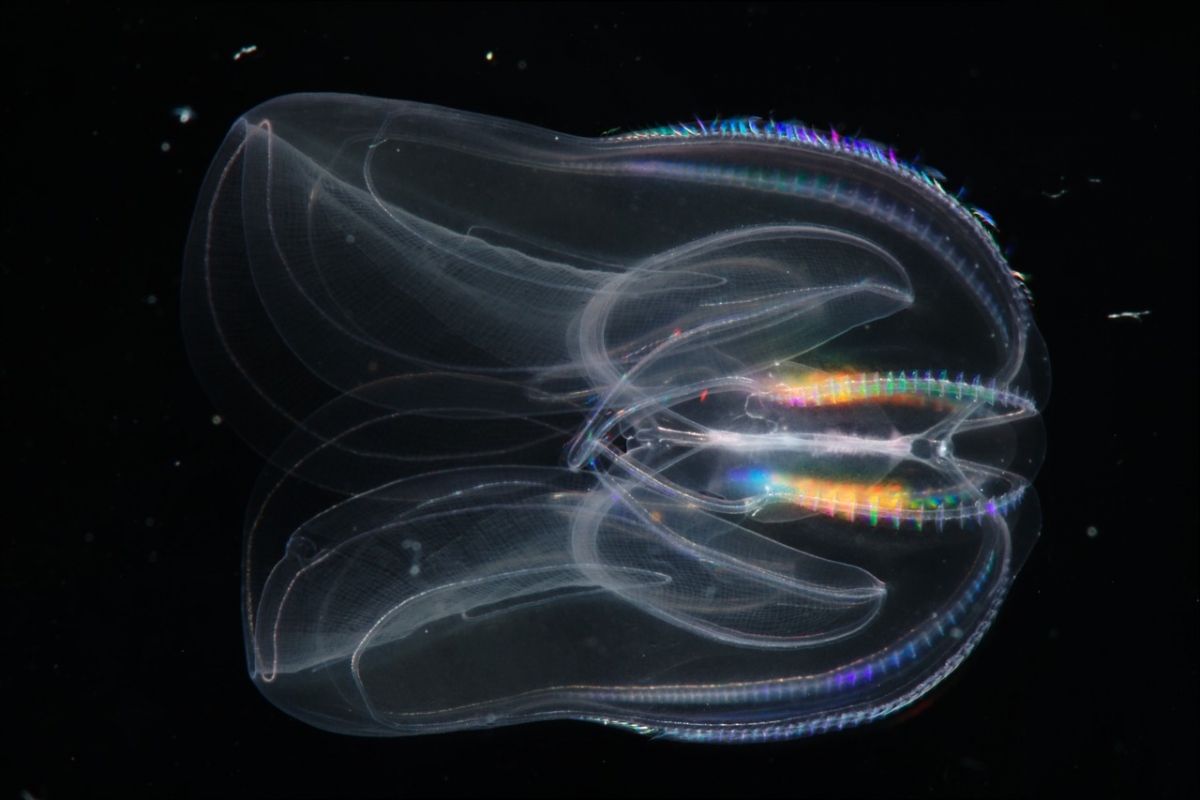
What is binary search tree (BST)?
A binary search tree (BST) is a node based binary tree data structure which has the following properties. The left subtree of a node contains only nodes with keys less than the node’s key. The right subtree of a node contains only nodes with keys greater than the node’s key. Both the left and right subtrees must also be binary search trees.
What are the conditions for a tree to be BST?
Here is an idea: A tree can not be BST if the left subtree contains any value greater than the node’s value and the right subtree contains any value smaller than the node’s value. In other words, the node value must be less than the maximum in the left sub-tree and greater than the minimum in the right sub-tree.
How do you know if a BST is valid?
A BST is valid if it has the following properties: All nodes in the left subtree have values less than the node’s value. All nodes in the right subtree have values greater than the node’s value Both left and right subtrees are also binary search trees.
What are the different types of BST?
BST and especially balanced BST (e.g. AVL Tree) are in this category. 1. Binary Search Tree 2. BST & Balanced BST (AVL Tree) 3. Motivation 3-1. What Kind of Table ADT? 3-2.
