
The steps for making a deep copy using serialization are:
- Ensure that all classes in the object's graph are serializable.
- Create input and output streams.
- Use the input and output streams to create object input and object output streams.
- Pass the object that you want to copy to the object output stream.
Full Answer
How do you make a deep copy of an object?
Copy Constructors are the easiest way to make a deep copy. We simply create a constructor which takes an object of the same class as input and returns a new object with the same values but new references (if referenced classes are involved).
When do we need to do a deep copy?
In general, if the variables of an object have been dynamically allocated then it is required to do a Deep Copy in order to create a copy of the object.
Is it possible to make a deep copy of a class?
Serialization is not always the preferred way of making a deep copy as it is a lot more expensive than the clone () method and not all classes are serializable. The complete code is shown below.
What is a deep copy in JavaScript?
So when the copy is mutated, the original also gets mutated. This is also known as shallow copying. If we instead want to copy an object so that we can modify it without affecting the original object, we need to make a deep copy . In JavaScript, we can perform a copy on objects using the following methods:

Introduction
While creating a copy of Java objects, we have the following two options for creating a copy
1. Deep Copy
Deep copy technique copies each mutable object in the object graph recursively. The object created through deep copy is not dependent upon the original object. We will cover few different options to make a deep copy of an object in Java.
2. Deep Copy Using External Libraries
Java build in mechanism provided a good support for the deep copy of an object, however, we can have a certain limitation
Summary
In this post, we discussed how to make a deep copy of an object in Java. We covered different options for creating a deep copy of the object and why to prefer a deep copy over a shallow copy.
Shallow Copy
In shallow copy, an object is created by simply copying the data of all variables of the original object. This works well if none of the variables of the object are defined in the heap section of memory .
Deep Copy
In Deep copy, an object is created by copying data of all variables and it also allocates similar memory resources with the same value to the object. In order to perform Deep copy, we need to explicitly define the copy constructor and assign dynamic memory as well if required.
1. Introduction
When we want to copy an object in Java, there are two possibilities that we need to consider, a shallow copy and a deep copy.
Java Copy Constructor
Here's how to create copy constructors in Java and why to implementing Cloneable isn't such a great idea.
2. Maven Setup
We'll use three Maven dependencies, Gson, Jackson, and Apache Commons Lang, to test different ways of performing a deep copy.
3. Model
To compare different methods of copying Java objects, we'll need two classes to work on:
4. Shallow Copy
A shallow copy is one in which we only copy values of fields from one object to another:
5. Deep Copy
A deep copy is an alternative that solves this problem. Its advantage is that each mutable object in the object graph is recursively copied.
6. External Libraries
The above examples look easy, but sometimes they don't work as a solution when we can't add an additional constructor or override the clone method.
What is Shallow Copy?
A shallow copy will simply copy the values of the fields of the original object into the new object. In shallow copy, if the original object has any references to other class objects (a class member is itself an object of some other class), then only the reference of that object is cloned.
What is Deep Copy?
A deep copy will create a new object for the referenced objects by the original class. This ensures that the original object and the cloned object are independent. For primitive data types, shallow cloning and deep cloning work in the same way as no references are involved.
Deep Copy using Serialization
Serialization is another simple way of performing a deep copy. S erializing an object and then deserializing it essentially produces a new object with new references but the same values. This will return a new object which is a deep copy of the original one. The object classes that need to be cloned must implement the Serializable interface.
Summary
A shallow copy will just copy the field values from the original object into the new object but we may get unexcepted results if the original object contains references to other objects. To solve this problem we use Deep Copy. A shallow copy and a deep copy will give the same results if the object only uses primitive fields.
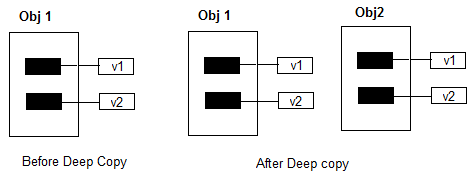