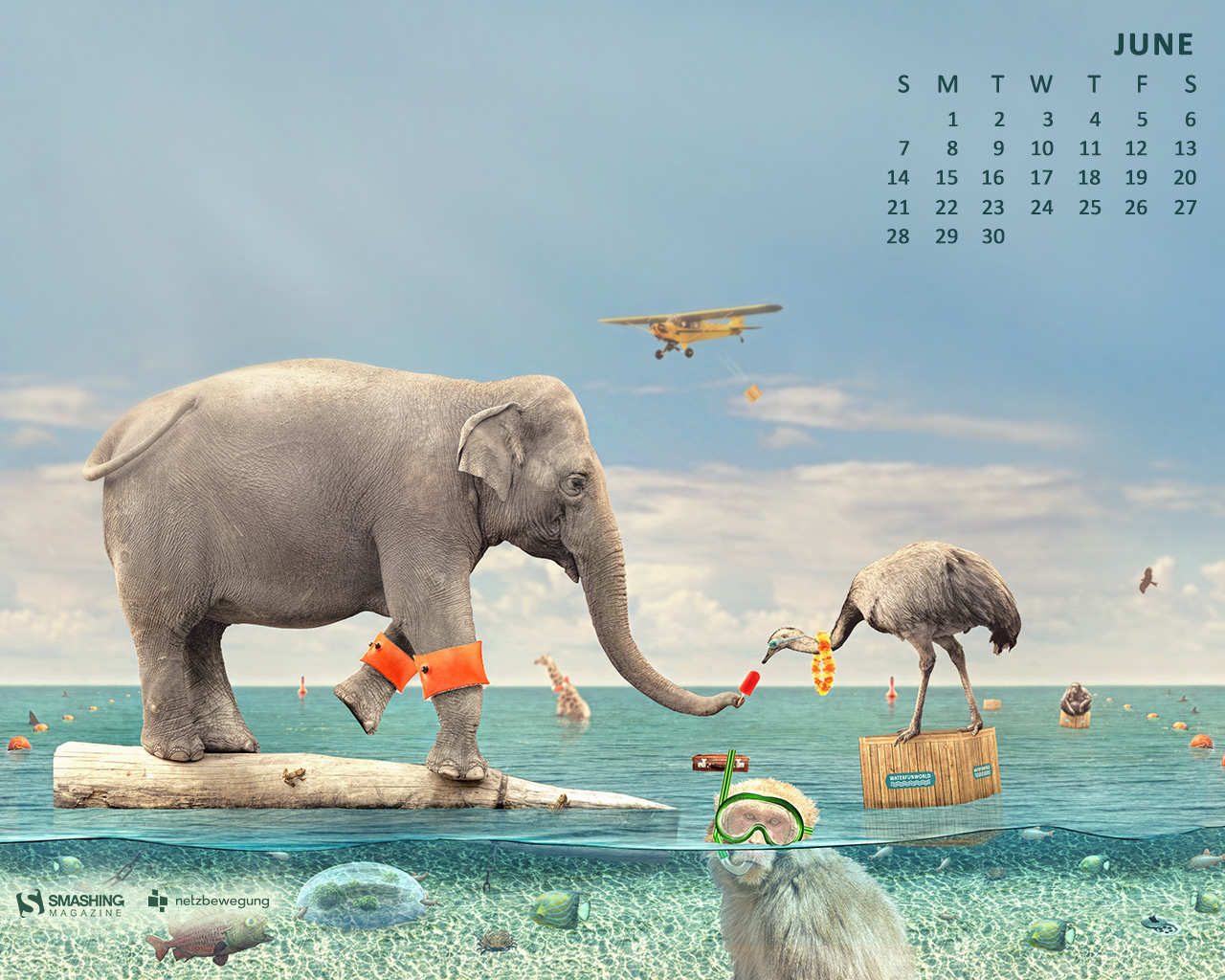
private static method means you can not invoke the method from outside the class as the method is private to the class. What does private static mean? “private” is an access specifier.
Why to use static method?
- Static methods inside a class are usually a good indication of a method that doesn’t belong to this particular class. ...
- Static methods are not polymorphic. ...
- Static methods can’t be used for abstraction and inheritance. ...
- Static methods are bad for testability. ...
What is static and non static method?
Static methods are class methods, and non-static methods are methods that belong to an instance of the class. Static methods can access other static methods and variables without having to create...
What are static methods in a Python class?
