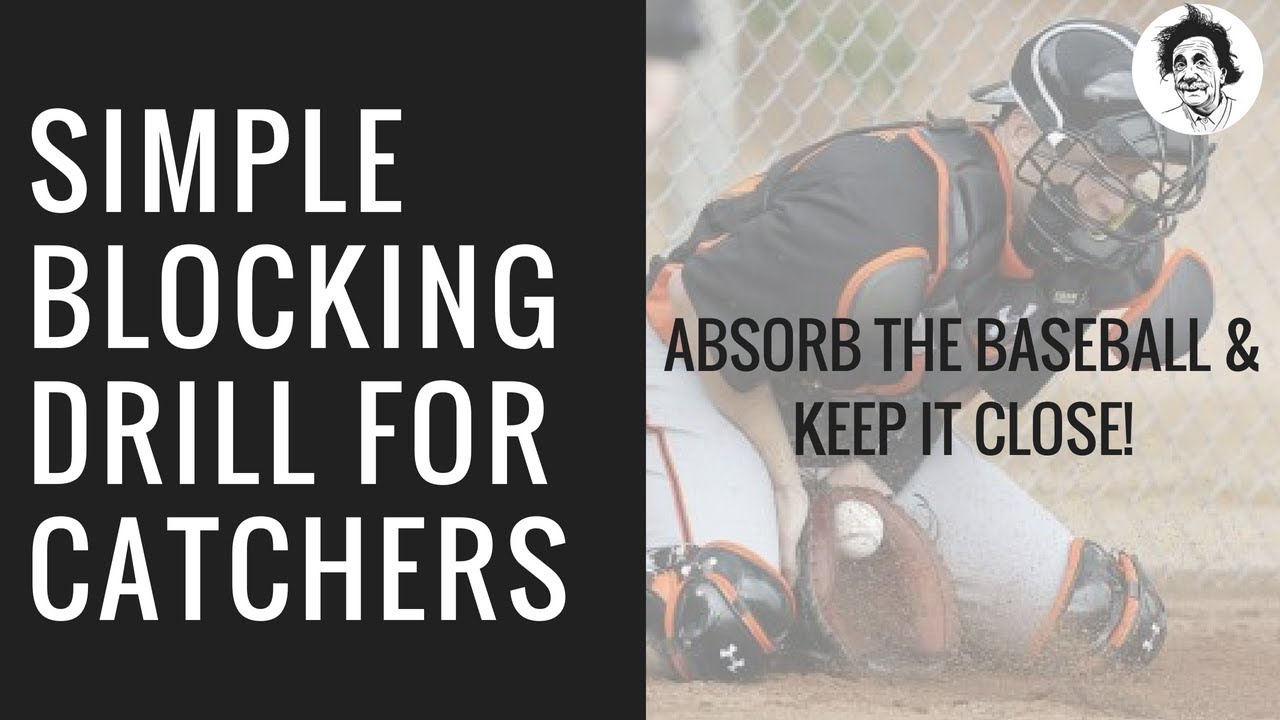
How do try catch blocks work in Java?
Java try and catch The try statement allows you to define a block of code to be tested for errors while it is being executed. The catch statement allows you to define a block of code to be executed, if an error occurs in the try block.
Are try catch blocks good practice?
So using few try-catch blocks shouldn't affect a performance at all. In some opinion writing code that way obfuscates the code and does not even recall "clean code", in others opinion it's better to use try only for lines which can actually throw any exception. It's up to you decide (or the team convention).
Do try blocks need a catch?
The try block contains set of statements where an exception can occur. A try block is always followed by a catch block, which handles the exception that occurs in associated try block. A try block must be followed by catch blocks or finally block or both.
Should everything be in a try catch?
Exactly. Putting everything in a try/catch statement is usually a sign of an inexperienced developer who doesn't know much about exceptions IME.
When should we use try catch?
try-catch statements are used in Java to handle unwanted errors during the execution of a program.Try: The block of code to be tested for errors while the program is being executed is written in the try block.Catch: The block of code that is executed when an error occurs in the try block is written in the catch block.
Is an empty catch block legal?
Yes, we can have an empty catch block. But this is a bad practice to implement in Java. Generally, the try block has the code which is capable of producing exceptions, if anything wrong in the try block, for instance, divide by zero, file not found, etc.
