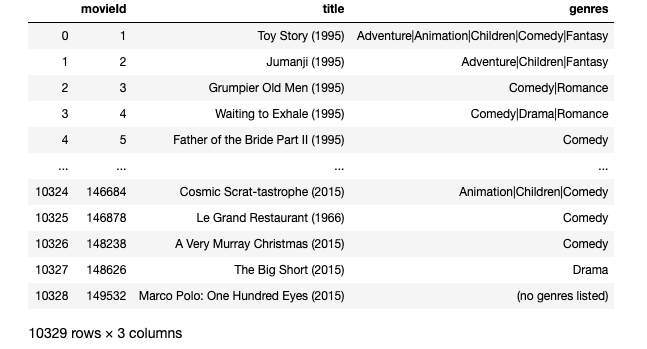
If a super class implements Serializable, then its sub classes do automatically. When an instance of a serializable class is deserialized, the constructor doesn’t run. If a super class doesn’t implement Serializable, then when a subclass object is deserialized, the super class constructor will run.
When should I implement the Serializable interface?
Implement the Serializable interface when you want to be able to convert an instance of a class into a series of bytes or when you think that a Serializable object might reference an instance of your class. Serializable classes are useful when you want to persist instances of them or send them over a wire.
How to make a Java object serializable?
To make a Java object serializable we implement the java.io.Serializable interface. The ObjectOutputStream class contains writeObject () method for serializing an Object. The ObjectInputStream class contains readObject () method for deserializing an object. 1. To save/persist state of an object. 2. To travel an object across a network.
What are the conditions for a class to be serialized successfully?
Notice that for a class to be serialized successfully, two conditions must be met − The class must implement the java.io.Serializable interface. All of the fields in the class must be serializable. If a field is not serializable, it must be marked transient.
What is the difference between Cloneable and Serializable interface?
Similar to the Cloneable interface for Java cloning in serialization, we have one marker interface, Serializable, which works like a flag for the JVM. Any class that implements Serializable interface directly or through its parent can be serialized, and classes that do not implement Serializable can not be serialized.
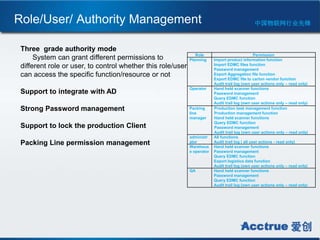
What is the use of implements Serializable?
Implement the Serializable interface when you want to be able to convert an instance of a class into a series of bytes or when you think that a Serializable object might reference an instance of your class. Serializable classes are useful when you want to persist instances of them or send them over a wire.
What happens if I don't implement Serializable?
If our class does not implement Serializable interface, or if it is having a reference to a non- Serializable class, then the JVM will throw NotSerializableException . All transient and static fields do not get serialized.
Is it necessary to implement Serializable?
If you want a class object to be serializable, all you need to do it implement the java. io. Serializable interface. Serializable in java is a marker interface and has no fields or methods to implement.
What happens if the object to be serialized?
Q6) What happens if the object to be serialized includes the references to other serializable objects? Ans) If the object to be serialized includes references to the other objects, then all those object's state also will be saved as the part of the serialized state of the object in question.
What happens if we don't use serialization in Java?
What happens if you try to send non-serialized Object over network? When traversing a graph, an object may be encountered that does not support the Serializable interface. In this case the NotSerializableException will be thrown and will identify the class of the non-serializable object.
What will happen if we don't implement Serializable Java?
The Student would not be Serializable, and it will act like a normal class. Serialization is the conversion of an object to a series of bytes, so that the object can be easily saved to persistent storage or streamed across a communication link.
Why is serialization required?
Serialization allows the developer to save the state of an object and re-create it as needed, providing storage of objects as well as data exchange. Through serialization, a developer can perform actions such as: Sending the object to a remote application by using a web service.
What is advantage of serialization in Java?
Serialization allows us to transfer objects through a network by converting it into a byte stream. It also helps in preserving the state of the object. Deserialization requires less time to create an object than an actual object created from a class. hence serialization saves time.
Why Serializable is an interface?
Serializable is a marker interface (has no data member and method). It is used to "mark" Java classes so that the objects of these classes may get a certain capability. The Cloneable and Remote are also marker interfaces. The Serializable interface must be implemented by the class whose object needs to be persisted.
What does implements serializable mean in Java?
To serialize an object means to convert its state to a byte stream so that the byte stream can be reverted back into a copy of the object. A Java object is serializable if its class or any of its superclasses implements either the java. io.
Can we serialize class without implementing serializable interface?
No. If you want to serialise an object it must implement the tagging Serializable interface.
What happens when you serialize and deserialize an object?
Serialization is a mechanism of converting the state of an object into a byte stream. Deserialization is the reverse process where the byte stream is used to recreate the actual Java object in memory. This mechanism is used to persist the object.
Can we serialize an object without implementing Serializable interface?
No. If you want to serialise an object it must implement the tagging Serializable interface.
Why do we need SerialVersionUID in Java?
The SerialVersionUID must be declared as a private static final long variable in Java. This number is calculated by the compiler based on the state of the class and the class attributes. This is the number that will help the JVM to identify the state of an object when it reads the state of the object from a file.
