
What makes a function pure?
A function is called pure function if it always returns the same result for same argument values and it has no side effects like modifying an argument (or global variable) or outputting something. The only result of calling a pure function is the return value. Examples of pure functions are strlen(), pow(), sqrt() etc. Examples of impure functions are printf(), rand(), time(), etc.
Can I make a pure virtual function static?
Virtual functions are invoked when you have a pointer or reference to an instance of a class. Static functions aren’t tied to the the instance of a class but they are tied to the class. C++ doesn’t have pointers-to-class, so there is no scenario in which you could invoke a static function virtually.
How about "pure virtual methods"?
A pure virtual function or pure virtual method is a virtual function that is required to be implemented by a derived class if the derived class is not abstract. Classes containing pure virtual methods are termed "abstract" and they cannot be instantiated directly.
What is advantage of virtual functions?
Advantages of Virtual Keyword in C++. Virtual functions are used to achieve runtime polymorphism.; If a class is derived from a class having a virtual function, then the function definition can be redefined in the derived class.

How do you fix a pure virtual function call?
Fix Runtime error R6025 Pure Virtual Function CallRepair the app using Apps & features.Uninstall and then reinstall the app.Check for an updated version of the program.Install Visual C++ Redistributable Packages.Repair Microsoft NET Framework 3.5.Troubleshoot in Clean Boot State.Run System File Checker (SFC) scan.
What is the purpose of pure virtual function?
A pure virtual function makes it so the base class can not be instantiated, and the derived classes are forced to define these functions before they can be instantiated. This helps ensure the derived classes do not forget to redefine functions that the base class was expecting them to.
Can a pure virtual function be called using an object?
As well as the standard case of calling a virtual function from the constructor or destructor of an object with pure virtual functions you can also get a pure virtual function call (on MSVC at least) if you call a virtual function after the object has been destroyed.
What happens when a virtual function is called?
A virtual function is a member function that you expect to be redefined in derived classes. When you refer to a derived class object using a pointer or a reference to the base class, you can call a virtual function for that object and execute the derived class's version of the function.
What is difference between virtual and pure virtual function?
A virtual function is a member function of base class which can be redefined by derived class. A pure virtual function is a member function of base class whose only declaration is provided in base class and should be defined in derived class otherwise derived class also becomes abstract.
Which is true about pure virtual function?
Which of the following is true about pure virtual functions? 1) Their implementation is not provided in a class where they are declared. 2) If a class has a pure virtual function, then the class becomes abstract class and an instance of this class cannot be created.
How do you write a pure virtual function?
A pure virtual function doesn't have the function body and it must end with = 0 . For example, class Shape { public: // creating a pure virtual function virtual void calculateArea() = 0; }; Note: The = 0 syntax doesn't mean we are assigning 0 to the function.
How do you create a pure virtual function?
A pure virtual function is declared by assigning 0 in declaration. See the following example.
What happens if you call a pure virtual function C++?
Calling a pure virtual function from a constructor is undefined behaviour even if it has an implementation.
What is virtual and pure virtual function explain with example?
A virtual function is a member function in a base class that can be redefined in a derived class. A pure virtual function is a member function in a base class whose declaration is provided in a base class and implemented in a derived class. The classes which are containing virtual functions are not abstract classes.
What is a pure virtual function Mcq?
Explanation: Pure virtual function is a virtual function which has no definition/implementation in the base class. 3.
Can virtual functions private?
A virtual function can be private as C++ has access control, but not visibility control. As mentioned virtual functions can be overridden by the derived class but under all circumstances will only be called within the base class.
What is pure virtual function Mcq?
Explanation: Pure virtual function is a virtual function which has no definition/implementation in the base class. 3.
Why do we use virtual functions in C++?
A virtual function in C++ helps ensure you call the correct function via a reference or pointer. The C++ programming language allows you only to use a single pointer to refer to all the derived class objects.
What is pure virtual function in C# net?
A pure virtual function is terminology of C++ but in C# if a function which is implemented in Derived class and that derived class is not an Abstract class.
What is virtual and pure virtual function explain with example?
