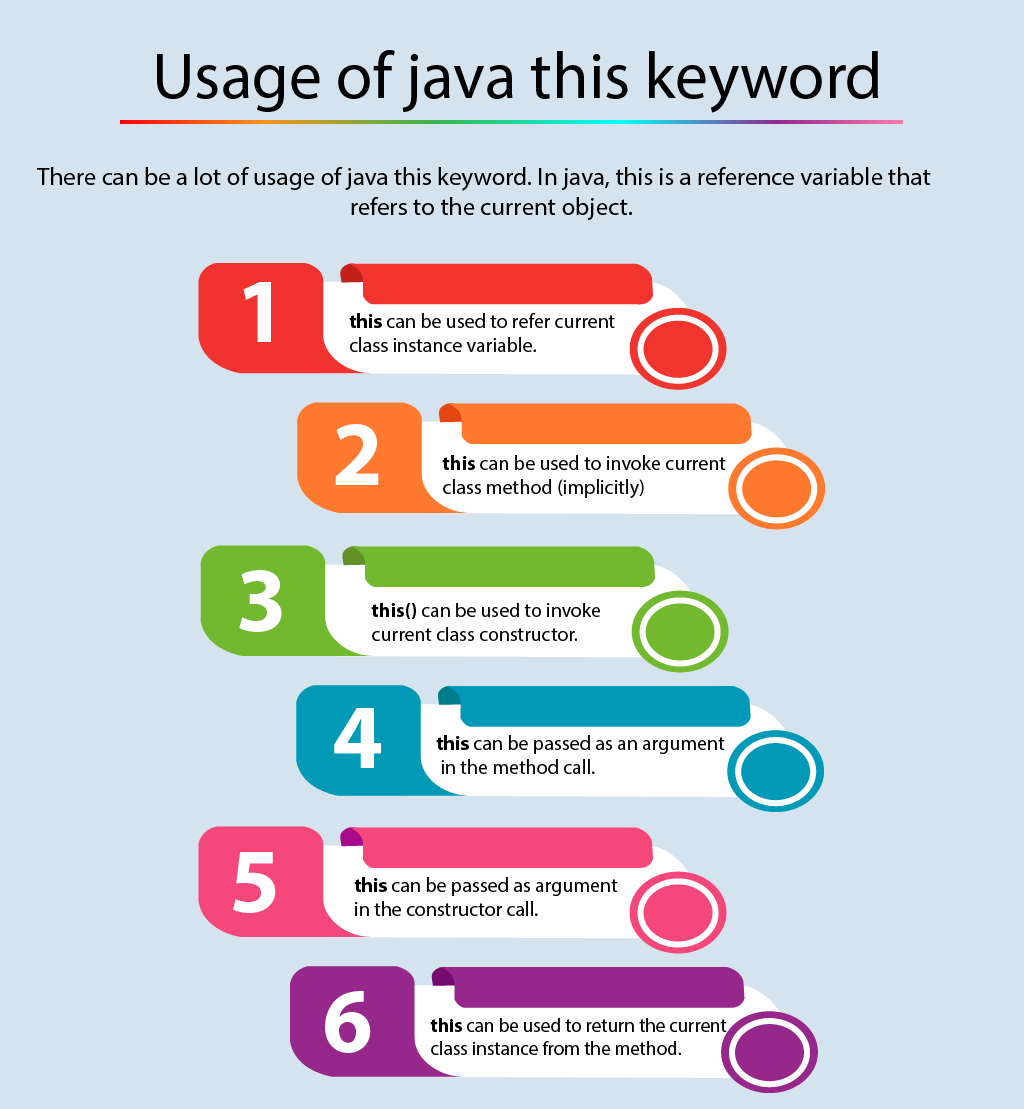
final (Java) From Wikipedia, the free encyclopedia In the Java programming language
Java
Java is a general-purpose programming language that is class-based, object-oriented, and designed to have as few implementation dependencies as possible. It is intended to let application developers write once, run anywhere, meaning that compiled Java code can run on all platforms tha…
Why to use final in Java?
Using final :
- clearly communicates your intent
- allows the compiler and virtual machine to perform minor optimizations
- clearly flags items which are simpler in behaviour - final says, " If you are looking for complexity, you won't find it here. "
What is the role of final in Java?
final is a non-access modifier for Java elements. The final modifier is used for finalizing the implementations of classes, methods, and variables. A final variable can be explicitly initialized only once. A reference variable declared final can never be reassigned to refer to a different object. However, the data within the object can be changed.
How does the "final" keyword in Java work?
