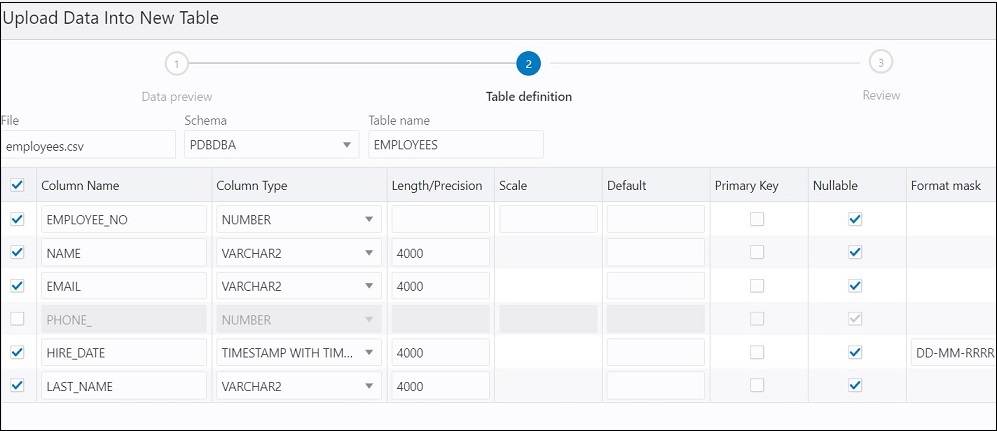
How do you update a specific column value in SQL?
- First, specify the table name that you want to change data in the UPDATE clause.
- Second, assign a new value for the column that you want to update. ...
- Third, specify which rows you want to update in the WHERE clause.
How do you add column values in SQL?
Here’s the syntax to add columns in SQL. ALTER TABLE table_name. ADD col_1_name data_type. col_2_name data_type … col_n_name data_type; Now that you know the syntax to add columns in SQL, use it to add two columns, ‘E_Address’ and ‘E_Salary,’ to your already existing ‘Employee’ table. ALTER TABLE Employee. ADD E_Address NVARCHAR(30),
How to change a value in SQL?
- Create a Database.
- Create a Table in the database, and Insert the data into the table.
- Show the table before value is updated.
- Change the value of a column in the table.
- Show the table after value is updated.
How to sum values of a column in SQL?
Sum of all values in a column:
- For this, we need to use the sum () function. We have to pass the column name as a parameter.
- This sum () function can be used with the SELECT query for retrieving data from the table.
- The below example shows to find the sum of all values in a column.
How to add column after another column in SQL?
- PostgreSQL ADD COLUMN
- PostgreSQL ADD COLUMN after another column
- PostgreSQL ADD COLUMN at position
- PostgreSQL ADD COLUMN with default value
- PostgreSQL ADD COLUMN integer default value
- PostgreSQL ADD COLUMN boolean
- PostgreSQL ADD COLUMN float
- PostgreSQL ADD COLUMN bigint
- PostgreSQL add calculated column
- PostgreSQL ADD COLUMN datetime
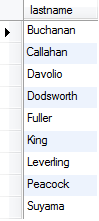
How do you update a specific column?
The Syntax for SQL UPDATE Command WHERE [condition]; The UPDATE statement lets the database system know that you wish to update the records for the table specified in the table_name parameter. The columns that you want to modify are listed after the SET statement and are equated to their new updated values.
How do you update a column value in SQL based on another column?
UPDATE table SET col = new_value WHERE col = old_value AND other_col = some_other_value; UPDATE table SET col = new_value WHERE col = old_value OR other_col = some_other_value; As you can see, you can expand the WHERE clause as much as you'd like in order to filter down the rows for updating to what you need.
How update same column with different values in SQL Server?
The UPDATE statement in SQL is used to update the data of an existing table in database. We can update single columns as well as multiple columns using UPDATE statement as per our requirement. UPDATE table_name SET column1 = value1, column2 = value2,...
How do I update a column in MySQL?
MySQL UPDATEFirst, specify the name of the table that you want to update data after the UPDATE keyword.Second, specify which column you want to update and the new value in the SET clause. ... Third, specify which rows to be updated using a condition in the WHERE clause.
Step 1: Create a Database
In the structured query language, database creation is the first step for storing the structured tables in the database.
