
Yes, your understanding is correct. computeIfAbsent () / computeIfPresent () methods on ConcurrentMap do not employ locking. Instead, they work concurrently with other modifications. Also note that the following is both non-thread-safe and bad practice:
Full Answer
What is the use of computeIfAbsent in Java?
HashMap computeIfAbsent() method in Java with Examples. The computeIfAbsent(Key, Function) method of HashMap class is used to compute value for a given key using the given mapping function, if key is not already associated with a value (or is mapped to null) and enter that computed value in Hashmap else null.
What is computeIfAbsent in ConcurrentHashMap?
The computeIfAbsent (Key, Function) method of ConcurrentHashMap class which attempts to compute its value using the given mapping function for specified key if key is not already associated with a value (or is mapped to null) and enter that computed value in map else null.
Is ConcurrentHashMap thread safe?
ConcurrentHashMap is fundamentally thread-safe and is more performant than the Collections.synchronizedMap wrapper around a non-thread-safe Map. It's actually a thread-safe map of thread-safe maps, allowing different activities to happen simultaneously in its child maps.
Is put in ConcurrentHashMap thread-safe?
ConcurrentHashMap class is thread-safe i.e. multiple threads can operate on a single object without any complications. At a time any number of threads are applicable for a read operation without locking the ConcurrentHashMap object which is not there in HashMap.
Is ConcurrentHashMap synchronized?
ConcurrentHashMap is thread-safe therefore multiple threads can operate on a single object without any problem. In ConcurrentHashMap, the Object is divided into a number of segments according to the concurrency level....Java.ConcurrentHashMapSynchronized HashMapIt locks some portion of the map.It locks the whole map.4 more rows•Aug 14, 2021
Can ConcurrentHashMap have null key?
ConcurrentHashMap does not allow null key or value. It will throw NullPointerException. ConcurrentHashMaps is not allowed null, to avoid ambiguities.
Is HashMap faster than ConcurrentHashMap?
HashMap performance is relatively high because it is non-synchronized in nature and any number of threads can perform simultaneously. But ConcurrentHashMap performance is low sometimes because sometimes Threads are required to wait on ConcurrentHashMap.
What is the difference between ConcurrentHashMap and synchronized HashMap?
synchronizedMap() requires each thread to acquire a lock on the entire object for both read/write operations. By comparison, the ConcurrentHashMap allows threads to acquire locks on separate segments of the collection, and make modifications at the same time.
Is LinkedHashMap synchronized?
Just like HashMap, LinkedHashMap implementation is not synchronized. So if you are going to access it from multiple threads and at least one of these threads is likely to change it structurally, then it must be externally synchronized.
Is TreeMap synchronized?
The implementation of a TreeMap is not synchronized. This means that if multiple threads access a tree set concurrently, and at least one of the threads modifies the set, it must be synchronized externally. This is typically accomplished by using the Collections.
Is HashSet synchronized?
1) Both HashMap and HashSet are not synchronized which means they are not suitable for thread-safe operations unitl unless synchronized explicitly. This is how you can synchronize them explicitly: HashSet: Set s = Collections.
Why is it bad to use a single thread?
When such a method is called by a thread, other threads cannot concurrently access the object. This can reduce concurrency performance as everything ends up running single-threaded. This approach is especially bad when an object is read more often than it is updated.
Which Java library can be accessed simultaneously by multiple threads?
For this reason, Java provides concurrent collections such as CopyOnWriteArrayList and ConcurrentHashMap that can be accessed simultaneously by multiple threads:
How to avoid concurrency issues?
The main way we can avoid such concurrency issues and build reliable code is to work with immutable objects. This is because their state cannot be modified by the interference of multiple threads.
How do threads communicate?
Threads communicate primarily by sharing access to the same objects. So, reading from an object while it changes can give unexpected results. Also, concurrently changing an object can leave it in a corrupted or inconsistent state.
Can collections be changed by multiple threads?
Like any other object, collections maintain state internally. This could be altered by multiple threads changing the collection concurrently. So, one way we can safely work with collections in a multithreaded environment is to synchronize them:
Can two threads update the if block at the same time?
For example, two threads could enter the if block at the same time and then update the list, each thread adding the foo value to the list.
