
A self-invoking (also called self-executing) function is a nameless (anonymous) function that is invoked immediately after its definition. An anonymous function is enclosed inside a set of parentheses followed by another set of parentheses (), which does the execution (function(){ console.log(Math.PI); })();
What is self-invoking function in JavaScript?
JavaScript Self invoking functions are nameless self-executing functions and invoked immediately after defining it. These self-invoking functions are man-made, these functions will execute automatically when followed by (). Without (), a function cannot be self-invoked.
How do I invoke a self-invoking expression?
A self-invoking expression is invoked (started) automatically, without being called. Function expressions will execute automatically if the expression is followed by (). You cannot self-invoke a function declaration. You have to add parentheses around the function to indicate that it is a function expression:
What is immediately invoked function expressions?
Javascript Web Development Object Oriented Programming The self-invoking function in JavaScript are known as Immediately Invoked Function Expressions (IIFE). Immediately Invoked Function Expressions (IIFE) is a JavaScript function that executes immediately after it has been defined so there is no need to manually invoke IIFE.
What is the difference between self-invoking and anonymous functions?
A self-invoking function is a function that executes automatically when it is defined. In other words, it calls itself. An anonymous function is defined inside a set of parentheses followed by another set of parentheses. It is also known as an anonymous executing function or an immediately invoked function expression ( IIFE ).

Why do we need self-invoking function?
Self-invoking functions are useful for initialization tasks and for one-time code executions, without the need of creating global variables. Parameters can also be passed to self-invoking functions as shown in the example below.
What is invoking of a function?
Invoking a JavaScript Function The code inside a function is not executed when the function is defined. The code inside a function is executed when the function is invoked. It is common to use the term "call a function" instead of "invoke a function".
What is invoking a function in JavaScript?
A function is invoked when the code inside the function is executed by calling it. The term invoking and calling a function is the same in JavaScript. A function can be called multiple times only after defining it once.
What is an IIFE and why do you want one?
Published May 20 2018 , Last Updated Jun 16 2020. An Immediately-invoked Function Expression (IIFE for friends) is a way to execute functions immediately, as soon as they are created. IIFEs are very useful because they don't pollute the global object, and they are a simple way to isolate variables declarations.
What are the 2 ways of invoking a function?
Two ways of invoking functions are: Pass by value. Pass by reference.
What does invoking mean in programming?
The process of activating. The term invoke is usually used to refer to a routine or function in a program. Call and invoke are synonymous terms in this sense.
Is calling and invoking a function same?
Function calling is when you call a function yourself in a program. While function invoking is when it gets called automatically. Here, when line 1 is executed, the function(constructor, i.e. s) is invoked. When line 2 is executed, the function sum is called.
How do you invoke a method?
Instead, you must follow these steps:Create a Class object that corresponds to the object whose method you want to invoke. See the section Retrieving Class Objects for more information.Create a Method. object by invoking getMethod on the Class object. ... Invoke the method by calling invoke .
How many methods are there to invoke a function?
two waysYou can invoke or call a function in two ways: call by value and call by function.
What is IIFE pattern?
IIFE (Immediately Invokable Function Expression) is a important concept in JavaScript. it is a commonly used Design Pattern which is used to wrap set of variables and functions which cannot be accessed outside the enclosed scope.
Can you explain IIFE with code?
An IIFE (Immediately Invoked Function Expression) is a JavaScript function that runs as soon as it is defined. The name IIFE is promoted by Ben Alman in his blog.
What is IIFE format?
Immediately Invoked Function Expression (IIFE) Module We can use this format to create a bundle for an application. It helps us to put things into namespaces to avoid variable collisions and keep code private. The following is a Rollup-generated file in IIFE format: This code works in a browser.
What is the difference between invoking and calling a function?
With call , you can write a method once and then inherit it in another object, without having to rewrite the method for the new object. So, the major difference between invoking and calling comes in terms of the this object. Calling let's you set the this value whereas invoking just ties it to the global object.
How do you invoke a method?
Instead, you must follow these steps:Create a Class object that corresponds to the object whose method you want to invoke. See the section Retrieving Class Objects for more information.Create a Method. object by invoking getMethod on the Class object. ... Invoke the method by calling invoke .
What is invoking in C++?
When a function is invoked, you pass a value to the parameter. This value is referred to as actual parameter or argument. The parameter list refers to the type, order, and number of the parameters of a function. Parameters are optional; that is, a function may contain no parameters.
What is meant by calling or invoking a function in Python?
Summary. To use functions in Python, you write the function name (or the variable that points to the function object) followed by parentheses (to call the function). If that function accepts arguments (as most functions do), then you'll pass the arguments inside the parentheses as you call the function.
Why Self Invoking Functions Work?
In JavaScript, each function has its own scope. Take this, for example, variables defined using ‘var’ inside functions cannot be accessed outside. By surrounding the applications with anonymous functions, we create a scope around the function where variables declared are only accessible by that function. We can make the variables declared accessible outside the function but by default we don’t have this feature applicable to her. Let us have a look at some of the key points of JavaScript Self Invoking Functions.
What are the advantages of self-invoking functions?
As these functions are unnamed or anonymous, expression of the function is immediately invoked even without using any identifier or closure and not spoiling the scope.
What is self invoking in JavaScript?
JavaScript Self invoking functions are nameless self-executing functions and invoked immediately after defining it . These self-invoking functions are man-made, these functions will execute automatically when followed by ( ). Without ( ), a function cannot be self-invoked.
What is an anonymous function?
These anonymous functions are arguments passed to higher-order functions that needs to return a function. If the function is used only once, using a self Invoking function is easier as it is light weighted syntactically compared to the named function.
