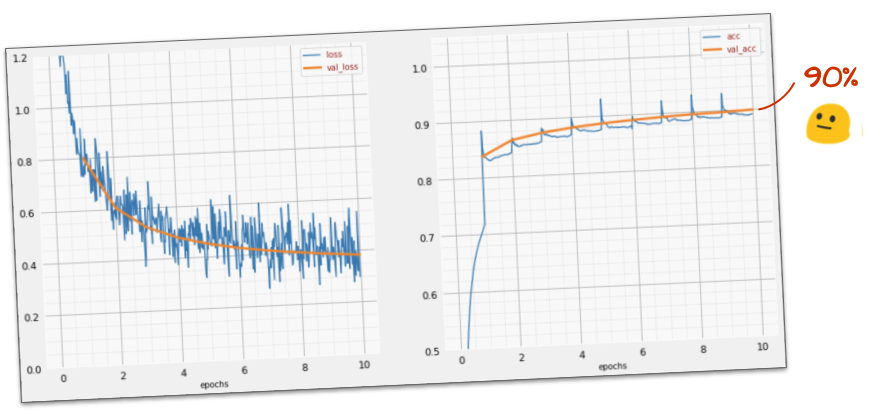
How to find shape of a tensor
- Step 1 - Import library import tensorflow as tf
- Step 2 - Take a Sample data Sample_data = tf.constant ( [ [ [1,1,4], [2,4,5]], [ [4,6,7], [8,9,6]]])
- Step 3 - Print the Shape print ("The Shape of Sample tensor is",tf.shape (Sample_data)) The Shape of Sample tensor is tf.Tensor ( [2 2 3], shape= (3,), dtype=int32)
How to find the dimension of a tensor in TensorFlow?
We begin from the outermost list to find out the tensor’s shape. By subtracting the first dimension then subtracting the next, we arrive at the tensor’s shape. Afterwards, we repeat this process for the inner lists and find their next dimensions. How Do You Find The Dimension Of A Tensor In Tensorflow?
How to get the shape of TensorFlow in Python?
Let us see how to get the shape of TensorFlow by using th e x.get_shape ().as_list () function in Python. To perform this particular task, we are going to use t he tensor.get_shape.as_list () function. This method returns a list of integer values that indicates the shape and the dimension of an array.
How do I reshape a tensor in TensorFlow?
# You can reshape a tensor to a new shape. The data maintains its layout in memory and a new tensor is created, with the requested shape, pointing to the same data. TensorFlow uses C-style "row-major" memory ordering, where incrementing the rightmost index corresponds to a single step in memory.
How to check if a shape is a tensor or not?
Either the shape contains a None (an axis-length is unknown) or the whole shape is None (the rank of the tensor is unknown). Except for tf.RaggedTensor, such shapes will only occur in the context of TensorFlow's symbolic, graph-building APIs: The keras functional API. To inspect a tf.Tensor 's data type use the Tensor.dtype property.
What is the function to find the shape of an image?
What is the dense layer in a neural network?
What is a Cifar10 dataset?
What is the first step in Keras?
See 1 more
About this website
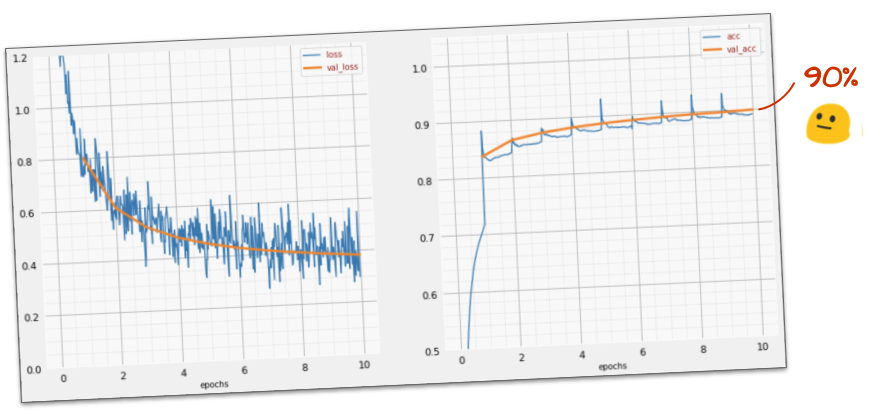
How do you determine the shape of a tensor?
shape() will return the shape of the symbolic tensor. In these cases, using tf. Tensor. shape will return more informative results.
