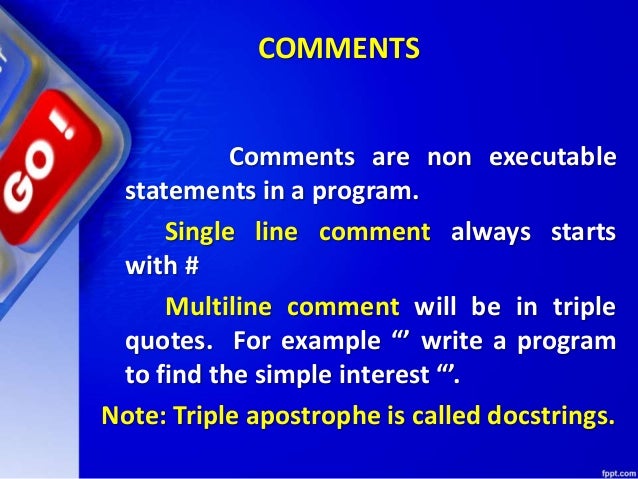
In Python, assert is a simple statement with the following syntax: assert expression[, assertion_message] Here, expression can be any valid Python expression or object, which is then tested for truthiness. If expression is false, then the statement throws an AssertionError. The assertion_message parameter is optional but encouraged.
What is the use of assert in Python?
Why we use assert in Python?
- We use assert for checking the outputs of the functions.
- We use assert as a debugging tool for testing the code. The error can only be raised when there is a bug in the code.
- We use assert for checking the values of arguments.
- We use assert for checking the valid input.
How to write if else in Python?
- Collect user input for value b which will be converted to integer type
- If value of b is equal to 100 then return " equal to 100 ", If this returns False then next if else condition would be executed
- If value of b is equal to 50 then return " equal to 50 ", If this returns False then next if else condition would be executed
How to write inline if statement for print in Python?
Python For Loops and If Statements Combined (Python for Data Science Basics #6)
- For loop within a for loop – aka the nested for loop. ...
- If statement within a for loop. Inside a for loop, you can use if statements as well. ...
- Break. There is a special control flow tool in Python that comes in handy pretty often when using if statements within for loops.
- Test Yourself! ...
- Solution. ...
- Conclusion. ...
What is an else statement in Python?
Python’s nested if/else statement: evaluate complex, dependent conditions
- # An if/else statement that depends on other if statements. A nested if/else statement is an if/else statement that is nested (meaning, inside) another if statement or if/else statement.
- # Python programs that use nested if/else statements. ...
- # Other types of Python if statements. ...
- # Summary. ...
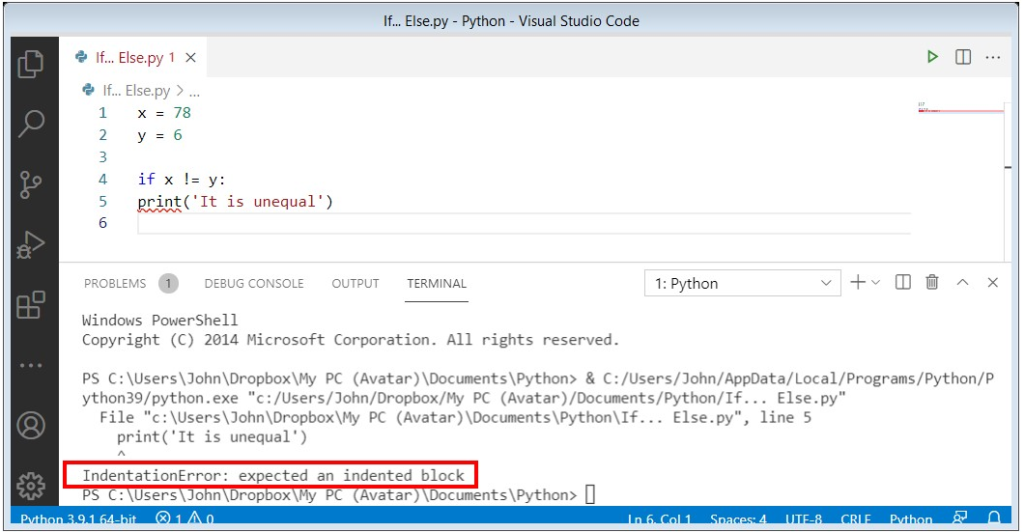
What is assert statement in Python?
