
Promise
- Description. A Promise is a proxy for a value not necessarily known when the promise is created. ...
- Constructor. Creates a new Promise object. ...
- Static methods. Wait for all promises to be resolved, or for any to be rejected. ...
- Instance methods. See the Microtask guide to learn more about how these methods use the Microtask queue and services.
- Examples. ...
What is a promise in JavaScript?
A promise is simply a placeholder for an asynchronous task which is yet to be completed. When you define a promise object in your script, instead of returning a value immediately, it returns a promise.
What is a future promise in programming language?
Futures and promises. In computer science, future, promise, delay, and deferred refer to constructs used for synchronizing program execution in some concurrent programming languages. They describe an object that acts as a proxy for a result that is initially unknown, usually because the computation of its value is not yet complete.
Why do we use promises instead of events?
They are easy to manage when dealing with multiple asynchronous operations where callbacks can create callback hell leading to unmanageable code. Prior to promises events and callback functions were used but they had limited functionalities and created unmanageable code.
How to create a promise using anonymous function?
A promise can be created using Promise constructor. Promise constructor takes only one argument which is a callback function (and that callback function is also referred as anonymous function too).
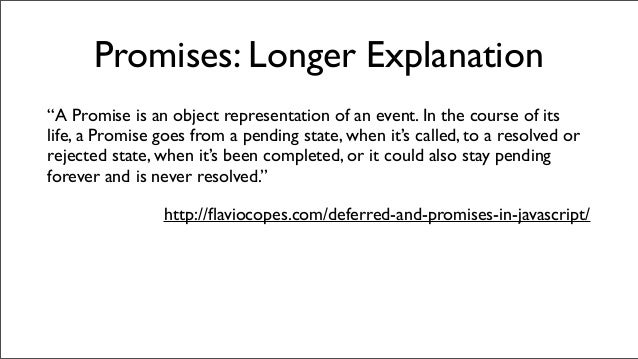
What is promise and why do we use it?
Promises are used to handle asynchronous operations in JavaScript. They are easy to manage when dealing with multiple asynchronous operations where callbacks can create callback hell leading to unmanageable code.
What is promise and how it works?
A promise is an object that may produce a single value some time in the future : either a resolved value, or a reason that it's not resolved (e.g., a network error occurred). A promise may be in one of 3 possible states: fulfilled, rejected, or pending.
What is promise and callback?
A callback function is passed as an argument to another function whereas Promise is something that is achieved or completed in the future. In JavaScript, a promise is an object and we use the promise constructor to initialize a promise.
What is promise and async?
A promise is used to handle the asynchronous result of an operation. JavaScript is designed to not wait for an asynchronous block of code to completely execute before other synchronous parts of the code can run. With Promises, we can defer the execution of a code block until an async request is completed.
What is an example of a promise?
as long as the sun rises at dawn each day. I promise to love you as long as you smile at me in your special way. I promise to give you some time on your own so to your own self be true. I promise to give you my help and support always, in all that you do.
What is the full meaning of promise?
Definition of promise (Entry 1 of 2) 1a : a declaration that one will do or refrain from doing something specified. b : a legally binding declaration that gives the person to whom it is made a right to expect or to claim the performance or forbearance of a specified act.
What is difference between async and await and promise?
Promise is an object representing intermediate state of operation which is guaranteed to complete its execution at some point in future. Async/Await is a syntactic sugar for promises, a wrapper making the code execute more synchronously. 2.
Is callback same as promise?
With callback we pass a callback into a function that would then get called upon completion. With promises, you attach callbacks on the returned promise object. A callback is a function that is to be executed after another function has finished executing. Async callbacks are functions that are passed as arguments.
What is difference between async and await?
The async keyword is used to define an asynchronous function, which returns a AsyncFunction object. The await keyword is used to pause async function execution until a Promise is fulfilled, that is resolved or rejected, and to resume execution of the async function after fulfillment.
Is await a promise?
The await operator is used to wait for a Promise . It can only be used inside an async function within regular JavaScript code; however it can be used on its own with JavaScript modules.
Why async is used?
Async means asynchronous. It allows a program to run a function without freezing the entire program. This is done using the Async/Await keyword. Async/Await makes it easier to write promises.
Is promise all asynchronous?
