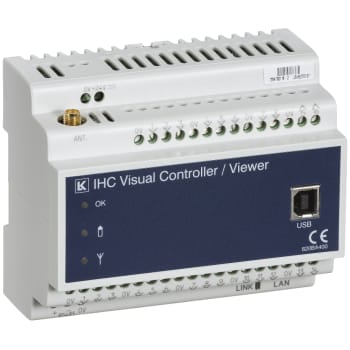
Why is it so hard to unit test MVC controllers?
The trouble is, in practice, controllers do most of their useful work as part of a framework, not in isolation. In unit tests, you (intentionally) don't get any of that. In this section I highlight some of the aspects of MVC controllers that you can't easily test or wouldn't want to test in a unit test.
How to unit test your web API controllers?
Arrange: Set up any prerequisites for the test to run. Act: Perform the test. Assert: Verify that the test succeeded. In the arrange step, you will often use mock or stub objects. That minimizes the number of dependencies, so the test is focused on testing one thing. Here are some things that you should unit test in your Web API controllers:
What is the use of controller in unit testing?
The controller is a class like any other class which has members like methods and properties etc. In Unit Testing, we create an instance of the target class and call its method for various inputs and assert the result as per expectation.
Should controller routing be part of unit tests?
You could certainly argue that's OK for unit tests—routing is a separate concern to the handling of a method. I can buy that, but routing is such a big part of what a controller is for, it feels like it's missing the point slightly.
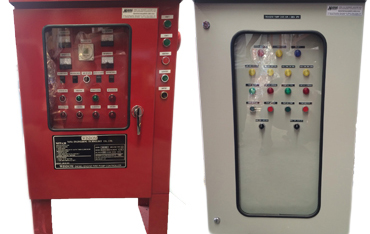
Do you need to unit test controllers?
You should TDD all the components/objects the controller uses and keep the controllers as thin as possible. Then everything is properly unit tested. What you want to be certain of is that everything works well together when performing web requests.
Do we write test cases for controller?
It's definitely possible to write pure unit tests for Spring MVC controllers by mocking their dependencies with Mockito (or JMock) as jherricks showed above.
How do you test a new controller?
1:016:24How to TEST your Xbox One Controller - YouTubeYouTubeStart of suggested clipEnd of suggested clipSo the app we're going to use is something called game controller tester. Now you can do it on yourMoreSo the app we're going to use is something called game controller tester. Now you can do it on your Xbox. Or you can do it on a PC. Here it is on a little Windows 10 laptop.
Does everything need to be unit tested?
The answer to the more general question is yes, you should unit test everything you can. Doing so creates a legacy for later so changes down the road can be done with peace of mind. It ensures that your code works as expected. It also documents the intended usage of the interfaces.
Can you unit test controllers?
When unit testing controller logic, only the contents of a single action are tested, not the behavior of its dependencies or of the framework itself. Set up unit tests of controller actions to focus on the controller's behavior. A controller unit test avoids scenarios such as filters, routing, and model binding.
How do you write a unit test case for a rest controller?
Writing a Unit Test for REST Controller First, we need to create Abstract class file used to create web application context by using MockMvc and define the mapToJson() and mapFromJson() methods to convert the Java object into JSON string and convert the JSON string into Java object.
Is controller drift fixable?
It's called controller drift, or analog stick drift, because one or both thumbsticks will drift, or move, in an undesired direction even when you aren't touching them. To fix Xbox One controller drift, you need to take the controller apart and repair or replace one or more components related to the analog sticks.
How do I stop my controller from drifting?
How to Fix PS4 Controller Analog Stick DriftReset your PS4 controller. Resetting the DualShock 4 can solve a lot of issues that suddenly pop up. ... Clean your PS4 controller. ... Get your PS4 controller repaired or replaced by Sony. ... Disassemble your PS4 controller to clean the analog stick. ... Replace the PS4 analog sticks.
How can I tell if my controller is drifting?
1:172:46HOW to FIND out if your PS5 controller has stick drift - YouTubeYouTubeStart of suggested clipEnd of suggested clipYou will see these analog sticks see as i move them they move. Now you can move the control likeMoreYou will see these analog sticks see as i move them they move. Now you can move the control like this all the way around now if you had of your controller.
What should I not unit test?
Unit Testing - What not to testDo not test anything that does not involve logic. For example: If there is a method in the service layer which simply invokes another method in the data access layer, don't test it.Do not test basic database operations. ... I don't need to validate objects at all layers.
Are unit tests a waste of time?
Unit testing is a waste of time, but it's a good way to test your code. You'll have to do it manually, and it's not always easy to fix problems. Testing is a way of getting things right, but you'll get stuck in testing time and have to deal with the problem.
Why are unit tests useless?