Python has built-in assert statement to use assertion condition in the program. assert statement has a condition or expression which is supposed to be always true. If the condition is false assert halts the program and gives an AssertionError.
What is assert in programming?
Assertions are statements that assert or state a fact confidently in your program. For example, while writing a division function, you're confident the divisor shouldn't be zero, you assert divisor is not equal to zero. Assertions are simply boolean expressions that check if the conditions return true or not.
What happens when the condition is not satisfied in an assert statement?
assert statement can also have a condition and a optional error message. If the condition is not satisfied assert stops the program and gives AssertionError along with the error message.
What is assert in Python?
Like few other programming languages, Python also has its built-in assert. statement, which is used to continuously execute the condition if assert evaluates to True, else it will return the AssertionError i.e., Exception.
Why do we use assertions in programming?
Programmers use assertion as a tool to debug the program. It brings down the location where the logical inconsistency is found and makes the assertion evaluations false during the execution time.
What is assertion in testing?
An assertion is nothing but a sanity check that can be turned off or turned on when the program testing is completed. The simplest method to think about an assertion is as an improvement on the “if statement”. During the testing of an expression, when the result of the test is false, then an exception can be raised.
How to do addition and subtraction in Python?
Step 1: Defining Addition and Subtraction function in Python. Step 2: Print the title ‘Choose calc operation to perform. Step 3: Ask for a choice that the user wants to perform calculation . Step 4: Ask the number 1 and number 2 that the user wants to perform calculation for. Step 5: Print the result.
How many subject marks can a Python program execute?
The condition is that subject marks can be aggregated. So, the program should execute only less than or equal to 5 subject marks. If more than 5 subject marks are entered, then the program should abort. Step 1: Defining sum function in Python. Step 2: Initialize array variables with 6 values.
Is assert a try catch?
However, it is not a try-catch in the programming. You can learn more about assert in python by joining the Python Training Course from Simplilearn that will teach you the fundamentals of Python, data operations, conditional statements, shell scripting, and Django.
Does Python have an assertion?
Not surprisingly, python also has its own assert statement that can be used by developers. Let us see the assertion flow now for quick understanding. High level illustration of an assertion in a program. Thus, the assert in Python or any other programming language does the following.
Assert Keyword in Python
In python assert keyword helps in achieving this task. This statement simply takes input a boolean condition, which when returns true doesn’t return anything, but if it is computed to be false, then it raises an AssertionError along with the optional message provided.
Practical Application
This has a much greater utility in testing and Quality assurance role in any development domain. Different types of assertions are used depending upon the application. Below is the simpler demonstration of a program that only allows only the batch with all hot food to be dispatched, else rejects the whole batch.
How to use assert?
As you would imagine, the assert keyword is built into Python. It must be followed by a condition, and you can optionally put a message that gets printed if the condition evaluates to false.
Assertion in Python functions
Let’s declare a simple Python function. It will take in a list as an argument, sum it, and then return the sum as a float. There are two problems you want to catch early on:
How to ignore assertions on runtime?
What’s particularly neat about assert statements is that you can ignore them when running the scripts, without modifying the code.
Should you use assert statements?
I’ve told it before, but I’ll repeat once again — assertion isn’t a replacement for try except blocks. You should always use try except for errors that will inevitably happen.
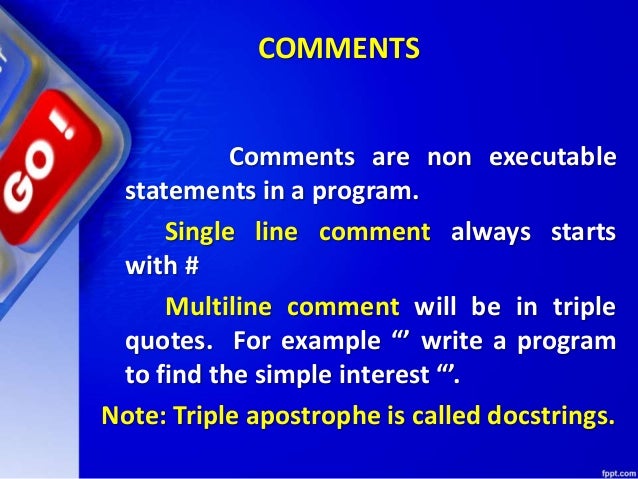