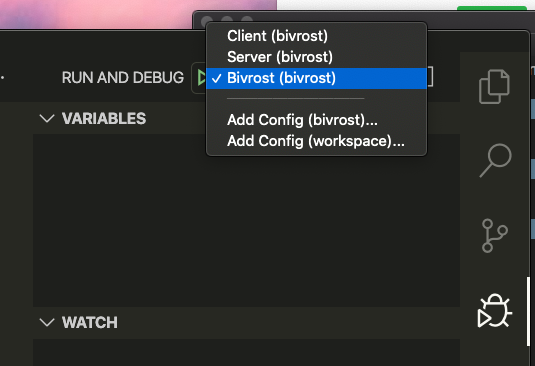
Does npm run build use webpack?
@Little_Programmer the correct order is npm run build (command in package. json) --> which runs webpack script (webpack tool) --> and then webpack reads the configurations(if any) and creates bundles.
What is webpack and do I need it?
This is why webpack exists. It's a tool that lets you bundle your JavaScript applications (supporting both ESM and CommonJS), and it can be extended to support many different assets such as images, fonts and stylesheets.
Why do we need webpack in Nodejs?
Webpack provides a great developer experience as it not only bundles JavaScript applications (supporting both EcmaScript Modules and CommonJS), but when paired with plugins and loaders it can be used for taking care of dependencies and assets, such as images, fonts, SASS, CSS, etx.
What is the use of webpack command?
webpack-command allows users to leverage any language that provides a require hook. To leverage this feature, define your configs as such for the following languages/compilers: Babel ES6 Modules: webpack.
Do I need Webpack with NPM?
It is highly unlikely that you will not need it. Webpack is a module bundler. It is mostly used to manage JavaScript codebases, most often for usage in the browser, and requires Node.
Can you use NPM without Webpack?
You don't need webpack nor babel to make an mpm module. Just put in any folder the files you want to distribute, specifying the main entry point and export elements on that file.
What is webpack and how it works?
When webpack processes your application, it internally builds a dependency graph from one or more entry points and then combines every module your project needs into one or more bundles, which are static assets to serve your content from.
Does webpack improve performance?
Webpack provides support for caching the build files generated to improve the build speed. We can achieve this by providing a cache option set to true in the webpack. config. js file.
What problem does webpack solve?
What Problem Does Webpack Solve? Webpack exists to give us a feature of JavaScript that exists in most other languages by default - modularization. As programmers, we want to put code in different files for the purposes of organization. Said another way, we don't want all our JavaScript in one file.
Is Webpack a compiler?
Webpack isn't a compiler it's a bundler, but like a compiler it parses your source files, Webpack bundles your code and you can set it up in a way that it also transpiles (transforms) newer JS syntax into older but more widely supported syntax and it also allows you to split your code into different modules using ...
How do I know if I am using Webpack?
If your project is using Webpack then there will be a webpack. config. js file in the root of the project.
How do I know if my Webpack is working?
Webpack has a web server called webpack-dev-server. If you go to http://localhost:8080/webpack-dev-server/, you should see your application running there, along with any log statements in your app. From the webpack docs: “The dev server uses Webpack's watch mode.
Do I need Webpack in server?
If you're trying to server-side render a frontend component that isn't in JavaScript, you'll need Webpack and plugins like vue-loader to compile the specialized syntax into JavaScript.
Is it necessary to use Webpack in React JS?
Webpack would need to be configured to support files beyond JavaScript and JSON, since it only supports those file types by default. It cannot just simply process CSS, or other file types, without the proper configurations in the config file. To address this issue, loaders would be used.
Is Webpack necessary with ES6?
For most browsers, yes, you can accomplish getting all needed code to the browser with just ES6 modules, without Webpack.
Is Webpack still popular?
Currently, the most common bundler is Webpack. Its popularity works to its advantage, as the community support around the tool is invaluable.
What is webpack used for?
webpack is used to compile JavaScript modules. Once installed, you can interact with webpack either from its CLI or API. If you're still new to webpack, please read through the core concepts and this comparison to learn why you might use it over the other tools that are out in the community.
How to pass custom parameters to webpack?
Custom parameters can be passed to webpack by adding two dashes between the npm run build command and your parameters , e.g. npm run build -- --color.
What does webpack.config.js do?