If a nonempty iterable is passed, and all of the promises fulfill, or are not promises, then the promise returned by this method is fulfilled asynchronously.
How important is a promise?
Promises are commitments People with strong relationships rank higher in emotional intelligence and are more likely to stay loyal to their commitments. Whether the commitment is to yourself or to someone else, making a promise is a commitment that you will keep your word. It is a commitment that reinforces trust.
What is a promise according to the Bible?
In the New Covenant scriptures, promise (epangelia) is used in the sense of God's design to visit his people redemptively in the person of his son Jesus Christ. W. E. Vine says that a promise is "a gift graciously bestowed, not a pledge secured by negotiation."
What happens if you break a promise?
What happens when a promise is broken? One of the most significant consequences of breaking promises you made to your partner is that they're more likely to lose their trust in you, which can sometimes irreparably damage your relationship.
How do you make a promise?
0:133:13Learn In Simple English how to Make Promises - YouTubeYouTubeStart of suggested clipEnd of suggested clipMaking a promise means giving your word to someone that. What you are saying is the truth it canMoreMaking a promise means giving your word to someone that. What you are saying is the truth it can also mean that you are giving guarantee to someone that you will do what you are saying to them.
Anurag Shrivastava Follow
Promises are a new way to process the asynchronous events in JavaScript. Node.js programmers are already familiar with nested callbacks to handle the asynchronous events. In the extreme cases when the depth of nested callbacks is too much then the code become difficult to read and maintain. Promises offer a way to get rid of nested callbacks.
What is a Promise?
In real life, a promise is an assurance given by somebody to somebody that something will happen. In programming, Promise means that a program calls a function in the anticipation that it will do some useful thing and return the result which calling program can use.
Understanding the Promises Using Code
We will order Pizza in a shop called Pizza Factory where we can place pizza order. Placing pizza order is a kind of promise which will either return a pizza to us which we can eat or it will fail because pizza is burnt. The following code models this situation:
Promises in Other Programming Languages
Promise functionality is gaining adoption in several programming languages either through external libraries or as the core part of language itself. Jdeferred is one such example from the Java language.
Deep Dive in Promises
If you are interested in deep diving in the topic of promises then please visit the following links:
About the Author
Anurag Shrivastava is an entrepreneur, tech geek, book author, blogger, and an expert in payments tech and fast data processing, based in The Netherlands, working at ING. He is involved in building the UPI enabled payments platform and the app known as HandyPay.
What is a promise in JavaScript?
Promises are a comparatively new feature of the JavaScript language that allow you to defer further actions until after a previous action has completed, or respond to its failure. This is useful for setting up a sequence of async operations to work correctly. This article shows you how promises work, how you'll see them in use with web APIs, ...
What are promises?
We looked at Promises briefly in the first article of the course, but here we'll look at them in a lot more depth.
Why are promises important?
Promises are important to understand because most modern Web APIs use them for functions that perform potentially lengthy tasks. To use modern web technologies you'll need to use promises.
What happens when a fulfilled promise is returned?
Bear in mind that the value returned by a fulfilled promise becomes the parameter passed to the next .then () block's callback function.
What is the finally method?
In more recent modern browsers, the .finally () method is available, which can be chained onto the end of your regular promise chain allowing you to cut down on code repetition and do things more elegantly. The above code can now be written as follows:
Why are promises important in asynchronous programming?
Promises are a good way to build asynchronous applications when we don’t know the return value of a function or how long it will take to return. They make it easier to express and reason about sequences of asynchronous operations without deeply nested callbacks, and they support a style of error handling that is similar to the synchronous try...catch statement.
What does "resolve" and "reject" mean?
resolve () and reject () are functions that you call to fulfil or reject the newly-created promise. In this case, the promise fulfills with a string of "Success!".
What is a Promise object in JavaScript?
A JavaScript Promise object contains both the producing code and calls to the consuming code:
What are the properties of a Promise object?
The Promise object supports two properties: state and result. While a Promise object is "pending" (working), the result is undefined. When a Promise object is "fulfilled", the result is a value. When a Promise object is "rejected", the result is an error object. myPromise.state.
How many arguments does Promise.then take?
Promise.then () takes two arguments, a callback for success and another for failure.
What is a promise in JavaScript?
Promises are used to handle asynchronous operations in JavaScript. They are easy to manage when dealing with multiple asynchronous operations where callbacks can create callback hell leading to unmanageable code.
What are the benefits of a promise?
Benefits of Promises. Improves Code Readability. Better handling of asynchronous operations. Better flow of control definition in asynchronous ...
How many states does a promise have?
A Promise has four states: fulfilled: Action related to the promise succeeded. rejected: Action related to the promise failed. pending: Promise is still pending i.e not fulfilled or rejected yet. settled: Promise has fulfilled or rejected. A promise can be created using Promise constructor. Syntax.
What is JavaScript used for?
JavaScript is best known for web page development but it is also used in a variety of non-browser environments. You can learn JavaScript from the ground up by following this JavaScript Tutorial and JavaScript Examples.
Promises
The Promise object represents the eventual completion (or failure) of an asynchronous operation, and its resulting value. A Promise is used to overcome issues with multiple callbacks and provides a better way to manage success and error conditions.
Async and await
Think of async and await as syntactic sugar wrapped around Promises to make them easier to work with. Below is an example of chaining Promises:
Sign up to our newsletter
Personalisation, Digital Transformation, Content, Marketing Automation and more...
What is the difference between a promise and a future?
Specifically, when usage is distinguished, a future is a read-only placeholder view of a variable, while a promise is a writable, single assignment container which sets the value of the future. Notably, a future may be defined without specifying which specific promise will set its value, and different possible promises may set the value ...
How did futures and promises come about?
Futures and promises originated in functional programming and related paradigms (such as logic programming) to decouple a value (a future) from how it was computed (a promise), allowing the computation to be done more flexibly, notably by parallelizing it. Later, it found use in distributed computing, in reducing the latency from communication round trips. Later still, it gained more use by allowing writing asynchronous programs in direct style, rather than in continuation-passing style .
How does futures reduce latency?
The use of futures can dramatically reduce latency in distributed systems. For instance, futures enable promise pipelining, as implemented in the languages E and Joule, which was also called call-stream in the language Argus .
What is implicit future?
Explicit futures can be implemented as a library, whereas implicit futures are usually implemented as part of the language. The original Baker and Hewitt paper described implicit futures, which are naturally supported in the actor model of computation and pure object-oriented programming languages like Smalltalk.
What is call by future?
The evaluation strategy of futures, which may be termed call by future, is non-deterministic: the value of a future will be evaluated at some time between when the future is created and when its value is used, but the precise time is not determined beforehand and can change from run to run. The computation can start as soon as the future is created ( eager evaluation) or only when the value is actually needed ( lazy evaluation ), and may be suspended part-way through, or executed in one run. Once the value of a future is assigned, it is not recomputed on future accesses; this is like the memoization used in call by need .
Who invented promise pipelining?
The promise pipelining technique (using futures to overcome latency) was invented by Barbara Liskov and Liuba Shrira in 1988, and independently by Mark S. Miller, Dean Tribble and Rob Jellinghaus in the context of Project Xanadu circa 1989.
Does Alice ML support promise?
Alice ML also supports futures that can be resolved by any thread, and calls these promises. This use of promise is different from its use in E as described above. In Alice, a promise is not a read-only view, and promise pipelining is unsupported. Instead, pipelining naturally happens for futures, including ones associated with promises.
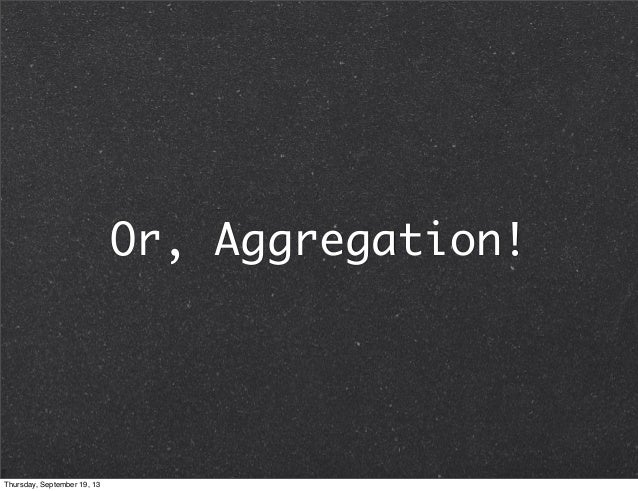