
We come across many different types of classes in C# programming:
- Abstract class
- Concrete class
- Sealed class
- Static class
- Instance class
- Partial class
- Inner/Nested class
What are classes and objects in C?
Classes and Objects are basic concepts of Object Oriented Programming which revolve around the real life entities. Class A class is a user defined blueprint or prototype from which objects are created.
How many types classes in C sharp?
Types of classes: 1. Abstract class 2. Partial class 3. Sealed class 4. Static class. There are four types of classes in C# .Net 1) Abstract class - An Abstract Class means that, no object of this class can be instantiated, but can make derivation of this. It can serve the purpose of base class only as no object of this class can be created.
Does C have classes?
This document describes the simplest possible coding style for making classes in C. It will describe constructors, instance variables, instance methods, class variables, class methods, inheritance, polymorphism, namespaces with aliasing and put it all together in an example project. 1. C Classes
What are the different storage classes in C?
There are four storage classes types in C:
- auto
- register
- static
- extern

What are the types of classes in C?
There are four different types of storage classes that we use in the C language:Automatic Storage Class.External Storage Class.Static Storage Class.Register Storage Class.
How many types of classes are there?
There are seven types of classes in Java: Static Class. Final Class. Abstract Class.
What are the three types of classes?
Sociologists generally posit three classes: upper, working (or lower), and middle.
What are classes types?
In computer science, a type class is a type system construct that supports ad hoc polymorphism. This is achieved by adding constraints to type variables in parametrically polymorphic types.
What is the difference between types and classes?
The class defines object's internal state and the implementation of its operations. In contrast, an object's type only refers to its interface - a set of requests to which it can respond. An object can have many types, and objects of different classes can have the same type.
What are the types of base class?
Base Classes and Derived ClassesBase classDerived classesShapeCircle, Triangle, Rectangle, Sphere, CubeLoanCarLoan, HomeImprovementLoan, MortgageLoanEmployeeFaculty, StaffAccountCheckingAccount, SavingsAccount1 more row
What is a static class?
A static class in C# is a class that cannot be instantiated. A static class can only contain static data members including static methods, static constructors, and static properties. In C#, a static class is a class that cannot be instantiated.
What is upper class and lower class?
The Upper Class vs. Its application to income, education, and social status varies based on location and other factors. Many people who make up the middle-class work as professionals and civil servants, and own property. The working or lower class refers to those who make up the lowest level of society.
How are classes divided?
One objective way some researchers divide individuals into economic classes is by looking at their income. From that data, they split earners into different classes such as poor, lower-middle class, middle class, upper-middle class and wealthy.
What are type classes used for?
Type classes are a powerful tool used in functional programming to enable ad-hoc polymorphism, more commonly known as overloading.
What is type class variable?
In object-oriented programming with classes, a class variable is a variable defined in a class of which a single copy exists, regardless of how many instances of the class exist. A class variable is not an instance variable. It is a special type of class attribute (or class property, field, or data member).
Are classes object types?
Object vs Class The difference between object and class should be intuitive to most programmers: Class is a blueprint or template from which objects are created. Object is an instance of a class. In above example A is a class, but a is pointing to an object.
What are classes?
A class is a user-defined data type. It holds its own data members and member functions, which can be accessed and used by creating an instance of that class. A class is like a blueprint for an object.
Types of classes
There are four distinct types of classes which are differentiated based on implementation. They are:
Stand Alone Classes
As the name suggests, these classes are neither child classes (i.e they are not derived out of any classes) nor are they parent classes (i.e they do not act as a base class)
Concrete Base Classes and Concrete Derived Classes
A concrete class has well defined member functions. Their functions are not virtual or pure virtual (explained later).
Abstract Base Classes and Abstract Derived Classes
Before we dive into the definition of the class, let us first understand what virtual functions and pure virtual functions are:
Abstract base class
A class containing the pure virtual function cannot be used to declare the objects of its own .Such classes are known as abstract base classes.
Abstract derived class
A class which is derived from an abstract base class is called as an abstract derived class.The main objective of the abstract derived class is to inherit the features of the abstract base class. The remaining functions are all virtual functions. It is still an abstract class and hence objects of the class cannot be defined.
What is a class in Java?
Classes are reference types that hold the object created dynamically in a heap. All classes have a base type of System.Object. The default access modifier of a class is Internal. The default access modifier of methods and variables is Private. Directly inside the namespaces declarations of private classes are not allowed.
What is C# programming?
