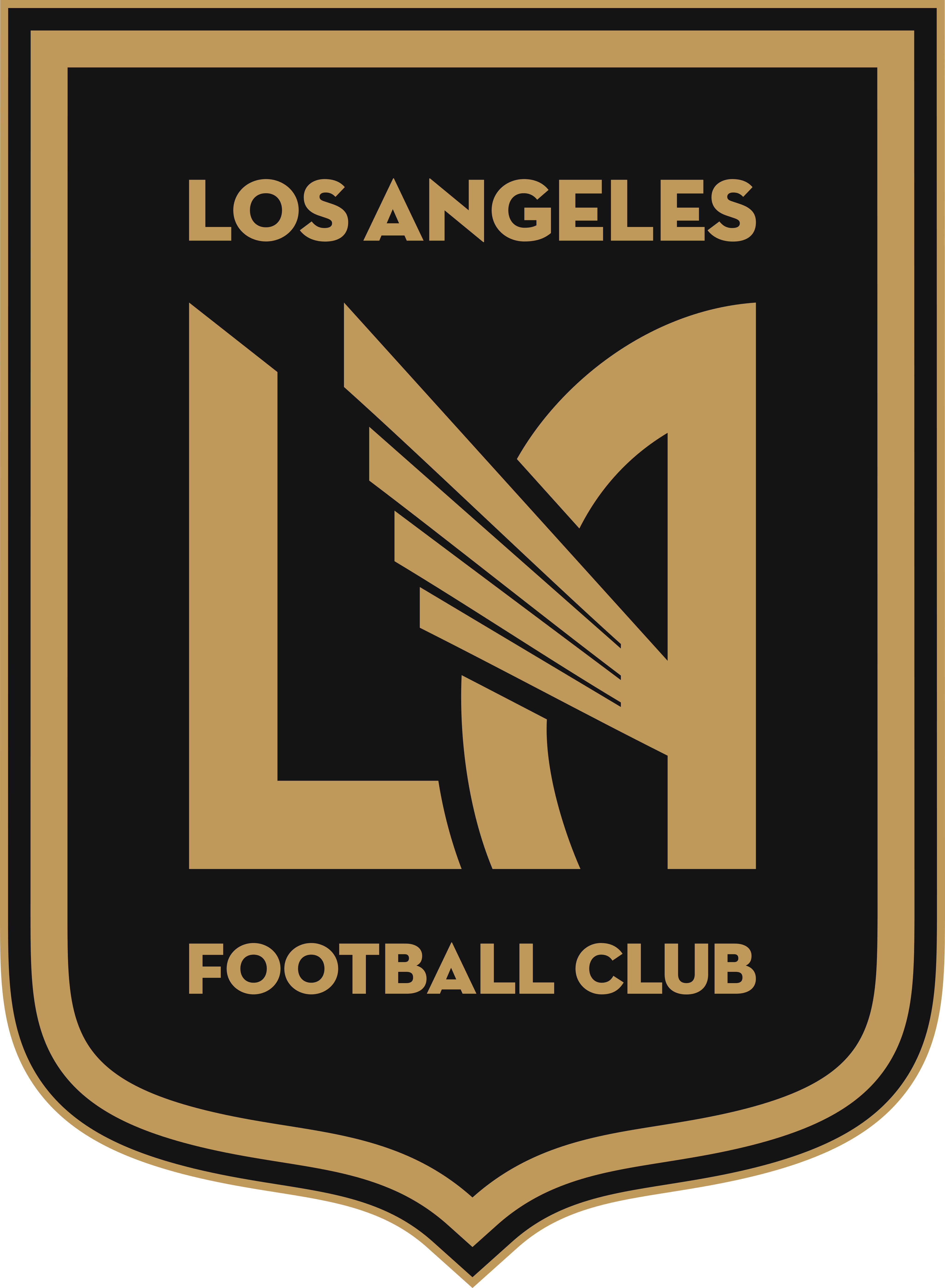
What is the difference between vector and array in C++?
