
What are the applications of stack?
Application of the Stack A Stack can be used for evaluating expressions consisting of operands and operators. Stacks can be used for Backtracking, i.e., to check parenthesis matching in an expression. It can also be used to convert one form of expression to another form. It can be used for systematic Memory Management.
What are the applications of queue?
Application of Queue in Data StructureManaging requests on a single shared resource such as CPU scheduling and disk scheduling.Handling hardware or real-time systems interrupts.Handling website traffic.Routers and switches in networking.Maintaining the playlist in media players.
What are the examples of stacks and queues?
Phone answering system: The person who calls first gets a response first from the phone answering system. The person who calls last gets the response last. Therefore, it follows first-in-first-out (FIFO) strategy of queue. Luggage checking machine: Luggage checking machine checks the luggage first that comes first.
What are the 6 applications of stack?
Following are the applications of stack:Expression Evaluation.Expression Conversion. i. Infix to Postfix. ii. Infix to Prefix. iii. Postfix to Infix. iv. Prefix to Infix.Backtracking.Memory Management.
Where are stacks used in real life?
Examples of stacks in "real life": The stack of trays in a cafeteria; A stack of plates in a cupboard; A driveway that is only one car wide.
Where is queue used in real life?
A real-world example of queue can be a single-lane one-way road, where the vehicle enters first, exits first. More real-world examples can be seen as queues at the ticket windows and bus-stops.
Which is one of common example of stack?
A pile of books, a stack of dinner plates, a box of pringles potato chips can all be thought of examples of stacks. The basic operating principle is that last item you put in is first item you can take out. That is, that a stack is a Last In First Out (LIFO) structure.
What are the applications of priority queue?
Applications of Priority queueIt is used in the Dijkstra's shortest path algorithm.It is used in prim's algorithm.It is used in data compression techniques like Huffman code.It is used in heap sort.It is also used in operating system like priority scheduling, load balancing and interrupt handling.
Is printing an application of queue?
Print queues A print queue on a computer has print jobs that are waiting to be sent to the print processor. The print processor then formats a print job and sends it to the printer or other print device (for example, a plotter) for printing.
Which is not an application of queue?
Explanation: The answer is d. The options a, b, and c are the applications of the Queue data structure while option d, i.e., balancing of symbols is not the application of the Queue data structure.
What are the different types of queues?
There are four different types of queues:Simple Queue.Circular Queue.Priority Queue.Double Ended Queue.
What is stack stacks with linked lists?
In particular, we maintain an instance variable first that stores a reference to the most recently inserted item. This policy allows us to add and remove items at the beginning of the linked list without accessing the links of any other items in the linked list.
What is stack in LIFO?
A stack is a collection that is based on the last-in-first-out (LIFO) policy. By tradition, we name the stack insert method push () and the stack remove operation pop () . We also include a method to test whether the stack is empty, as indicated in the following API:
What is a queue in a FIFO?
A queue supports the insert and remove operations using a first-in first-out (FIFO) discipline. By convention, we name the queue insert operation enqueue and the remove operation dequeue, as indicated in the following API:
What are the two types of data types used to manipulate arbitrarily large collections of objects?
In this section, we introduce two closely-related data types for manipulating arbitrarily large collections of objects: the stack and the queue . Stacks and queues are special cases of the idea of a collection. Each is characterized by four operations: create the collection, insert an item, remove an item, and test whether the collection is empty .
How to use function call abstraction?
Function-call abstraction. Most programs use stacks implicitly because they support a natural way to implement function calls, as follows: at any point during the execution of a function, define its state to be the values of all of its variables and a pointer to the next instruction to be executed. The natural way to implement the function-call abstraction is to use a stack. To call a function, push the state on a stack. To return from a function call, pop the state from the stack to restore all variables to their values before the function call and resume execution at the next instruction to be executed.
How to list files with a stack?
Listing files with a stack. Write a program that takes the name of a directory as a command line argument, and prints out all of the files contained in this directory and any subdirectories. Also prints out the file size (in bytes) of each file. Use a stack instead of a queue. Repeat using recursion and name your program DirectoryR.java . Modify DirectoryR.java so that it prints out each subdirectory and its total size. The size of a directory is equal to the sum of all of the files it contains or that its subdirectories contain.
How to implement a generic collection?
To implement a generic collection, we specify a type parameter , such as Item, in angle brackets and use that type parameter in our implementation instead of a specific type. For example, Stack.java is generic version of LinkedStackOfStrings.java
What is stack in programming?
A stack is a very effective data structure for evaluating arithmetic expressions in programming language s. An arithmetic expression consists of operands and operators.
What do you mean by Stack?
A Stack is a widely used linear data structure in modern computers in which insertions and deletions of an element can occur only at one end, i.e., top of the Stack. It is used in all those applications in which data must be stored and retrieved in the last.
Why is the stack the best data structure for evaluating the postfix expression?
Stack is the ideal data structure to evaluate the postfix expression because the top element is always the most recent operand. The next element on the Stack is the second most recent operand to be operated on.
What is backtracking in stack?