All the unit tests are suddenly rendered useless. Some test code may be reused but all in all the entire test suite has to be rewritten. This means that unit tests increase maintenance liabilities because they are less resilient against code changes. Coupling between modules and their tests is introduced!
How do you write a JUnit test case for a spring rest controller?
Now, we are gonna unit test one of the REST controller using Mockito.Introduction.Implementation. Add Dependencies. Create Utility Class. Test with Mock User. Using @WithMockUser Annotation. Using @WithUserDetails Annotation. Using Custom Annotation. ... Run JUnit Tests.References.Source Code.Conclusion.
How do I make a MockMvc instance?
You can obtain a MockMvc instance through the following two methods of MockMvcBuilders:standaloneSetup(). Registers one or more @Controller instances and allows programmatically configuring the Spring MVC infrastructure to build a MockMvc instance. ... webAppContextSetup().
How do you write JUnit test cases for JPA repository?
You can create a @DataJpaTest and @Autowire your repository into it. For example: @RunWith(SpringRunner. class) @DataJpaTest public class MyJpaTest { @Autowired private ChartRepository chartRepository; @Test public void myTest() { ... } }
How do you write a test case for PUT method?
2:068:48API Tests for HTTP PUT method - JavaScript API Automation - YouTubeYouTubeStart of suggested clipEnd of suggested clipSo i will just create a new it block. Name this put slash users this one would be with id. So i'mMoreSo i will just create a new it block. Name this put slash users this one would be with id. So i'm going to do id and then over here we need obviously a data. So something that we will be changing.
What happens when you test the first controller?
If you're testing the first type of controller, where the action method contains all the business logic for the action, then you're going to struggle. These controllers are doing everything, so the "unit" here is really too large to easily test. You're likely going to have to rely on mocking lots of services, which increases the complexity of tests, while generally reducing their efficacy.
Why do you use filters in unit testing?
When filters are used like this, to extract common logic, they make controllers easier to test.
What is thick controller?
Thick controllers—The action method contains all the logic for implementing the behaviour. The MVC controller likely has additional services injected in the constructor, and the controller takes care of everything. This is the sort of code you often see in code examples online. You know the sort—where an EF Core DbContext, or IService is injected and manipulated in the action method body:
Do controllers work in isolation?
The trouble is, in practice, controllers do most of their useful work as part of a framework, not in isolation. In unit tests, you (intentionally) don't get any of that.
Can you unit test a controller?
Again, we're talking about unit tests of controllers, but model binding is a key part of the controller in practice, and likely won't be unit tested separately. You could have a method argument that's impossible to bind to a request, and unit tests won't identify that. Effectively, you may be calling your controller method with values that cannot be generated in practice.
Is routing OK for unit tests?
You could certainly argue that's OK for unit tests—routing is a separate concern to the handling of a method. I can buy that, but routing is such a big part of what a controller is for, it feels like it's missing the point slightly.
Can you make a mistake using read only properties?
Granted, a mistake like that, using read-only properties, is very unlikely in practice. But there are also pretty common mistakes like typos in property names that mean model binding would fail. Those won't be picked up in unit tests, where strong typing means you just set the property directly.
What is unit testing?
In Unit Testing, we create an instance of the target class and call its method for various inputs and assert the result as per expectation.
What is controller class?
The controller is a class like any other class which has members like methods and properties etc.
Is there confusion in developers on what is scoped under the unit testing?
While performing Unit testing there is always confusion in developers on what is scoped under the Unit Testing.
Do you have to wait for integration of different modules in a unit test?
On the good side of unit test cases, you need not have wait for the integration of different modules ( require in Integration test and real API Test) helping in real-time identifying the breaking changes. Most Unit test cases run as you type your code, hence it is easy to be tested or identify breaking changes.
Can you add more test cases to a unit test?
There is absolutely no problem in adding more test cases including Custom attribute validation or [ Authorize] attribute validation within the Unit Test cases.
Is a unit test async?
As good practices mark your Unit test method as async if Controller methods are Asynchronous in nature.
Why is the controller more testable?
Notice the controller uses dependency injection to inject an IProductRepository. That makes the controller more testable, because you can inject a mock repository. The following unit test verifies that the Get method writes a Product to the response body. Assume that repository is a mock IProductRepository.
What is the pattern of a unit test?
A common pattern in unit tests is "arrange-act-assert": Arrange: Set up any prerequisites for the test to run. Act: Perform the test. Assert: Verify that the test succeeded. In the arrange step, you will often use mock or stub objects.
What does "not found" mean in a unit test?
The Get method calls NotFound () if the product is not found. For this case, the unit test just checks if the return type is NotFoundResult.
Does a unit test execute an action?
Notice that the unit test doesn't execute the action result. You can assume the action result creates the HTTP response correctly. (That's why the Web API framework has its own unit tests!)
Why is unit testing important?
Unit testing is a means for providing higher quality software and not an aim per se. Having too many redundant unit tests will cause a future maintenance headache and will cost more project development time.
Why Do Controllers Get Fat?
I did blog earlier on how views become fat Three Steps to Get Fat-Razor MVC Views on Diet, some points are common and some are specific to controllers:
What is unit testing?
UNIT TESTING is a type of software testing where individual units or components of the software are tested. The purpose is to validate that each unit of the software code performs as expected.
Why is it important to write a unit test?
Follow naming conventions strictly. When writing unit tests, it is important to be able to determine which properties and methods of an object are public and which are private implementation details of the object itself. This is because unit tests should only test the publicly defined APIs, as these are the only APIs that are guaranteed to exist and produce stable results.
Why do we need a test case?
The key purpose of a test case is to ensure if different features within an application are working as expected. It helps the tester, validate if the software is free of defects and if it is working as per the expectations of the end-users. Other benefits of test cases include: Test cases ensure good test coverage.
Do we have to follow coding rules for testable code?
We have to follow some coding rules for testable code. Those are given bellow
Is TestNG a good alternative to JUnit?
TestNG is also a good alternative to jUnit in java. Mocking. Mocking is the act of removing external dependencies from a unit test in order to create a controlled environment around it. Mocking is a process used in unit testing when the unit being tested has external dependencies.
Can a developer forget to run unit tests?
However, consider the human factor: A developer might forget to run unit tests after making changes and submit potentially non-working code to a common branch. To avoid this, many companies apply a continuous development approach. Tools for continuous integration are used for this, allowing developers to run unit tests automatically. Thus, any unwanted changes in the code will be detected by a cold, logical machine.
Is a test case reusable?
Test cases are reusable for the future — anyone can reference them and execute the test.
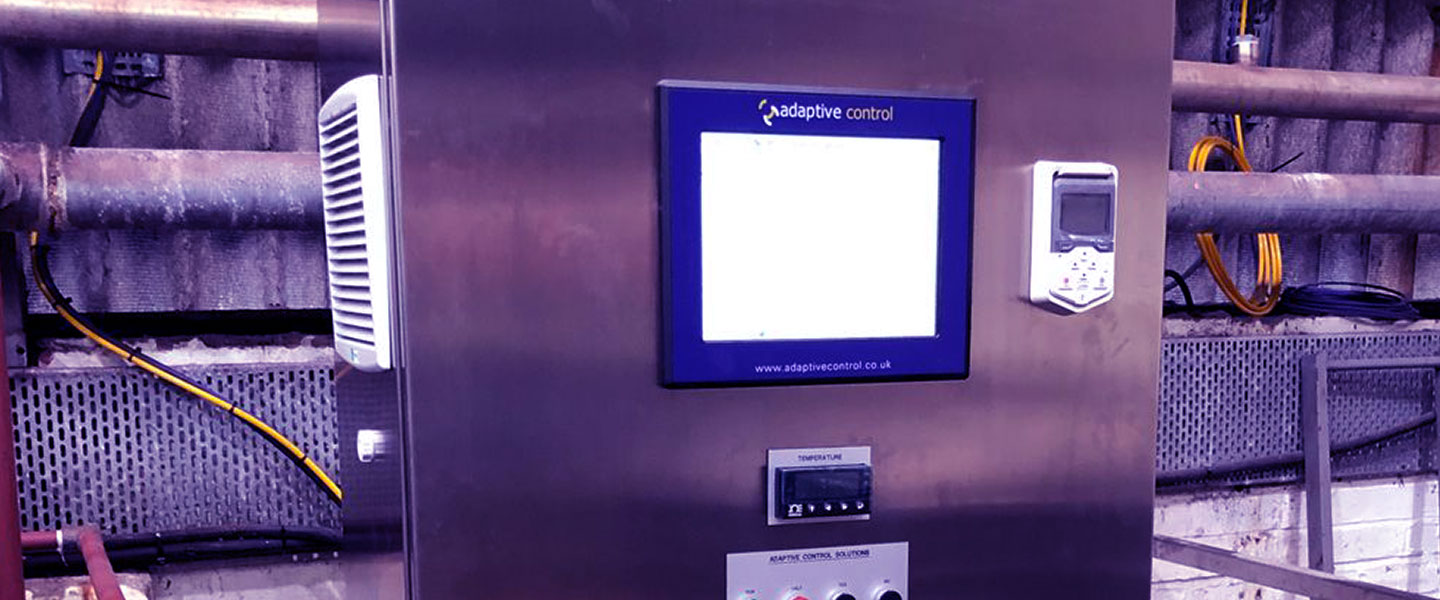