Additionally, arrays can only store primitive data types, like int or float. A vector is a type of array you find in object oriented languages like C++. Like arrays, they can store multiple data values. However, unlike arrays, they cannot store primitive data types.
What is a vector in C++ STL?
Vector in C++ STL. Vectors are same as dynamic arrays with the ability to resize itself automatically when an element is inserted or deleted, with their storage being handled automatically by the container. Vector elements are placed in contiguous storage so that they can be accessed and traversed using iterators.
How do I create a vector in C?
However, C is not an object oriented language, so creating a true vector is virtually impossible. We can, however, create a pseudo vector in C in a couple of different ways. You can use a data structure to hold a vector. You will want to create your own data type (vector type) by using the typedef keyword: Empower your team. Lead the industry.
How does a pointer step through a vector in C++?
However, as the loop progresses, the pointer is made to step through the vector elements by using the pointer-increment operation. As the pointer always points to the vector element to be processed, the value of the current element is obtained by dereferencing the pointer, as *pa.
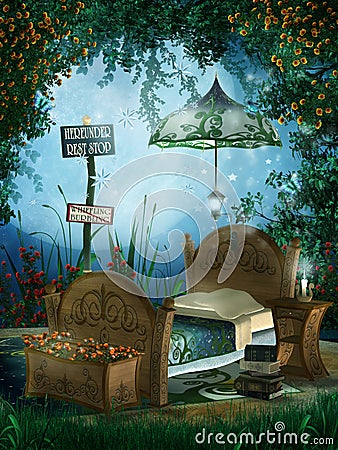
What is a vector in C program?
In C++, vectors are used to store elements of similar data types. However, unlike arrays, the size of a vector can grow dynamically. That is, we can change the size of the vector during the execution of a program as per our requirements. Vectors are part of the C++ Standard Template Library.
What is vector data type in C?
Vector data types are defined by the type name (char, uchar, ushort, int, uint, float, long, and ulong) followed by a literal value n which corresponds to the number of elements in the vector. These data types work with standard C operators, such as +, -, and *.
Is vector same as array in C?
Vector is template class and is C++ only construct whereas arrays are built-in language construct and present in both C and C++. Vector are implemented as dynamic arrays with list interface whereas arrays can be implemented as statically or dynamically with primitive data type interface.
Is vector an array?
Vector is a sequential container to store elements and not index based. Array stores a fixed-size sequential collection of elements of the same type and it is index based. Vector is dynamic in nature so, size increases with insertion of elements. As array is fixed size, once initialized can't be resized.
Can you use vector in C?
Vectors are a modern programming concept, which, unfortunately, aren't built into the standard C library. Vectors are same as dynamic arrays with the ability to resize itself automatically when an element is inserted or deleted, with their storage being handled automatically by the container.
What is an example of vector data?
Vector data is represented as a collection of simple geometric objects such as points, lines, polygons, arcs, circles, etc. For example, a city may be represented by a point, a road may be represented by a collection of lines, and a state may be represented as a polygon.
Why are arrays called vectors?
We can think of a vector as a list that has one dimension. It is a row of data. An array is a list that is arranged in multiple dimensions. A two-dimensional array is a vector of vectors that are all of the same length.
What is vector vs array?
A Vector is a sequential-based container whereas an array is a data structure that stores a fixed number of elements (elements should of the same type) in sequential order. Vectors are sometimes also known as dynamic arrays.
What is a vector in programming?
A vector, in programming, is a type of array that is one dimensional. Vectors are a logical element in programming languages that are used for storing a sequence of data elements of the same basic type. Members of a vector are called components.
Why is it called vector?
The term vector comes from engineering/physics. Vectors represent 2 and 3 dimensional lines that have a direction.
What is difference between vector and list?
In vector, each element only requires the space for itself only. In list, each element requires extra space for the node which holds the element, including pointers to the next and previous elements in the list. Insertion at the end requires constant time but insertion elsewhere is costly.
Which is faster vector or array?
