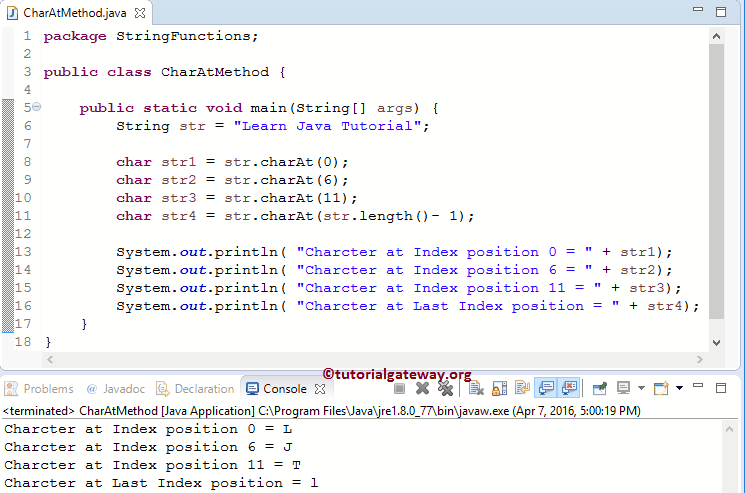
Output in Java
- System.out.println - This statement is used to print text or values in separate lines on the screen. This statement breaks the line after printing text or values on the screen.
- System.out.print - This statement is used to print text or values in the same line on the screen. This statement does not break the line after printing text or values on the screen.
- print(): This method in Java is used to display a text on the console. ...
- println(): This method in Java is also used to display a text on the console. ...
- printf(): This is the easiest of all methods as this is similar to printf in C.
What is input method in Java?
Sets the input method context, which is used to dispatch input method events to the client component and to request information from the client component. This method is called once immediately after instantiating this input method. Parameters:
How to take input from user in Java?
- import Scanner,
- make a new Scanner, and
- set it up so that it starts taking Input from the user.
What is an output stream in Java?
Output Stream consists of methods which perform:
- Write a byte to current Outputstream : public void write (int)throws IOException
- Write array of byte to current output stream : public void write (byte [])throws IOException
- Flushes the current OutputStream: public void flush ()throws IOException
- Close current Output Stream. : public void close ()throws IOException
How do you accept user input in Java?
How to accept input from keyboard in java?
- Using Scanner Class
- Using Console Class
- Using InputStreamReader and BufferedReader Class
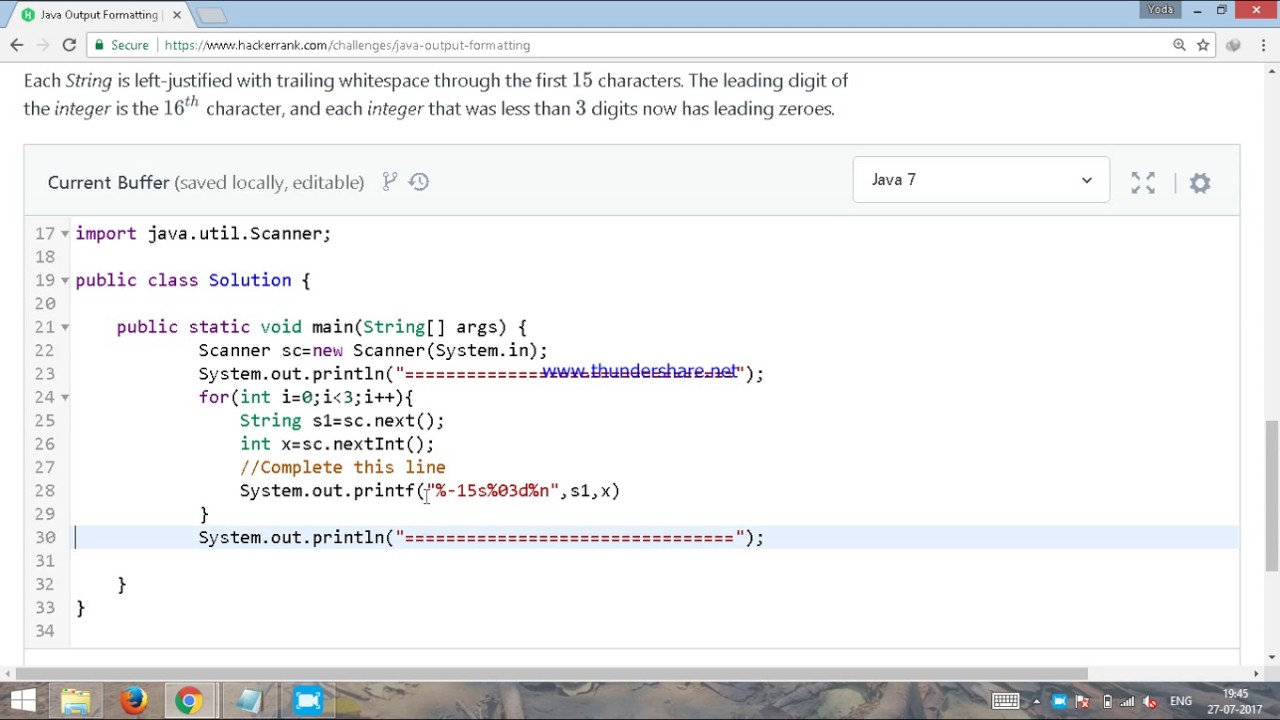
How do you write output in Java?
Let's take an example to output a line.class AssignmentOperator { public static void main(String[] args) { System.out.println("Java programming is interesting."); } } ... class Output { public static void main(String[] args) { System.out.println("1.More items...
What is output method in Java?
Java input and output is an essential concept while working on java programming. It consists of elements such as input, output and stream. The input is the data that we give to the program. The output is the data what we receive from the program in the form of result.
What is the command to print an output in Java?
println() is used to print an argument that is passed to it. The statement can be broken into 3 parts which can be understood separately as: System: It is a final class defined in the java. lang package. out: This is an instance of PrintStream type, which is a public and static member field of the System class.
