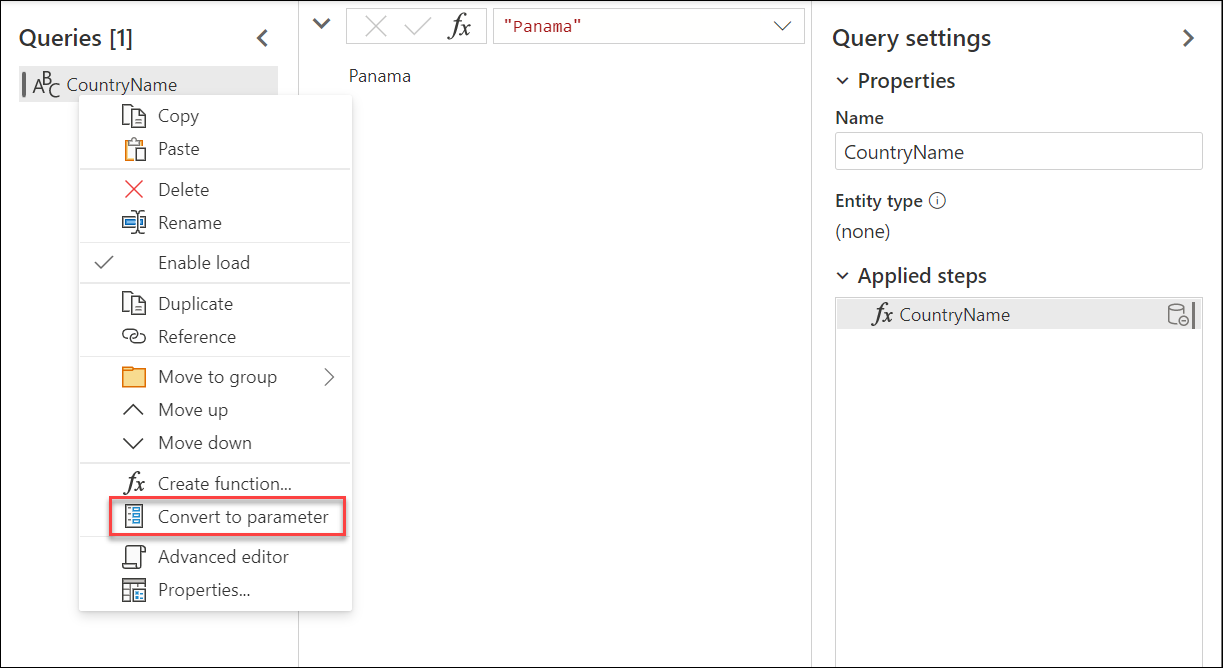
- By using default value: You can implement optional parameters by using default value. ...
- By using Method Overloading: You can implement optional parameters concept by using method overloading. ...
- By using OptionalAttribute: You can implement optional parameters by using OptionalAttribute. ...
How to make method parameters optional in C #?
In this article, you will learn about how to make method parameters optional in C#. There are 4 ways we can make method parameters optional and they are given below. Using method overloading. Using parameter arrays. Specify parameter defaults.
How do you make a parameter optional in Python?
We can implement optional parameters by assigning a default value for the parameters. This is the easiest and simple way to make the methods parameter optional. In this way, we just need to define the optional parameter with its default values when we create our methods.
How to pass parameter with specific names for the optional parameters?
I am passing a parameter with the specific names for the optional parameters by using the name of the parameter colon and the value (example, c:2). You can make function parameter optional, using [OptionalAttribute] or [Optional] in front of method parameter by adding namespace, using System.wmRuntime.InteropServices; as shown below.
How do I use a default parameter?
You can also use a default parameter value, by using the equals sign ( = ). If we call the function without an argument, it uses the default value ("Norway"): A parameter with a default value, is often known as an " optional parameter ". From the example above, country is an optional parameter and "Norway" is the default value.

How do you make a parameter optional?
We can make a parameter optional by assigning default values for that parameter, like:public static void Sum(int a,int b , int[] n=null){int res=a+b;if(n!= null){foreach(int no in n){res+=no;More items...•
How do you declare an optional parameter in a function?
To declare optional function parameters in JavaScript, there are two approaches: Using the Logical OR operator ('||'): In this approach, the optional parameter is Logically ORed with the default value within the body of the function. Note: The optional parameters should always come at the end on the parameter list.
How do you define optional parameters?
Optional parameters are defined at the end of the parameter list, after any required parameters. If the caller provides an argument for any one of a succession of optional parameters, it must provide arguments for all preceding optional parameters. Comma-separated gaps in the argument list aren't supported.
Can out parameter be optional?
When a method with an omitted optional parameter is called, a stack frame containing the values of all the parameters is created, and the missing value(s) are simply filled with the specified default values. However, an "out" parameter is a reference, not a value.
