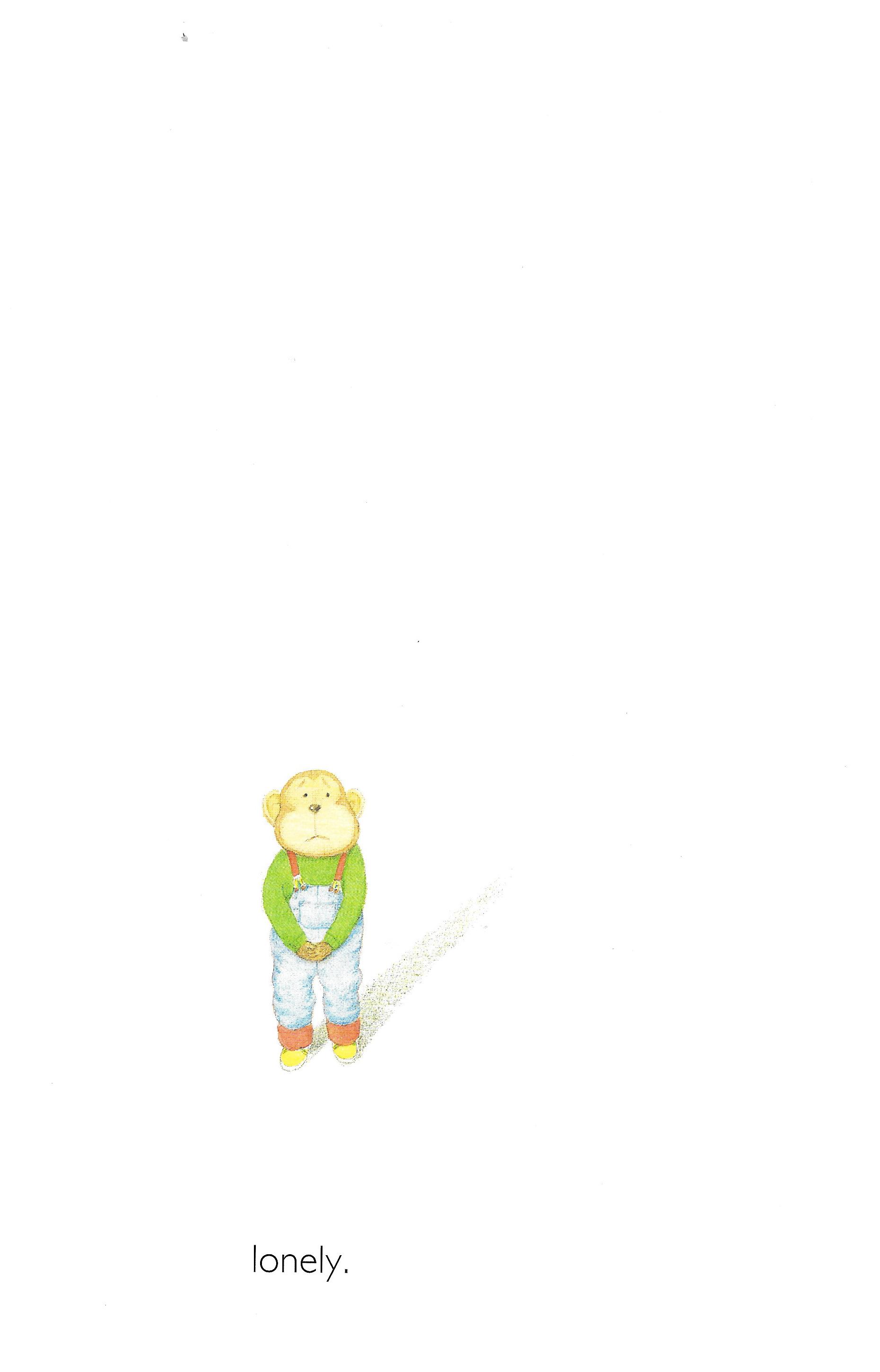
C Program to concatenate string without using strcat ()
- Initially, the program will prompt the user to enter two strings for the concatenation.
- Then we invoke the method concatenateString ().
- In void concatenateString (), while and for loops are used to iterate the length of the string and append the first...
- After the concatenation, the concatenated string is printed on...
- Get the two Strings to be concatenated.
- Declare a new Strings to store the concatenated String.
- Insert the first string in the new string.
- Insert the second string in the new string.
- Print the concatenated string.
How do I concatenate two strings in C without strlcat?
C program to Concatenate Two Strings without using strlcat() This string concatenation program allows the user to enter two string values or two character array. Next, it will use For Loop to iterate each and every character present in that arrays, and joins them (or concatenate them)
What is string concatenation in C?
String Concatenation is a process when one string is appended to the end of another string. 2. C Program to concatenate string without using strcat () Let’s discuss the execution (kind of pseudocode) for the program to concatenate string without using strcat () in C.
How to concatenate two strings in Python?
Approach: 1 Get the two Strings to be concatenated 2 Declare a new Strings to store the concatenated String 3 Insert the first string in the new string 4 Insert the second string in the new string 5 Print the concatenated string More ...