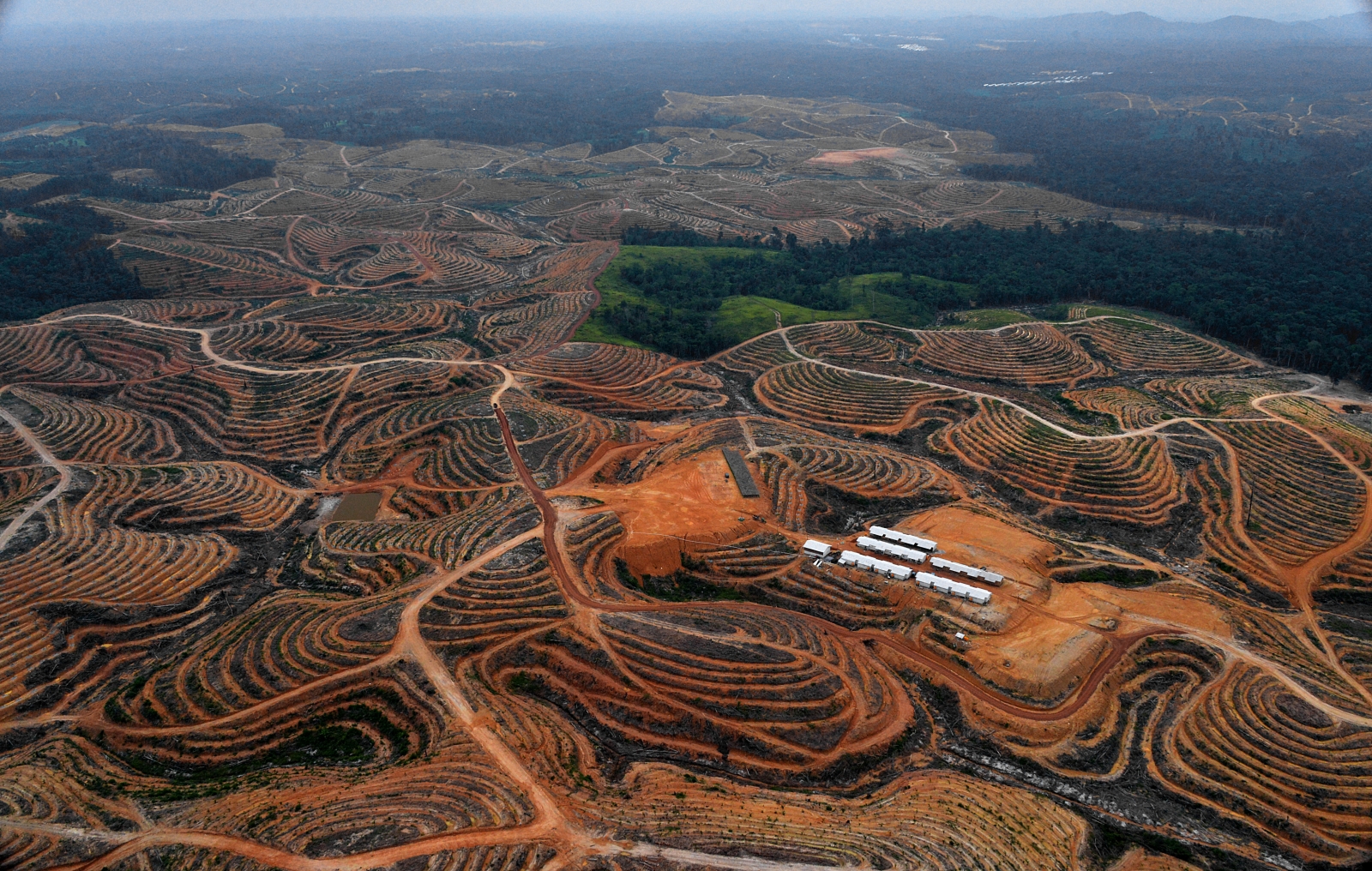
What makes a tree a BST?
A binary search tree (BST) is a binary tree where every node in the left subtree is less than the root, and every node in the right subtree is of a value greater than the root.
Is BST same as binary tree?
Difference between a Binary Tree and a Binary Search Tree A nonlinear data structure known as a Binary Tree is one in which each node can have a maximum of two child nodes. A BST is a binary tree with nodes that has right and left subtrees that are also binary search trees.
Is single node tree a BST?
Structurally, a complete binary tree consists of either a single node (a leaf) or a root node with a left and right subtree, each of which is itself either a leaf or a root node with two subtrees.
Is empty tree a BST?
// A node of a tree. // code allows for duplicate keys. // Base case. An empty tree is a BST.
Is digital search tree similar to binary search tree?
