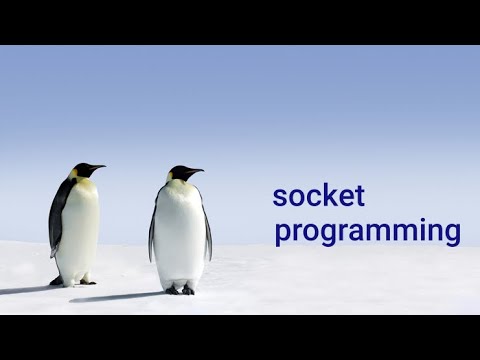
Server will get so many hits from different clients, and then server has to identify each client uniquely to reply every request. To achieve this we use “ip address of client (32 bit) + port number (16 bit) of the process”. This is called Socket (48 bit).
What is a socket in a server?
Server will get so many hits from different clients, and then server has to identify each client uniquely to reply every request. To achieve this we use “ip address of client (32 bit) + port number (16 bit) of the process”. This is called Socket (48 bit). Any network communication should goes through socket.
How do I know which socket a client is coming from?
Call getpeername on the accepted socket to see which client port the connection is coming from. If two clients connect to your server, you now have three sockets -- the original listening socket, and the sockets of each of the two clients. You can have many clients connected to the same server port 8000 at the same time.
How many sockets can be connected to a server at once?
If two clients connect to your server, you now have three sockets -- the original listening socket, and the sockets of each of the two clients. You can have many clients connected to the same server port 8000 at the same time. And, many clients can be connected from the same client port (e.g. port 12345), only not from the same IP address.
How can I tell the difference between two client ports?
And, many clients can be connected from the same client port (e.g. port 12345), only not from the same IP address. From the same client IP address, e.g. 10.0.0.2, each client connection to the server port 8000 will be from a unique client port, e.g. 12345, 12377, etc. You can tell the clients apart by their combination of IP address and port.
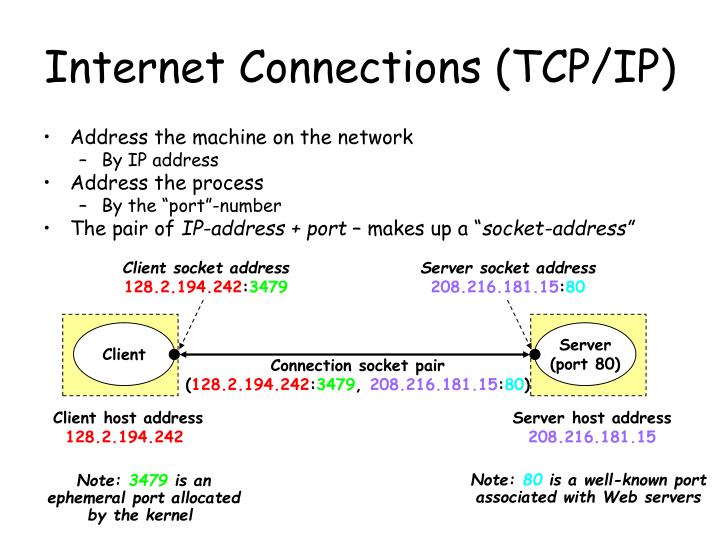
How do I find client IP address in socket programming?
OK assuming you are using IPV4 then do the following: struct sockaddr_in* pV4Addr = (struct sockaddr_in*)&client_addr; struct in_addr ipAddr = pV4Addr->sin_addr; If you then want the ip address as a string then do the following: char str[INET_ADDRSTRLEN]; inet_ntop( AF_INET, &ipAddr, str, INET_ADDRSTRLEN );
How does the server know the client's IP address and port in order to reply?
Every tcp/ip packet has source and destination in it. When the listening socket is created and receives the packet it has the source address from the packet. After that it uses that data to send the response.
How do I find my client IP address?
Getting the Client IP AddressUse the system environment variable $_SERVER["REMOTE_ADDR"] . One benefit is that on Pantheon this takes into account if the X-Forwarded-For header is sent in cases when a request is filtered by a proxy.Use Drupal's ip_address() function.
What is IP address in socket programming?
If the IP address is like an office building main phone number, a socket is like the extension numbers for offices. So the IP and socket, often called the port, uniquely identify an "office" (server process). You will see unique identifiers like 192.168. 2.100:80 where 80 is the port.
How does a server identify a client?
Use the user's IP address to identify the user. Use all browser data passed to the server (not just user agent, but also OS, screen resolution, and a number of other things) to generate a unique browser fingerprint.
How does a server know client?
1 Answerthe requesting IP address.the HTTP headers, including requested URL and HTTP method.the HTTP request body, if any.if it's HTTPS, any data exchanged during the TLS handshake, which is usually not very relevant for identifying anything significant.
What is the client's IP address?
Client IP addresses describe only the computer being used, not the user. If multiple users share the same computer, they will be indistinguishable. Many Internet service providers dynamically assign IP addresses to users when they log in.
How do I find someone by IP address?
How to Find a User Name From the IP AddressOpen up the "Start" menu.Click on "Run."Enter "Command" (minus the quotation marks) and press "OK." ... Type "nbtstat --a ip" (minus the quotation marks); replace "ip" with the IP. ... Write down the output; this will be the machine name that corresponds to the.More items...
How do I get client IP from request?