Subscribe to our Newsletter, and get personalized recommendations.
- To create constants.
- To prevent inheritance.
- To prevent methods from being overridden.
What is the use of final key words in Java?
final keyword in java
- Use of final keyword in java: A variable declared with final keyword is known as final variable. ...
- Example: FinalExample1.java ...
- Output: Exception in thread "main" java. ...
- Example: FinalExample2.java ...
- Output: Exception in thread "main" java. ...
- Example: FinalExample3.java ...
- Output: Exception in thread "main" java. ...
- Example: FinalExample4.java ...
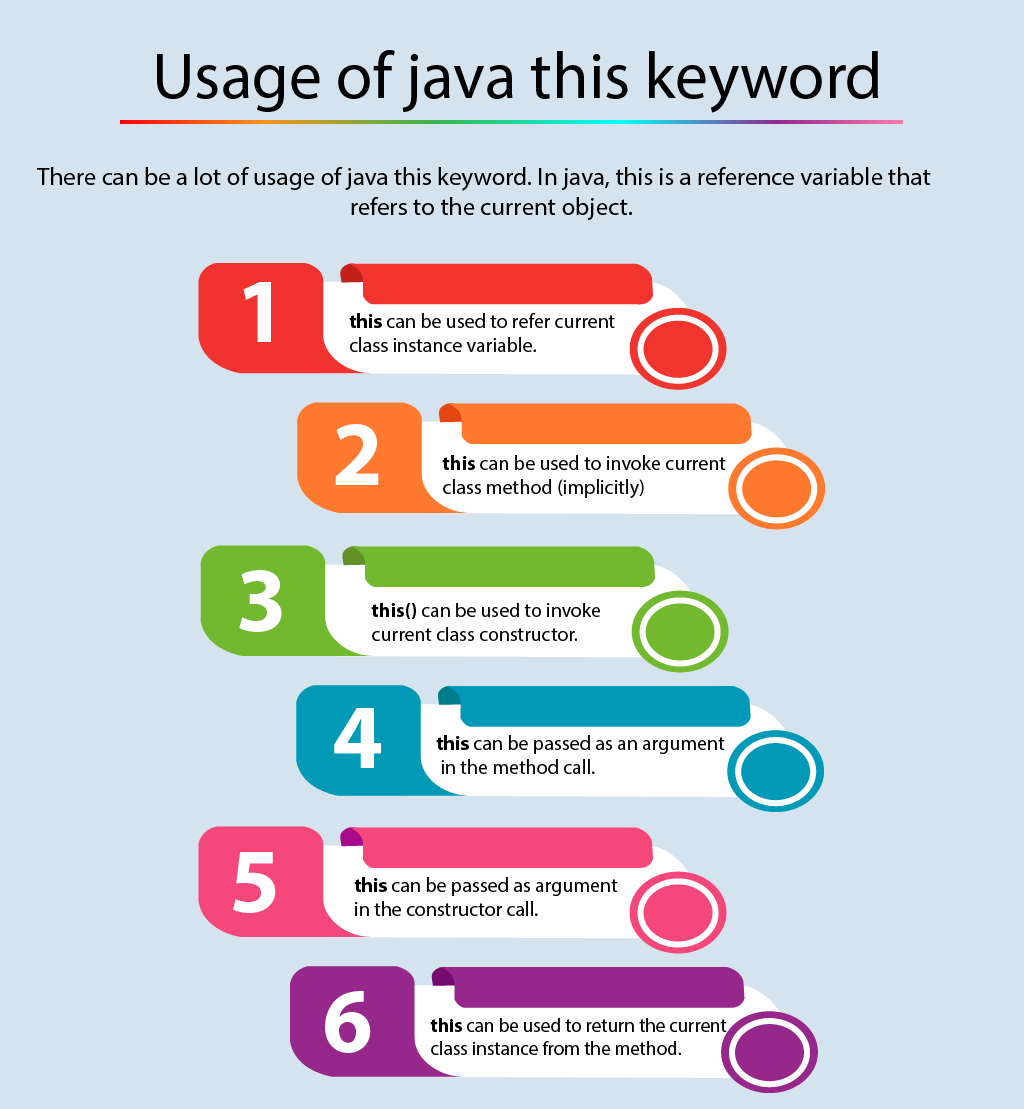
What does a final class in Java mean?
A final class is a class that can't be extended. Also methods could be declared as final to indicate that cannot be overridden by subclasses. Preventing the class from being subclassed could be particularly useful if you write APIs or libraries and want to avoid being extended to alter base behaviour.