The digital search tree functions to search and insert are quite similar to the corresponding functions for binary search trees. The essential difference is that the subtree to move to is determined by a bit in the search key rather than by the result of the comparison of the search key and the key in the current node.
Which are binary trees?
In computer science, a binary tree is a tree data structure in which each node has at most two children, which are referred to as the left child and the right child.
Is a node a tree?
A technical definition A tree is a collection of entities called nodes . Nodes are connected by edges . Each node contains a value or data , and it may or may not have a child node . The first node of the tree is called the root .
Can binary tree have 1 child?
A binary tree is a tree in which no node has more than two children, and every child is either a left child or a right child even if it is the only child its parent has. A full binary tree is one in which every internal node has two children.
Which of the following is not a binary search tree?
Splay Tree Was this answer helpful?
Is null node A BST?
A BST is considered a data structure made up of nodes, like Linked Lists. These nodes are either null or have references (links) to other nodes. These 'other' nodes are child nodes, called a left node and right node.
Are binary trees always sorted?
Generally binary tree's aren't sorted for you.
Is binary tree valid?
A BST is valid if it has the following properties: All nodes in the left subtree have values less than the node's value. All nodes in the right subtree have values greater than the node's value. Both left and right subtrees are also binary search trees.
What is difference between B-tree and binary tree?
B-tree is called a sorted tree as its nodes are sorted in inorder traversal. While binary tree is not a sorted tree. It can be sorted in inorder, preorder, or postorder traversal.
What is the difference between binary search tree BST and heap?
The Heap differs from a Binary Search Tree. The BST is an ordered data structure, however, the Heap is not. In computer memory, the heap is usually represented as an array of numbers. The heap can be either Min-Heap or Max-Heap.
Why is it called a binary search tree?
Binary search tree is a data structure that quickly allows us to maintain a sorted list of numbers. It is called a binary tree because each tree node has a maximum of two children. It is called a search tree because it can be used to search for the presence of a number in O(log(n)) time.
What are binary trees with threads called?
Such binary trees with threads are known as threaded binary trees. Each node in a threaded binary tree either contains a link to its child node or thread to other nodes in the tree.
How to compare BST?
If the compared node doesn't match then you either proceed to the right child or the left child, which depends on the outcome of the following comparison: If the node that you are searching for is lower than the one you were comparing it with , you proceed to the left child, otherwise (if it's larger) you go to the right child. Why? Because the BST is structured (as per its definition), that the right child is always larger than the parent and the left child is always lesser.
What is a perfect binary tree?
Perfect Binary Tree A Binary tree is Perfect Binary Tree in which all internal nodes have two children and all leaves are at the same level.
How many children does a subtree have?
One subtree (one child): You have to make sure that after the node is deleted, its child is then connected to the deleted node's parent. Two subtrees (two children): You have to find and replace the node you want to delete with its inorder successor (the leftmost node in the right subtree).
What is breadth first search?
It begins at the root node and travels in a lateral manner (side to side), searching for the desired node. This type of search can be described as O (n) given that each node is visited once and the size of the tree directly correlates to the length of the search.
What is binary search tree?
What is a Binary Search Tree? A tree is a data structure composed of nodes that has the following characteristics: Each tree has a root node at the top (also known as Parent Node) containing some value (can be any datatype). The root node has zero or more child nodes.
What is the number of leaf nodes in a binary tree?
In Full Binary Tree, number of leaf nodes is equal to number of internal nodes plus one. Complete Binary Tree: A Binary Tree is complete Binary Tree if all levels are completely filled except possibly the last level and the last level has all keys as left as possible.
How many children does a binary search tree have?
This means that every node on its own can be a tree. A binary search tree (BST) adds these two characteristics: Each node has a maximum of up to two children. For each node, the values of its left descendent nodes are less than that of the current node, which in turn is less than the right descendent nodes (if any).
What is a BST tree?
A binary search tree (BST) is a node-based binary tree data structure which has the following properties. The left subtree of a node contains only nodes with keys less than the node’s key. The right subtree of a node contains only nodes with keys greater than the node’s key. Both the left and right subtrees must also be binary search trees.
Which subtrees must be binary search trees?
Both the left and right subtrees must also be binary search trees.
How to check if a tree is binary?
Check if a Binary Tree is BST : Simple and Efficient Approach 1 The left subtree of a node contains only nodes with keys less than the node’s key. 2 The right subtree of a node contains only nodes with keys greater than the node’s key. 3 Both the left and right subtrees must also be binary search trees.
Which subtree always contains elements greater than the root?