Python @staticmethod. There can be some functionality that relates to the class, but does not require any instance (s) to do some work, static methods can be used in such cases. A static method is a method which is bound to the class and not the object of the class. It can’t access or modify class state.
What is public static?
public: The access modifier of the class is public. static: The scope of the method is made to be static which means that all the member variables and the return type will be within the scope of static. void: This keyword in the syntax flow represents that there is no return type handled in the current method.
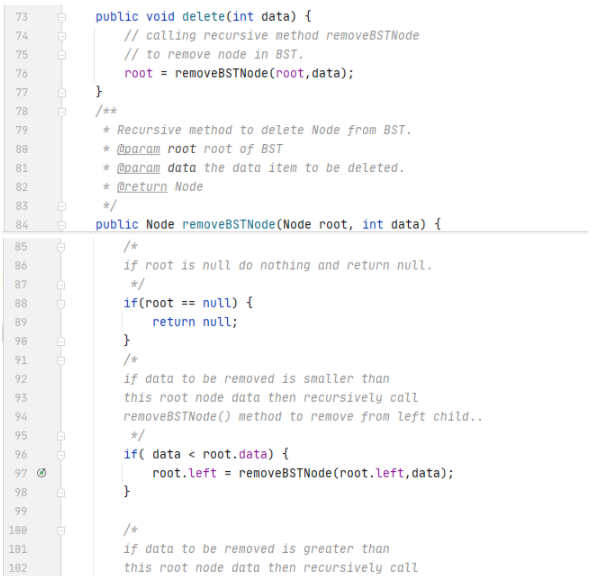
What does private static mean?
"private" is an access specifier. It tells you that the member is only visible inside the class - other classes can't access the private members of a class. "static" means that the variable is a class-level variable; there's only one variable, which is shared by all instances of the class.
What is the point of private static?
Private static variables are useful in the same way that private instance variables are useful: they store state which is accessed only by code within the same class. The accessibility (private/public/etc) and the instance/static nature of the variable are entirely orthogonal concepts.
What is private static and public static?
public static - can be accessed from within the class as well as outside the class. private static - can be access from within the class only.
What is the use of private static methods in C#?
A private constructor prevents the class from being instantiated. The advantage of using a static class is that the compiler can check to make sure that no instance members are accidentally added. The compiler will guarantee that instances of this class cannot be created.
Can you have a private static method?
Yes, we can have private methods or private static methods in an interface in Java 9. We can use these methods to remove the code redundancy. Private methods can be useful or accessible only within that interface only. We can't access or inherit private methods from one interface to another interface or class.
What is the difference between private static and public constructors?
1. Static constructor is called before the first instance of class is created, wheras private constructor is called after the first instance of class is created. 2. Static constructor will be executed only once, whereas private constructor is executed everytime, whenever it is called.
What are private static methods in Java?
Private static methods are methods that can only be accessed within the same class. They are usually used to encapsulate code only used by the class itself, but they don't use the instance members. Having private static methods can improve the maintainability of your code by making it more modular.
Is a static method public or private?
Static methods can be public or private. The static keyword is placed right after the public/private modifier and right before the type of variables and methods in their declarations.
Can a class be private static?
A static class is a class that is created inside a class, is called a static nested class in Java. It cannot access non-static data members and methods. It can be accessed by outer class name. It can access static data members of the outer class, including private.
Why do we use private methods?
Private methods are useful for breaking tasks up into smaller parts, or for preventing duplication of code which is needed often by other methods in a class, but should not be called outside of that class.
Why do we need private methods?
This is what private methods are used for. It is used to hide the inner functionality of any class from the outside world. Private methods are those methods that should neither be accessed outside the class nor by any base class.
Why would you use a private method?
Private methods are typically used when several methods need to do the exact same work as part of their responsibility (like notifying external observers that the object has changed), or when a method is split in smaller steps for readability.
What is the point of a private class?
Private classes are useful for creating building blocks that are implementing internal functionality that you don't necessarily want visible to other projects using a library.
Why would you make a field Private?
Private fields are considered to be internal to the object. So the outside world doesn't need to know about how the data is stored inside the object. This means you can easily change the internal representation of data of an object while everyone else still uses the same accessors / mutatorsmutatorsIn computer science, a mutator method is a method used to control changes to a variable. They are also widely known as setter methods. Often a setter is accompanied by a getter (together also known as accessors), which returns the value of the private member variable.https://en.wikipedia.org › wiki › Mutator_methodMutator method - Wikipedia to do its work.
