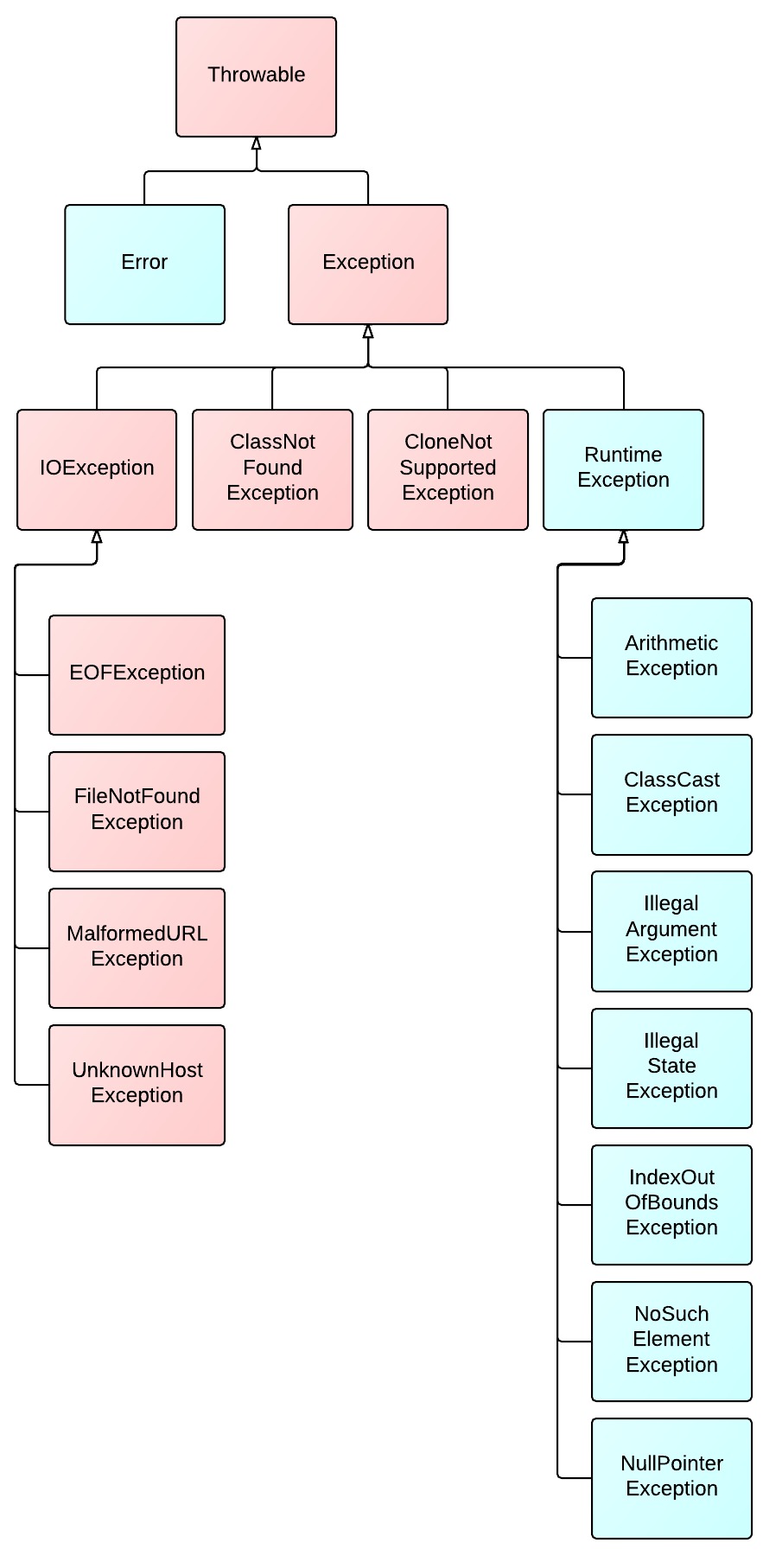
How many elements can a stack store in Java?
The size of the Stack object is given by the java.util.Stack.size () method. It returns the total number of elements in the stack. The following example prints the stack size.
Is the stack garbage collected in Java?
In the Java™ SDK shipped for AIX®, Linux® and Windows®, the mark stack is created during the first phase of garbage collection when live objects are marked for retention. Objects are taken off the stack and any references held by the object are added.
How to wrap each object of a collection in Java?
- booleanhasNext (): This returns true if the ListIterator has more elements when traversing the list in the forward direction.
- booleanhasPrevious (): This returns true if the ListIterator has more elements when traversing the list in the reverse direction.
- element next (): This returns the next element in the list.
Where is the queue class in the Java collections?
The queue interface is provided in java.util package and it implements the Collection interface. The queue implements FIFO i.e. First In First Out. This means that the elements entered first are the ones that are deleted first. A program that demonstrates queue in Java is given as follows − Now let us understand the above program.

What is stack in collection?
The Stack class is part of Java's collections framework. Following is the class hierarchy of Stack in Java - The Stack class extends Vector which implements the List interface. A Vector is a re-sizable collection. It grows its size to accommodate new elements and shrinks the size when the elements are removed.
What is a stack in Java?
Java Collection framework provides a Stack class that models and implements a Stack data structure. The class is based on the basic principle of last-in-first-out. In addition to the basic push and pop operations, the class provides three more functions of empty, search, and peek.
Are stack and queue collections in Java?
Stack and Queue are fundamental data structures in Java Collections Framework. They are used to store the same type of data and retrive the data in specific order. Stack and Queue both are Linear Data Structures. Stack follows the LIFO principle i.e. Last In First Out.
What is stack class in Java?
Advertisements. Stack is a subclass of Vector that implements a standard last-in, first-out stack. Stack only defines the default constructor, which creates an empty stack. Stack includes all the methods defined by Vector, and adds several of its own.