A virtual function is a member function in a base class that can be redefined in a derived class. A pure virtual function is a member function in a base class whose declaration is provided in a base class and implemented in a derived class. The classes which are containing virtual functions are not abstract classes.
Why can't we provide an implementation of all functions in a base class?
Sometimes implementation of all function cannot be provided in a base class because we don’t know the implementation. Such a class is called abstract class. For example, let Shape be a base class. We cannot provide implementation of function draw () in Shape, but we know every derived class must have implementation of draw ().
Can an animal class have an abstract function?
Similarly an Animal class doesn’t have implementation of move () (assuming that all animals move), but all animals must know how to move. We cannot create objects of abstract classes. A pure virtual function (or abstract function) in C++ is a virtual function for which we can have implementation, But we must override that function in ...
Why does my virtual function call fail?
The runtime error pure virtual function call may happen due to antivirus and software conflicts. To check if there are any software conflicts, you can try performing a clean boot. A clean boot allows you to boot Windows with a minimal set of drivers and startup programs.
What is a R6025 call?
The r6025 pure virtual function call is a runtime error that crashes some software. When the error pops up, you may get the message stating “Runtime Error! Program: C:Program Files Program Name R6025 – pure virtual function call”.
Can dynamic dispatch be pure virtual?
If you want to take one thing away from it, then it is that dynamic dispatch cannot find a pure-virtual function. And of course that you must never call virtual functions within a constructor.
Can you call a non-pure virtual function from a constructor?
One may actually call a non-pure virtual function from a constructor or destructor, but then you must know that what is going to get called is the method from the class itself and not anything polymorphic.
Can a compiler do virtual dispatch?
Edit: Here's some more explanation. Compilers are perfectly allowed and encouraged to perform virtual dispatch statically if they can do so. In that case, it is determined at compile time already which actual function will be called. This happens when you say foo () or this->foo () in the Base constructor, or when you say x.Base::foo () in some other context where Derived x; is your object. When the dispatch happens statically, then either the implementation of Base::foo () is called directly, or you get a linker error if there is no implementation.
Can you instantiate a class with pure virtual functions?
This cannot happen under "normal" conditions, because the compiler won't let you instantiate a class with pure-virtual functions, and so the target of an ordinary dynamic dispatch is always a function for which an implementation must exist (or at least you'd get a linker error if you don't link it).
Does a dipatch end at a pure virtual function?
But there is one more situation, which is the one in question: The compiler decides to perform the dispatch at runtime, for whatever reason, and the dipatch ends at a pure-virtual function. In that case your program terminates. It is irrelevant whether the function is implemented or not, but is simply doesn't have an entry in the polymorphic class hierarchy (think of it as a "null pointer in the vtable", hence the = 0 ). For this to happen, the dynamic type of the object must be the type of an abstract base class, and the dispatch has to happen dynamically. The former can only be achieved inside the base constructor of a derived object, and the latter requires you to convince the compiler not to dispatch the call statically. This is where the difference between this->foo () (static) and Base * p = this; p->foo (); (dynamic) comes in. (Also contrast this to x.Base::foo (), which is dispatched statically .)
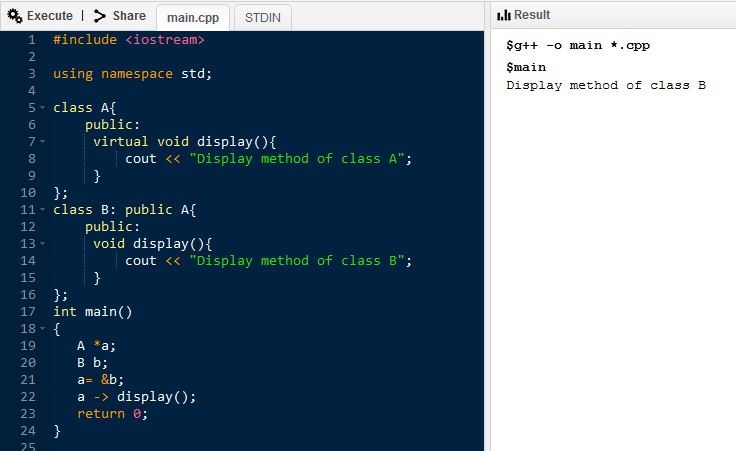