How do you input and output a string in Java?
Example of next() methodimport java.util.*;class UserInputDemo2.{public static void main(String[] args){Scanner sc= new Scanner(System.in); //System.in is a standard input stream.System.out.print("Enter a string: ");String str= sc.next(); //reads string before the space.More items...
How do you input in Java?
Example of integer input from userimport java.util.*;class UserInputDemo.{public static void main(String[] args){Scanner sc= new Scanner(System.in); //System.in is a standard input stream.System.out.print("Enter first number- ");int a= sc.nextInt();More items...
What is file input and output in Java?
Java I/O (Input and Output) is used to process the input and produce the output. Java uses the concept of a stream to make I/O operation fast. The java.io package contains all the classes required for input and output operations. We can perform file handling in Java by Java I/O API.
How do you print a line in Java?
PrintStream. println() method prints an array of characters and then terminate the line. This method behaves as though it invokes print(char[]) and then println(). Using this method you can print the data on the console.
How do I print a code?
0:031:26Printing Code / Syntax in Visual Studio Code - YouTubeYouTubeStart of suggested clipEnd of suggested clipThere is information on how to do this but in short we're going to press the f1. Key then we'reMoreThere is information on how to do this but in short we're going to press the f1. Key then we're going to select your type print. Code.
What is System Out :: Println?
System. out. println is a Java statement that prints the argument passed, into the System. out which is generally stdout.
What are the 3 ways to input in Java?
In the Java program, there are 3 ways we can read input from the user in the command line environment to get user input, Java BufferedReader Class, Java Scanner Class, and Console class.
How do you display a string in Java?
The most basic way to display a string in a Java program is with the System. out. println() statement. This statement takes any strings and other variables inside the parentheses and displays them.
What are the methods of Java?
In Java, there are two types of methods: User-defined Methods: We can create our own method based on our requirements. Standard Library Methods: These are built-in methods in Java that are available to use.