What is collection class in Java?
Collections class is a member of the Java Collections Framework. The java. util. Collections package is the package that contains the Collections class. Collections class is basically used with the static methods that operate on the collections or return the collection.
Is stack a type in Java?
Java Stack Class In Java, Stack is a class that falls under the Collection framework that extends the Vector class. It also implements interfaces List, Collection, Iterable, Cloneable, Serializable. It represents the LIFO stack of objects. Before using the Stack class, we must import the java.
Is stack a FIFO?
Stacks are based on the LIFO principle, i.e., the element inserted at the last, is the first element to come out of the list. Queues are based on the FIFO principle, i.e., the element inserted at the first, is the first element to come out of the list.
Is stack an interface?
Interface Stack
Is stack a priority queue?
A stack requires elements to be processed in Last in First Out manner. The idea is to associate a count that determines when it was pushed. This count works as a key for the priority queue. So the implementation of stack uses a priority queue of pairs, with the first element serving as the key.
How stack is implemented in Java?
Stack Implementation in Javapush inserts an item at the top of the stack (i.e., above its current top element).pop removes the object at the top of the stack and returns that object from the function. ... isEmpty tests if the stack is empty or not.isFull tests if the stack is full or not.More items...
What is stack memory in Java?
Stack Memory in Java is used for static memory allocation and the execution of a thread. It contains primitive values that are specific to a method and references to objects referred from the method that are in a heap. Access to this memory is in Last-In-First-Out (LIFO) order.
Is empty stack Java?
Stack. empty() is used to check if a stack is empty or not. This method requires no parameters. It returns true if the stack is empty and false if the stack is not empty.
What is a stack in Java?
Java Collection framework provides a Stack class that models and implements a Stack data structure. The class is based on the basic principle of last-in-first-out. In addition to the basic push and pop operations, the class provides three more functions of empty, search, and peek. The class can also be said to extend Vector and treats the class as a stack with the five mentioned functions. The class can also be referred to as the subclass of Vector. The below diagram shows the hierarchy of the Stack class :
How to add an element to a stack?
1. Adding Elements: In order to add an element to the stack, we can use the push () method. This push () operation place the element at the top of the stack.
Is stack class a legacy class?
Note: Please note that the Stack class in Java is a legacy class and inherits from Vector in Java. It is a thread-safe class and hence involves overhead when we do not need thread safety. It is recommended to use ArrayDeque for stack implementation as it is more efficient in a single-threaded environment. Java.
Introduction
Data structures are the basic need of every program. It's time-consuming to create a data structure from scratch every time. For this purpose, programming languages provide built-in data structures. Standard Template Library (STL) in C++, Collection Framework in Java, serves this purpose.
What is Collection Framework in Java?
A single unit of objects in Java is referred to as a collection. A collection is a framework that allows us to store and modify a group of objects. Java Collections can perform all data operations such as searching, sorting, insertion, manipulation, and deletion.
Stack Class in Java
The Stack class is based on the principle of Last in First out (LIFO). The element inserted last will be the first to be removed. The Stack class provided by Java Collections implements five interfaces. The list of those interfaces are given below:
The constructor of Stack Class
The Stack class in Java contains only one constructor. It creates an empty Stack.
Methods of Stack Class
This built-in Stack in Java also contains predefined methods. Some of the important methods are given below:
More about legacy Classes in Java
As we’ve seen above, legacy classes were included in Java from the initial versions. But when Collections were introduced, they were on the verge of depreciation. To make them compatible, Java restructured those classes. They are not obsolete, but their better alternatives are present in Java.
Frequently asked questions
Q1. What is Collection in Java? Answer: The collection represents a single unit of objects, i.e. a group. The collection framework represents a unified architecture for storing and manipulating groups of objects. It has Interfaces and its implementations, i.e., classes.
What is stack class?
The Stack class provides methods to add, remove, and search data in the Stack. It also provides a method to check if the stack is empty. We will discuss these methods in the below section.
What are stacks used for?
Answer: Following are the main applications of the stack: 1 Expression evaluation and conversions: Stack is used for converting expressions into postfix, infix, and prefix. It is also used to evaluate these expressions. 2 The stack is also used for parsing syntax trees. 3 The stack is used to check parentheses in an expression. 4 The stack is used for solving backtracking problems. 5 Function calls are evaluated using stacks.
Why are stacks better than memory?
Answer: Variables stored on stack are destroyed automatically when returned. Stacks are a better choice when memory is allocated and deallocated. Stacks also clean up the memory. Apart from that stacks can be used effectively to evaluate expressions and parse the expressions.
Can stacks be implemented in arrays?
The stack can also be implemented using a linked list just like how we have done using arrays. One advantage of using a linked list for implementing stack is that it can grow or shrink dynamically. We need not have a maximum size restriction like in arrays.
Is a stack a collection?
Answer: Yes. The stack is a legacy collection in Java that is available from Collection API in Java 1.0 onwards. Stack inherits the Vector class of the List interface.
Worthy Note
You should consider using the Deque class as it provides a more complete and consistent set of LIFO operations than the Stack class.
Adding elements (Pushing)
Adding elements to myStack is done by using the push (Object obj) method. This will add an item to the top of the stack.
Removing elements (Popping)
Removing elements is just a matter of calling the method pop (). This will remove an item from the top of the stack.
Size of Collection
Returning the number of elements in a Stack is as easy as calling the size () method.
Iterating through the Collection
Creating an iterator and looping through the collection is quite easy and straight forward.
Output
Reversing a Phrase using a Stack In the end, it's not the years in your life that count. It's the life in your years - Abraham Lincoln Size of myStack is now 0 Tokenizing and pushing all the words onto the Stack...