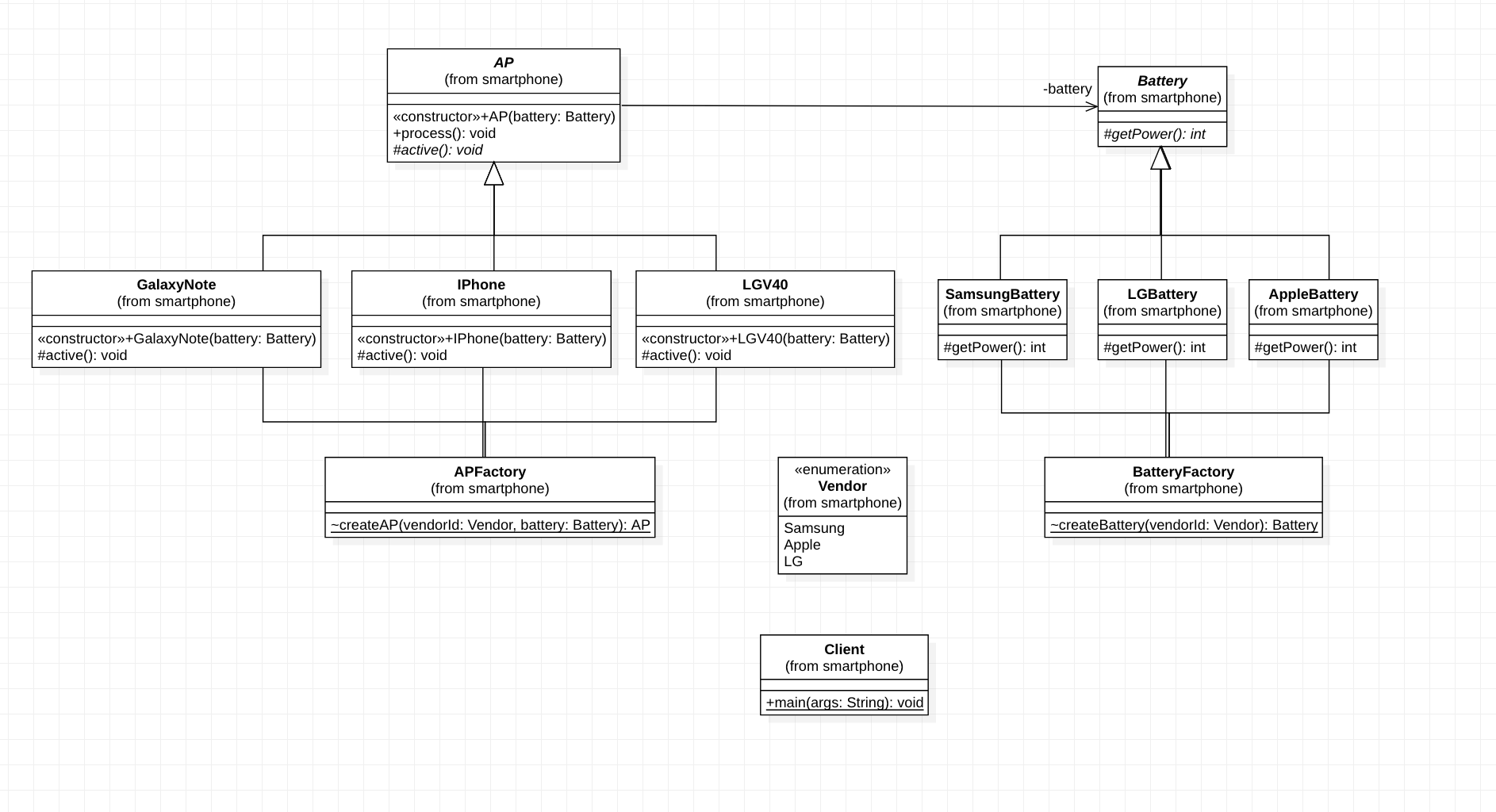
When to use the abstract factory pattern?
When to use Abstract Factory Design Method?
- When the client doesn’t need to know how the final product is actually created.
- When the system demands the creation of a library of products, for which only interfaces are required, not the implementation.
- When the system needs to be configured with one of a multiple-family of objects.
How to use factory design pattern?
When to use the Factory Design Pattern in real-time applications?
- The Object needs to be extended to the subclasses
- Classes don’t know what exact sub-classes it has to create
- The Product implementation going to change over time and the Client remains unchanged
What is abstract factory method?
abstract factory is one level higher in abstraction than factory method. factory method abstracts the way objects are created , while abstract factory also abstracts the way factories are created which in turn abstracts the way objects are created.