What does a final object mean Java?
final means that you can't change the object's reference to point to another reference or another object, but you can still mutate its state (using setter methods e.g). Whereas immutable means that the object's actual value can't be changed, but you can change its reference to another one.
What is final value in Java?
In Java, the final keyword can be used while declaring an entity. Using the final keyword means that the value can't be modified in the future. This entity can be - but is not limited to - a variable, a class or a method.
What is a final argument in Java?
The final keyword when used for parameters/variables in Java marks the reference as final. In case of passing an object to another method, the system creates a copy of the reference variable and passes it to the method. By marking the new references final, you protect them from reassignment.
What is a final variable?
You can declare a variable in any scope to be final. . The value of a final variable cannot change after it has been initialized. Such variables are similar to constants in other programming languages.
Why is String final in Java?
The string is immutable means that we cannot change the object itself, but we can change the reference to the object. The string is made final to not allow others to extend it and destroy its immutability.
What is static and final in Java?
The static keyword means the value is the same for every instance of the class. The final keyword means once the variable is assigned a value it can never be changed. The combination of static final in Java is how to create a constant value.
What is final and finally keyword in Java?
Final is a keyword and is used as access modifier in Java. Finally is a block in Java used for Exception Handling. Finalize is a method in Java used for Garbage Collection. Application. Final in Java is used with variables, methods, and classes to set access permissions.
Why should we use final?
First of all, the final keyword is used to make a variable constant. Constant means it does not change.
How do you call a final class in Java?
We can declare Java methods as Final Method by adding the Final keyword before the method name. The Method with Final Keyword cannot be overridden in the subclasses. The purpose of the Final Method is to declare methods of how's definition can not be changed by a child or subclass that extends it.
How do you declare a final variable?
There are three ways to initialize a final variable:You can initialize a final variable when it is declared. This approach is the most common. ... A blank final variable can be initialized inside an instance-initializer block or inside the constructor. ... A blank final static variable can be initialized inside a static block.
How do you declare a final string in Java?
1) Java final variableclass Bike9{final int speedlimit=90;//final variable.void run(){speedlimit=400;}public static void main(String args[]){Bike9 obj=new Bike9();obj.run();More items...
What is the final keyword in Java?
The final keyword in java is used to restrict the user. The java final keyword can be used in many context. Final can be: variable. method. class. The final keyword can be applied with the variables, a final variable that have no value it is called blank final variable or uninitialized final variable. It can be initialized in the constructor only.
What is a final variable that is not initialized?
A final variable that is not initialized at the time of declaration is known as blank final variable. If you want to create a variable that is initialized at the time of creating object and once initialized may not be changed, it is useful. For example PAN CARD number of an employee.
What is static blank final variable?
static blank final variable. A static final variable that is not initialized at the time of declaration is known as static blank final variable. It can be initialized only in static block.
Can a final variable speedlimit be changed?
There is a final variable speedlimit, we are going to change the value of this variable, but It can't be changed because final variable once assigned a value can never be changed.
What is final in Java?
Jump to navigation Jump to search. In the Java programming language, the final keyword is used in several contexts to define an entity that can only be assigned once. Once a final variable has been assigned, it always contains the same value. If a final variable holds a reference ...
What does declaring a variable final mean?
The reason for this is that declaring a variable final only means that this variable will point to the same object at any time. The object that the variable points to is not influenced by that final variable though. In the above example, the origin's x and y coordinates can be freely modified.
What is the analog of final variables in C++?
C/C++ analog of final variables. Further information: const (computer programming) In C and C++, the analogous construct is the const keyword. This differs substantially from final in Java, most basically in being a type qualifier: const is part of the type, not only part of the identifier (variable).
What is a blank final instance variable?
A blank final instance variable of a class must be definitely assigned in every constructor of the class in which it is declared; similarly, a blank final static variable must be definitely assigned in a static initializer of the class in which it is declared; otherwise, a compile-time error occurs in both cases.
How many times can a final variable be initialized?
A final variable can only be initialized once, either via an initializer or an assignment statement. It does not need to be initialized at the point of declaration: this is called a "blank final" variable. A blank final instance variable of a class must be definitely assigned in every constructor of the class in which it is declared; similarly, ...
What is public final position?
public final Position pos; where pos is an object with three properties pos .x, pos.y and pos.z. Then pos cannot be assigned to, but the three properties can, unless they are final themselves. Like full immutability, the use of final variables has great advantages, especially in optimization.
What is the property of a final variable?
If a final variable holds a reference to an object, then the state of the object may be changed by operations on the object, but the variable will always refer to the same object (this property of final is called non-transitivity ). This applies also to arrays, because arrays are objects; if a final variable holds a reference to an array, ...
