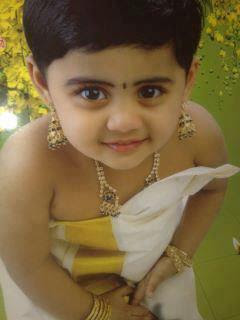
- RequiredFieldValidator. This is an elementary validation control. Almost all the forms have some fields that are mandatory to be filled up by the user before proceeding forward.
- RangeValidator. The RangeValidator control simply specifies the permitted range within which the input value should fall.
- RegularExpressionValidator. RegularExpressions, or simply Regex, are patterns that define the format of the text. If the text is in the same format, Regex returns true, else false.
- CompareValidator. The CompareValidator control compares the value of one control with either a fixed value or a value in another control.
- CustomValidator. ASP.Net also allows the freedom of writing your own validator. This eases the task of a developer to validate the form at the client side itself.
- ValidationSummary. The ValidationSummary control does not perform any validation. Its purpose is to display a summary of all the errors on the page.
- RequiredFieldValidator.
- RangeValidator.
- CompareValidator.
- RegularExpressionValidator.
- CustomValidator.
- ValidationSummary.
How many types of validation controls are there in ASP NET?
There are six types of validation controls in ASP.NET RequiredFieldValidation Control CompareValidator Control RangeValidator Control RegularExpressionValidator Control CustomValidator Control ValidationSummary
What is a validator in ASP NET?
ASP.NET - Validators. ASP.NET validation controls validate the user input data to ensure that useless, unauthenticated, or contradictory data don't get stored.
What is the difference between one validation control and multiple validation?
One validation control will validate only one input control but multiple validate control can be assigned to a input control. Usually, Validation is invoked in response to user actions like clicking submit button or entering data.
What is the validationsummary control used for?
The ValidationSummary control is reporting control, which is used by the other validation controls on a page. You can use this validation control to consolidate errors reporting for all the validation errors that occur on a page instead of leaving this up to each and every individual validation control.
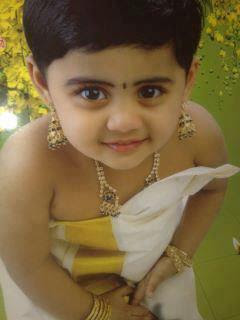
How many types of validation server controls are there?
6 typesThere are 6 types of validation controls available : RequiredFieldValidator. CompareValidator. RangeValidator.
What is validation control how many types of validation control?
Define Validation Control in ASP.NET.Sr. No.Validation control1.RequiredFieldValidation Control2.CompareValidator Control3.RangeValidator Control4.RegularExpressionValidator Control2 more rows
How many types of validation controls are there in ASP.NET Explain with examples?
Validation can be classified into two types based on client and server validation: Client side Validation. Server side Validation.
How many types of validation are there in ASP NET MVC?
The following three type of validations we can do in ASP.NET MVC web applications: HTML validation / JavaScript validation. ASP.NET MVC Model validation. Database validation.
What are the different types of validation controls?
ASP.NET provides the following validation controls:RequiredFieldValidator.RangeValidator.CompareValidator.RegularExpressionValidator.CustomValidator.ValidationSummary.
What is validation control and its types?
ASP.Net provides various validation controls that validate the user data to ensure that the data entered by the user are satisfied with the condition. ASP.Net provides RequiredFieldValidator, RangeValidator, CompareValidator, RegularExpressionValidator, CustomValidator and ValidationSummary.
What are validation controls?
Validation controls are used to, Implement presentation logic. To validate user input data. Data format, data type and data range is used for validation.
What is validation in ASP.NET MVC?
Validation is an important aspect in ASP.NET MVC applications. It is used to check whether the user input is valid. ASP.NET MVC provides a set of validation that is easy-to-use and at the same time, it is also a powerful way to check for errors and, if necessary, display messages to the user.