What is an abstract factory?
Abstract Factory patterns work around a super-factory which creates other factories. This factory is also called as factory of factories. This type of design pattern comes under creational pattern as this pattern provides one of the best ways to create an object.
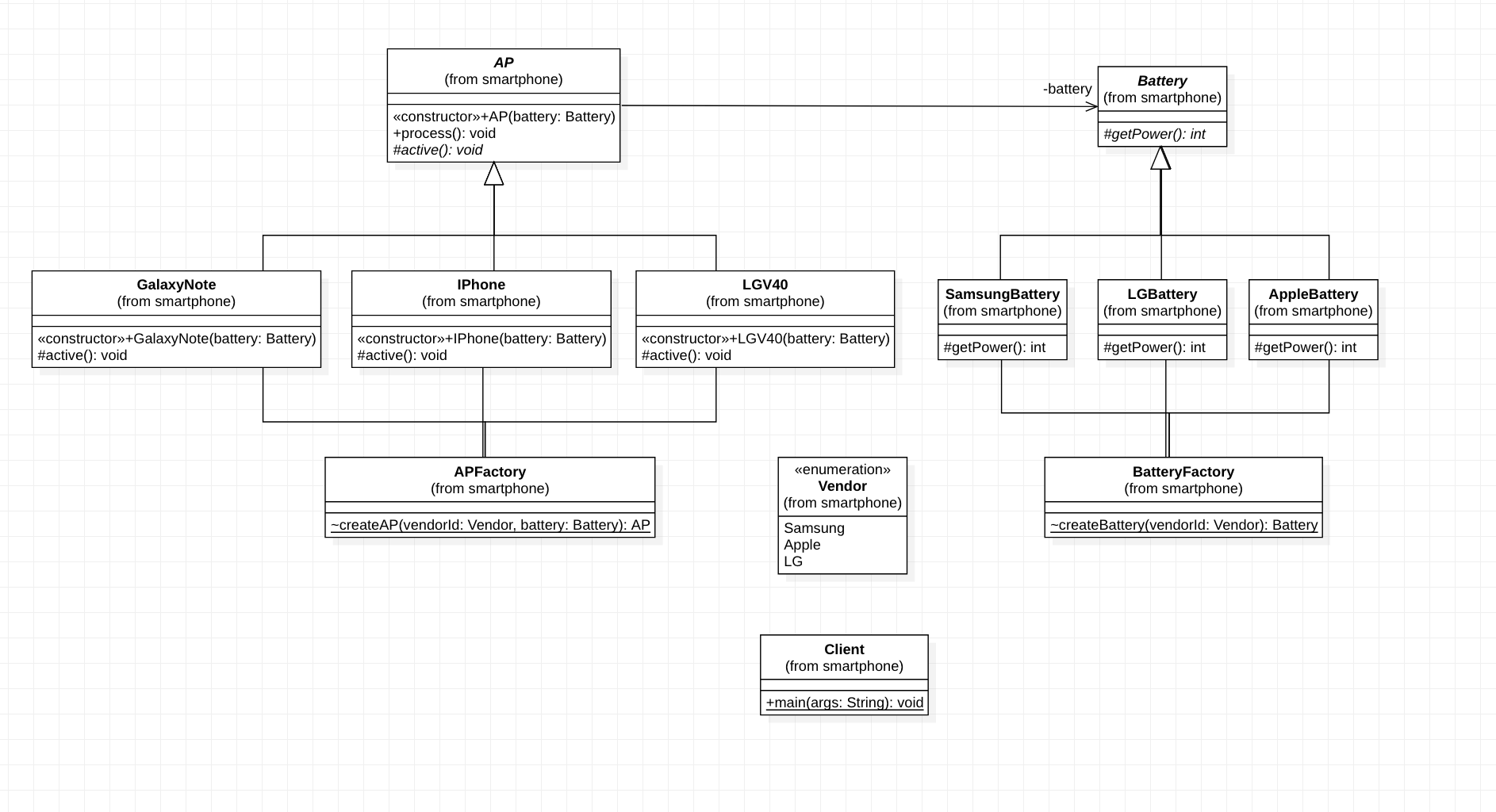
What is meant by Abstract Factory design pattern?
Abstract Factory Pattern says that just define an interface or abstract class for creating families of related (or dependent) objects but without specifying their concrete sub-classes. That means Abstract Factory lets a class returns a factory of classes.
What is the difference between Abstract Factory and Factory Pattern?
Factory Method pattern is responsible for creating products that belong to one family, while Abstract Factory pattern deals with multiple families of products. Factory Method uses interfaces and abstract classes to decouple the client from the generator class and the resulting products.