A std::vector can never be faster than an array, as it has (a pointer to the first element of) an array as one of its data members. But the difference in run-time speed is slim and absent in any non-trivial program. One reason for this myth to persist, are examples that compare raw arrays with mis-used std::vectors.
What is a vector data structure?
Vector is a data structure, used to store spatial data. Vector data is comprised of lines or arcs, defined by beginning and end points, which meet at nodes. The locations of these nodes and the topological structure are usually stored explicitly.
What are the types of raster data?
raster data types. There are three types of raster data that can be stored in a geodatabase: raster datasets, raster catalogs, and raster as attributes. Raster datasets are single images that are stored in the database.
What is the data type for each item in the vector?
A vector is a collection of elements that are most commonly of mode character , logical , integer or numeric .
Which of the following items are considered vector data?
Vector data is split into three types: point, line (or arc), and polygon data.
Is vector ordered in C++?
No vectors are not ordered in C++. Vector elements are placed in adjacent storage so that they can be accessed and travel across using iterators. In vectors, data is inserted at the end of it. Inserting an element at the end takes differential time, as sometimes there may be a need of extending the vector. Removing the last element takes only constant time because no resizing occurs. Inserting and erasing at the beginning or in the middle of the vector is linear in time.
What is vector in C++?
Vectors in C++ are the dynamic arrays that are used to store data. Unlike arrays, which are used to store sequential data and are static in nature, Vectors provide more flexibility to the program. Vectors can resize itself automatically when an element is inserted or deleted depending on the need of the task to be executed.
What is swap function?
swap (): swap () function is used to swap the contents of one vector with another vector of the same type. Sizes may differ.
Why does erasing an element at the end of a vector take differential time?
Removing the last element takes only constant time because no resizing occurs. Inserting and erasing at the beginning or in the middle of the vector is linear in time.
What does the end function do in a vector?
end (): The end () function returns an iterator pointing to the last element in the vector.
What does max_size do?
max_size (): The max_size () function returns the maximum number of elements that the vector can hold.
What does assign mean in a vector?
assign (): It assigns a new value to the vector elements by replacing old ones.
What is pointer pa used for?
One such alternative is given below in which pointer pa is used as a control variable of the for loop and the pointers are incremented in element access expressions using the postfix increment operator.
What is the address of the ith array element in the scanf statement?
This program segment reads the first n elements of vector a from the keyboard. Observe that the . expression pa+i, which is the address of the ith array element, is used in the scanf statement in place of &a [i ]. Alternatively, we can write this code using pointer pa to control the loop as shown below.
How to use pa+k?
Consider a pointer pa that points to vector element a [ i ]. When an integer k is added to this pointer, we get a pointer that points to an element k elements past the current pointer position, i.e., pa+ k points to element a [i+k]. We can access this element, without modifying the current pointer position, using the expression * (pa+k). Similarly, pa – k points to an element k elements before the current pointer position, i.e., a [i-k].
What are the three expressions of pointer dereference?
The pointer dereference and increment/decrement operations can be combined to obtain three expressions: ++*pa, *++pa and *pa++.
How to manipulate vectors?
There is another more convenient and efficient method to manipulate a vector using-a pointer variable in which we make the pointer step through the vector elements. In this method, first initialize a pointer to point to the first element and then set up a loop to process each element as before. However, as the loop progresses, the pointer is made to step through the vector elements by using the pointer-increment operation. As the pointer always points to the vector element to be processed, the value of the current element is obtained by dereferencing the pointer, as *pa.
How does the power of arrays improve?
The power of arrays is enhanced further by having a close correspondence between arrays and pointers. The name of an array of type T is equivalent to a pointer to type T, whose value is the starting address of that array, i. e., the address of element 0. This correspondence between an array name and a pointer allows us to access array elements using pointer notation in addition to the subscript notation. We can write efficient code that runs faster using pointers for array processing.
How to advance a pointer by k array?
We can also advance a pointer by k array elements by using the assignment pa+= k. However, we have to be careful while performing such operations as the resulting pointer may point to an element beyond the existing array elements, in which case, we should not access that element.