Why does ConcurrentHashMap work?
In many cases it works fine just because the contract of ConcurrentHashMap takes care of the potential synchronization issues related to reading/writing to the map. But there are cases where it's not enough, and a developer gets race conditions which are hard to predict, and even harder to find/debug and fix.
Is concurrent map threadsafe?
The point of this example was to introduce a common pitfall of blindly relying on ConcurrentHashMap as a majical synchronzed datastructure which is threadsafe and therefore should solve all our concurrency issues regarding multiple threads working on a shared Map. ConcurrentHashMap is, indeed, threadsafe. But it only means that all read/write operations on such map are internally synchronized. And sometimes it's just not enough for our concurrent environment needs, and we have to use some special treatment which will guarantee atomic execution. A good practice will be to use one of the atomic methods implemented by ConcurrentHashMap, i.e: computeIfAbsent (..), putIfAbsent (..), etc.
What happens if a key is not already associated with a value?
If the specified key is not already associated with a value, attempts to compute its value using the given mapping function and enters it into this map unless null.
Can an iterator be used by only one thread?
However, iterators are designed to be used by only one thread at a time. Bear in mind that the results of aggregate status methods including size, isEmpty, and containsValue are typically useful only when a map is not undergoing concurrent updates in other threads.
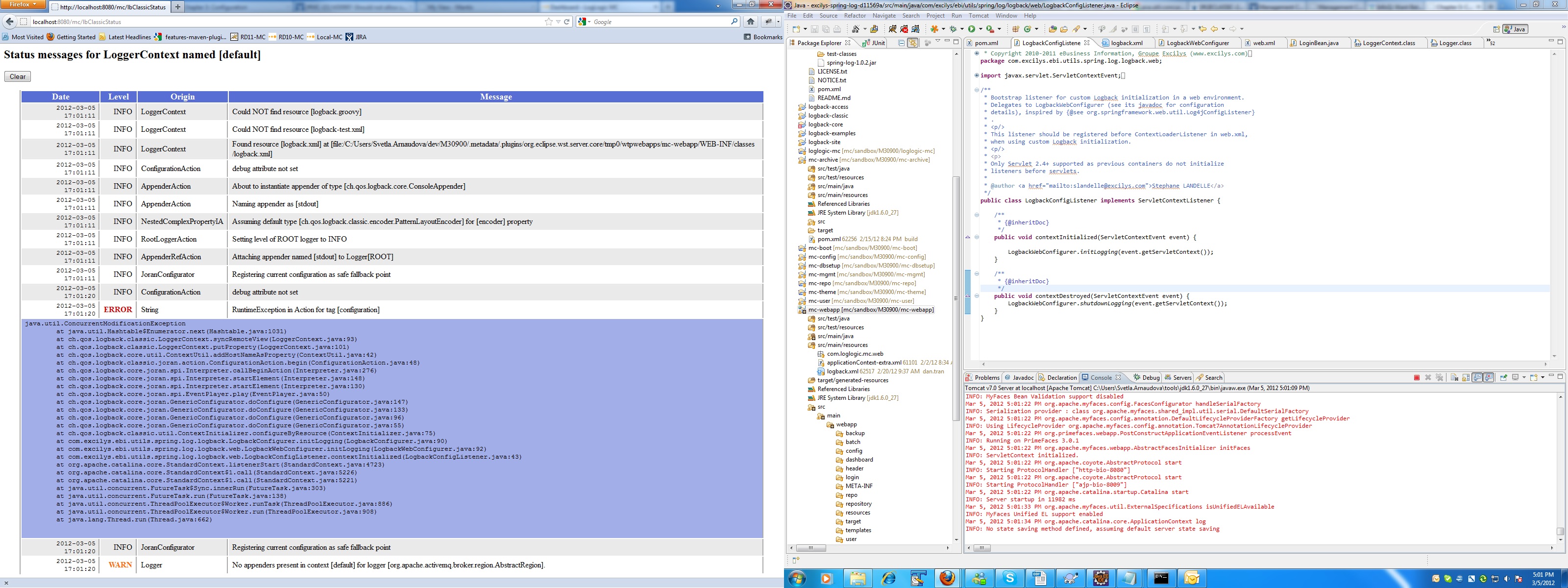