Are private static variables bad?
Static variables are generally considered bad because they represent global state and are therefore much more difficult to reason about. In particular, they break the assumptions of object-oriented programming.
Should static variables be public or private?
Static variables are created when the program starts and destroyed when the program stops. Visibility is similar to instance variables. However, most static variables are declared public since they must be available for users of the class.
Why use a static method in Java?
A fairly common reason (in Java) would be for initializing immutable field variables in a constructor by using a simple private static method to reduce constructor clutter.
Why is static used in Java?
A fairly common reason (in Java) would be for initializing immutable field variables in a constructor by using a simple private static method to reduce constructor clutter. It is private: external classes should not see it. It is static: it can perform some operation, independent 1 of the state of the host class.
Why do you call static methods without an instance?
1: Your instances have reason to call a static method you don't want called directly, perhaps because it shares data between all instances of your class. 2: Your public static methods have subroutines that you don't want called directly. The method is still called without an instance, just not directly. Of course, "it helps the class make sense" is ...
Why do you call static methods?
1: Your instances have reason to call a static method you don't want called directly, perhaps because it shares data between all instances of your class.
How many reputations do you need to answer a highly active question?
Highly active question. Earn 10 reputation (not counting the association bonus) in order to answer this question. The reputation requirement helps protect this question from spam and non-answer activity.
Which is better, static or nonstatic?
0. Static is always better than non-static and worse than a free functions, which is worse than a pure function. Private is always better than protected which is better than public. 'Need to know' principle.
Can a static method be called without an instance?
2: Your public static methods have subroutines that you don't want called directly. The method is still called without an instance, just not directly. Of course, "it helps the class make sense" is a fine reason all on its own. Share.
What is static private method?
A static private method provides a way to hide static code from outside the class. This can be useful if several different methods (static or not) need to use it, i.e. code-reuse.
What does static mean in a function?
I understand that static means that an object doesn't need to be instantiated for that property/method to be available. I also understand how this applies to private properties and methods and public methods. What I'm trying to understand is what static private function gains you. For example:
Why is a function static?
Making a function a static member of a class rather than a free function gives two advantages: It gives the function access to private and protected members of any object of the class, if the object is static or is passed to the function; It associates the function with the class in a similar way to a namespace.
Why is request_parser static?
It's static, since it doesn't require access to any member variables of request_parser objects. Hence, making it static decouples the function since it reduces the amount of state which the function can access.
What does "help" mean in a sentence?
Asking for help, clarification, or responding to other answers.
Can a pthread be static?
One common application of this is to abstract a pthread implementation in somewhat of an object oriented way. Your thread function must be static, but declaring it private limits the accessibility of that function to the class (to all but the most determined). The thread can be passed an instance of the class it's being "hidden" in, and now has access to perform logic using the object's member data.
Do you have to recompile a member function?
However, changing the type of any member function, public or private, static or not , will require all clients to recompile. If this needs to be done for a private function which those clients can never use, that's a waste of resources. Therefore, I usually move as many functions as possible from the class' private parts into an unnamed namespace in the implementation file.
Is a thread static or private?
Your thread function must be static, but declaring it private limits the accessibility of that function to the class (to all but the most determined). The thread can be passed an instance of the class it's being "hidden" in, and now has access to perform logic using the object's member data. Simplistic Example:
Can a member call is_char?
Only member functions can call is_char () and no static member function is calling is_char (). So is there a reason why these functions are static?
What is a class name in Java?
Class name is used to invoke the static method as static member does not depend on any instance of the class.
What is a static modifier?
Static modifier is used to create variables and methods that will exist independently of any instance created for the class. Static members exists before any instance of the class is created.
What is private static method?
think of private static methods as the membeers that belong to the class and not of any instance of the class.
Can a private member be called from outside the class?
And since the member is private it can not be called from outside the class.
What is public variable?
A public variable is accessible from anywhere (well, anywhere where the class is accessible). A private variable is only accessible inside the class. A static variable belongs to the class rather than to an instance of a class. Notice that the variable DEPARTMENT is also final, which means that it cannot be modified once it is set.
Where is y in a dog?
y can be accessed only from inside Dog via either Dog.y or just y. X can be accessed anywhere via Dog.X or, if you're either in the class or using using static Dog as a header, just X.
What is the meaning of "back up"?
Making statements based on opinion; back them up with references or personal experience.
Where is a public variable accessible?
A public variable is accessible from anywhere (well, anywhere where the class is accessible).