How do you exit a try catch block?
“exit try catch java” Code Answerpublic class MyClass {public static void main(String[ ] args) {try {int[] myNumbers = {1, 2, 3, 4, 5, 6};System. out. println(myNumbers[10]);} catch (Exception e) {System. out. println("Something went wrong. check again");}More items...•
Does try catch stop execution?
The “try… First, the code in try {...} is executed. If there were no errors, then catch (err) is ignored: the execution reaches the end of try and goes on, skipping catch . If an error occurs, then the try execution is stopped, and control flows to the beginning of catch (err) .
Should you always use try-catch Javascript?
You shouldn't use the try-catch statement if you know an error is going to occur, because in this case you would want to debug the problem, not mask it.
Why is try-catch important?
By requiring a try-catch block, Java is forcing you to deal with the exceptions that can happen in your code and make a decision on what to do with them, in order to allow your code to fail gracefully.
Is it good to use try-catch in node JS?
If your encryption/decryption calls are synchronous (depends on the library, module, function you use), try/catch is ok to use it, otherwise depending on how you've used it, it might be useless. Try/catch is ok to catch a programming error or buggy code, for example from libraries you don't know might be buggy.
Where to place code statements to handle exceptions?
Place any code statements that might raise or throw an exception in a try block, and place statements used to handle the exception or exceptions in one or more catch blocks below the try block. Each catch block includes the exception type and can contain additional statements needed to handle that exception type.
Can CLR throw exceptions?
Most code can throw an exception, and some exceptions, like OutOfMemoryException, can be thrown by the CLR itself at any time. While applications aren't required to deal with these exceptions, be aware of the possibility when writing libraries to be used by others.
What happens if an exception is thrown inside a catch block?
If an exception is thrown inside the catch-block and that exception is not caught, the catch-block is interrupted just like the try-block would have been.
What happens when a throw statement is executed?
When a throw statement is executed, the JVM examines each stack frameto find out if that frame can handle the exception. It can if its method contains a try-catch block which contains the calling instruction, and the type of block's exception is a supertype (or the same as) of the thrown exception. If such a frame is found, the frame restores its execution from the instruction pointed to from the try-catch block.
When a procedure (function, method) is called, what is the current stack frame?
When a procedure (function, method) is called, the current stack frame is appended with the address of the calling instruction, so as to restore execution of that frame at the correct instruction (next after calling instruction).
What happens when an exception is thrown?
When an exception is thrown, the complete calling-stack information is attached not to some magic object, but to the exception object that is created. This doesn't happen while the exception "bubbles up" - it happens when it is created and it always contains the full call-chain.
When an exception is thrown, the method stops execution?
When an exception is thrown the method stops execution right after the "throw" statement. Any statements following the "throw" statement are not executed. The program resumes execution when the exception is caught somewhere by a "catch" block.
Does a function need to know if it is surrounded by a try-catch block?
The called function doesn't need to know it is surrounded by a try-catch-block, it just creates an Exception-object that contains the call-chain and passes it up to the calling method. This method has to decide wether it handles the Exception because it is caught by some catch-clause or if it passes it further up. Exception that aren't caught bubble up till they reach the top of the calling-chain and the VM handles them - usually by printing the stack-trace and terminating.
What is try.catch used for?
If an error occurs, we can use try...catch to catch the error and execute some code to handle it:
What is try statement?
The try statement allows you to define a block of code to be tested for errors while it is being executed.
What is throw statement in Java?
The throw statement is used together with an exception type. There are many exception types available in Java: ArithmeticException, FileNotFoundException, ArrayIndexOutOfBoundsException, SecurityException, etc:
What happens when Java code is executed?
When an error occurs, Java will normally stop and generate an error message.
What happens if there is no catch block after try?
Even if there is no catch block after try, finally block will still execute.
Does the finally block get run?
Yes, the finally block gets run whether there is an exception or not.
Does try catch finally execute?
Any code outside the try-catch-finally statement will not execute if you returned the method inside the try/catch, but the finallystatement will. please see my additional answer here
Does finallyblock always execute before method returns?
The finallyblock will always execute before the method returns.
How to use a catch block in Java?