Is it better to use strlen or strcat?
It is better to factor out your strcat logic to a separate function. If you make use of pointer arithmetic, you don't need the strlen function:
How do you concatenate two strings without using the library function?
C Program to Concat Two Strings without Using Library Function#include
How do you concatenate 2 strings?
You concatenate strings by using the + operator. For string literals and string constants, concatenation occurs at compile time; no run-time concatenation occurs. For string variables, concatenation occurs only at run time.
How do you concatenate a string without conc method in Java?
There are some other possible ways to concatenate Strings in Java,String concatenation using StringBuilder class. ... String concatenation using format() method. ... String concatenation using String. ... String concatenation using StringJoiner class (Java Version 8+) ... String concatenation using Collectors.
What is the most efficient way to concatenate many strings together?
When concatenating three dynamic string values or less, use traditional string concatenation.When concatenating more than three dynamic string values, use StringBuilder .When building a big string from several string literals, use either the @ string literal or the inline + operator.
How do you concatenate strings in Excel?
There are two ways to do this:Add double quotation marks with a space between them " ". For example: =CONCATENATE("Hello", " ", "World!").Add a space after the Text argument. For example: =CONCATENATE("Hello ", "World!"). The string "Hello " has an extra space added.
Which operator can be used in string concatenation?
Operator + is used to concatenate strings, Example String s = “i ” + “like ” + “java”; String s contains “I like java”.
What is the best way to concatenate strings in Java?
Using + Operator The + operator is one of the easiest ways to concatenate two strings in Java that is used by the vast majority of Java developers. We can also use it to concatenate the string with other data types such as an integer, long, etc.
How can I add two strings without using operator in Java?
Programimport java. util. *;public static void main(String args[])String str1,str2;Scanner sc = new Scanner(System. in);System. out. println("Enter the 1st string");str1=sc. nextLine();System. out. println("Enter the 2nd string");str2=sc. nextLine();More items...
How do you concatenate strings in Java?
Concatenating StringsUsing the "+" operator − Java Provides a concatenation operator using this, you can directly add two String literals.Using the concat() method − The concat() method of the String class accepts a String value, adds it to the current String and returns the concatenated value.More items...•
How do I optimize a string for concatenation?
Using '+' operatorUsing '+' operator.Using concat() inbuilt method.Using StringBuilder.Using StringBuffer.
Is string interpolation more efficient than concatenation?
String concatenation is a little faster for a very small number of arguments, but requires more memory. After 20+ arguments concat is better by time and memory.
Is string interpolation faster than string format?
The following is a simple code I have, with a bunch of “for loops” with 100,000 iterations for each test: And I was really surprised to see that interpolation is the slowest of all. String concat with '+' took: 2,090 milliseconds. String Builder took: 1 milliseconds.
Which method will you use to combine two strings into one text?
concat() With concat , you can create a new string by calling the method on a string.
How do you use concatenate?
Here are the detailed steps:Select a cell where you want to enter the formula.Type =CONCATENATE( in that cell or in the formula bar.Press and hold Ctrl and click on each cell you want to concatenate.Release the Ctrl button, type the closing parenthesis in the formula bar and press Enter.
Can we add two strings in C?
In C, the strcat() function is used to concatenate two strings. It concatenates one string (the source) to the end of another string (the destination). The pointer of the source string is appended to the end of the destination string, thus concatenating both strings.
How do you concatenate two strings in Python?
Using '+' operator Two strings can be concatenated in Python by simply using the '+' operator between them. More than two strings can be concatenated using '+' operator.
C program to Concatenate Two Strings without using strlcat ()
This program allows the user to enter two string values or two-character array. Next, it will use For Loop to iterate each character present in that arrays, and joins them (or concatenate them). Please refer strcat function as well.
C program to Concatenate Two Strings Using While Loop
This program for string concatenation is the same as above, but this time we are using While Loop (Just replacing Programming For Loop with While Loop).
Concatenate using Functions
This functions program for string concatenation in c is the same as above. However, this time we are using the Functions concept to separate the logic from the main program.
Concatenate Two using Pointers
This string concatenation in c program is same as second example, but this time we are using Pointers concept.
Concatenate using Pointer Functions
This program for string concatenation in c is the same as above. However, this time we are passing pointers to Functions to separate the logic from the main program.
1. What is String Concatenation?
String Concatenation is a process when one string is appended to the end of another string.
2. C Program to concatenate string without using strcat ()
Let’s discuss the execution (kind of pseudocode) for the program to concatenate string without using strcat () in C.
3. Conclusion
In this C Programming example, we have discussed what is String concatenation and how to concatenate string without using strcat () method.
C program to concatenate two strings without using strcat ()
In this tutorial, we will learn how to concatenate two strings without using strcat () function in C programming language. We will take the input from the user. User will enter both strings, our program will concatenate the strings and print out the result. To store the strings, we will use two arrays.
Explanation
Create two char array variables to store the strings. These variables are firstString and secondString. The size of these arrays are 100.
