
- Definition from WhatIs.com Java Comparator is an interface for sorting Java objects. Invoked by “java.util.comparator,” Java Comparator compares two Java objects in a “compare (Object 01, Object 02)” format. Using configurable methods, Java Comparator can compare objects to return an integer based on a positive, equal or negative comparison.
How to implement comparator in Java?
- Employee names are extracted into an empNames List as explained before the code snippet.
- empNames List is then sorted using the Comparator.naturalOrder () method which returns a Comparator instance of String ’s natural comparison order based on the empName ’s generic type of String.
- Employee names are sorted in natural comparison order, i.e. ...
How to use compareTo method in Java?
Java String compareTo() method. We have following two ways to use compareTo() method: int compareTo(String str) Here the comparison is between string literals. For example string1.compareTo(string2) where string1 and string2 are String literals. int compareTo(Object obj) Here the comparison is between a string and an object.
How to compare two object arrays in Java?
Two arrays object reference e1 and e2 are deeply equal if they hold any of the following condition:
- e1=e2
- equals (e2) returns true.
- If e1 and e2 are both the same primitive type the overloading of the method Arrays.equals (e1, e2) returns true.
- If e1 and e2 are both arrays of object reference types, the method Arrays.deepEquals (e1, e2) returns true.
What is compare method in Java?
Java String compareTo ()
- if s1 > s2, it returns positive number
- if s1 < s2, it returns negative number
- if s1 == s2, it returns 0
See more

What does equals mean in Java?
The equals ( ) method, shown here, tests whether an object equals the invoking comparator −. obj is the object to be tested for equality. The method returns true if obj and the invoking object are both Comparator objects and use the same ordering. Otherwise, it returns false.
What is obj in Java?
obj is the object to be tested for equality. The method returns true if obj and the invoking object are both Comparator objects and use the same ordering. Otherwise, it returns false. Overriding equals ( ) is unnecessary, and most simple comparators will not do so.
What is obj1 and obj2?
obj1 and obj2 are the objects to be compared. This method returns zero if the objects are equal. It returns a positive value if obj1 is greater than obj2. Otherwise, a negative value is returned. By overriding compare ( ), you can alter the way that objects are ordered.
What is a Java Comparator?
Java Comparator interface is used to order the objects of a user-defined class.
What is a comparator in Java 8?
Java 8 Comparator interface is a functional interface that contains only one abstract method. Now, we can use the Comparator interface as the assignment target for a lambda expression or method reference.
Which class provides comparison logic based on the name?
This class provides comparison logic based on the name. In such case, we are using the compareTo () method of String class, which internally provides the comparison logic.
1. When to Use Comparator Interface
Java Comparator interface imposes a total ordering on the objects which may not have a desired natural ordering.
2. Overriding compare () Method
To enable total ordering on objects, we need to create a class that implements the Comparator interface. Then we need to override its compare (T o1, T o2) method.
3. Using Comparator With
Use Collections.sort (list, Comparator) method sort a list of objects in order imposed by provided comparator instance.
5. Conclusion
In this tutorial, we learned about Comparator interface of Java collection framework. It helps in imposing a total order on objects without any change to the source code of that class.
What is a comparator in Java?
Comparator interface in Java is used to define the order of objects of the custom or user defined class.
What is the Comparator interface in Java?
The Comparator interface contains two methods, compare and equals.
What does the equals method return?
The equals method returns true if the specified object is equal to this comparator object.
Can you sort an object in ep class by salary?
It is not possible to sort the objects of the Emp class by salary in ascending order as well as by age in descending order if the Emp class implements the Comparable interface. It is because the class can have only one implementation of the comareTo method.
Why do we use a comparator?
The reason why your method takes a Comparator is so that the caller can choose how the strings should be compared.
Does String have a comparator?
Since the String class already implements the Comparable interface — a sort of sibling to Comparator that lets a class define its own comparison method — here's a handy generic class that lets you use any Comparable through the Comparator interface:
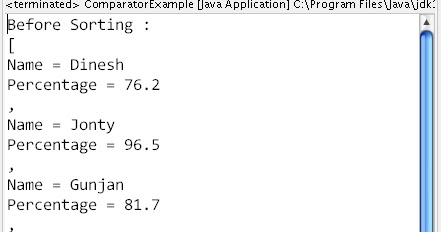