In this way, all of the steps are satisfied while constructing the tree. The left subtree always contains elements lesser than the root, while the right subtree always contains elements greater than the root. Now, this concept applies to both the root as well as the intermediate nodes in the trees as well (carefully think about it for a second!).
What is binary search tree?
Well, binary search trees or BST are a special variant of trees that come with a very unique condition for filling in the left and right children of every node in the tree. In order to understand the basics of the binary search tree, one needs to understand the basics of a binary tree first. The binary tree is a tree where each node (except the leaves) has two children. Each node can have one parent and a maximum of two children.
What is the root of a tree?
The root is the starting node for a tree from which all of the nodes are added as children. The root does not have a parent since it is the "first node" in the tree.
What is a node in a binary tree?
Node - Every unit that makes up the tree is called a node. Each node holds some data and points towards other nodes in the tree. For a binary tree, each node has two children - one left child and one right child.
Why is binary search tree less complex?
Because of the unique properties of a binary search tree, the algorithm used for searching in a binary search tree is much less complex and requires lesser time to execute. To search for an element, simply follow the below steps:-
What are the leaves on a tree?
Leaves - Like a real-world tree, every tree in the digital world has leaves. These leaves are the ultimate nodes in the tree, marking the terminals of the tree. Leaves do not have any children since they technically mark the "borders" for the points where the tree ends.
How many ways can trees be traversed?
All trees can be traversed in three main ways. Each particular approach gives us a different arrangement of nodes. The three main approaches used are:-
What is BST in data science?
BST (and especially balanced BST like AVL Tree) is an efficient data structure to implement a certain kind of Table (or Map) Abstract Data Type (ADT).
How to select a key in BST?
Or in another word, find the k -th smallest element in the BST. That is, Select (1) = FindMin ( ) and Select ( N) = FindMax ().
What is an Avl tree?
An Adelson-Velskii Landis (AVL) tree is a self-balancing BST that maintains it's height to be O (log N) when having N vertices in the AVL tree.
What is binary search tree?
A Binary Search Tree (BST) is a binary tree in which each vertex has only up to 2 children that satisfies BST property: All vertices in the left subtree of a vertex must hold a value smaller than its own and all vertices in the right subtree of a vertex must hold a value larger than its own (we have assumption that all values are distinct integers in this visualization and small tweak is needed to cater for duplicates/non integer). Try clicking Search (7) for a sample animation on searching a random value ∈ [1..99] in the random BST above.
Why do we use binary search in BST?
Because of the way data (distinct integers for this visualization) is organised inside a BST, we can binary search for an integer v efficiently (hence the name of Binary Search Tree).
What is the minimum integer in the right subtree of v?
If v has a right subtree, the minimum integer in the right subtree of v must be the successor of v. Try Successor (23) (should be 50).
What is BST in a database?
BST is a collection of nodes arranged in a way where they maintain BST properties. Each node has a key and an associated value. While searching, the desired key is compared to the keys in BST and if found, the associated value is retrieved.
How to search for an element in a subtree?
Whenever an element is to be searched, start searching from the root node. Then if the data is less than the key value, search for the element in the left subtree. Otherwise, search for the element in the right subtree. Follow the same algorithm for each node.
Which root node has all less-valued keys on the left sub-tree and the higher valued keys on?
We observe that the root node key (27) has all less-valued keys on the left sub-tree and the higher valued keys on the right sub-tree.
How to search a binary tree?
Data Structure - Binary Search Tree 1 The value of the key of the left sub-tree is less than the value of its parent (root) node's key. 2 The value of the key of the right sub-tree is greater than or equal to the value of its parent (root) node's key.
What is the value of the key of the left sub-tree?
The value of the key of the left sub-tree is less than the value of its parent (root) node's key. The value of the key of the right sub-tree is greater than or equal to the value of its parent (root) node's key.
What does "insert" mean in a tree?
Insert − Inserts an element in a tree.