Java catch block is used to handle the Exception by declaring the type of exception within the parameter. The declared exception must be the parent class exception ( i.e., Exception) or the generated exception type. However, the good approach is to declare the generated type of exception.
What is the Java try block followed by?
Java try block must be followed by either catch or finally block.
How to check if an exception is handled?
The JVM firstly checks whether the exception is handled or not. If exception is not handled, JVM provides a default exception handler that performs the following tasks: 1 Prints out exception description. 2 Prints the stack trace (Hierarchy of methods where the exception occurred). 3 Causes the program to terminate.
Can you use multiple catch blocks with a single try block?
However, the good approach is to declare the generated type of exception. The catch block must be used after the try block only. You can use multiple catch block with a single try block. If playback doesn't begin shortly, try restarting your device.
Can you use catch block to handle exceptions?
Here, we can see that the catch block didn't contain the exception code. So, enclose exception code within a try block and use catch block only to handle the exceptions.
What is a try block?
The try block contains set of statements where an exception can occur. A try block is always followed by a catch block, which handles the exception that occurs in associated try block. A try block must be followed by catch blocks or finally block or both.
Why do we need other catch handlers?
This is because in generic exception handler you can display a message but you are not sure for which type of exception it may trigger so it will display the same message for all the exceptions and user may not be able to understand which exception occurred. Thats the reason you should place is at the end of all the specific exception catch blocks
Can you catch different exceptions in different catch blocks?
You can catch different exceptions in different catch blocks. When an exception occurs in try block, the corresponding catch block that handles that particular exception executes. For example if an arithmetic exception occurs in try block then the statements enclosed in catch block for arithmetic exception executes.
Can you have multiple try blocks?
A single try block can have multiple catch blocks associated with it, you should place the catch blocks in such a way that the generic exception handler catch block is at the last (see in the example below). The generic exception handler can handle all the exceptions but you should place is at the end, if you place it at the before all ...
Can a try block have a number of catch blocks?
1. As I mentioned above, a single try block can have any number of catch blocks. 2. A generic catch block can handle all the exceptions. Whether it is ArrayIndexOutOfBoundsException or ArithmeticException or NullPointerException or any other type of exception, this handles all of them. To see the examples of NullPointerException ...
When is a catch block used?
When a catch -block is used, the catch -block is executed when any exception is thrown from within the try -block. For example, when the exception occurs in the following code, control transfers to the catch -block.
What is a catch block?
A catch -block contains statements that specify what to do if an exception is thrown in the try -block. If any statement within the try -block (or in a function called from within the try -block) throws an exception, control is immediately shifted to the catch -block. If no exception is thrown in the try -block, the catch -block is skipped.
What is finally block?
The finally -block contains statements to execute after the try -block and catch -block (s) execute, but before the statements following the try...catch...finally -block. Note that the finally -block executes regardless of whether an exception is thrown. Also, if an exception is thrown, the statements in the finally -block execute even if no catch -block handles the exception.
What happens if no exception is thrown in the try block?
If no exception is thrown in the try -block, the catch -block is skipped. The finally -block will always execute after the try -block and catch -block (s) have finished executing. It always executes, regardless of whether an exception was thrown or caught. You can nest one or more try statements.
What happens when the finally block returns a value?
If the finally -block returns a value, this value becomes the return value of the entire try-catch-finally statement, regardless of any return statements in the try and catch -blocks. This includes exceptions thrown inside of the catch -block:
What is a try.catch statement?
The try...catch statement marks a block of statements to try and specifies a response should an exception be thrown.
What is the exception_var in a try block?
When an exception is thrown in the try -block, exception_var (i.e., the e in catch (e) ) holds the exception value. You can use this identifier to get information about the exception that was thrown. This identifier is only available in the catch -block's scope. If you don't need the exception value, it could be omitted.
What is a finally block?
The finally block is an optional block that runs regardless of whether there were errors or not.
What happens if the except block fails?
If it fails, the except block catches the exception raised.
What is optional finally block?
The optional finally block. This runs a piece of code regardless of whether there were errors or not. This is an optional block of code you don’t need in the error handling scheme.
Can you add an optional else block in Python?
You can add an optional else block after except blocks in Python. This runs a piece of code if there were no errors thrown.
Is there a try except structure?