Which pair of parentheses continues the call to the function?
the second pair of parentheses continues the call to the function.
Is ready a self-invoking function?
This is useful in initialization, adding event listeners, etc. In jQuery, instead of using document. ready, a self-invoked function was used.
Can parameters be passed to self invoked functions?
Parameters can also be passed to these Self invoked functions, generally, references to global objects are passed as parameters. Not too many arguments should be passed as functions become large and users need to scroll for the parameters. This Self Invoking function are mainly for variable scoping.
What are Self-Invoking Functions in JavaScript
In JavaScript, a “ Self-Invoking ” function is a type of function that is invoked or called automatically after its definition. The execution of such an anonymous function is done by enclosing it in a parenthesis set followed by another set of parenthesis.
How Self-Invoking Functions work in JavaScript
As the self-invoking functions are defined anonymously in JavaScript, there are no local or global variables except for the declarations in the body of the functions. When you initialize a self-invoking function, it immediately runs and can be executed one time. No reference will be saved for the self-invoking function, including the return value.
Syntax of Self-Invoking Functions in JavaScript
Now, let’s check out the syntax of the self-invoking functions in JavaScript:
Example: Using Self-Invoking Functions in JavaScript
In the following example, we will define a self-invoking function that prints out “ Hi! I am calling myself ” as soon as the function definition code is executed. Note that we do not have to call the defined self-invoking function ourselves:
Example2: Difference between Self-Invoking function and Normal function
If you are a JavaScript beginner, then at this point, you might get confused between the syntax and functionality of a normal function and a self-invoking function. No worries! This section will assist you in this regard.
Conclusion
Self-Invoking function is a type of function that is invoked or called automatically after its definition when followed by the parentheses set () and primarily used for the initialization tasks. This write-up demonstrated the syntax and usage of self-invoking functions in JavaScript to wrap the code inside a function scope.
Why is my self-invoking function not working?
So because your 'self-invoking function' is a basic part of javascript, there is no possible way it's not working unless the insides aren't working or your environment is messed up . You could copy-paste your code onto a new blank page, and it would work fine. Something else must be going wrong:
Does Safari self invoke function work?
This self invoking function with return value will work in all current browsers(Safari, Chrome and Firefox) without issue. This function executes immediately, automatically and anonymously.
Is self-invoking a part of JavaScript?
"Self-invoking functions" are not really a part of javascript, it's just a term that people are calling a specific pattern of code (like AJAX, etc.); these patterns should work anywhere that javascript works.
Does console.log work?
This function definitely works. I would check your browser's console for any js errors in your page. Perhaps you could try to put a simple console.log function at the beginning of your script to see if any JavaScript is being called in the first place.
When do functions execute?
Function expressions will execute automatically if the expression is followed by ().
What is a function constructor?
The Function () Constructor. As you have seen in the previous examples, JavaScript functions are defined with the function keyword. Functions can also be defined with a built-in JavaScript function constructor called Function (). You actually don't have to use the function constructor.
Why do JavaScript functions have semicolons?
They are "saved for later use", and will be executed later, when they are invoked (called upon). Semicolons are used to separate executable JavaScript statements. Since a function declaration is not an executable statement, it is not common to end it with a semicolon.
What is an arrow function?
Arrow functions allows a short syntax for writing function expressions.
What happens after a function expression is stored in a variable?
After a function expression has been stored in a variable, the variable can be used as a function:
Can you omit the return keyword?
You can only omit the return keyword and the curly brackets if the function is a single statement. Because of this, it might be a good habit to always keep them:
Is arrow function supported in IE11?
Arrow functions are not supported in IE11 or earlier.
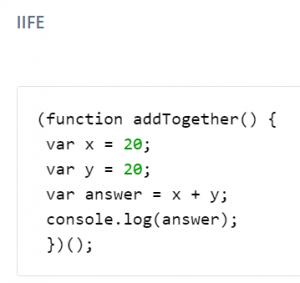
Syntax
Why Self Invoking Functions Work?
- In JavaScript, each function has its own scope. Take this, for example, variables defined using ‘var’ inside functions cannot be accessed outside. By surrounding the applications with anonymous functions, we create a scope around the function where variables declared are only accessible by that function. We can make the variables declared accessible outside the functio…
Advantages of Self Invoking Functions/Anonymous Functions
- As these functions are unnamed or anonymous, expression of the function is immediately invoked even without using any identifier or closure and not spoiling the scope.
- These anonymous functions are arguments passed to higher-order functions that needs to return a function. If the function is used only once, using a self Invoking function is easier as it is light...
- As these functions are unnamed or anonymous, expression of the function is immediately invoked even without using any identifier or closure and not spoiling the scope.
- These anonymous functions are arguments passed to higher-order functions that needs to return a function. If the function is used only once, using a self Invoking function is easier as it is light...
- These functions also accept arguments but not many arguments should be passed as it not recommended. Even the names of arguments being simpler, code would look complex and clumsy.
- Used for creating artificial namespace which do not exist in JavaScript.
Conclusion
- With this, we conclude our topic ‘JavaScript Self Invoking Functions’, We have seen what it means and how is it used in coding. Illustrated a few examples on how to use Anonymous function, and also have seen the difference between Named function and Anonymous/ Self Invoked functions. These functions can help us in creating a scope and prevent polluting global namespace. Also u…
Recommended Articles
- This is a guide to JavaScript Self Invoking Functions. Here we discuss the Introduction to JavaScript Self Invoking Functions and how it works along with Examples and Code Implementation. You can also go through our other suggested articles to learn more – 1. JavaScript String Format With Methods 2. How Does Anonymous Function Works in JavaScript…