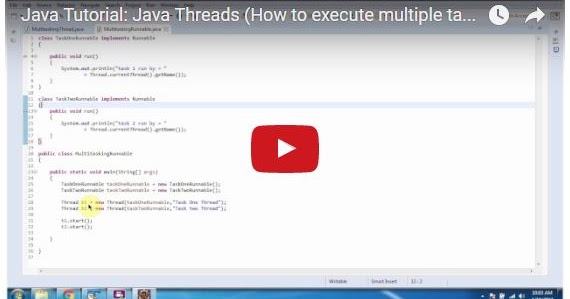
Commonly used methods of Thread class:
- public void run():is used to perform action for a thread.
- public void start():starts the execution of the thread.JVM calls the run() method on the thread.
- public void sleep(long miliseconds):Causes the currently executing thread to sleep (temporarily cease execution) for the specified number of milliseconds.
- public void join():waits for a thread to die.
What is threading in Java and how it works?
Each program can have multiple associated threads. Each thread has a priority which is used by the thread scheduler to determine which thread must run first. Java provides a thread class that has various method calls in order to manage the behavior of threads by providing constructors and methods to perform operations on threads.
What is the start () method of a thread?
A thread in a program starts with a method () frequently used in this class only known as the start () method. This method looks out for the run () method which is also a method of this class and begins executing the bod of run () method. here keep an eye over the sleep () method which will be discussed later below.
What is each thread priority in Java?
Each thread has a priority which is used by thread scheduler to determine which thread must run first. Java provides a thread class that has various method calls inorder to manage the behaviour of threads. Note: Every class that is used as thread must implement Runnable interface and over ride it’s run method.
How do I run a runnable in a thread?
In your "main" thread, create a new Thread class, passing the constructor an instance of your Runnable, then call start () on it. start tells the JVM to do the magic to create a new thread, and then call your run method in that new thread. Take a look at Java's concurrency tutorial to get started.

How do you run a thread in Java?
If the class extends the Thread class, the thread can be run by creating an instance of the class and call its start() method:Extend Example. public class Main extends Thread { public static void main(String[] args) { Main thread = new Main(); thread. ... Implement Example. ... Example. ... Example.
What method is called to run a thread?
Summarystart()run()Creates a new thread and the run() method is executed on the newly created thread.No new thread is created and the run() method is executed on the calling thread itself.Can't be invoked more than one time otherwise throws java.lang.IllegalStateExceptionMultiple invocation is possible1 more row•Jan 23, 2019
How do you initiate a thread?
The start() method of thread class is used to begin the execution of thread. The result of this method is two threads that are running concurrently: the current thread (which returns from the call to the start method) and the other thread (which executes its run method).
How do you call a thread from another thread in Java?
In Java Threads, if any thread is in sleeping or waiting state (i.e. sleep() or wait() is invoked), calling the interrupt() method on the thread, breaks out the sleeping or waiting state throwing InterruptedException.
What is run () in Java?
The run() method is available in the thread class constructed using a separate Runnable object. Otherwise, this method does nothing and returns. We can call the run() method multiple times. The run() method can be called in two ways which are as follows: Using the start() method.
How do you call a method in Java?
To call a method in Java, simply write the method's name followed by two parentheses () and a semicolon(;). If the method has parameters in the declaration, those parameters are passed within the parentheses () but this time without their datatypes specified.
How do you initialize a thread in Java?
How to Create a Java Threadpublic void run( )public class MyClass implements Runnable { public void run(){ System. out. println("MyClass running"); ... Thread t1 = new Thread(new MyClass ()); t1. start();public class MyClass extends Thread { public void run(){ System. out. ... MyClass t1 = new MyClass (); T1. start();
How do I run a runnable thread?
To use the Runnable interface to create and start a thread, you have to do the following:Create a class that implements Runnable .Provide a run method in the Runnable class.Create an instance of the Thread class and pass your Runnable object to its constructor as a parameter.Call the Thread object's start method.
What is runnable and callable in Java?
Callable interface and Runnable interface are used to encapsulate tasks supposed to be executed by another thread. However, Runnable instances can be run by Thread class as well as ExecutorService but Callable instances can only be executed via ExecutorService.
How do I run one thread after another?
We can use use join() method of thread class. To ensure three threads execute you need to start the last one first e.g. T3 and then call join methods in reverse order e.g. T3 calls T2. join, and T2 calls T1. join.
How do you call a thread from another thread?
You can use delegate, for example. Show activity on this post. It'll be executed on the main thread, since it's that thread that calls the method. If you want dosomething to run in the separate thread, have it called within run() and store the result in a myThread field for later retrieval.
