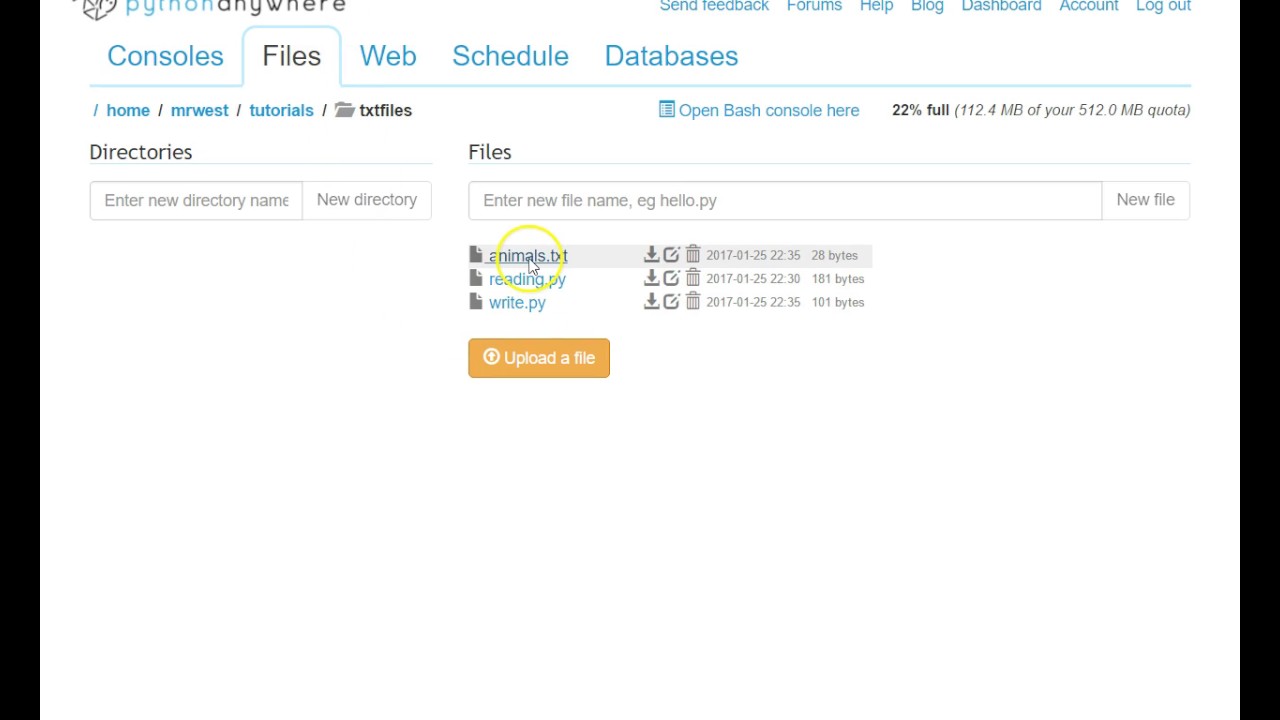
How to split text into multiple lines?
Split a Text Cell Into Rows
- First, separate values from cell B1 into columns based on a delimiter. Select a text cell (B1) and in the Ribbon, go to Data > Text to Columns.
- In Step 1 of the Text to Columns Wizard, leave the default file type (Delimited), and click Next.
- In Step 2, check Semicolon under Delimiters, and click Next. ...
How do you split in Python?
Python Server Side Programming Programming. The easiest way to split list into equal sized chunks is to use a slice operator successively and shifting initial and final position by a fixed number. In following example, a list with 12 elements is present. We split it into 3 lists each of length 4.
How do I use split in Python?
- First, the input string is split at ‘@’ with the default value for max split, i.e. -1. It means all the possible splits are returned.
- The string is then split at ‘@’ with different max_splits values, i.e. 0, 1, 2, 3, 4, 5.
- The same program can be written in a concise manner using for loop.
How to paste multiple lines of text into Python input?