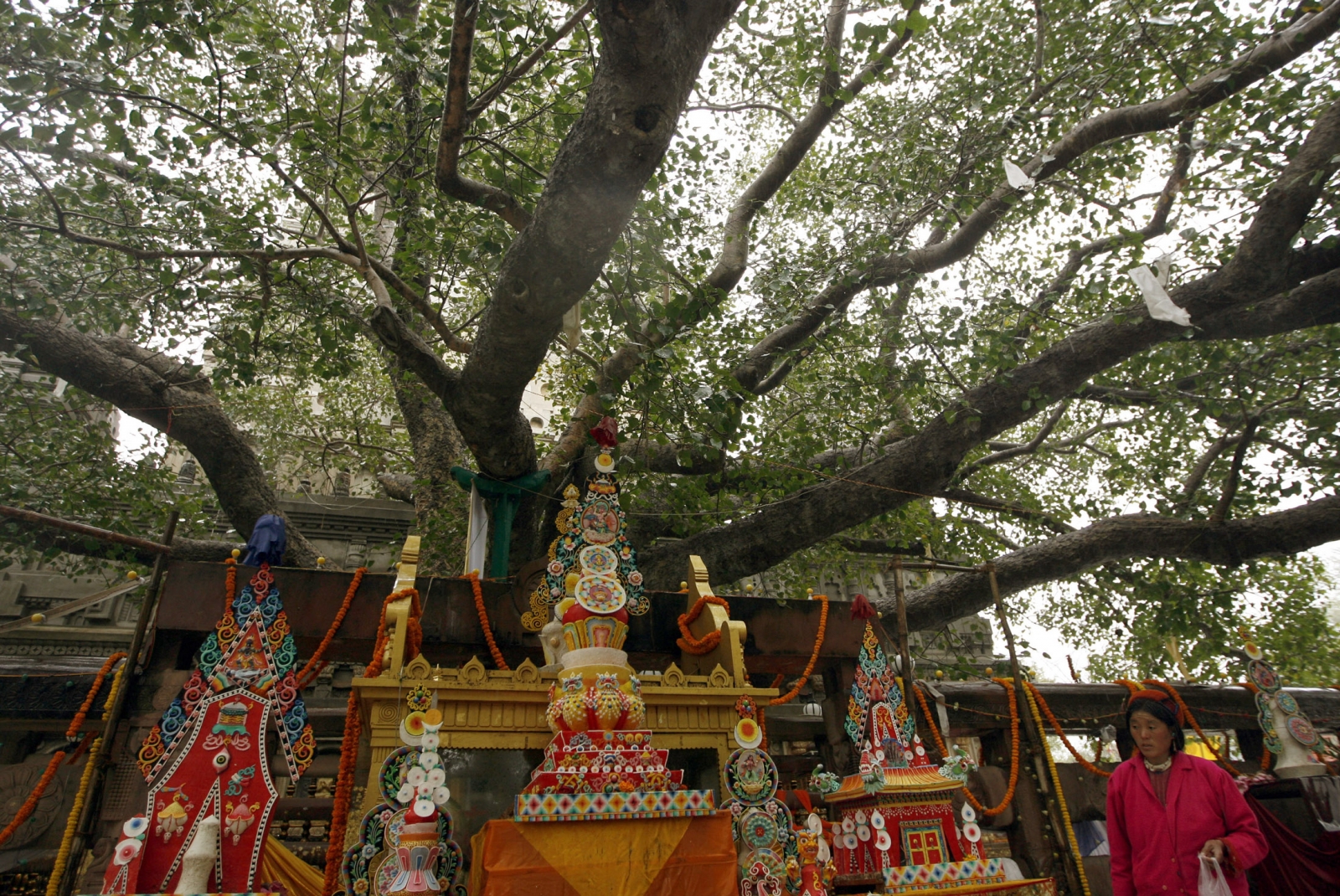
Basic Operations on A BST
- Create: creates an empty tree.
- Insert: insert a node in the tree.
- Search: Searches for a node in the tree.
- Delete: deletes a node from the tree.
Special Types of Bt
- Heap
- Red-black tree
- B-tree
- Splay tree
Runtime
- Data structure: BST 1. Worst-case performance: O(n) 2. Best-case performance: O(1) 3. Average performance: O(log n) 4. Worst-case space complexity: O(1) Where nis the number of nodes in the BST. Worst case is O(n) since BST can be unbalanced.
Let's Look at A Couple of Procedures Operating on Trees.
- Since trees are recursively defined, it's very common to write routines that operate on trees that are themselves recursive. So for instance, if we want to calculate the height of a tree, that is the height of a root node, We can go ahead and recursively do that, going through the tree. So we can say: 1. For instance, if we have a nil tree, then its height is a 0. 2. Otherwise, We're 1 plus the max…
Pre-Order
- This traversal first accesses the current node value, then traverses the left and right sub-trees respectively.
Post-Order
- This traversal puts the root value at last, and goes over the left and right sub-trees first. The relative order of the left and right sub-trees remain the same. Only the position of the root changes in all the above mentioned traversals.