If a webpack.config.js is present, the webpack command picks it up by default. We use the --config option here only to show that you can pass a configuration of any name. This will be useful for more complex configurations that need to be split into multiple files.
What is distribution code?
First we'll tweak our directory structure slightly, separating the "source" code ( ./src) from our "distribution" code ( ./dist ). The "source" code is the code that we'll write and edit. The "distribution" code is the minimized and optimized output of our build process that will eventually be loaded in the browser. Tweak the directory structure as follows:
Does webpack transpile code?
Behind the scenes, webpack actually " transpiles " the code so that older browsers can also run it. If you inspect dist/main.js, you might be able to see how webpack does this, it's quite ingenious! Besides import and export, webpack supports various other module syntaxes as well, see Module API for more information.
Does ES2015 support import and export?
The import and export statements have been standardized in ES2015. They are supported in most of the browsers at this moment, however there are some browsers that don't recognize the new syntax. But don't worry, webpack does support them out of the box.
What happens if a dependency is missing?
If a dependency is missing, or included in the wrong order, the application will not function properly.
What is the difference between development and production?
The goals of development and production builds differ greatly. In development, we want strong source mapping and a localhost server with live reloading or hot module replacement. In production, our goals shift to a focus on minified bundles, lighter weight source maps, and optimized assets to improve load time. With this logical separation at hand, we typically recommend writing separate webpack configurations for each environment.
What is node_env?
Technically, NODE_ENV is a system environment variable that Node.js exposes into running scripts. It is used by convention to determine dev-vs-prod behavior by server tools, build scripts, and client-side libraries. Contrary to expectations, process.env.NODE_ENV is not set to 'production' within the build script webpack.config.js, see #2537. Thus, conditionals like process.env.NODE_ENV === 'production' ? ' [name]. [contenthash].bundle.js' : ' [name].bundle.js' within webpack configurations do not work as expected.
Why key off process.env.NODE_ENV?
Many libraries will key off the process.env.NODE_ENV variable to determine what should be included in the library. For example, when process.env.NODE_ENV is not set to 'production' some libraries may add additional logging and testing to make debugging easier. However, with process.env.NODE_ENV set to 'production' they might drop or add significant portions of code to optimize how things run for your actual users. Since webpack v4, specifying mode automatically configures DefinePlugin for you:
Why avoid inline eval?
Avoid inline-*** and eval-*** use in production as they can increase bundle size and reduce the overall performance.
Does Webpack v4+ minify?
Webpack v4+ will minify your code by default in production mode.
Can you use source maps in production?
We encourage you to have source maps enabled in production, as they are useful for debugging as well as running benchmark tests. That said, you should choose one with a fairly quick build speed that's recommended for production use (see devtool ). For this guide, we'll use the source-map option in the production as opposed to the inline-source-map we used in the development:
Does react drop bundle size?
If you're using a library like react, you should actually see a significant drop in bundle size after adding DefinePlugin. Also, note that any of our local /src code can key off of this as well, so the following check would be valid:
What is ECMAScript in JavaScript?
As any language, Javascript also has versions named ECMAScript (short for ES). Currently, most browsers support ES5. ES5 used to be good even though it was painful to code in it. Remember, this not reading from inside callback functions? The new version of Javascript, ES6, also known as ES2015 (specs of the language were finalized in June 2015) makes Javascript great again. If you want to learn about ES6, check out the links at the end of this article. All the great features of ES6 come with one big problem — majority of browsers do not fully support them. That’s when Babel comes to play. Babel is a JS transpiler that converts new JS code into old ones. It is a very flexible tool in terms of transpiling. One can easily add presets such as es2015, es2016, es2017, or env; so that Babel compiles them to ES5.
Why is it important to separate devdependencies from dependencies?
Note that separating devDependencies from dependencies is only useful for the developer because unlike NodeJS projects for frontend applications we need all the dependencies to successfully build the application. Separat ing tools into devDepend encies make it easier to distinguish between build tools (e.g Babel) and application’s real dependencies (e.g React).
What is NPM in CLI?
NPM stands for Node Package Manager. It is what its name describes. It is a package manager for Node based environments. It keeps track of all the packages and their versions and allows the developer to easily update or remove these dependencies. All of these external dependencies are being stored inside a file called called package.json. The initial file can be created easily using CLI npm init (assuming NodeJS is installed in the system). When you install a package using NPM, the packages get downloaded from a dedicated registry. There are lot of features of NPM like publishing. If you like to learn more about NPM, check out the links at the bottom.