The Best Solution for "How to paste multiple lines of text into python input [duplicate]" : raw_input accepts any input up until a new line character is entered . The easiest way to do what you are asking it to accept more entries, until an end of file is encountered.
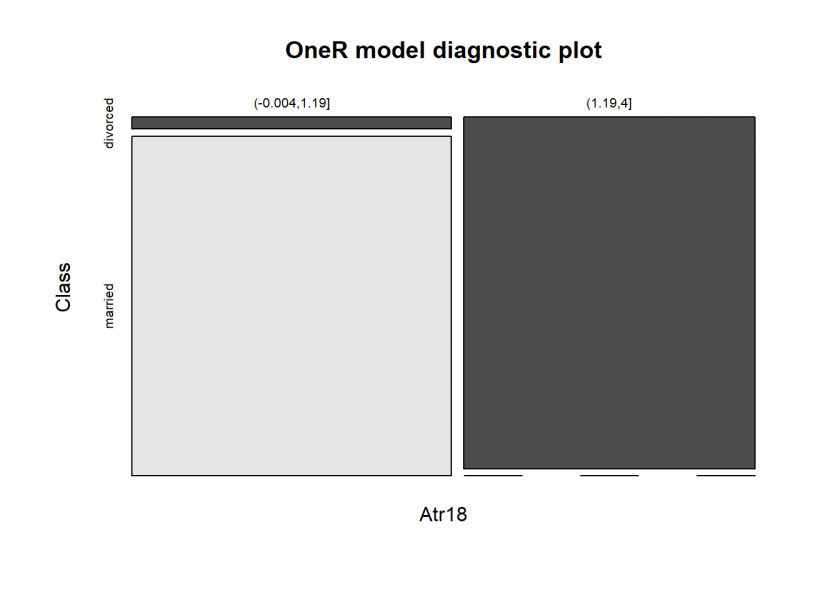
How do you split a line into two parts in Python?
Use Split () Function This function splits the string into smaller sections. This is the opposite of merging many strings into one. The split () function contains two parameters. In the first parameter, we pass the symbol that is used for the split.
How do you split a line break in Python?
Python String splitlines() method is used to split the lines at line boundaries. The function returns a list of lines in the string, including the line break(optional). Parameters: keepends (optional): When set to True line breaks are included in the resulting list.
How do I split a line in a file?
To split a file into pieces, you simply use the split command. By default, the split command uses a very simple naming scheme. The file chunks will be named xaa, xab, xac, etc., and, presumably, if you break up a file that is sufficiently large, you might even get chunks named xza and xzz.
How do I split a text file?
How to split a TXT document onlineSelect and upload your TXT document for splitting.Specify desired page numbers and click Split Now button.Once your TXT document is splitted click on Download Now button.Use Email button to send download link over email.
How do I split a line in a string?
Split a string on newlines in C#Using String. Split() method. The standard way to split a string in C# is using the String.Split() method. It splits a string into substrings and returns a string array. ... Using Regex. Split() method. Alternatively, we can split a string using a regular expression.
How do you break a line through a string?
In Windows, a new line is denoted using “\r\n”, sometimes called a Carriage Return and Line Feed, or CRLF. Adding a new line in Java is as simple as including “\n” , “\r”, or “\r\n” at the end of our string.
How do you split data in Python?
The split() method splits a string into a list. You can specify the separator, default separator is any whitespace. Note: When maxsplit is specified, the list will contain the specified number of elements plus one.
How does split function work in Python?
The split() function works by scanning the given string or line based on the separator passed as the parameter to the split() function. In case the separator is not passed as a parameter to the split() function, the white spaces in the given string or line are considered as the separator by the split() function.
How do you read and split a file in Python?
Use open with readline() or readlines() . The former will return a line at a time, while the latter returns a list of the lines. Use split(delimiter) to split on the comma. Lastly, you need to cast each item to an integer: int(foo) .
What is use of split command?
Split command in Linux is used to split large files into smaller files. It splits the files into 1000 lines per file(by default) and even allows users to change the number of lines as per requirement.
