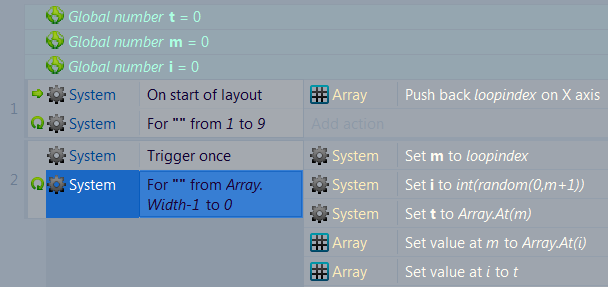
What is the shuffle algorithm?
What is an array in JavaScript?
Does JavaScript shuffle arrays?

How do you randomly shuffle an array in Python?
random. shuffle(x) , the array will be shuffled along the first axis as desired. However, using random. shuffle(x) will cause repetitions.
How do you randomly shuffle an array in CPP?
Shuffle an Array using STL in C++ STL contains two methods which can be used to get a shuffled array. These are namely shuffle() and random_shuffle(). This method rearranges the elements in the range [first, last) randomly, using g as a uniform random number generator.
Can we randomize a array?
In a fixed size array, randomization is possible only for the array elements. as the size is fixed, it is not possible to change.
What is random shuffle in C++?
C++ Algorithm random_shuffle() reorders the elements of a range by putting them at random places. The first version uses an internal random number generator and the second version uses a random number generator which is a special kind of function object that is explicitly passed as an argument.
How do you shuffle data in C++?
C++ Algorithm shuffle() function reorders the elements of a range by putting them at random places, using g as uniform random number generator....Example 2#include
How do you randomly shuffle a linked list in C++?
The safest way to shuffle a linked list is copy the data to an array, shuffle the array, and then copy the results of the array back to your list. This is assuming you have functions that goes through your list, and functions to copy data back to your list.
How do you shuffle a set in C++?
You can use std::shuffle to randomize the order of elements of pretty much any ordered structure. You can use this on an std::string , which would rearrange the individual characters.
How would you randomize a list's items in place?
The method shuffle() can be used to randomize the items of a list in place.
What library does shuffle in math?
Not really an answer to the original question, but MathArrays.shuffle from the commons-math3 library does the job.
Which class has an efficient method for shuffling, that can be copied, so as not to depend on it?
Collectionsclass has an efficient method for shuffling, that can be copied, so as not to depend on it:
Which is faster, an array or an object array?
As you can see below, an array is faster than a list, and a primitive array is faster than an object array.
Can you use arrays.asList() on a primitive array?
You can't use Arrays.asList()on a primitive array. And you wouldn't need to convert it back because it's just a wrapper.
What happens if you don't pass random generating function?
If we don’t pass our random generating function in random_shuffle then it uses its unspecified random values due to which all successive values are correlated.
How does g random number generator work?
This method rearranges the elements in the range [first, last) randomly, using g as a uniform random number generator. It swaps the value of each element with that of some other randomly picked element. It determines the element picked by calling g ().#N#Template
What is the shuffle algorithm?
Shuffling an array of values is considered one of the oldest problems in computer science. Shuffling is possible with the Fisher-Yates shuffle algorithm for generating a random permutation of a finite sequence. That is to say, the algorithm shuffles the sequence.
What is an array in JavaScript?
The JavaScript array class is used in the construction of arrays, which are high-level and list-like objects. Arrays can be used for storing several values in a single variable. An array can be described as a unique variable that is capable of holding more than one value at the same time.
Does JavaScript shuffle arrays?
In contrary to languages like Ruby and PHP, JavaScript does not have an built-in method of shuffling the array. However, there is a method that allows shuffling the sequence. Let’s discuss a particular case.