Backtracking is another application of Stack. It is a recursive algorithm that is used for solving the optimization problem.
What is the reverse order of the calling sequence?
Thus the program that calls several functions in succession can be handled optimally by the stack data structure. Control returns to each function at a correct place, which is the reverse order of the calling sequence.
What is stack in data?
Stack A stack is a linear data structure in which elements can be inserted and deleted only from one side of the list, called the top. A stack follows the LIFO (Last In First Out) principle, i.e., the element inserted at the last is the first element to come out.
What is a queue in a list?
Queue: A queue is a linear data structure in which elements can be inserted only from one side of the list called rear, and the elements can be deleted only from the other side called the front.
Where does insertion take place in a queue?
Insertion and deletion in queues takes place from the opposite ends of the list. The insertion takes place at the rear of the list and the deletion takes place from the front of the list.
How many pointers are there in a queue?
In queues we maintain two pointers to access the list. The front pointer always points to the first element inserted in the list and is still present, and the rear pointer always points to the last inserted element.
When to use queue?
The queue is used when things don’t have to be processed immediately, but have to be processed in First In First Out order like Breadth First Search. This property of Queue makes it also useful in the following kind of scenarios. When a resource is shared among multiple consumers.
What is a queue in a system?
A Queue is a linear structure which follows a particular order in which the operations are performed. The order is First In First Out (FIFO). A good example of a queue is any queue of consumers for a resource where the consumer that came first is served first. In this article, the different types of queues are discussed.
What are the different types of queues?
There are five different types of queues which are used in different scenarios. They are: Circular Queue: Circular Queue is a linear data structure in which the operations are performed based on FIFO (First In First Out) principle and the last position is connected back to the first position to make a circle. It is also called ‘Ring Buffer’.
How many types of queues are there?
There are five different types of queues which are used in different scenarios. They are:
Can deque be used as clockwise?
Since Deque supports both stack and queue operations, it can be used as both. The Deque data structure supports clockwise and anticlockwise rotations in O (1) time which can be useful in certain applications. Also, the problems where elements need to be removed and or added both ends can be efficiently solved using Deque.
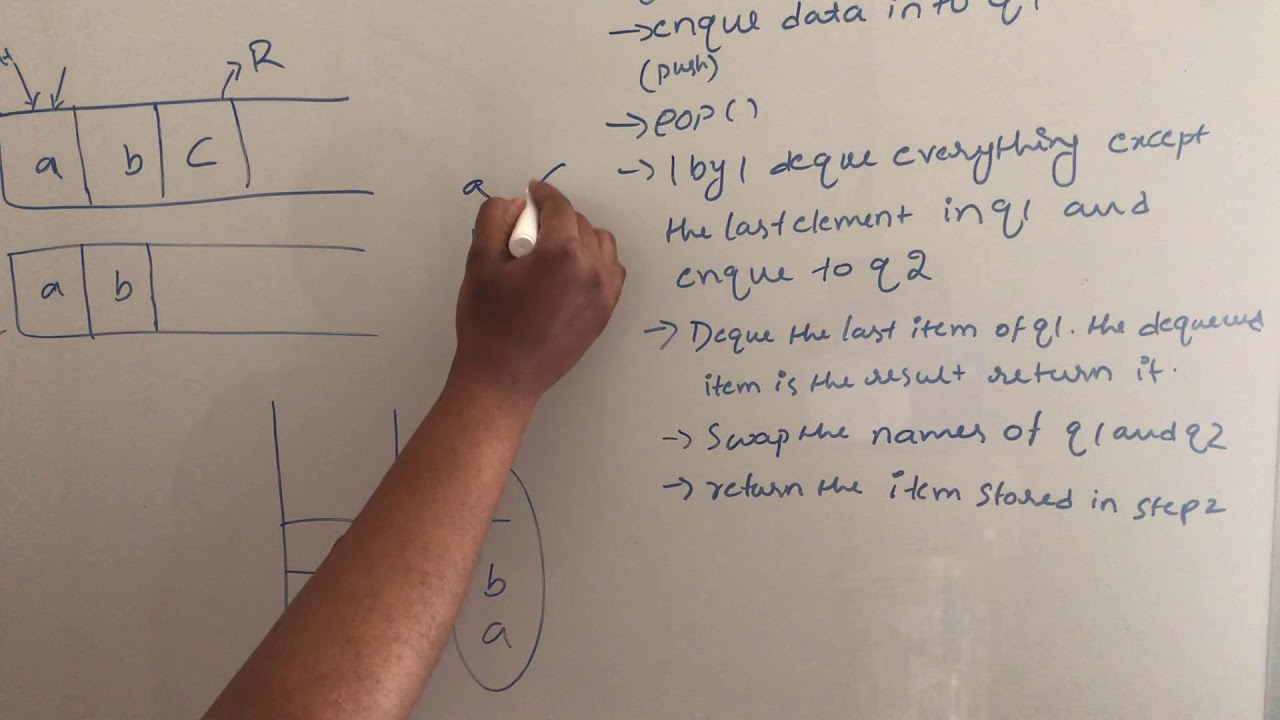