
Consider the below points about the power function in Java:
- It will return 1.0, if the second argument is positive or negative zero.
- It will return NaN, if the second argument is not a number.
- It will return the same result as the first argument, if the b (second argument) is 1.
...
PowerFunc1. java:
- public class PowerFunc1 {
- public static void main(String[] args)
- {
- double a = 5;
- double b = 2;
- System. out. println(Math. pow(a, b)); // return a^b i.e. 5^2.
- }
- }
How to create a process in Java?
Java.lang.ProcessBuilder class in Java. This class is used to create operating system processes. Each ProcessBuilder instance manages a collection of process attributes. The start () method creates a new Process instance with those attributes. The start () method can be invoked repeatedly from the same instance to create new subprocesses with ...
How to calculate power of a number in Java?
Java program to calculate the power of a number. Java Programming Java8 Object Oriented Programming. Read the base and exponent values from the user. Multiply the base number by itself and multiply the resultant with base (again) repeat this n times where n is the exponent value. 2 ^ 5 = 2 X 2 X 2 X 2 X 2 (5 times)
How to activate Java in the browser?
To enable/disable Java in the Safari browser:
- Select Safari -> Preferences from the menu toolbar.
- In the preferences, window click on the Security icon.
- Make sure the Enable Java checkbox is checked if you want Java enabled or unchecked if you want it disabled.
- Close the preferences window and the change will be saved.
How do you set path in Java?
- Open command prompt
- Copy the path of jdk/bin directory
- Write in command prompt:SET PATH=copied_path

How do you find the power value in Java?
In this program, we use Java's Math. pow() function to calculate the power of the given base . We can also compute the power of a negative number using the pow() method.
How do you write 10 to the power 9 in Java?
pow() to take a large exponent. Since 109 fits into an int and is also exactly representable as a double , you can do this: int exp = (int) Math. pow(10, 9); BigInteger answer = BigInteger.
How do you write a power of 2 in Java?
The java. lang. Math. pow() is used to calculate a number raise to the power of some other number.If the second parameter is positive or negative zero then the result will be 1.0.If the second parameter is 1.0 then the result will be same as that of the first parameter.More items...•
How do you type to the power of 2 in Java?
The power function in Java is Math. pow()....PowerFunc1. java:public class PowerFunc1 {public static void main(String[] args){double a = 5;double b = 2;System. out. println(Math. pow(a, b)); // return a^b i.e. 5^2.}}
How do you write a power of 10 in Java?
Math. pow(10, exponent)
How do you write a superscript in Java?
You can write text as superscript or subscript using the Chunk class, and it's setTextRise() method. You use a positive text rise value for superscript, and a negative text rise value for subscript.
How do you write 1e9 in Java?
1e9 simply means (1) * (10^9) Typecast 1e9 to int since by default is is double => (int)1e9.
How do you write scientific notation in Java?
The format of scientific notation in Java is exactly the same as you have learned and used in science and math classes. Remember that an uppercase 'E' or lowercase 'e' can be used to represent "10 to the power of". Scientific notation can be printed as output on the console if it passes a certain number.
What is a math.pow function?
The java.lang.Math .pow () is used to calculate a number raise to the power of some other number. This function accepts two parameters and returns the value of first parameter raised to the second parameter. There are some special cases as listed below:
What happens if the second parameter is 1.0?
If the second parameter is 1.0 then the result will be same as that of the first parameter.
What is the method to do exponents in Java?
Java provides an inbuilt method to directly do exponents i.e Math.pow (). The method simply accepts two parameters. Most importantly the two parameters must be of double type.
Can you use for loop in Java?
Basics are the building block for any programming language. So, we can simply use a for-loop and make our own logic in Java. Below is a Java program to do exponent of a number without Math.pow ().
What is a math.pow?
You can use Math.pow (value, power).
Is math.pow faster than multiplying?
I did the benchmarking with Math.pow (x,2) and x*x, the result is that Math.pow () is easily forty times slower than manually multiplying it by itself, so i don't recommend it for anything where a little bit of performance is required.
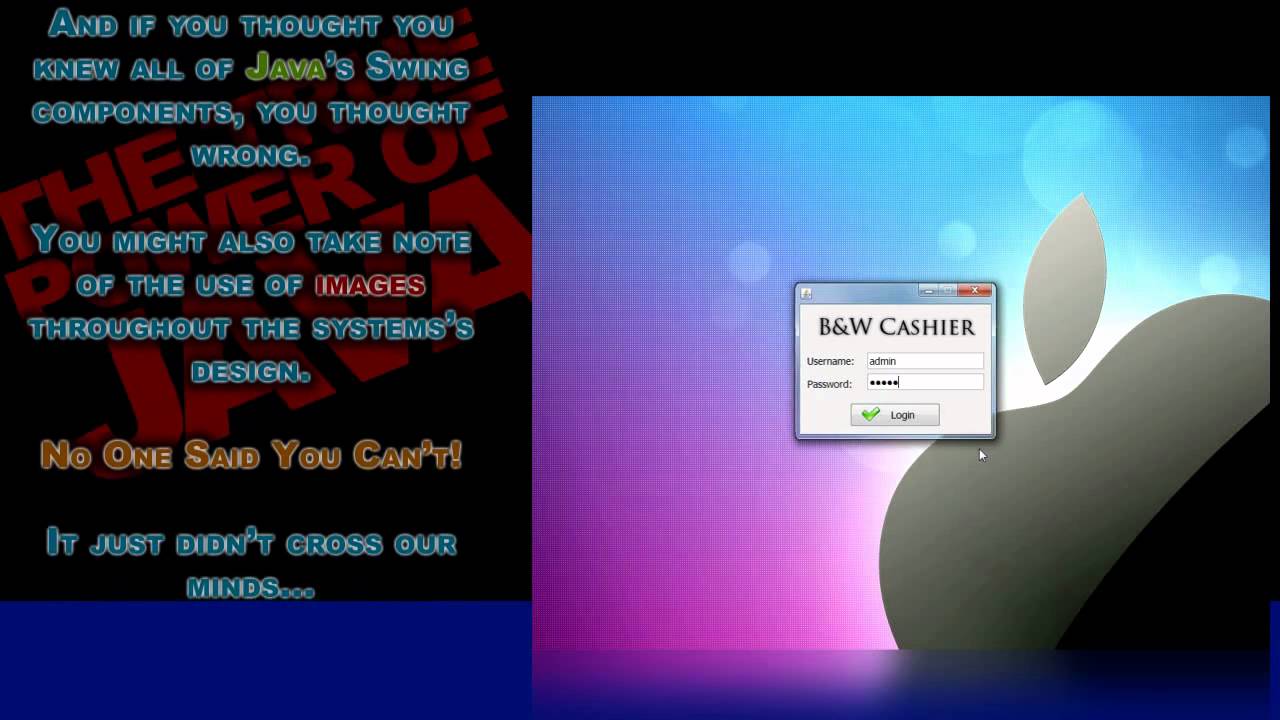