Can we join two threads?
Thread class provides the join() method which allows one thread to wait until another thread completes its execution. If t is a Thread object whose thread is currently executing, then t. join() will make sure that t is terminated before the next instruction is executed by the program.
What is the start () and run () method of thread class?
start method of thread class is implemented as when it is called a new Thread is created and code inside run() method is executed in that new Thread. While if run method is executed directly than no new Thread is created and code inside run() will execute on current Thread and no multi-threading will take place.
When start () method on the thread is called?
If you call start() method on Thread, Java Virtual Machine will call run() method and two threads will run concurrently now - Current Thread and Other Thread or Runnable implementation.
Why we use run method in thread?
Java Thread run() method The run() method of thread class is called if the thread was constructed using a separate Runnable object otherwise this method does nothing and returns. When the run() method calls, the code specified in the run() method is executed. You can call the run() method multiple times.
Why thread is called Start method?
The purpose of start() is to create a separate call stack for the thread. A separate call stack is created by it, and then run() is called by JVM. Let us see what happens if we don't call start() and rather call run() directly.
How to create a new thread in Java?
In your "main" thread, create a new Thread class, passing the constructor an instance of your Runnable, then call start () on it. start tells the JVM to do the magic to create a new thread, and then call your run method in that new thread.
How many lines of code can you use in Java 8?
In Java 8 you can do this with one line of code.
What is subscribe on in RXJava?
The subscribeOn () method specifies which scheduler to run the action on - RxJava has several predefined schedulers, including Schedulers.io () which has a thread pool intended for I/O operations, and Schedulers.computation () which is intended for CPU intensive operations.
Is it worth creating a new thread each time?
If your method is going to be called frequently, then it may not be worth creating a new thread each time, as this is an expensive operation. It would probably be best to use a thread pool of some sort. Have a look at Future, Callable, Executor classes in the java.util.concurrent package.
What is a Java thread?from w3schools.com
Java Threads. Threads allows a program to operate more efficiently by doing multiple things at the same time. Threads can be used to perform complicated tasks in the background without interrupting the main program.
How to create a thread in Java?from javatpoint.com
In Java, we can also create a thread by implementing the runnable interface. The runnable interface provides us both the run () method and the start () method.
Why is it important to use threads?from javatpoint.com
Another benefit of using thread is that if a thread gets an exception or an error at the time of its execution, it doesn't affect the execution of the other threads. All the threads share a common memory and have their own stack, local variables and program counter. When multiple threads are executed in parallel at the same time, this process is known as Multithreading.
What is a thread model?from javatpoint.com
These states are as follows: 1) New (Ready to run) A thread is in New when it gets CPU time. 2) Running. A thread is in a Running state when it is under execution. 3) Suspended.
Why is there no way to know which order the code will run?from w3schools.com
Concurrency Problems. Because threads run at the same time as other parts of the program, there is no way to know in which order the code will run. When the threads and main program are reading and writing the same variables, the values are unpredictable.
What is a run method?from javatpoint.com
The method is used for starting a thread that we have newly created. It starts a new thread with a new callstack. After executing the start () method, the thread changes the state from New to Runnable. It executes the run () method when the thread gets the correct time to execute it.
When is a thread suspended?from javatpoint.com
A thread is in the Suspended state when it is temporarily inactive or under execution.
What is a thread in Java?
Thread a line of execution within a program. Each program can have multiple associated threads. Each thread has a priority which is used by thread scheduler to determine which thread must run first. Java provides a thread class that has various method calls inorder to manage the behaviour of threads. Note: Every class that is used as thread must ...
What is an allocate thread?
Allocates a new Thread object so that it has targeted as its run object, has the specified name as its name, and belongs to the thread group referred to by group, and has the specified stack size.
What is a thread class?
Thread class provide constructors and methods to create and perform operations on a thread.Thread class extends Object class and implements Runnable interface.
What happens if you don't extend a thread?
If you are not extending the Thread class, your class object would not be treated as a thread object. So you need to explicitly create the Thread class object. We are passing the object of your class that implements Runnable so that your class run () method may execute.
Can you use thread class to spawn new threads?
We can directly use the Thread class to spawn new threads using the constructors defined above.
How does a thread start?
The start thread performs the following tasks: 1 It stats a new thread 2 The thread moves from New State to Runnable state. 3 When the thread gets a chance to execute, its target run () method will run.
What is the start method?
The start () method internally calls the run () method of Runnable interface to execute the code specified in the run () method in a separate thread.
