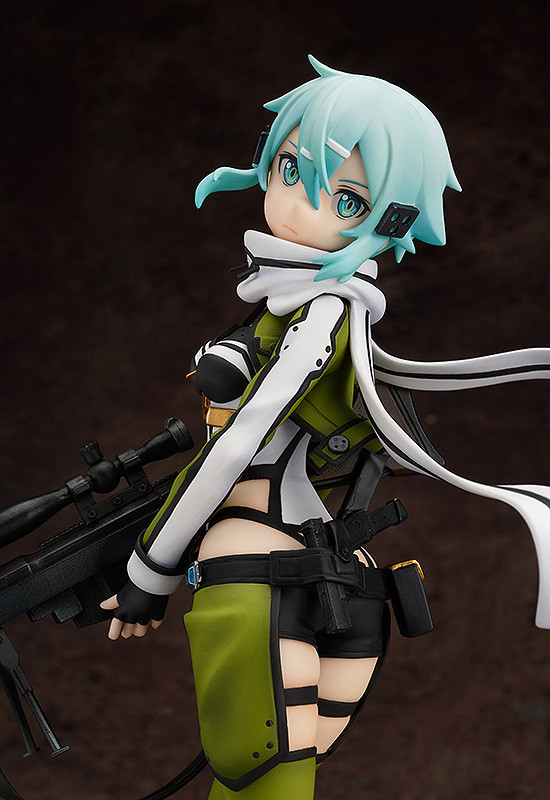
What is a test spy?
What does true mean in spy?
What does "return true" mean in spy?
What is a spy object?
What is sinon.spy?
What is spy.args?
What is spy.returnValues?
See 2 more
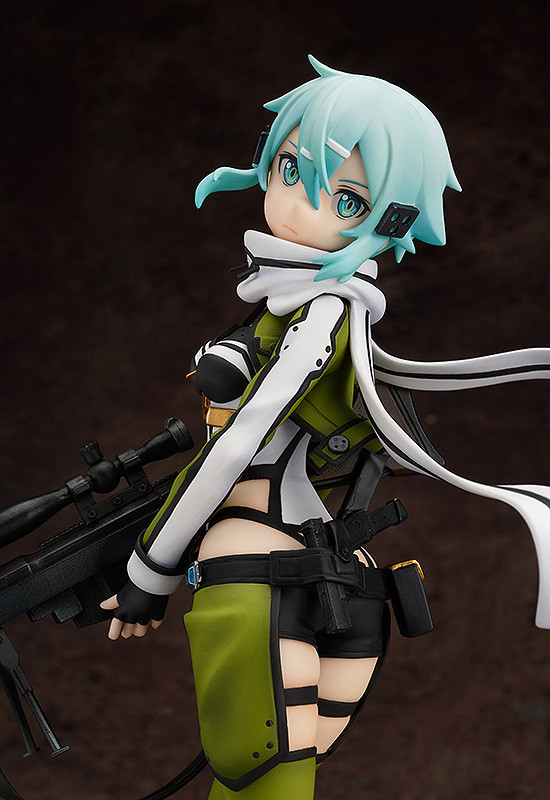
What is Sinon used for?
Sinon JS is a popular JavaScript library that lets you replace complicated parts of your code that are hard to test for “placeholders,” so you can keep your unit tests fast and deterministic, as they should be.
How does Sinon stub work?
To stub a dependency (imported module) of a module under test you have to import it explicitly in your test and stub the desired method. For the stubbing to work, the stubbed method cannot be destructured, neither in the module under test nor in the test.
What is Sinon test?
Sinon. JS is also an open-source framework. It provides spies, stubs, and mocks for use in JavaScript unit tests. It works with any unit testing framework and has no external dependencies. This article is only going to brush the surface of Sinon.
What does Sinon stub return?
The sinon. stub() substitutes the real function and returns a stub object that you can configure using methods like callsFake() . Stubs also have a callCount property that tells you how many times the stub was called.
What is stubbing in testing?
A stub is a small piece of code that takes the place of another component during testing. The benefit of using a stub is that it returns consistent results, making the test easier to write. And you can run tests even if the other components are not working yet.
What is a stub vs mock?
Stub: a dummy piece of code that lets the test run, but you don't care what happens to it. Substitutes for real working code. Mock: a dummy piece of code that you verify is called correctly as part of the test. Substitutes for real working code.
What is Spy in JavaScript?
A test spy is a function that records arguments, return value, the value of this and exception thrown (if any) for all its calls. There are two types of spies: Some are anonymous functions, while others wrap methods that already exist in the system under test.
What is Sinon sandbox?
JS. Sandboxes removes the need to keep track of every fake created, which greatly simplifies cleanup. var sandbox = require("sinon"). createSandbox(); var myAPI = { hello: function () {} }; describe("myAPI.hello method", function () { beforeEach(function () { // stub out the `hello` method sandbox.