Instead, there is a try-except structure dedicated for error handling. This works similarly to the try-catch you’ve seen in some other languages.
Is there such a thing as try-catch in Python?
In Python, there is no such thing as try-catch. Instead, Python uses the try-except approach to deal with errors and exceptions.
Can you have multiple except blocks in Python?
In Python, you can have multiple except blocks for handling different types of errors separately.
What is a catch block?
The Catch block contains the actions to execute when it receives an error from the Try block. There can be multiple Catch blocks in a Try statement.
What is a catch block in PowerShell?
PowerShell try catch blocks (and optional finally block) are a way to cast a net around a piece of code and catch any errors that return.
What is the purpose of the $ErrorActionPreference variable?
The $ErrorActionPreference variable is used to change the way PowerShell treats non-terminating errors. By default, the $ErrorActionPreference value is set to Continue. Changing the value of the $ErrorActionPreference variable to STOP forces PowerShell to treat all errors as terminating errors.
What is a try statement?
A simple Try statement contains a Try and a Catch block. The Finally block is optional.
Can a script modify $ErrorActionPreference?
Now, refer back to the example used in the Non-Terminating Errors section in this article. The script can modified to include the change in $ErrorActionPreference like the code shown below:
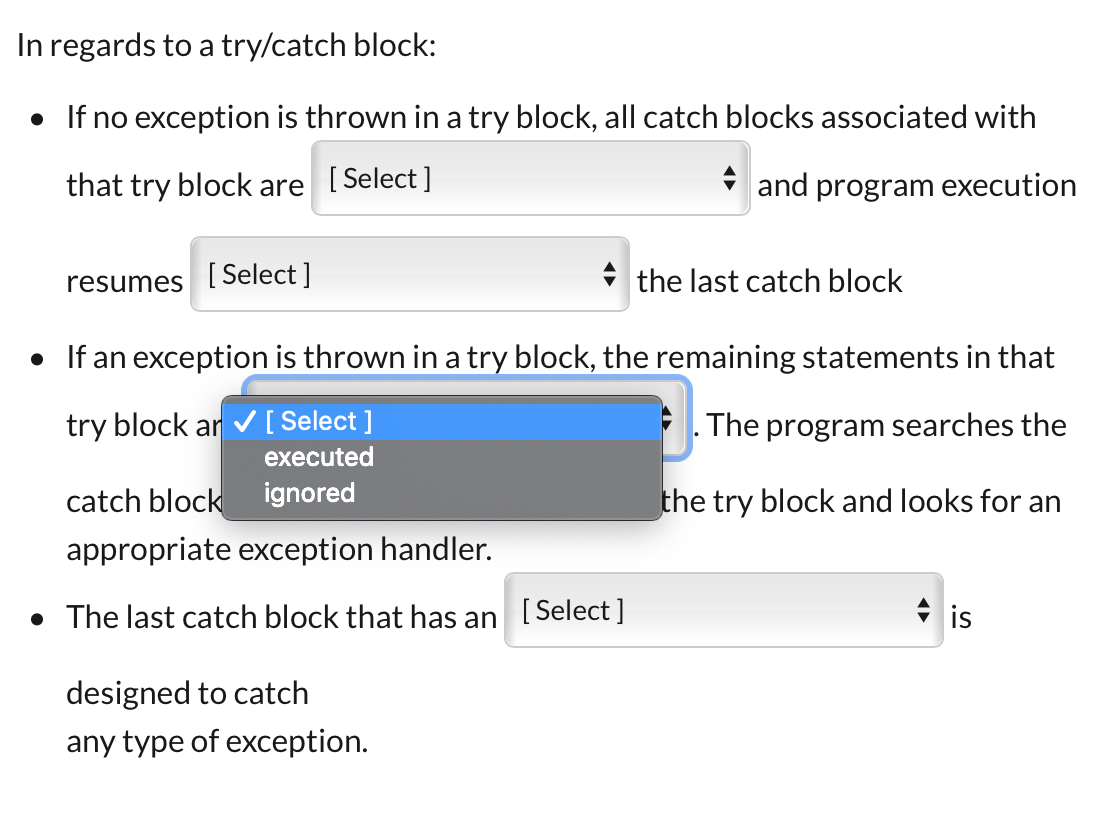