What shape is my keras tensor?
1 AnswerIf you want to get a tensor shape you should use int_shape function from keras. backend .The first dimension is set to be a batch dimension so int_shape(y_true)[0] will return you a batch size. You should use int_shape(y_true)[1] .
What does the shape of a tensor mean?
A tensor is a vector or matrix of n-dimensions that represents all types of data. All values in a tensor hold identical data type with a known (or partially known) shape. The shape of the data is the dimensionality of the matrix or array. A tensor can be originated from the input data or the result of a computation.
How do you find the shape of a data set?
To get the shape of Pandas DataFrame, use DataFrame. shape. The shape property returns a tuple representing the dimensionality of the DataFrame. The format of shape would be (rows, columns).
How do you get the shape of a tensor in PyTorch?
To get the shape of a tensor as a list in PyTorch, we can use two approaches. One using the size() method and another by using the shape attribute of a tensor in PyTorch.
What is the input shape?
In a Keras layer, the input shape is generally the shape of the input data provided to the Keras model while training. The model cannot know the shape of the training data. The shape of other tensors(layers) is computed automatically.
What is the difference between tensor rank and shape?
So, Rank is defined as the number of dimensions of that tensor. And, Tensor Shape represents the size of the each dimension. A tensor with rank 0 is a zero-dimensional array. The element of a zero-dimensional array is a point.
What is size of a tensor?
The number of dimensions a tensor has is called its rank and the length in each dimension describes its shape . has a rank of 2 , a shape of [2, 3] and a length of 6.
What does a tensor look like?
2:1611:47What the HECK is a Tensor?!? - YouTubeYouTubeStart of suggested clipEnd of suggested clipInto three rows. And three columns each corresponds to a specific direction in three-dimensionalMoreInto three rows. And three columns each corresponds to a specific direction in three-dimensional space seriously it's that easy rank is the amount of information you need to find a specific component.
What is shape in Tensorflow?
Shape: The length (number of elements) of each of the axes of a tensor. Rank: Number of tensor axes. A scalar has rank 0, a vector has rank 1, a matrix is rank 2. Axis or Dimension: A particular dimension of a tensor. Size: The total number of items in the tensor, the product of the shape vector's elements.
What does shape () do in pandas?
shape attribute in Pandas enables us to obtain the shape of a DataFrame. For example, if a DataFrame has a shape of (80, 10) , this implies that the DataFrame is made up of 80 rows and 10 columns of data.
How do you determine the shape of skewness?
If the skewness is between -0.5 & 0.5, the data are nearly symmetrical. If the skewness is between -1 & -0.5 (negative skewed) or between 0.5 & 1(positive skewed), the data are slightly skewed. If the skewness is lower than -1 (negative skewed) or greater than 1 (positive skewed), the data are extremely skewed.
How do I check my Keras model weights?
How to get the weights of Keras model?layer. get_weights(): returns the weights of the layer as a list of Numpy arrays.layer. set_weights(weights): sets the weights of the layer from a list of Numpy arrays.
How do I find my Keras and TensorFlow version?
Check TensorFlow Version in Virtual EnvironmentStep 1: Activate Virtual Environment. To activate the virtual environment, use the appropriate command for your OS: For Linux, run: virtualenv
How do I know if my Keras model is using my GPU?
0:131:48How to check if TensorFlow or Keras is using GPU - YouTubeYouTubeStart of suggested clipEnd of suggested clipStart training your model and open task manager go to performance tab and look for GPU memoryMoreStart training your model and open task manager go to performance tab and look for GPU memory consumption and GPU utilization if your model is using GPU.
What is input shape Keras?