What is Sinon restore?
The highlighted sentence is the key. The restore functions in sinon only work on spies and stubs that replace an existing method, and our spy was created as an anonymous (standalone) spy. Therefore, restore does not reset the call count, which will continue increasing as the spy function is called in subsequent tests.
What is Sinon chai?
Sinon–Chai provides a set of custom assertions for using the Sinon. JS spy, stub, and mocking framework with the Chai assertion library. You get all the benefits of Chai with all the powerful tools of Sinon.
How do you mock a promise in Sinon?
promise();Creating a promise with a fake executor. var executor = sinon. fake(); var promise = sinon. ... Creating a promise with custom executor. var promise = sinon. promise(function (resolve, reject) { // ... ... promise. status. ... promise. resolvedValue. ... promise. rejectedValue. ... promise. resolve(value) ... promise. reject(value)
How do you clear a Sinon stub?
reset() and restore() One important function to remember is sinon. reset() , which resets both the behavior and history of all stubs. If you just want to reset a specific stub you can use stub. reset() .
How do I stub an object in Sinon?
If you need to check that a certain value is set before a function is called, you can use the third parameter of stub to insert an assertion into the stub: var object = { }; var expectedValue = 'something'; var func = sinon. stub(example, 'func', function() { assert. equal(object.
What does sandbox stub do?
sandbox. Causes all stubs and mocks created from the sandbox to return promises using a specific Promise library instead of the global one when using stub. rejects or stub. resolves . Returns the stub to allow chaining.
What does it mean to stub a request?
What is a Stub? In testing land, a stub replaces real behavior with a fixed version. In the case of HTTP requests, instead of making the actual call, a stub fakes the call and provides a canned response that can be used to test against.
How do I use stubs in node JS?
Stubs can be wrapped into existing functions....When to use stubs?Prevent a specific method from being called directly.Controlling method behavior down a specific path from a test to force the code. For example: Error handling.Replacing the problematic pieces of code.Testing asynchronous code easy.
Using Spies for Testing in JavaScript with Sinon.js - Stack Abuse
When you run the test, you should get the following output: $ mocha index.test.js test getPhotosByAlbumId should getPhotosByAlbumId (570ms) 1 passing (587ms) Done in 2.53s. Please note that this test made an actual network request to the API. The spy wraps around the function, it does not replace its functionality!. Also, Sinon.js test stubs are already spies!
Best Practices for Spies, Stubs and Mocks in Sinon.js
Learn about differences between spies, stubs and mocks, when and how to use them, and get a set of best practices to help you avoid common pitfalls.
sinon spy by example · In My Opinion
What is Sinon Spy ? According to the xUnit patterns definition, test spy is designed to act as an observation point by recording the method calls made to it by the SUT (system under test) as it is exercised.
How to spy on an imported function using Sinon?
Let's say we want to test that a specific function is called by another function using Sinon. fancyModule.js export const fancyFunc = () => { console.log('fancyFunc') } export default const
Verifying function call and inspecting arguments using sinon spies
In your case, you are trying to see if bar was called, so you want to spy bar rather than foo.. As described in the doc: . function bar(x,y) { console.debug(x, y); } function foo(z) { bar(z, z+1); } // Spy on the function "bar" of the global object. var spy = sinon.spy(window, "bar"); // Now, the "bar" function has been replaced by a "Spy" object // (so this is not necessarily what you want to ...
Sinon Tutorial: JavaScript Testing with Mocks, Spies & Stubs
Conclusion. Testing real-life code can sometimes seem way too complex and it’s easy to give up altogether. But with help from Sinon, testing virtually any kind of code becomes a breeze.
How Sinon Spy works?
You need to make test spy first to allow it observe the execution of your desire method and then assert your expectation with actual calls.
What does "calledwithExactly" mean?
calledWithExactly assert that the method called with all the args with 1 by 1 match . in the above example myMethod is called with arg1 and arg2 in the first time and arg1 and undefined on the second time.
What does "call with match" mean?
called with match check if the arguments of the spied method contain our expected types. alwaysCalledWithMatch assert if all the calls contain our expected match. Therefore it will be fail for below assertion as it did not called with two args on the second call.
What is the first argument in a spy?
The first argument will is nth call of the spy and the second one is the nth index arguments (both started from zero).
How to check if a method calls before or after another method?
In order to check that if a method call before or after another method you can use, calledBefore and calledAfter.
How many times does myMethod call?
Similar to above method it will assure that myMethod will be only call three times, during the code execution.
Does spied return expected string?
it will assert that the spied method return expected string or object. alwaysReturned assert that the method returns expected value. you can access to the values of retuned value by returnValues [index of nth call].
What Makes Sinon so Important and Useful?