Code implementation of the vector in C
First, we need to create a structure that stores the data and also track the stored data. I am also creating here another structure to store the function pointer which points to the vector function (because C does not support the function in a structure like C++).
Example Code of a vector in C
In this example, I am creating a vector of string using the pushback function. After creation the vector I am displaying the stored string with there index. I have also set the string using the index. You should remember that the address of the data should be valid and you have to care about the dangling pointer.
Why do vectors consume more memory?
Therefore, compared to arrays, vectors consume more memory in exchange for the ability to manage storage and grow dynamically in an efficient way. Compared to the other dynamic sequence containers ( deques, lists and forward_lists ), vectors are very efficient accessing its elements (just like arrays) and relatively efficient adding ...
Why do vectors need to be reallocated?
This array may need to be reallocated in order to grow in size when new elements are inserted, which implies allocating a new array and moving all elements to it .
What is sequence container?
Elements in sequence containers are ordered in a strict linear sequence. Individual elements are accessed by their position in this sequence.
What is vector programming?
Vectors are a modern programming concept, which, unfortunately, aren’t built into the standard C library. They are found in C++, which is an object oriented programming extension of C. Essentially, vectors replace arrays in C++. In this tutorial, we’re going to give you an overview of how you can replicate vectors in C.
What is vector in C++?
A vector is a type of array you find in object oriented languages like C++. Like arrays, they can store multiple data values. However, unlike arrays, they cannot store primitive data types. They only store object references – they point to the objects that contain the data instead of storing the objects themselves.
Is vectors object oriented in C?
You can see why vectors sound more useful than arrays, and why they might be useful in C. However, C is not an object oriented language, so creating a true vector is virtually impossible.
Can you use a data structure to hold a vector?
You can use a data structure to hold a vector. You will want to create your own data type (vector type) by using the typedef keyword:
Do you have to declare the size of a vector?
Also, you don’t have to declare the size of a vector. It will grow, or shrink, as you fill it with object references or remove them. Vectors also have several safety features that make them easier to use than arrays, and chances of your program crashing are much less.
Is C a complete language?
However, C is a complete language in itself and it is still used everywhere, even more than four decades after it was created. C does have some advantages over its successors. It is a low level language, which means that it runs close to machine level languages. This makes it faster to compile and run than C++ or other languages.
Is C a good programming language?
However, C is a complete language in itself and it is still used everywhere, even more than four decades after it was created. C does have some advantages over its successors. It is a low level language, which means that it runs close to machine level languages. This makes it faster to compile and run than C++ or other languages. C compilers are also widely available, and can be used on almost any machine imagine.
What functions can initialize vector iterators?
We can initialize vector iterators using the begin () and end () functions.
What is vector iterator?
Vector iterators are used to point to the memory address of a vector element. In some ways, they act like pointers in C++.
Why is the size of a vector not specified?
Notice that we have not specified the size of the vector during the declaration. This is because the size of a vector can grow dynamically so it is not necessary to define it.
What is vector header in C++?
In C++, the vector header file provides various functions that can be used to perform different operations on a vector.
What is vector class?
The vector class provides various methods to perform different operations on vectors. We will look at some commonly used vector operations in this tutorial:
What does the end function mean in a vector?
The end () function points to the theoretical element that comes after the final element of the vector. For example,
What does push_back do in vector?
Here, the push_back () function adds elements 6 and 7 to the vector.
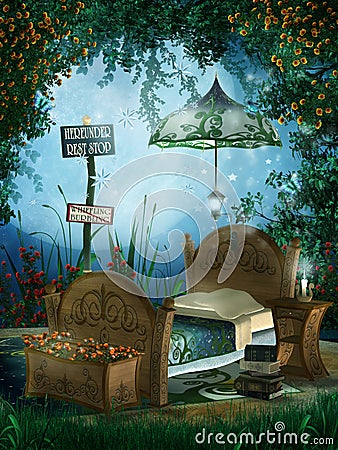