What is StdOut Java?
The StdOut class provides methods for printing strings and numbers to standard output. Getting started. To use this class, you must have StdOut. class in your Java classpath. If you used our autoinstaller, you should be all set.
What is standard output Java?
The standard output in Java is the PrintStream class accessed through the System. out field. By default, standard output prints to the display, also called the console. PrintStream allows the printing of different data types converting all data to bytes. Two of its most useful methods include print and println methods.
What is a void method Java?
The void keyword specifies that a method should not have a return value.
Formatting output using System.out.printf ( ) Method
The implementation of this method is very easy as it is similar to the printf ( ) function in C programming.
Java String Format Specifiers
Here, we are providing a table of format specifiers supported by the Java String.
Example: print () and println ()
In the above example, we have shown the working of the print () and println () methods. To learn about the printf () method, visit Java printf ().
Example: Print Concatenated Strings
class PrintVariables { public static void main(String [] args) { Double number = -10.6; System.out.println ("I am " + "awesome."); System.out.println ("Number = " + number); } }
Example: Get Integer Input From the User
In the above example, we have created an object named input of the Scanner class. We then call the nextInt () method of the Scanner class to get an integer input from the user.
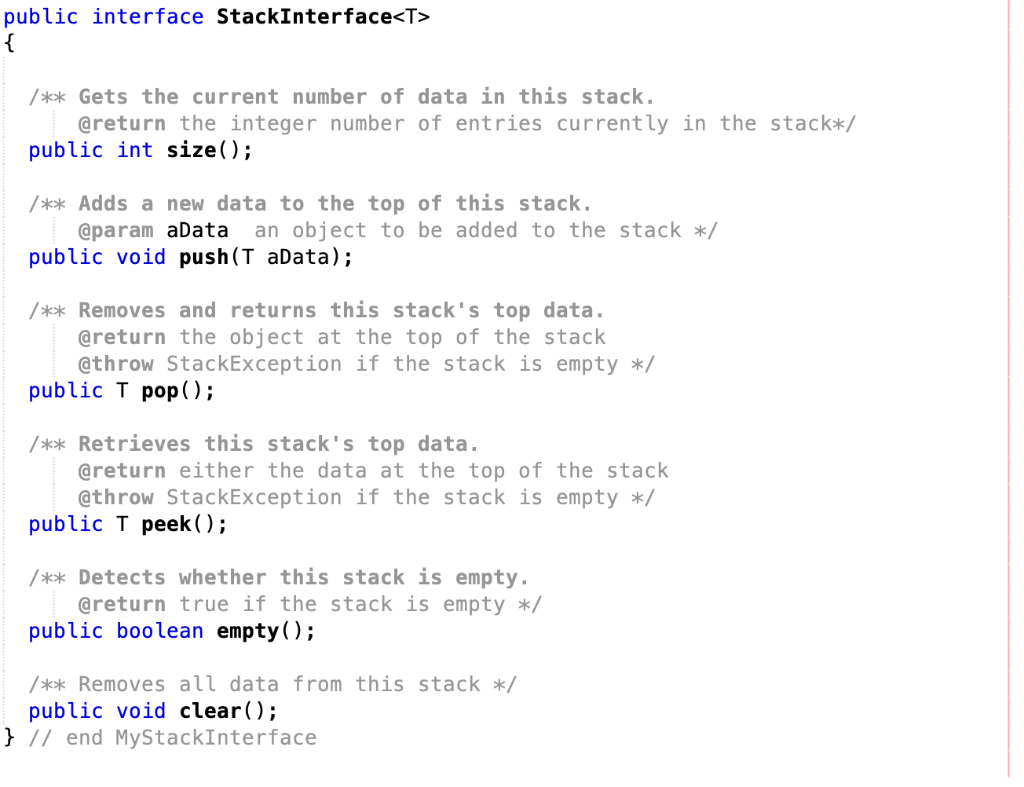