
- You can add properties to almost everything. ...
- Functions are objects. ...
- for...in loops iterate over property names, not values. ...
- Variable scoping. ...
- Variables that aren't explicitly declared can be global. ...
- Understand how .prototype works.
See more
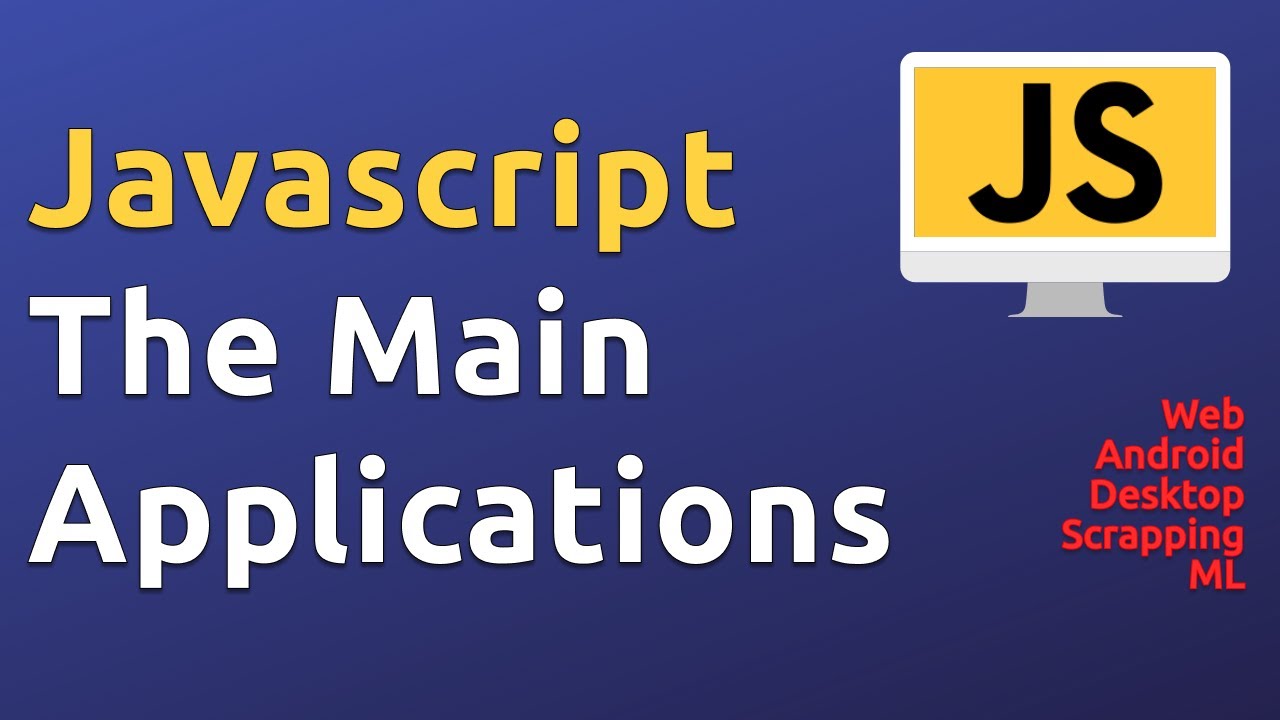
What are 5 things JavaScript can do?
Here are some basic things JavaScript is used for:Adding interactive behavior to web pages. JavaScript allows users to interact with web pages. ... Creating web and mobile apps. ... Building web servers and developing server applications. ... Game development.
Is JavaScript OK for beginners?
JavaScript is beginner-friendly The great thing about it is that it comes installed on every modern web browser—there's no need to set up any kind of development environment, which means you can start coding with JavaScript right away!
How can I teach myself JavaScript?
The 5 Best Ways to Learn JavaScript FastSelf-Guided Websites and Courses. The Internet is, above all else, a repository of knowledge. ... Books. When in doubt, read a book. ... Coding Boot Camps. Maybe the self-taught route isn't for you. ... Meetups and Networking Events. ... Starting Your Own Projects.
How many days it will take to learn JavaScript?
Here's the short answer: most programmers agree that it takes six to nine months to develop a working proficiency in JavaScript. And even then, you'll spend years learning new skills and developing your understanding of it.
Is JavaScript worth learning in 2022?