Should dto be Serializable?
An Object needs to be serialized before being stored in a database because you do not and cannot wish to replicate the dynamically changing arrangement of system memory.
What do you do if an object should not or does not need to be serialized?
Only implement what you need, and don't try to make your class serializable just for the sake of it. If your object has a reference (transitive or direct) to any non-serializable object, and this reference is not marked with the transient keyword, then your object won't be serializable.
Why implement serializable interface?
Because you want to store or send an object. It makes storing and sending objects easy. It has nothing to do with security. Share.
Can an application use serialized forms?
By imposing one scheme, without any way of changing the scheme, applications can not use a scheme most appropriate for them.
Is there a reason to use Java serialization?
This is the recommendation in Effective Java, 3rd Edition by Joshua Bloch: There is no reason to use Java serialization in any new system you write . Oracle's chief architect, Mark Reinhold, is on record as saying removing the current Java serialization mechanism is a long-term goal.
How to know if a Java class is serializable?
If you are curious to know if a Java Standard Class is serializable or not, check the documentation for the class. The test is simple: If the class implements java.io.Serializable, then it is serializable; otherwise, it's not.
What happens after serialization?
After a serialized object has been written into a file, it can be read from the file and deserialized that is , the type information and bytes that represent the object and its data can be used to recreate the object in memory. Most impressive is that the entire process is JVM independent, meaning an object can be serialized on one platform ...
What is serialization in Java?
Java provides a mechanism, called object serialization where an object can be represented as a sequence of bytes that includes the object's data as well as information about the object's type and the types of data stored in the object. After a serialized object has been written into a file, ...
Which class contains many write methods for writing various data types?
The ObjectOutputStream class contains many write methods for writing various data types, but one method in particular stands out −
What is serializable interface?
The Serializable interface is present in java.io package. It is a marker interface. A Marker Interface does not have any methods and fields. Thus classes implementing it do not have to implement any methods. Classes implement it if they want their instances to be Serialized or Deserialized.
What is deserialization in Java?
Deserialization is the reverse process where the byte stream is used to recreate the actual Java object in memory. This mechanism is used to persist the object. Deserialization is done using ObjectInputStream. Thus it can be used to make an eligible for saving its state into a file.
Why does object class not implement serializable interface?
The Object class does not implement Serializable interface because we may not want to serialize all the objects, e.g. serializing a thread does not make any sense because thread running in my JVM would be using my system's memory, persisting it and trying to run it in your JVM would make no sense.
What happens if a serializable class doesn't declare a serialVersionUID?
If a serializable class doesn't declare a serialVersionUID, the JVM will generate one automatically at run-time. If we change our class structure, e.g. remove/add fields, that version number also changes, and according to the JVM, our class is not compatible with the class version of the serialized object.
What Are Serialization and Deserialization?
In Java, we create several objects that live and die accordingly, and every object will certainly die when the JVM dies. But sometimes, we might want to reuse an object between several JVMs or we might want to transfer an object to another machine over the network.
Why is version number used in JVM?
It is used to verify that the serialized and deserialized objects have the same attributes and thus are compatible with deserialization.
What is serializable interface?
Similar to the Cloneable interface for Java cloning in serialization, we have one marker interface, Serializable, which works like a flag for the JVM. Any class that implements Serializable interface directly or through its parent can be serialized, and classes that do not implement Serializable can not be serialized.
What is readresolve used for?
In simple words, readResolve can be used to change the data that is deserialized through the readObject method, and writeReplace can be used to change the data that is serialized through writeObject. Java serialization can also be used to deep clone an object.
What is serialization in computer science?
Well, serialization allows us to convert the state of an object into a byte stream, which then can be saved into a file on the local disk or sent over the network to any other machine. And deserialization allows us to reverse the process, which means reconverting the serialized byte stream to an object again.
What is serialization in Java?
Serialization is a mechanism of converting the state of an object into a byte stream. Deserialization is the reverse process where the byte stream is used to recreate the actual Java object in memory. This mechanism is used to persist the object.
What are some examples of Java marker interfaces?
It is used to “mark” java classes so that objects of these classes may get certain capability. Other examples of marker interfaces are:- Cloneable and Remote. Points to remember. 1. If a parent class has implemented Serializable interface then child class doesn’t need to implement it but vice-versa is not true. 2.
What is transient variable?
In case of transient variables:- A variable defined with transient keyword is not serialized during serialization process.This variable will be initialized with default value during deserialization. (e.g: for objects it is null, for int it is 0).
Can an object serialized on one platform be deserialized on another?
The byte stream created is platform independent. So, the object serialized on one platform can be deserialized on a different platform .
Is a constructor of an object always called when deserialized?
Static data members and transient data members are not saved via Serialization process.So, if you don’t want to save value of a non-static data member then make it transient. 4. Constructor of object is never called when an object is deserialized.
Does serialization runtime use serialVersionUID?
If a serializable class doesn’t explicitly declare a serialVersionUID, then the serialization runtime will calculate a default one for that class based on various aspects of class, as described in Java Object Serialization Specification. However it is strongly recommended that all serializable classes explicitly declare serialVersionUID value, since its computation is highly sensitive to class details that may vary depending on compiler implementations, any change in class or using different id may affect the serialized data.