In Java, you can use HttpServletRequest. getRemoteAddr() to get the client's IP address that's accessing your Java web application....ReferencesWiki X-Forwarded-For.ServletRequest JavaDoc.HttpServletRequest JavaDoc.How to get Server IP address in Java.
Do sockets have IP addresses?
A socket consists of the IP address of a system and the port number of a program within the system. The IP address corresponds to the system and the port number corresponds to the program where the data needs to be sent: Sockets can be classified into three categories: stream, datagram, and raw socket.
How does a socket server work?
In a connection-oriented client-to-server model, the socket on the server process waits for requests from a client. To do this, the server first establishes (binds) an address that clients can use to find the server. When the address is established, the server waits for clients to request a service.
How does socket programming work?
Socket programming is a way of connecting two nodes on a network to communicate with each other. One socket(node) listens on a particular port at an IP, while the other socket reaches out to the other to form a connection. The server forms the listener socket while the client reaches out to the server.
How do I find my client port?
Type “Cmd” in the search box. Open Command Prompt. Enter the netstat -a command to see your port numbers.
How do I find the client port number for server socket programming?
This can be done using a bind() system call specifying a particular port number in a client-side socket. Below is the implementation Server and Client program where a client will be forcefully get assigned a port number.
How do I test the connection between two ports on a server?
21-Aug-2020•Knowledge Open Windows PowerShell through the Start menu. Enter the command test-netconnection IPAddress -port XXXXX. ... Press Enter. Wait for the test to complete. If the result is True then there is nothing blocking communication between the client and server.
How will you know if a particular port is enabled between source and destination?
Method 1 of 5: Checking if a Local Router Port is Open (Windows) Enable Telnet for Windows. You can use Telnet to check if a certain port is open on your local router or access point.
What is socket in a server?
A Socket is bounded to a local-port. The client will open a connection to the server (by the Operating System/drivers/adapters/hardware/line/.../line/hardware/adapters/drivers/Server OS). This "connection" is done by a protocol, called the IP (Internet Protocol) when you are connected to the Internet.
How does the Internet Protocol identify nodes on a network?
The Internet Protocol will identify nodes on a network by two things: their IP-address and their port. The TCP/IP-protocol will send messages using the IP, and making sure messages are correctly received.
What happens when a client is trying to connect to a server?
If client is trying to connect to the server, the server "accepts" this client (i.e. creates a "client socket" associated with this client).
What is the protocol used to send cookies?
In the web browser/server example, the http protocol (which is an application) protocol hold information about who is this browser in the parameters of the request (the browser transmit cookie informations with every request). The http server can then set/read cookie information to known if the browser connected before and eventually maintain a server side session for that browser.
What happens if there are two sockets?
To answer your second question: if there are two Sockets: the "new" and the "old". Since, by the Internet Protocol, a connection is a pair of nodes, and nodes can only use one port at a time for a connection, the ports of "new" and "old" must be different.
How many ports does an Internet protocol use?
By definition, the Internet Protocol defines a connection as pair of nodes (thus four things: two IP-adresses and two ports). Also, the Internet Protocol defines that one node can only use one port at a time to initiate a connection with another node (note: this only applies for the client, not the server).
Why don't clients specify ports?
clients don't specify a port because it's randomly choosen from the free ones (> 1024 if I'm not wrong) from the underlying operating system
Where is the IP address of a system?
The IP address of the system is located by “ inet addr”. It is your system IP address.
What is a socket in Linux?
Socket. The socket is end point connection for communication between two machines. It is like data connectivity path between two wireless terminals. The socket is required at both sides of server and client. You can refer to the tutorial socket and How to create socket in Linux.
What is server client?
Server-client model is communication model for sharing the resource and provides the service to different machines. Server is the main system which provides the resources and different kind of services when client requests to use it.
What is TCP protocol?
TCP is a connection oriented protocol which stands for transfer control protocol. It is a reliable and secure protocol. In TCP, the receiver can generate the acknowledgement of received packet so sender (client) does need to wait for the acknowledgement and if the back response doesn’t come or any packet error is generated, it will resend to the client.
What happens if you don't run server side code?
Note: If you do not run server-side code and try to run first client side code, connection refused error will come.
Why is IP address important?
IP address plays an important role in communication between server-client models.
What is port in networking?
In terms of networking, the port is the location where information is sent. Various protocols assign a different port number. The specific port number is required to communicate between server-client according to which protocol is used.
What is the protocol used to connect to a client?
We know that in Computer Networks, communication between server and client using TCP/IP protocol is connection oriented (which buffers and bandwidth are reserved for client). Server will get so many hits from different clients, and then server has to identify each client uniquely to reply every request. To achieve this we use “ip address of client (32 bit) + port number (16 bit) of the process”. This is called Socket (48 bit). Any network communication should goes through socket.
How to communicate with client server?
Procedure in Client-Server Communication 1 Socket: Create a new communication 2 Bind: Attach a local address to a socket 3 Listen: Announce willingness to accept connections 4 Accept: Block caller until a connection request arrives 5 Connect: Actively attempt to establish a connection 6 Send: Send some data over connection 7 Receive: Receive some data over connection 8 Close: Release the connection
What is the maximum number of clients that can connect to a server?
6. listen() also an inbuilt function the 2nd argument 20 says that maximum 20 number of clients can connect to that server. So maximum 20 queue process can be handled by server.
What is socket() function?
1. socket() function creates a new socket inside kernel and returns an integer which used as socket descriptor.
How to know if a server is running?
To know the server is running or not we can check using netstat command.