As we know, C# is a pure Object Oriented Programming language that provides the ability to reuse existing code. To reuse existing code C# provides various types of object oriented concepts to complete the realastic requirements of a specific business.To learn about the various programming languages, please refer to the following article of mine:
What is abstract class?
What an Abstract class is. An Abstract class is a class that provides a common definition to the subclasses and this is the type of class whose object is not created. Some key points of Abstract classes are: Abstract classes are declared using the abstract keyword. We cannot create an object of an abstract class.
How are abstract classes declared?
Abstract classes are declared using the abstract keyword. We cannot create an object of an abstract class. If you want to use it then it must be inherited in a subclass. An Abstract class contains both abstract and non-abstract methods. The methods inside the abstract class can either have an implementation or no implementation.
What is a sealed class?
A Sealed class is a class that cannot be inherited and used to restrict the properties. The following are some key points: A Sealed class is created using the sealed keyword. Access modifiers are not applied to a sealed class. To access the sealed members we must create an object of the class. For example:
Can methods inside an abstract class be private?
An Abstract class has only one subclass. Methods inside the abstract class cannot be private.
Can inheritance be applied to partial classes?
If you seal a specific part of a partial class then the entire class is sealed, the same as for an abstract class. Inheritance cannot be applied on partial classes. The classes that are written in two class files are combined together at run time.
What is partial class?
Partial class. The partial keyword indicates that other parts of the class, struct, or interface can be defined in the namespace. All the parts must use the partial keyword. All the parts must be available at compile time to form the final type.
What are the characteristics of static class?
Characteristics of static class. Static class cannot instantiated using a new keyword. Static items can only access other static items. For example, a static class can only contain static members, e.g. variable, methods etc. A static method can only contain static variables and can only access other static items.
What does an abstract class mean?
Abstract class. A class with abstract modifier indicate that class is abstract class. An abstract class cannot be instantiated. The purpose of an abstract class is to provide a common definition of a base class that multiple derived classes can share.
Do all partial classes have the same access?
All the partial class definitions must be in the same assembly and namespace. All the parts must have the same accessibility like public or private, etc. If any part is declared abstract, sealed or base type then the whole class is declared of the same type.
What is the key to C programming?
Coding with C is highly centered upon using variables in every program. Those are the key aspects of C programming. Every variable in C has two properties; type and storage classes. Among them, the type refers to the data type of the variable, and storage classes in C determine the scope, lifetime, and visibility of the variable.
Is there a storage class specifier?
There is at least one storage class specifier is given in the variable declaration. But, in case, there is no storage class specifier specified, the following rules are followed: 1. Variables declared within a function are considered as auto. 2. Functions declared within a function are considered as an extern.
The auto Storage Class
The auto storage class is the default storage class for all local variables.
The register Storage Class
The register storage class is used to define local variables that should be stored in a register instead of RAM. This means that the variable has a maximum size equal to the register size (usually one word) and can't have the unary '&' operator applied to it (as it does not have a memory location).
The static Storage Class
The static storage class instructs the compiler to keep a local variable in existence during the life-time of the program instead of creating and destroying it each time it comes into and goes out of scope. Therefore, making local variables static allows them to maintain their values between function calls.
The extern Storage Class
The extern storage class is used to give a reference of a global variable that is visible to ALL the program files. When you use 'extern', the variable cannot be initialized however, it points the variable name at a storage location that has been previously defined.
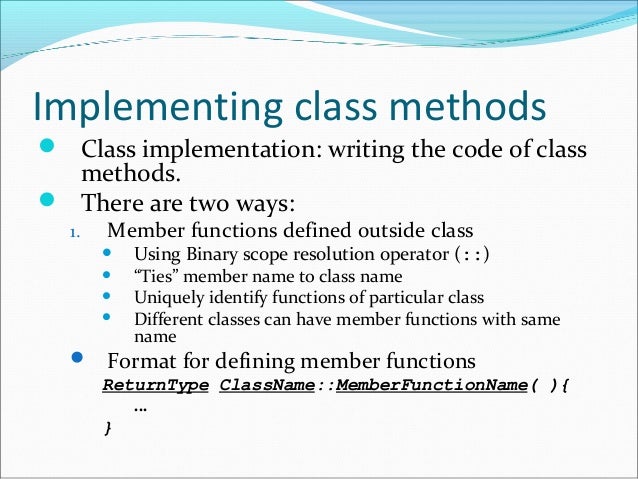