Step 2: Create a Table and Insert the data
After database creation, you have to use the following syntax to create the table:
Step 3: View the Table before updating the values
After table creation and data insertion, you can view the inserted data of the Bikes table by typing the following query in your SQL application:
Step 4: Change the value of a particular column in the table
If you want to change the Color of any bike, you have to type the following query in SQL:
Step 5: View the Table after updating the values
To check the result of the query executed in the 4th step, you have to type the following SELECT command in SQL:
Change the value of Multiple Columns in the table
If you want to update the values of multiple columns in the Bikes table, then you have to write the below query in SQL:
SQL UPDATE one column example
Suppose Janet, who has employee id 3, gets married so that you need to change her last name in the employees table.
SQL UPDATE multiple columns
For example, Janet moved to a new house, therefore, her address changed. Now, you have to change it in the employees table by using the following statement:
SQL UPDATE multiple rows
The following UPDATE statement increases the salary by 2% for employees whose salary is less than $2000:
SQL UPDATE from SELECT
The following query selects sales person who has was in charge of more than 100 orders:
SQL UPDATE one row example
Suppose the employee id 192 Sarah Bell changed her last name from Bell to Lopez and you need to update her record in the employees table.
SQL UPDATE multiple rows example
Now, Nancy wants to change all her children’s last names from Bell to Lopez. In this case, you need to update all Nancy’s dependents in the dependents table.
SQL UPDATE with subquery example
Sometimes when employees change their last names, you update the employees table only without updating the dependents table.
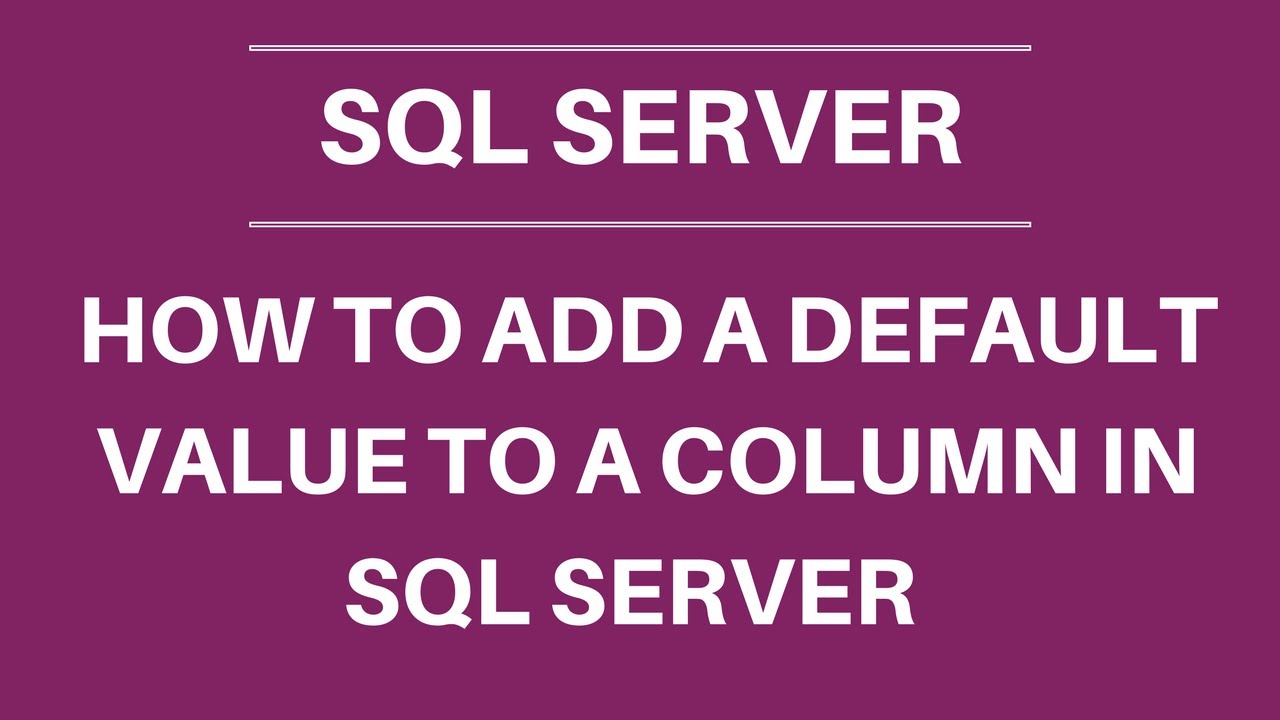