What is final method in Java?
What is a final method in Java? The final modifier for finalizing the implementations of classes, methods, and variables. We can declare a method as final, once you declare a method final it cannot be overridden. So, you cannot modify a final method from a sub class.
Can you modify a final method from a subclass?
So, you cannot modify a final method from a sub class. The main intention of making a method final would be that the content of the method should not be changed by any outsider.
What is final in Java?
final is a reserved keyword in Java to restrict the user and it can be applied to member variables, methods , class and local variables. Final variables are often declared with the static keyword in Java and are treated as constants. For example: public static final String hello = "Hello";
What does "final class" mean in Java?
A final class means that no other class can extend that final class. When Java Run Time ( JRE) knows an object reference is in type of a final class (say F), it knows that the value of that reference can only be in type of F. Ex:
What is a foo in a test class?
foo is an instance variable. When we create Test class object then the instance variable foo, will be copied inside the object of Test class. If we assign foo inside the constructor, then the compiler knows that the constructor will be invoked only once, so there is no problem assigning it inside the constructor.
What is the final keyword in Java?
The final keyword in java is used to restrict the user. The java final keyword can be used in many context. Final can be: variable. method. class. The final keyword can be applied with the variables, a final variable that has no value, is called blank final variable or uninitialized final variable.
Can you see a foo in multiple objects?
But foo can be seen by multiple objects and if every object which is created by using the new keyword which will ultimately invoke the Test constructor which changes the value at the time of multiple object creation (Remember static foo is not copied in every object, but is shared between multiple objects.)
What does it mean when someone mentions a final object?
1) When someone mentions a final object, it means that the reference cannot be changed, but its state (instance variables) can be changed. 2) An immutable object is one whose state can not be changed, but its reference can be changed. Ex: String x = new String ("abc"); x = "BCG"; ref variable x can be changed to point a different string, ...
When to use final keyword?
There is another user case where final keyword can be used i.e. in a method argument: Can be used for variable which should not be changed. When you make it static final it should be initialized in a static initialization block. The final keyword indicates that a variable may only be initialized once.
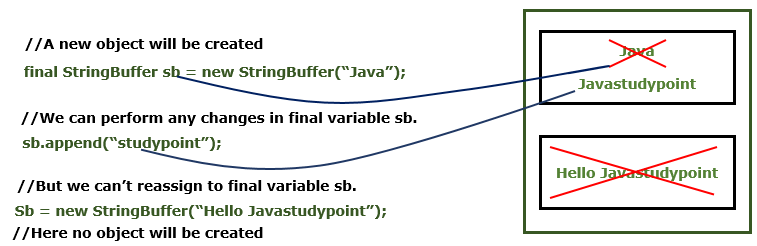
Overview
In the Java programming language, the final keyword is used in several contexts to define an entity that can only be assigned once.
Once a final variable has been assigned, it always contains the same value. If a final variable holds a reference to an object, then the state of the object may be changed by operations on the object, but the variable will always refer to the same object (this property of final is called non-tr…
Final classes
A final class cannot be subclassed. As doing this can confer security and efficiency benefits, many of the Java standard library classes are final, such as java.lang.System and java.lang.String.
Example:
Final methods
A final method cannot be overridden or hidden by subclasses. This is used to prevent unexpected behavior from a subclass altering a method that may be crucial to the function or consistency of the class.
Example:
A common misconception is that declaring a method as final improves efficiency by allowing th…
Final variables
A final variable can only be initialized once, either via an initializer or an assignment statement. It does not need to be initialized at the point of declaration: this is called a "blank final" variable. A blank final instance variable of a class must be definitely assigned in every constructor of the class in which it is declared; similarly, a blank final static variable must be definitely assigned in a static initializer of the class in which it is declared; otherwise, a compile-time error occurs in both case…
C/C++ analog of final variables
In C and C++, the analogous construct is the const keyword. This differs substantially from final in Java, most basically in being a type qualifier: const is part of the type, not only part of the identifier (variable). This also means that the constancy of a value can be changed by casting (explicit type conversion), in this case known as "const casting". Nonetheless, casting away constness and then modifying the object results in undefined behavior if the object was originally declared const. Jav…
C# analogs for final keyword
C# can be considered as similar to Java, in terms of its language features and basic syntax: Java has JVM, C# has .Net Framework; Java has bytecode, C# has MSIL; Java has no pointers (real memory) support, C# is the same.
Regarding the final keyword, C# has two related keywords:
1. The equivalent keyword for methods and classes is sealed