How do you split a file into chunks in Python?
To split a big binary file in multiple files, you should first read the file by the size of chunk you want to create, then write that chunk to a file, read the next chunk and repeat until you reach the end of original file.
How do I read a delimited text file in Python?
The solutions below contains 5 main steps:Step 1: Open the text file using the open() function. ... Read through the file one line at a time using a for loop.Split the line into an array. ... Output the content of each field using the print method.Once the for loop is completed, close the file using the close() method.
What does '\ r mean in Python?
In Python strings, the backslash "\" is a special character, also called the "escape" character. It is used in representing certain whitespace characters: "\t" is a tab, "\n" is a newline, and "\r" is a carriage return.
How do you print on separate lines in Python?
The new line character in Python is \n . It is used to indicate the end of a line of text. You can print strings without adding a new line with end =
What is string in Python?
String in Python can comprise of numeric or alphanumeric text, and it is usually used to store data directories or print messages. The string.split () function is generally used to manipulate the string.
What does maxsplit do?
maxsplit: It is a number, which tells us to split the string into a maximum of the provided number of times. If it is not provided, then there is no limit.
How to read a file in Python?
To read a file in Python, use the with statement with the open () method.
How to join two strings in Python?
To join two strings in Python, use the concatenation. To split the strings in Python, use the split () method.
What happens if a sep is not specified?
If the sep is not specified or specified as None, the result will be an empty list.
Can you split lines with a special symbol?
If your input line contains a special symbol like @ or &, you can split the line from that symbol, bypassing that as a separator.
How to split a string with multiple delimiters?
The given string or line with multiple delimiters is separated using a split function called re.split () function.
How does split work?
The split () function works by scanning the given string or line based on the separator passed as the parameter to the split () function.
How to split a string in a list?
A Split () Function Can Be Used in Several Ways. They Are: 1 Splitting the string based on the delimiter space 2 Splitting the string based on the first occurrence of a character 3 Splitting the given file into a list 4 Splitting the string based on the delimiter new line character 5 Splitting the string based on the delimiter tab 6 Splitting the string based on the delimiter comma 7 Splitting the string based on multiple delimiters 8 Splitting the string into a list 9 Splitting the string based on the delimiter hash 10 Splitting the string by passing maxsplit parameter 11 Splitting the string into a character array 12 Splitting the string based on one of the substrings from the given string as the delimiter
What is the name of the function that splits a string into a list?
The given string or line can be split into a list consisting of each character as the elements of the list using the split function called list () function.
How to specify the maximum number of splits a split function can perform on a given string?
The maximum number of splits that a split () function can perform on a given string or line can be specified using the maxsplit parameter and passed as an argument to the split () function.
How to separate a string with a tab?
The given string or line is separated using the split () function with a tab as the delimiter.
What is the function for manipulating strings in Python?
Manipulation of strings is necessary for all of the programs dealing with strings. In such cases, you need to make use of a function called split () function in Python.
How to extract substrings from strings?
We can combine the index () function with slice notation to extract a substring from a string. The index () function will give us the start and end locations of the substring. Once we know the location of the symbols ( $ ’s in this case), we’ll extract the string using slice notation.
What is slice notation in Python?
When it comes to splitting strings, slice notation is an obvious choice for Python developers. With slice notation, we can find a subsection of a string.
What are the parameters of a slice in Python?
Slice objects take three parameters: start, stop and step. The first two parameters tell Python where to start and end the slice, while the step parameter describes the increment between each step.
What library does Python use?
Python comes with the re library. With regex, we can search text with a fine tooth comb, looking for specific words, phrases, or even words of a certain length.
What is the function for splitting strings in Python?
The Python standard library comes with a function for splitting strings: the split () function. This function can be used to split strings between characters. The split () function takes two parameters. The first is called the separator and it determines which character is used to split the string.
What is split function?
With the split () function, we can split strings into substrings.
What is the function used to separate strings between characters?
If we have a text that is divided by multiple identical characters, we can use the split () function to separate the strings between the characters.