When would you use the Abstract Factory pattern?
When to Use Abstract Factory Pattern: The client is independent of how we create and compose the objects in the system. The system consists of multiple families of objects, and these families are designed to be used together. We need a run-time value to construct a particular dependency.
Can we use abstract class in factory design pattern?
Short answer: Yes. Actually, since Java 8 you can provide default implementations for the methods in an interface and the only differences to an abstract class are constructors (which you don't use anyway apparently) and fields which should be private, so there's very little difference.
What are the types of factory pattern?
We also discussed their four different types, i.e., Singleton, Factory Method, Abstract Factory and Builder Pattern, their advantages, examples and when should we use them.
Why do we use factory design pattern?
Advantage of Factory Design Pattern Factory Method Pattern allows the sub-classes to choose the type of objects to create. It promotes the loose-coupling by eliminating the need to bind application-specific classes into the code.
What is the purpose of Abstract Factory?
The purpose of the Abstract Factory is to provide an interface for creating families of related objects, without specifying concrete classes. This pattern is found in the sheet metal stamping equipment used in the manufacture of Japanese automobiles.
What problem does Abstract Factory solve?
The Abstract Factory design pattern solves problems like: How can an application be independent of how its objects are created? How can a class be independent of how the objects it requires are created? How can families of related or dependent objects be created?
What is abstract design?
What is meant by an 'abstract design'? Abstract design uses the language of shape, form, colour and gestural marks to create work that does not attempt to depict visual reality accurately. Indeed, 'abstract' itself means 'to withdraw or separate something from something else'.
What is factory design pattern?
The factory method is a creational design pattern, i.e., related to object creation. In the Factory pattern, we create objects without exposing the creation logic to the client and the client uses the same common interface to create a new type of object.
What is Abstract Factory pattern Mcq?
The Abstract Factory pattern helps you control the classes of objects that an application creates. Because a factory encapsulates the responsibility and the process of creating product objects, it isolates clients from implementation classes. Clients manipulate instances through their abstract interfaces.
How do you implement a factory pattern?
Design Pattern - Factory PatternImplementation. ... Create an interface. ... Create concrete classes implementing the same interface. ... Create a Factory to generate object of concrete class based on given information. ... Use the Factory to get object of concrete class by passing an information such as type. ... Verify the output.
What is the difference between factory and builder pattern?
A Factory Design Pattern is used when the entire object can be easily created and object is not very complex. Whereas Builder Pattern is used when the construction process of a complete object is very complex.
What is the difference between the Factory Method and a simple factory?
Use the Factory method when you want to make a Framework where you want to take a control from the creation of the Object to the management of that Object. That's unlike the Simple factory, where you only care about the creation of a product, not how to create and manage it.
What is the difference between Singleton design pattern and factory design pattern?
The Singleton pattern ensures that only one instance of the class exists and typically provides a well-known, i.e., global point for accessing it. The Factory pattern defines an interface for creating objects (no limitation on how many) and usually abstracts the control of which class to instantiate.
What is abstract class vs interface?
The Abstract class and Interface both are used to have abstraction. An abstract class contains an abstract keyword on the declaration whereas an Interface is a sketch that is used to implement a class.
What is an abstract factory?
In simple words we can say, the Abstract Factory is a super factory that creates other factories. This Abstract Factory is also called the Factory of Factories.
What are the two subclasses of the Animal Factory?
So, here we have two factories i.e. Land Animal Factory and Sea Animal Factory. Please have a look at the following diagram. The Land Animal Factory and Sea Animal Factory are the subclasses of Animal Factory. Here, the Animal Factory is nothing but your Abstract Factory.
What class will implement the speak method?
The Cat class will implement that Speak method and return Meow. Similarly, the Lion class will implement the Speak () method and will return Roar and in the say the Dog class will implement the Speak () method and return Bark bark. The Cat, Lion, and Dog are living in the Land, so they belong to the Land Animal group.
What is the essence of the Abstract Factory Pattern?from en.wikipedia.org
The essence of the Abstract Factory Pattern is to "Provide an interface for creating families of related or dependent objects without specifying their concrete classes."
Why does the factory object use a hashmap?from oodesign.com
The registration is made from outside of the factory and because the objects are created using reflection the factory is not aware of the objects types.
How does a factory object insulate client code from object creation?from en.wikipedia.org
This insulates client code from object creation by having clients ask a factory object to create an object of the desired abstract type and to return an abstract pointer to the object.
What is the difference between abstract and factory?from geeksforgeeks.org
The factory method is just a method, it can be overridden in a subclass, whereas the abstract factory is an object that has multiple factory methods on it.
