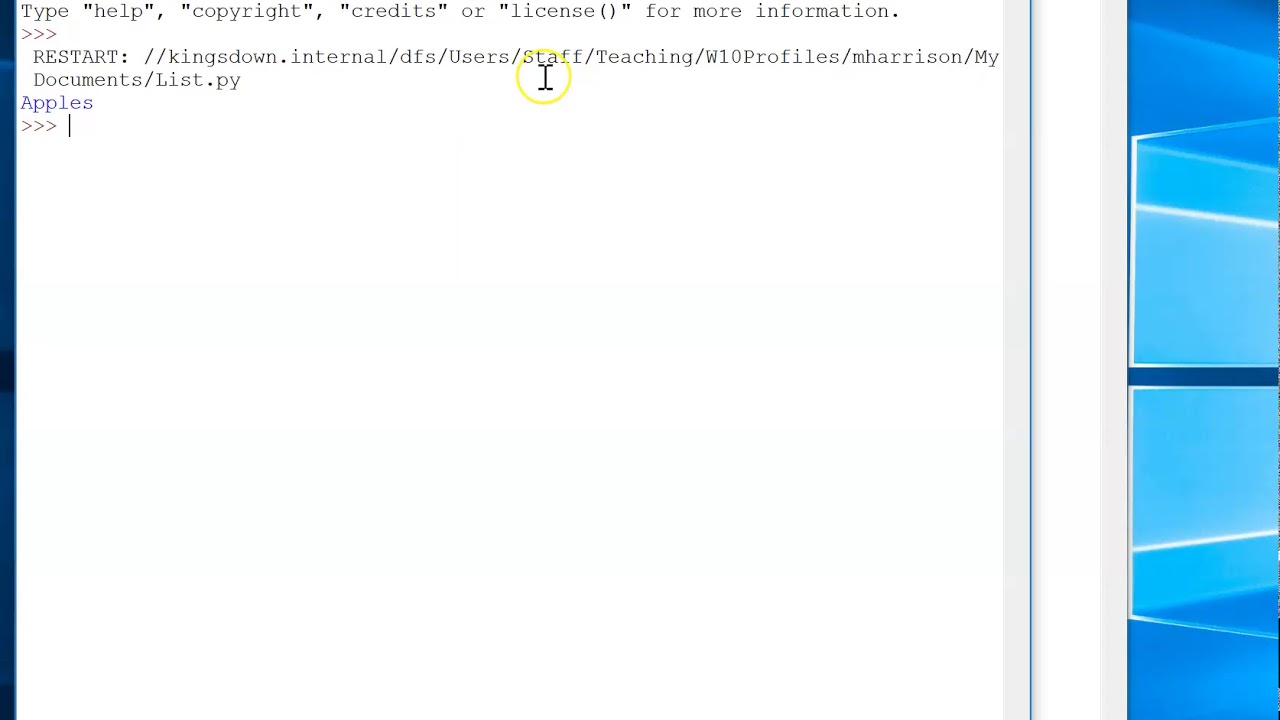
Python Lists
- List items are ordered, changeable, and allow duplicate values. List items are indexed, the first item has index [0],the second item has index etc.
Accessing Values in Lists
Updating Lists
Delete List Elements
Basic List Operations
- The list is a most versatile datatype available in Python which can be written as a list of comma-separated values (items) between square brackets. Important thing about a list is that items in a list need not be of the same type. Creating a list is as simple as putting different comma-separated values between square brackets. For example − Similar...
Indexing, Slicing, and Matrixes
- To access values in lists, use the square brackets for slicing along with the index or indices to obtain value available at that index. For example − When the above code is executed, it produces the following result −