Yes, JavaScript is worth learning in 2022. It ranks as the most widely used programming language and it has held this position for nine years in a row. JavaScript is the only programming language native to web browsers. Therefore, as long as the Internet is around, it will always be relevant.
Should I learn JavaScript or HTML first?
HTMLIdeally you'll learn HTML first, then CSS, and then finish with JavaScript, as they build on each other in that order.
Is JavaScript difficult to learn?
Arguably, JavaScript is one of the easiest programming languages to learn, so it serves as a great first language for anyone brand new to coding. Even the most complex lines of JavaScript code can be written one by one, in fragments. It can also be tested in the web browser at the same time.
Can I learn JavaScript in 3 months?
However, unlike CSS and HTML, JavaScript is not something that can be aced in just two weeks. But, it can be done in just three months! Most employers will be happy to hire you as their web developers if you just master some of the JavaScript basics. Of course, learning never stops.
When was JavaScript first used?
JavaScript has clearly come a long way since it was created ( purportedly in ten days) in 1995. Now, technologies like node.js allow JavaScript to be run outside of any browser. Some common use cases for JavaScript as a general scripting language include:
What does "what's this" mean in JavaScript?
What is this ?” In other languages, this means the instance of the class that you’re looking at, but in JavaScript it means the object that your function got called on. If you’d done this:
What is unobtrusive JavaScript?
This approach, sometimes called “unobtrusive JavaScript,” has several advantages, including: It usually makes HTML code more concise and readable.
How to make sure variables aren't global in JavaScript?
That means if you want to make sure your variables aren’t global, you should put them in a function, and then call that function. (function() { var message = "hello world"; alert (message); }) ();
How does JavaScript avoid problems?
JavaScript avoids these issues entirely by only allowing one piece of code to run at a time. This is great, but it requires us to write our code in a different way. Most languages create a layer of abstraction over operations where the program is waiting for something to happen. For example, in Java, you can call Thread.sleep (100), and the rest of your procedure won’t run until 100 milliseconds have passed.
What are the three types of primitive data types in JavaScript?
JavaScript has only three primitive data types: String, Number, and Boolean. Everything else (if it’s not null or undefined) can have properties added to it. Note: Even though String is a primitive type ( "hi" ), there is another incarnation of String in JavaScript which is an object ( new String ("hi") ).
Is JavaScript a drawback?
This works fine for small tutorials, but it has some serious drawbacks when you’re writing real applications. It mixes the programmatic behavior of the UI elements (represented by JavaScript) into the structure and data that is (represented by HTML). HTML should not be deciding how it interacts with the JavaScript. Instead, separate your JavaScript code from the HTML, and let it decide how it interacts with the page.
What is JavaScript?
Javascript is a programming language that operates important features on many websites.
What is the difference between JavaScript and DHTML?
The line between DHTML and Javascript can sometimes get blurry, but a rule of thumb I use is simple: DHTML is about the web page, period. The items on a web page, the events that a web page might want to act on (like moving your mouse over text), and the resulting changes to the web page that you might want to have happen resulting from those events (like changing the color of text). Javascript, however, is really web, and HTML, independent. It adds programming things like loops, and variables and functions. You could write a small program to calculate Pi in Javascript, and then perhaps use DHTML to do something interesting on the web page with it.
What is the language used to make a web page look and act?
So in a nutshell, HTML, DHTML and Javascript are all programming languages that are used to make your web pages look and act the way we do.
What is HTML in web design?
HTML, or HyperText Markup Language, is the basic language of the web. It describes how a page should look. The browser follows the HTML instructions as it reads and presents the page. Once the page is complete, the browser’s done, and the HTML “program” is over.
What is the language of a web page?
And, naturally, there are several different types of programming languages that can be used. In (over) simplified terms: HTML, or HyperText Markup Language , is the basic language of the web. It describes how a page should look.
Is JavaScript web or HTML?
Javascript, however, is really web, and HTML, independent. It adds programming things like loops, and variables and functions. You could write a small program to calculate Pi in Javascript, and then perhaps use DHTML to do something interesting on the web page with it. ActiveX is the next level up.
Is JavaScript a programming language?