How do you add optional parameters in react?
To add in an optional path parameter in React Router, you just need to add a ? to the end of the parameter to signify that it is optional. And then in your component you could access the GET parameters using the location.search prop that is passed in by React Router.
How do you make a parameter?
Creating a parameter is similar to adding a normal criterion to a query:Create a select query, and then open the query in Design view.In the Criteria row of the field you want to apply a parameter to, enter the text that you want to display in the parameter box, enclosed in square brackets.More items...
What is optional parameter in C?
A method that contains optional parameters does not force to pass arguments at calling time. It means we call method without passing the arguments. The optional parameter contains a default value in function definition. If we do not pass optional argument value at calling time, the default value is used.
What is named and optional parameters in C#?
Microsoft introduced support for named and optional parameters in C# 4.0. While a named parameter is used to specify an argument based on the name of the argument and not the position, an optional parameter can be used to omit one or more parameters in the method signature.
What are the differences between mandatory parameters and optional parameters?
The main difference between required parameters and optional parameters is that mandatory parameters must be provided when the function is called, while optional parameters automatically supplies the value if it's not provided.
Can we have multiple optional parameters in C#?
C# Multiple Optional Parameters If you want to define multiple optional parameters, then the method declaration must be as shown below. The defined GetDetails() method can be accessed as shown below. GetDetails(1); GetDetails(1, "Rohini");
Which kind of parameters Cannot have a default?
An IN OUT parameter cannot have a default value. An IN OUT actual parameter or argument must be a variable.
How do I send optional parameters in REST API?
You can then use @DefaultValue if you need it: @GET @Path("/job/{param1}/{param2}") public Response method(@PathParam("param1") String param1, @PathParam("param2") String param2, @QueryParam("optional1") String optional1, @QueryParam("optional2") @DefaultValue("default") String optional2) { ... }
How do you make an optional parameter in Java?
Use Varargs to Have Optional Parameters in Java In Java, Varargs (variable-length arguments) allows the method to accept zero or multiple arguments. The use of this particular approach is not recommended as it creates maintenance problems.
How do I declare optional parameters in typescript?
In Typescript, making optional parameters is done by appending the “?” at the end of the parameter name in the function when declaring the parameters and the parameters which are not marked with “?” i.e not optional parameter are called as default parameters or normal parameters where it is must and compulsory to pass ...
Are function parameters optional?
Optional parameters are great for simplifying code, and hiding advanced but not-often-used functionality. If majority of the time you are calling a function using the same values for some parameters, you should try making those parameters optional to avoid repetition.
What is optional argument in Python?
In Python, when we define functions with default values for certain parameters, it is said to have its arguments set as an option for the user. Users can either pass their values or can pretend the function to use theirs default values which are specified.
Named arguments
Named arguments free you from matching the order of parameters in the parameter lists of called methods. The parameter for each argument can be specified by parameter name.
Optional arguments
The definition of a method, constructor, indexer, or delegate can specify its parameters are required or optional. Any call must provide arguments for all required parameters, but can omit arguments for optional parameters.
COM interfaces
Named and optional arguments, along with support for dynamic objects, greatly improve interoperability with COM APIs, such as Office Automation APIs.
Overload resolution
A method, indexer, or constructor is a candidate for execution if each of its parameters either is optional or corresponds, by name or by position, to a single argument in the calling statement, and that argument can be converted to the type of the parameter.
Default Parameter Value
You can also use a default parameter value, by using the equals sign ( = ).
Example
A parameter with a default value, is often known as an " optional parameter ". From the example above, country is an optional parameter and "Norway" is the default value.
Function and Optional Parameters
When we write a program, we assign the default arguments to a function, which are also known as optional parameters if we call the function with the default parameter without passing any argument to it. It will use the default parameters we assigned to it at the beginning of the program.
Multiple Optional Parameters
You can write multiple optional parameters in a program. Here is a sample of how you can write more than one parameter in a program.
Optional Parameters and Overloading
Method overloading can be used to provide the concepts of optional arguments. We generate methods with the same name but a different argument list when we use method overloading. This approach is not the only way to implement an optional parameter, but it is a good starting point.
Advantages of Optional Parameters
An optional parameter is a value specified in a function declaration that is assigned by the compiler if the function’s caller does not give a value for the argument with a default value.
Dis-advantages of Optional Parameters
You can’t re-define a default parameter after it’s been supplied in a declaration or definition, even if it’s the same value. You can, however, add default parameters that were not specified in prior declarations.
Conclusion
In the above article, we have seen the concept of optional parameters in C++. You have seen what optional parameters in C++ are, why the optional parameter is used, and how to implement multi-optional parameters. Here is a quick review of what we have learned in this article:
