
Most Commonly Used Scala Collections
- 4.1. List Scala lists internally represent an immutable linked list. ...
- 4.2. Set Scala Set is a collection of unique elements. ...
- 4.3. Map A Map is a collection of key/value pairs where keys are always unique. ...
- 4.4. Tuple A Tuple is a collection that gives us a way to store different items in the same container. ...
What are the different types of collections in Scala?
There are two types of collections in Scala – mutable and immutable. Let’s point out some differences. 2.1. Mutable Collections A mutable collection updates or extends in place. This means we can add, change or remove elements of a collection as a side effect.
What is the use of collection immutable in Scala?
Scala.collection.immutable contains all the immutable collections. It does not allow you to modify data. Scala imports this package by default. If you want mutable collection, you must import scala.collection.mutable package in your code. The scala.collection.immutable package contains all the immutable abstract classes and traits for collections.
What is a map in Scala?
A Map is a collection of key/value pairs where keys are always unique. Scala provides mutable and immutable versions of it. By default, an immutable version of the map is imported:
How safe are Scala collections?
Safe: This one has to be experienced to sink in. The statically typed and functional nature of Scala’s collections means that the overwhelming majority of errors you might make are caught at compile-time. The reason is that (1) the collection operations themselves are heavily used and therefore well tested.
See more
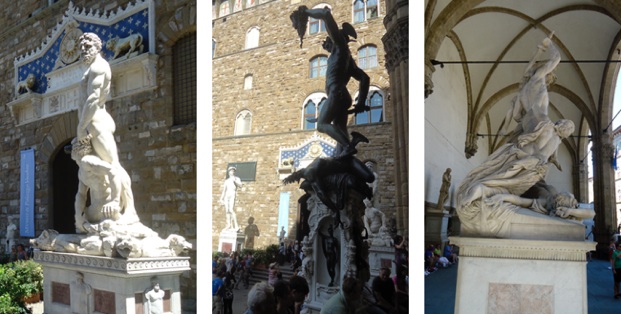
What is list and collection in Scala?
Specific to Scala, a list is a collection which contains immutable data, which means that once the list is created, then it can not be altered. In Scala, the list represents a linked list. In a Scala list, each element need not be of the same data type.
Which is used to collect data in Scala?
Collect function is used to collect elements from the given collection. Collect function can be used with the collection data structure to pick up some elements which satisfy the given condition. Collect function can be used with the mutable and immutable collection data structure in scala.
Does Scala have immutable collections?
A collection in package scala. collection. immutable is guaranteed to be immutable for everyone. Such a collection will never change after it is created.
What is mutable collection?
A mutable collection can be updated or extended in place. This means you can change, add, or remove elements of a collection as a side effect. Immutable collections, by contrast, never change.
What does collect () do in spark?
PySpark Collect() – Retrieve data from DataFrame. Collect() is the function, operation for RDD or Dataframe that is used to retrieve the data from the Dataframe. It is used useful in retrieving all the elements of the row from each partition in an RDD and brings that over the driver node/program.
What are tuples in Scala?
In Scala, a tuple is a value that contains a fixed number of elements, each with its own type. Tuples are immutable. Tuples are especially handy for returning multiple values from a method.
Which is immutable in Scala?
In Scala, all number types, strings, and tuples are immutable.
What is iterator in Scala?
An iterator is not a collection, but rather a way to access the elements of a collection one by one. The two basic operations on an iterator it are next and hasNext. A call to it. next() will return the next element of the iterator and advance the state of the iterator.
What is the difference between SEQ and list in Scala?
A Seq is an Iterable that has a defined order of elements. Sequences provide a method apply() for indexing, ranging from 0 up to the length of the sequence. Seq has many subclasses including Queue, Range, List, Stack, and LinkedList. A List is a Seq that is implemented as an immutable linked list.
Is array immutable in Scala?
The Scala List class holds a sequenced, linear list of items. Following are the point of difference between lists and array in Scala: Lists are immutable whereas arrays are mutable in Scala.
What is ArrayBuffer in Scala?
Scala provides a data structure, the ArrayBuffer, which can change size when initial size falls short. As array is of fix size and more elements cannot be occupied in an array, ArrayBuffer is an alternative to array where size is flexible. Internally ArrayBuffer maintains an array of current size to store elements.
Why is immutability important in Scala?
Immutable objects and data structures are first-class citizens in Scala. This is because they prevent mistakes in distributed systems and provide thread-safe data.
What can be used instead of collect in spark?
Collect action will try to move all data in RDD/DataFrame to the machine with the driver and where it may run out of memory and crash. Instead, you can make sure that the number of items returned is sampled by calling take or takeSample , or perhaps by filtering your RDD/DataFrame.
Does Scala have Dataframes?
The DataFrame API is available in Scala, Java, Python, and R. In Scala and Java, a DataFrame is represented by a Dataset of Row s. In the Scala API, DataFrame is simply a type alias of Dataset[Row] . While, in Java API, users need to use Dataset
How do I get spark data?
To read a CSV file you must first create a DataFrameReader and set a number of options.df=spark.read.format("csv").option("header","true").load(filePath)csvSchema = StructType([StructField(“id",IntegerType(),False)])df=spark.read.format("csv").schema(csvSchema).load(filePath)More items...•
What is foldLeft in Scala?
foldLeft() method is a member of TraversableOnce trait, it is used to collapse elements of collections. It navigates elements from Left to Right order. It is primarily used in recursive functions and prevents stack overflow exceptions.
How many loops are needed for collection processing?
This code is much more concise than the one to three loops required for traditional collection processing (three loops for an array, because the intermediate results need to be buffered somewhere else). Once you have learned the basic collection vocabulary you will also find writing this code is much easier and safer than writing explicit loops.
What is collection framework?
The collections framework is the heart of the Scala 2.13 standard library. It provides a common, uniform, and all-encompassing framework for collection types. This framework enables you to work with data in memory at a high level, with the basic building blocks of a program being whole collections, instead of individual elements.
Why is Scala statically typed?
The statically typed and functional nature of Scala’s collections means that the overwhelming majority of errors you might make are caught at compile-time. The reason is that (1) the collection operations themselves are heavily used and therefore well tested.
What is universal collection?
Universal: Collections provide the same operations on any type where it makes sense to do so. So you can achieve a lot with a fairly small vocabulary of operations. For instance, a string is conceptually a sequence of characters. Consequently, in Scala collections, strings support all sequence operations. The same holds for arrays.
How many methods are needed to solve collection problems?
Easy to use: A small vocabulary of 20-50 methods is enough to solve most collection problems in a couple of operations. No need to wrap your head around complicated looping structures or recursions. Persistent collections and side-effect-free operations mean that you need not worry about accidentally corrupting existing collections with new data. Interference between iterators and collection updates is eliminated.
What is parallel collection?
Parallel: The scala-parallel-collections module provides parallel execution of collections operations across multiple cores. Parallel collections generally support the same operations as sequential ones. You can turn a sequential collection into a parallel one simply by invoking the par method.
What is concise in algebra?
Concise: You can achieve with a single word what used to take one or several loops. You can express functional operations with lightweight syntax and combine operations effortlessly, so that the result feels like a custom algebra.
What is Scala Collection Immutable?
Scala.collection.immutable contains all the immutable collections. It does not allow you to modify data. Scala imports this package by default. If you want mutable collection, you must import scala.collection.mutable package in your code.
What is a mutable collection in Scala?
Scala provides rich set of collection library. It contains classes and traits to collect data. These collections can be mutable or immutable. You can use them according to your requirement. Scala.collection.mutable package contains all the mutable collections. You can add, remove and update data while using this package.
What is Scala Traversable?
Scala Traversable. It is a trait and used to traverse collection elements. It is a base trait for all scala collections. It implements the methods which are common to all collections.
What is a collection in Scala?
Collections in Scala are nothing but a container where the list of data items can be placed and processed together within the memory. It consists of both mutable (scala.collection.mutable) and immutable (scala.collection.immutable) collections which in-turn varies functionally with Val and Var and also, Scala collections provide a wide range of flexible built-in methods, which can be used to perform various operations like transformations & actions, directly on the data items.
What is traversable in data?
Traversable: helps us to traverse through the entire collection and it implements the behavior that are common to all the collections irrespective of the data types. It simply means Traversable lets us traverse the collections repeatedly in terms of for each method.
Why is a collection useful?
As we have seen so far, collections are way useful to store and retrieve formatted items of the respective data types. Also, it comes with various methods to add/ change or delete items from the collection. It is even adaptable to use in most critical scenarios, as it provides mutable and immutable collections.
What does lazy collection mean in Scala?
It means, the memory will not be allocated immediately and it creates the new collection when there is a demand. Collection classes can be converted into Lazy Collection by creating a view on the collection. Let us look into the Scala REPL example for a better understanding.
What is lazy collection?
In a Lazy Collection, the transformations will not create another collection upfront. It means, the memory will not be allocated immediately and it creates the new collection when there is a demand. Collection classes can be converted into Lazy Collection by creating a view on the collection.
Why can a collection be traversed only once?
Note: By using an iterator, the collection can be traversed only once, because each element is consumed during the iteration process.
Does mutable collection have +=?
Note: Mutable collections doesn’t have += method to append and reassign.
What is set in Scala?
Set. It is a collection of elements that are of the same type but do not contain the same elements. By default, scala uses an immutable set. Scala provides mutable and immutable versions of Set. If you want to use an immutable set then use Set and if you want to use mutable set the use mutable.
What is set.++ used for?
Set.++ () – It is used to concatenate the two or more sets.
What is iterator used for?
It is used to access the elements of the collection one by one. Two important operations of an iterator are –
What is a tuple productiterator?
Tuple.productIterator () – It is used to iterate over all the elements of a Tuple.
What is a tuple?
Tuples. It is a collection of heterogeneous types of objects that is different types of objects which combine a fixed number of items together. A tuple that contains an Int and a String: val i = (1, "intellipaat") To access tuple elements ‘._’ is used. e.g.
What is the infix operator for a list?
All lists are built from two more fundamental constructors that are Nil and::.Nil represents an empty list. The infix operator:: expresses list extension. That is, x:: xs represents a list whose first element is x, which is followed by list xs. You can also define the list as follows:
What is set.intersect used for?
Set.intersect – It is used to find the common values between two sets.
What is a Scala set?
Scala Set is a collection of pairwise different elements of the same type. In other words, a Set is a collection that contains no duplicate elements. TreeSet implements immutable sets and keeps elements in sorted order.
What is a set in Scala?
Scala Set is a collection of pairwise different elements of the same type. In other words, a Set is a collection that contains no duplicate elements. HashSet implements immutable sets and uses hash table. Elements insertion order is not preserved.
What is lazy collection?
Lazy collections have elements that may not consume memory until they are accessed, like Ranges. Additionally, collections may be mutable (the contents of the reference can change) or immutable (the thing that a reference refers to is never changed). Note that immutable collections may contain mutable items.
What is the upper limit of a tuple in Scala?
Tuples are of type Tuple1, Tuple2, Tuple3 and so on. There currently is an upper limit of 22 in the Scala if you need more, then you can use a collection, not a tuple. For each TupleN type, where 1 <= N <= 22, Scala defines a number of element-access methods. Given the following definition −.
What is a listmap in Scala?
Scala map is a collection of key/value pairs. Any value can be retrieved based on its key. Keys are unique in the Map, but values need not be unique. ListMap implements immutable map and uses list to implement the same. It is used with small number of elements.
What is the purpose of Scala?
Scala provides a data structure, the ListBuffer, which is more efficient than List while adding/removing elements in a list. It provides methods to prepend, append elements to a list.
What is a collection in Scala?
Scala has a rich set of collection library. Collections are containers of things. Those containers can be sequenced, linear sets of items like List, Tuple, Option, Map, etc. The collections may have an arbitrary number of elements or be bounded to zero or one element (e.g., Option).
What is iterable trait?
The Iterable trait is the next trait from the top of the hierarchy and a base trait for iterable collections. It defines an iterator which allows us to loop through a collection’s elements one at a time. When we use an iterator, we can traverse the collection only once.
What is a Scala list?
Scala lists internally represent an immutable linked list. It maintains the order of elements and can contain duplicates as well. This class is optimal for last-in-first-out (LIFO), stack-like access patterns.
What are the two types of collections in Scala?
There are two types of collections in Scala – mutable and immutable. Let’s point out some differences.
What is a set in Scala?
Scala Set is a collection of unique elements. By default, Scala uses an immutable set. It doesn’t maintain any order for storing elements.
What are the three main categories of Scala?
Scala’s collection classes begin with the Traversable and Iterable traits. These traits branch into three main categories: List, Set, and Map.
What class implements abstract members?
This List class comes with two implementing case classes, scala.Nil and scala.:: , that implement the abstract members isEmpty, head, and tail. A Scala list containing elements can be represented using x :: xs, where x is the head and the xs is the remaining list. Nil represents an empty list:
What is the operation of a list?
A list has O (1) prepend and head/tail access. Most other operations are O (n) though; this includes length, append, reverse, and also the index-based lookup of elements.
What is mutable collection in Scala?
Scala collections systematically distinguish between mutable and immutable collections. A mutable collection can be updated or extended in place. This means you can change, add, or remove elements of a collection as a side effect. Immutable collections, by contrast, never change.
What is the difference between a root and an immutable collection?
The difference between root collections and immutable collections is that clients of an immutable collection have a guarantee that nobody can mutate the collection, whereas clients of a root collection only promise not to change the collection themselves. Even though the static type of such a collection provides no operations for modifying ...
What is the last package in the collection hierarchy?
The last package in the collection hierarchy is collection.generic. This package contains building blocks for implementing collections. Typically, collection classes defer the implementations of some of their operations to classes in generic. Users of the collection framework on the other hand should need to refer to classes in generic only in exceptional circumstances.
What are the three types of collections?
Most of the classes in the collections hierarchy exist in three variants: root, mutable, and immutable. The only exception is the Buffer trait which only exists as a mutable collection.
Can you use both mutable and immutable collections?
A useful convention if you want to use both mutable and immutable versions of collections is to import just the package collection.mutable.
Does Scala have immutable collections?
By default, Scala always picks immutable collections. For instance, if you just write Set without any prefix or without having imported Set from somewhere, you get an immutable set, and if you write Iterable you get an immutable iterable collection, because these are the default bindings imported from the scala package.
Is a collection immutable?
A collection in package scala .collection.immutable is guaranteed to be immutable for everyone. Such a collection will never change after it is created. Therefore, you can rely on the fact that accessing the same collection value repeatedly at different points in time will always yield a collection with the same elements.
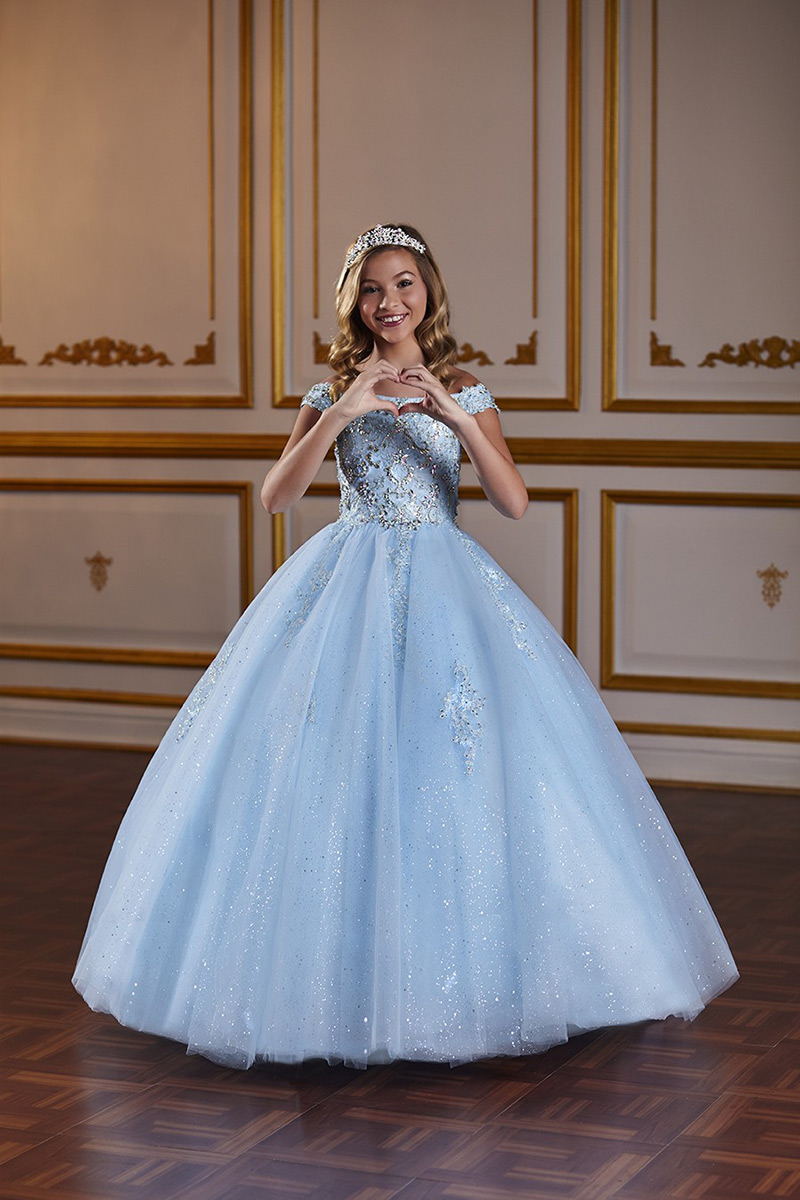