Is a private variable accessible in a class?
A public variable is accessible everywhere in the code - a private variable is only accessible within the class itself. In this case you're using Employee.salary within the Employee class, so that's fine.
Can you access a static variable from outside of a class?
X can be accessed anywhere via Dog.X or, if you're either in the class or using using static Dog as a header, just X. Obviously, you cannot access private static variable from outside the class, and you can access public static variable from everywhere.
Can static be accessed from within a class?
public static - can be accessed from within the class as well as outside the class.
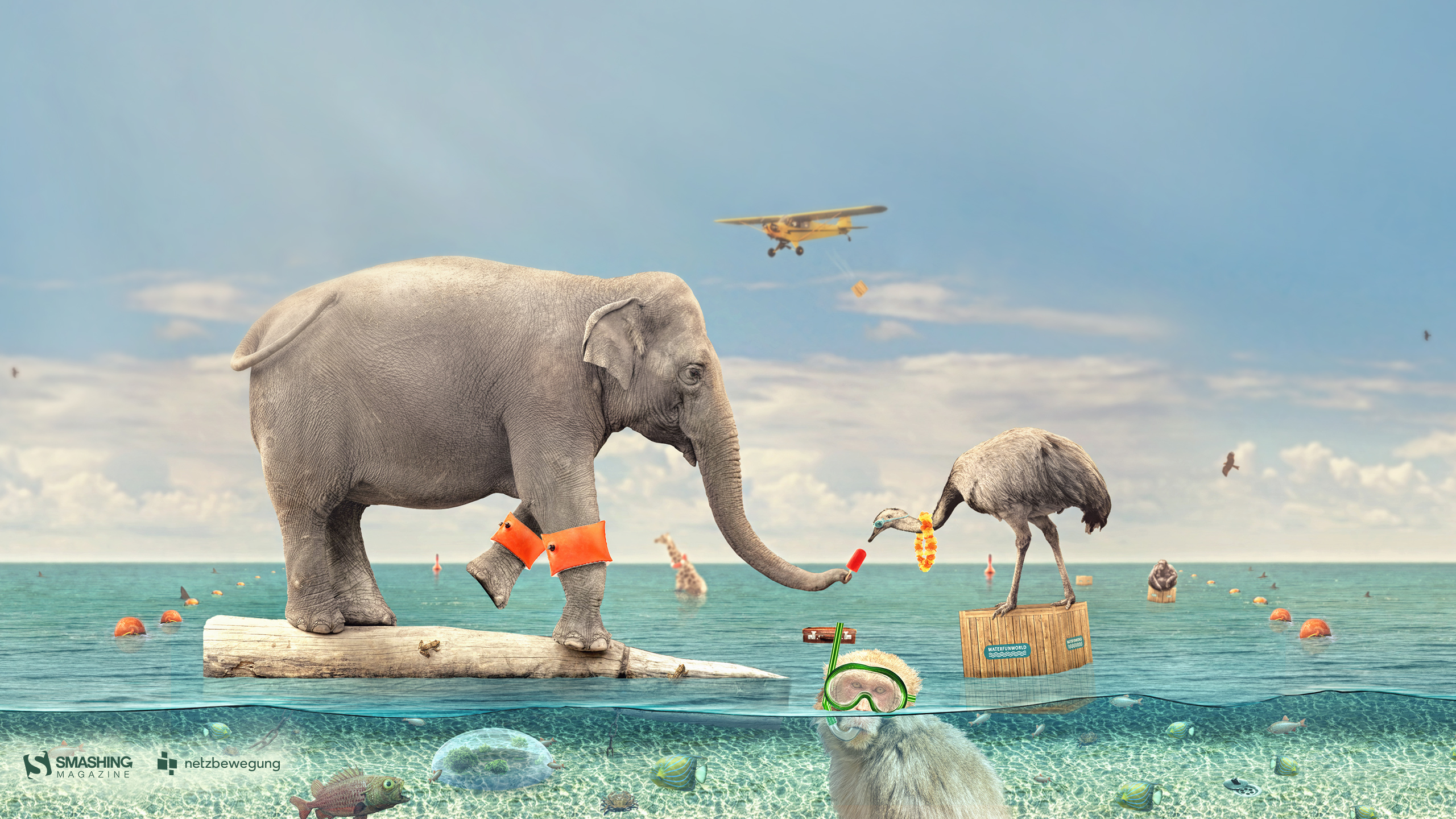