Javascript is not specifically HTML related, but interacts with HTML and DHTML when used in a browser. It’s a more traditional programming language, in that you can write a series of instructions to compute what kinds of actions should be taken based on various conditions, repeat things a variable number of times, and just generally take more complex and sophisticated actions. Small applications and games have been written entirely in Javascript.
JavaScript Tutorial
We'll cover the fundamentals of JavaScript and how to use it in projects and at work in this JavaScript lesson.
Create Stunning websites and web apps
Trying to build out all user interfaces and components for your website or web app from scratch can become a very tedious task. A huge reason why we created Contrast Bootstrap to help reduce the amount of time we spend doing that, so we can focus on building some other aspects of the project.
What is JavaScript used for?
JavaScript is best known for web page development but it is also used in a variety of non-browser environments. You can learn JavaScript from the ground up by following this JavaScript Tutorial and JavaScript Examples.
What is scope in JavaScript?
Scope means variable access. What variable do I have access to when a code is running? In javascript by default, you’re always in root scope i.e. the window scope. The scope is simply a box with a boundary for variables, functions, and objects. These boundaries put restrictions on variables and determine whether you have access to the variable or not. It limits the visibility or availability of a variable to the other parts of the code. You must have a clear understanding of this concept because it helps you to separates logic in your code and also improves the readability. A scope can be defined in two ways…
Why does JavaScript get unexpected results?
A lot of developers get unexpected results when they are not clear with the concept of Hoisting in javascript. In javascript, you can call a function before it is defined and you won’t get an error ‘Uncaught ReferenceError’. The reason behind this is hoisting where the javascript interpreter always moves the variables and function declaration to the top of the current scope (function scope or global scope) before the code execution. Let’s understand this with example.
Why use IIFE in JavaScript?
So the primary reason to use IIFE is to immediately executes the code and obtain data privacy.
What is the best programming language for a beginner?
According to Stack Overflow Developer Survey 2019 Javascript is #1 programming language. The language is widely used by 95% of all the websites which you can check here. Whether it’s a small startup or a big company, most of them are working on some kind of website or an app that requires a good knowledge of this language. A lot of frameworks and libraries are there for javascript. These frameworks and libraries can be easily learned if your javascript fundamentals are clear. A lot of concepts are confusing and overwhelming for developers but a good knowledge of these concepts will help you in the along run. Frameworks and libraries come and go but the fundamentals always remain the same. It’s easy to build any kind of application and learn any framework and libraries if the fundamentals are clear. Also, it will help you in interviews as well. Let’s discuss some of the basic concepts of javascript which are important to learn for any javascript developer.
How to notify JS that we are working with promises?
To notify JS that we are working with promises we need to wrap ‘await’ inside an ‘async’ function. In the above example, we (a)wait for two things: response and posts. Before we can convert the response to JSON format, we need to make sure we have the response fetched, otherwise we can end up converting a response that is not there yet, which will most likely prompt an error.
Is JavaScript everywhere?
JavaScript is Everywhere. Millions of webpages are built on JavaScript and it’s not going anywhere at least for now. On one side HTML and CSS give styling to the web pages but on the other side, it’s the magic of JavaScript that makes your web page alive. Today this language is not just limited to your web browser.
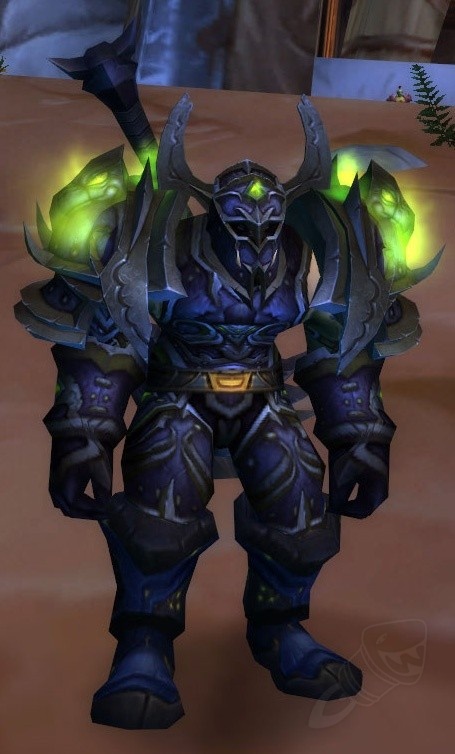