What port does Webpack run on?
Webpack Dev Server runs in port 8080 by default. Open your browser and type in http://localhost:8080 and you are all set.
What is a webpack?
Webpack is a modular build tool that has two sets of functionality — Loaders and Plugins. Loaders transform the source code of a module. For example, style-loader adds CSS to DOM using style tags. sass-loader compiles SASS files to CSS. babel-loader transpiles JS code given the presets. Plugins are the core of Webpack. They can do things that loaders can’t. For example, there is a plugin called UglifyJS that minifies and uglifies the output of webpack.
What is a Babel?
Babel is a JS transpiler that converts new JS code into old ones. It is a very flexible tool in terms of transpiling. One can easily add presets such as es2015, es2016, es2017, or env; so that Babel compiles them to ES5. Here is an example — a code written in ES6: class Test {. calculatePowers () {.
What language has a package manager?
Every language that we use has some form of package manager, either official or a 3rd party one. PHP has Composer, Python has PIP/Pipenv, Java has Gradle etc.
How to pass custom parameters to webpack?
Custom parameters can be passed to webpack by adding two dashes between the npm run buildcommand and your parameters , e.g. npm run build -- --colors.
When calling webpack via its path on Windows, must you use backslashes instead?
Note that when calling webpack via its path on windows, you must use backslashes instead, e.g. node_modules.binwebpack -- config webpack.config.js.
When installing a package that will be bundled into your production bundle, should you use npm install?
When installing a package that will be bundled into your production bundle, you should use npm install --save. If you're installing a package for development purposes (e.g. a linter, testing libraries, etc.) then you should use npm install --save-dev. More information can be found in the npm documentation.
What happens if a script is not included in the library?
If a dependency is missing, or included in the wrong order, the application will not function properly. If a dependency is included but not used, the browser will be forced to download unnecessary code.
Does webpack require configuration?
As of version 4, webpack doesn’t require any configuration, but most projects will need a more complex setup, which is why webpack supports a configuration file. This is much more efficient than having to manually type in a lot of commands in the terminal, so let’s create one to replace the CLI line options used above:
What is distribution code?
The "source" code is the code that we'll write and edit. The "distribution" code is the minimized and optimized output of our build process that will eventually be loaded in the browser:
Why do we need to adjust package.json?
We also need to adjust our package.json file in order to make sure we mark our package as private, as well as removing the main entry . This is to prevent an accidental publish of your code.
What problem is webpack solving?
Historically when building a JavaScript application, your JavaScript code would be separated by files (these files may or may not have been actual modules). Then in your index.html file, you'd have to include <script> tags to every JavaScript file you had.
How does webpack work?
1) webpack grabs the entry point located at ./app/index.js . 2) It examines all of our import and require statements and creates a dependency graph. 3) webpack starts creating a bundle, whenever it comes across a path we have a loader for, it transforms the code according to that loader then adds it to the bundle. 4) It takes the final bundle and outputs it at dist/index_bundle.js.
What is webpack module?
At its core, webpack is a module bundler. It examines all of the modules in your application, creates a dependency graph, then intelligently puts all of them together into one or more bundle (s) that your index.html file can reference.
What is a plugin in a bundle?
Plugins. We’ve seen how you can use loaders to work on individual files before or while the bundle is being generated . Unlike loaders, plugins allow you to execute certain tasks after the bundle has been created. Because of this, these tasks can be on the bundle itself, or just to your codebase.
What is the point of webpack?
Remember, the whole point of webpack is to “examine all of your modules, (optionally) transform them, then intelligently put all of them together into one or more bundle (s)” If you think about that process, in order to do that, webpack needs to know three things. The entry point of your application.
What happens if you load a script that depended on React before loading the React script?
If you loaded a script that depended on React before loading the React script, things would break. Because webpack (intelligently) creates a bundle for you, both of those problems go away. You don’t have to worry about forgetting a <script> and you don’t have to worry about the order.
How many packages are needed to use webpack?
Assuming you’ve initialized a new project with npm, there are two packages you need to install to use webpack, webpack and webpack-cli.