What is ViewBag and ViewData?
ViewData is a dictionary of objects that is derived from ViewDataDictionary class and accessible using strings as keys. ViewBag is a dynamic property that takes advantage of the new dynamic features in C# 4.0. ViewData requires typecasting for complex data type and check for null values to avoid error.
BaseValidator Class
The validation control classes are inherited from the BaseValidator class hence they inherit its properties and methods. Therefore, it would help to take a look at the properties and the methods of this base class, which are common for all the validation controls:
RequiredFieldValidator Control
The RequiredFieldValidator control ensures that the required field is not empty. It is generally tied to a text box to force input into the text box.
RangeValidator Control
The RangeValidator control verifies that the input value falls within a predetermined range.
CompareValidator Control
The CompareValidator control compares a value in one control with a fixed value or a value in another control.
RegularExpressionValidator
The RegularExpressionValidator allows validating the input text by matching against a pattern of a regular expression. The regular expression is set in the ValidationExpression property.
CustomValidator
The CustomValidator control allows writing application specific custom validation routines for both the client side and the server side validation.
ValidationSummary
The ValidationSummary control does not perform any validation but shows a summary of all errors in the page. The summary displays the values of the ErrorMessage property of all validation controls that failed validation.
Why is validation important in ASP.NET?
Validation is important part of the user interface of a Web application. ASP.NET provides a list of validator controls to validate user input. In this article, I will explain the Validation Controls in ASP.NET and how to use them effectively.
What is requiredfieldvalidator?
The RequiredFieldValidator control is simple validation control, which checks to see if the data is entered for the input control. You can have a RequiredFieldValidator control for each form element on which you wish to enforce Mandatory Field rule.
Why is ASP.NET important?
An important aspect of creating ASP.NET Web pages for user input is to be able to check that the information users enter is valid. ASP.NET provides a set of validation controls that provide an easy-to-use but powerful way to check for errors and, if necessary, display messages to the user.
When is validation invoked?
Usually, Validation is invoked in response to user actions like clicking submit button or entering data. Suppose, you wish to perform validation on page when user clicks submit button. Server validation will only performed when CauseValidation is set to true.
Can you use ASP.NET validation?
You can use ASP.NET validation, which will ensure client, and server validation. It work on both end; first it will work on client validation and than on server validation. At any cost server validation will work always whether client validation is executed or not. So you have a safety of validation check.
Does server validation need to be the same as client validation?
The server side validation you write does not need to provide the exact same validation as that of the client side validation. The client side validation can check for the user input data for range and type and server side validation can check for matching of data with database.
Validation Controls in ASP.NET
In fact, ASP.NET provides following six types of validation controls that you can use to validate the data of a Web Form.
Summary
This article has explained the server-side validation using several Examples of Validation Controls in ASP.NET. Basically, ASP.NET provides six validation controls that we can use to perform validation of input values entered by the user in a Web Form control.
What is required field validator?
Required field validator can be used to verify whether user is providing input into the textbox or not, if user is not providing input text error message will be displayed.
What is controltovalidate?
1. ControltoValidate: Specify standard webserver controlID to be validated with user input. Text: Specify text to be displayed if user input is invalid, this will be displayed with validation control.
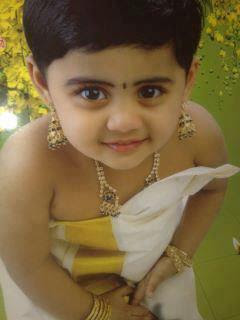
BaseValidator Class
RequiredFieldValidator Control
RangeValidator Control
CompareValidator Control
RegularExpressionValidator
CustomValidator
ValidationSummary
Validation Groups
Example
- The CustomValidator control allows writing application specific custom validation routines for both the client side and the server side validation. The client side validation is accomplished through the ClientValidationFunction property. The client side validation routine should be written in a scripting language, such as JavaScript or VBScript, wh...