The input shape In Keras, the input layer itself is not a layer, but a tensor. It's the starting tensor you send to the first hidden layer. This tensor must have the same shape as your training data. Example: if you have 30 images of 50x50 pixels in RGB (3 channels), the shape of your input data is (30,50,50,3) .
How to determine input shape in keras? - Data Science Stack Exchange
The number of rows in your training data is not part of the input shape of the network because the training process feeds the network one sample per batch (or, more precisely, batch_size samples per batch).
TF Keras how to get expected input shape when loading a model?
Stack Overflow for Teams is moving to its own domain! When the migration is complete, you will access your Teams at stackoverflowteams.com, and they will no longer appear in the left sidebar on stackoverflow.com.. Check your email for updates.
Ultimate Guide to Input shape and Model Complexity in Neural Networks
Summary of the Deep neural network model. Since each input feature is connected to each neuron in the hidden layer, the total number of connections is the product of the input feature size (m) and the hidden layer size (n).
TensorFlow get shape
In this section, we will learn how to get the shape in TensorFlow Python.
TensorFlow get shape of dataset
In this section, we will learn how to get the shape of dataset in TensorFlow Python.
TensorFlow get shape as list
Here we will see how to get the TensorFlow tensor shape as a list in Python.
TensorFlow get shape none
In this section, we will learn how to get the none shape in TensorFlow Python.
TensorFlow get shape of placeholder
In this Program, we will learn how to get the shape of placeholder in TensorFlow Python.
What is the function to find the shape of an image?
We can use the “.shape” function to find the shape of the image. It returns a tuple of integers that represent the shape and color mode of the image.
What is the dense layer in a neural network?
The “Dense” layer is a deeply connected neural network layer . The “Dropout” layer is used to prevent the model from overfitting. The first layer is known as the input layer, the middle layers are called hidden layers, and the last layer is the output layer.
What is a Cifar10 dataset?
In this example, we will use the cifar10 dataset. This dataset is a collection of 60,000 colored images of size 32×32 and channel 3 (RGB). This dataset is used for object detection in machine learning. let’s create a Keras model that accepts (32,32,3) input shapes.
What is the first step in Keras?
The first step always is to import important libraries. We will be using the above libraries in our code to read the images and to determine the input shape for the Keras model.
How to inspect a TF.Tensor?
To inspect a tf.Tensor 's data type use the Tensor.dtype property.
What is a tensor array?
Tensors are multi-dimensional arrays with a uniform type (called a d type ). You can see all supported dtypes at tf.dtypes.DType.
What is a ragged tensor?
A tensor with variable numbers of elements along some axis is called "ragged". Use tf.ragged.RaggedTensor for ragged data.
How many axes does a matrix tensor have?
A "matrix" or "rank-2" tensor has two axes:
What happens when you flatten a tensor?
If you flatten a tensor you can see what order it is laid out in memory.
What is the meaning of the word "shape"?
Some vocabulary: Shape: The length (number of elements) of each of the axes of a tensor. Rank: Number of tensor axes. A scalar has rank 0, a vector has rank 1, a matrix is rank 2. Axis or Dimension: A particular dimension of a tensor. Size: The total number of items in the tensor, the product shape vector.
What is a scalar tensor?
Here is a "scalar" or "rank-0" tensor . A scalar contains a single value, and no "axes".
What is the function to find the shape of an image?
We can use the “.shape” function to find the shape of the image. It returns a tuple of integers that represent the shape and color mode of the image.
What is the dense layer in a neural network?
The “Dense” layer is a deeply connected neural network layer . The “Dropout” layer is used to prevent the model from overfitting. The first layer is known as the input layer, the middle layers are called hidden layers, and the last layer is the output layer.
What is a Cifar10 dataset?
In this example, we will use the cifar10 dataset. This dataset is a collection of 60,000 colored images of size 32×32 and channel 3 (RGB). This dataset is used for object detection in machine learning. let’s create a Keras model that accepts (32,32,3) input shapes.
What is the first step in Keras?
The first step always is to import important libraries. We will be using the above libraries in our code to read the images and to determine the input shape for the Keras model.