Threads and multithreading in java with pdf notes
Threads are the entities within a process. Whereas, multithreading is a process of executing multiple threads continuously. In this article, we will focus on discussing what are t hreads and multithreading in java with their pdf notes. Notes are provided at the end of this article.
What are threads?
Definition:- The single sequential flow of control within a program is called a thread.
Life cycle of a Thread
A thread can be in one of the five states. There are only 4 states in the thread life cycle in java new, runnable, non-runnable, and terminated. There is no running state.
Thread Priority
Priorities are represented by a number between 1 and 10. In most cases, the thread scheduler schedules the threads according to their priority ( known as preemptive scheduling ). But it is not guaranteed because it depends on the JVM specification that which scheduling it chooses.
What is multithreading?
Definition:- Multithreading is a process of executing multiple threads continuously.
Why do we use Multithreading?
If an application has several tasks to perform, those tasks will be performed when the thread can get to them.
Which applications can use multithreading?
Any kind of application that has distinct tasks which can be performed independently.
What should we call the start method on?
We should call the start () method on threads in the NEW state (the equivalent of not started). Otherwise, Java will throw an instance of IllegalThreadStateException exception.
Why is thread reusability important?
Thread reusability, in particular, is very important: in a large-scale application, allocating and deallocating many thread objects creates a significant memory management overhead.
What is the new framework in Java 1.8?
Java 1.8 introduced a new framework on top of the Future construct to better work with the computation's result: the CompletableFuture.
Can we switch our mindset from starting threads to submitting tasks?
Thanks to this powerful framework, we can switch our mindset from starting threads to submitting tasks.
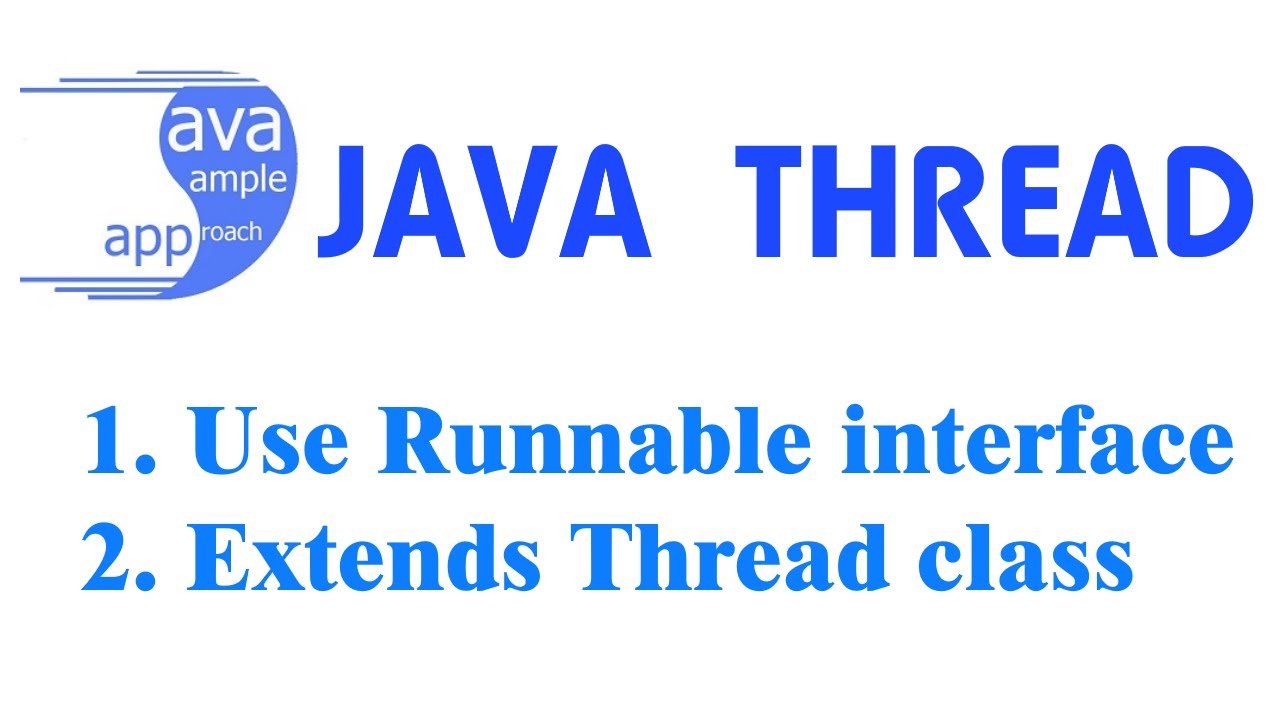