Put simply, Sinon allows you to replace the difficult parts of your tests with something that makes testing simple.
What is Sinon mock?
Mocks, which make replacing whole objects easier by combining both spies and stubs. In addition, Sinon also provides some other helpers, although these are outside the scope of this article: Fake timers, which can be used to travel forwards in time, for example triggering a setTimeout.
How to install Sinon?
You can either install Sinon via npm with npm install sinon, use a CDN, or download it from Sinon’s website
Why are stubs used in test doubles?
Stubs. Stubs are the go-to test-double because of their flexibility and convenience. They have all the functionality of spies, but instead of just spying on what a function does, a stub completely replaces it. In other words, when using a spy, the original function still runs, but when using a stub, it doesn’t.
How do mocks work?
You define your expected results up front by telling the mock object what needs to happen, and then calling the verification function at the end of the test.
Why do we need a stub?
They are primarily useful if you need to stub more than one function from a single object. If you only need to replace a single function, a stub is easier to use.
Why is Ajax so hard to test?
All of these are hard to test because you can’t control them in code. If you’re using Ajax, you need a server to respond to the request, so as to make your tests pass.
Why Use Spies?
Spies excel in giving insight into the behavior of the function we are testing. While validating the inputs and outputs of a test is crucial, examining how the function behaves can be crucial in many scenarios:
What is the getAlbumByID method?
To recap, the getAlbumById () method calls a JSON API that fetches a list of photos from an album whose ID we pass as a parameter. Previously, we stubbed the request.get () method to return a fixed list of photos.
Why do we spy on request.get?
This time, we will spy on the request.get () method so we can verify that our function makes an HTTP request to the API. We'll also check that it made the request once, which is good as we don't want a bug that spams the API's endpoint.
Why do we use spying in testing?
A spy in testing gives us a way of tracking calls made to a method so that we can verify that it works as expected. We use spies to check whether a method was called or not called, how many times it was called, with what arguments it was called, and also the value it returned when called.
What is a spy in testing?
A spy is an object in testing that tracks calls made to a method. By tracking its calls, we can verify that it is being used in the way our function is expected to use it.
What is an anonymous function?
An anonymous function that tracks arguments, values, and calls made to a method.
Why initialize NPM?
Initialize NPM so you can keep track of the packages you install:
What is a spy call?
A spy call is an object representation of an invididual call to a spied function, which could be a fake, spy, stub or mock method.
What does true mean in spy call?
Returns true if the spy call occurred before another call, and no calls to any other spy occurred in-between.
Does calledon accept matcher spycall?
Returns true if obj was this for this call. calledOn also accepts a matcher spyCall.calledOn (sinon.match (fn)) (see matchers ).
When Do You Need Test Doubles?
To best understand when to use test-doubles, we need to understand the two different types of functions we can have. We can split functions into two categories:
Why do we use stubs in code?
You can use them to help test asynchronous code more easily. Stubs can be used to replace problematic code, i.e. the code that makes writing tests difficult.
What happens if a test fails before it is cleaned up?
Without it, if your test fails before your test-doubles are cleaned up, it can cause a cascading failure – more test failures resulting from the initial failure.
What is sinon.config?
sinon.config controls the default behavior of some functions like sinon.test. It also has some other available options.
What would happen if setupnewuser threw an exception in this test?
If setupNewUser threw an exception in this test, that would mean the spy would never get cleaned up, which would wreak havoc in any following tests.
Is Sinon easy to use?
However, getting started with Sinon might be tricky. You get a lot of functionality in the form of what it calls spies, stubs and mocks, but it can be difficult to choose when to use what. They also have some gotchas, so you need to know what you’re doing to avoid problems.
Why do we need to use test doubles in Database.save?
If we want to test setupNewUser, we may need to use a test-double on Database.save because it has a side effect. In other words, we can say that we need test-doubles when the function has side effects .
What is a test spy?
A test spy is a function that records arguments, return value, the value of this and exception thrown (if any) for all its calls. There are two types of spies: Some are anonymous functions, while others wrap methods that already exist in the system under test.
What does true mean in spy?
Returns true if spy was called exactly once in total and that one call was using the exact provided arguments and no others.
What does "return true" mean in spy?
Returns true if spy always returned the provided value.
What is a spy object?
Spy objects are objects returned from sin on.spy (). When spying on existing methods with sinon.spy (object, method), the following properties and methods are also available on object.method.
What is sinon.spy?
sinon.spy (object, "method") creates a spy that wraps the existing function object.method. The spy will behave exactly like the original method (including when used as a constructor), but you will have access to data about all calls. The following is a slightly contrived example:
What is spy.args?
Array of arguments received, spy.args [0] is an array of arguments received in the first call.
What is spy.returnValues?
Array of return values, spy.returnValues [0] is the return value of the first call.