What is factory instantiation?from oodesign.com
The factory instantiates a new concrete product and then returns to the client the newly created product (casted to abstract product class).
What is product in concrete?from geeksforgeeks.org
Product: Defines a product object to be created by the corresponding concrete factory and implements the AbstractProduct interface.
When you design an application, do you need a factory?from oodesign.com
When you design an application just think if you really need it a factory to create objects. Maybe using it will bring unnecessary complexity in your application. If you have many objects of the same base type and you manipulate them mostly casted to abstract types, then you need a factory. If you're code should have a lot of code like the following, you should reconsider it:
What is an abstract pattern?
Abstract Factory design pattern is a part of Creational pattern. Abstract Factory pattern is almost similar to Factory Pattern and is considered to have a extra layer of abstraction over factory pattern.
What is concrete factory?
ConcreteFactory : Implements the operations declared in the Abstract Factory to create concrete product objects.
Why is it difficult to support new kind of products?
That’s because the Abstract Factory interface fixes the set of products that can be created.
What is abstract base class?
Classes that contain at least one pure virtual function are called as abstract base classes.
What is a product button?
The products (menu and button) is platform dependant that is on Windows or Linux. A user (Client) will use the products. There are two options at this point: The user (Client) has take care to identify the platform the product is supported and use accordingly to avoid any unexpected errors.
Can you change the class of a concrete factory?
Exchanging Product Families easily: The class of a concrete factory appears only once in an application, that is where it’s instantiated. This makes it easy to change the concrete factory an application uses.
What is abstract factory?
Abstract Factory is a creational design pattern, which solves the problem of creating entire product families without specifying their concrete classes. Abstract Factory defines an interface for creating all distinct products but leaves the actual product creation to concrete factory classes.
Why do libraries use factory?
Identification: The pattern is easy to recognize by methods, which return a factory object. Then, the factory is used for creating specific sub-components.
Does client code work with factory?
Since a factory corresponds to a single product variant, all its products will be compatible. Client code works with factories and products only through their abstract interfaces. This lets the client code work with any product variants, created by the factory object.
Preface
I came across the Abstract Factory Design Pattern a few weeks ago, after days of study I think I managed to grasp the concept of this design pattern. So I decided to share some learning experiences about it. First, I will show example code of “before and after” applying the design pattern.
Without Abstract Factory
I’m using furniture as an example. The furniture is categorized by design, which are Classic, Contemporary, and Scandinavian. The furniture itself has 3 different objects, which are Cabinet, Chair and Dining Table, so there will be 9 different combinations, as the table below shows.
Abstract Factory with Interface
First, for the furniture itself. Since all the furniture share a common method, I create an Interface named IFurniture to be implemented by all furniture classes.
Abstract Factory with Abstract Class, Inheritance
Same Abstract Factory Design Pattern can be achieved by using abstract class inheritance methods.
Nested 3 Level Abstract Factory?
I read a few articles and one pdf book about the Abstract Factory for the past few days, but I never found any example with more than 2 levels. So, I decided to add one more level, try to create something like the ‘Abstract Factory of the Abstract Factory’.
Conclusion
Abstract Factory is one of the popular design patterns. I hope you enjoy my writing and can have a better understanding of this pattern. Again, please feel free to let me know if I did something wrong in this article.
What is the essence of the Abstract Factory Pattern?
The essence of the Abstract Factory Pattern is to "Provide an interface for creating families of related or dependent objects without specifying their concrete classes."
What is Abstract Factory?
The Abstract Factory design pattern is one of the twenty-three well-known GoF design patterns that describe how to solve recurring design problems to design flexible and reusable object-oriented software, that is, objects that are easier to implement, change, test, and reuse.
What is a class diagram example?
Class diagram example The method createButton on the GUIFactory interface returns objects of type Button. What implementation of Button is returned depends on which implementation of GUIFactory is handling the method call.
How does a factory object insulate client code from object creation?
This insulates client code from object creation by having clients ask a factory object to create an object of the desired abstract type and to return an abstract pointer to the object.
Why is creating objects in a class inflexible?
Creating objects directly within the class that requires the objects is inflexible because it commits the class to particular objects and makes it impossible to change the instantiation later independently from (without having to change) the class. It stops the class from being reusable if other objects are required, and it makes the class hard to test because real objects cannot be replaced with mock objects.
What is factory in programming?
A factory is the location of a concrete class in the code at which objects are constructed. The intent in employing the pattern is to insulate the creation of objects from their usage and to create families of related objects without having to depend on their concrete classes. This allows for new derived types to be introduced with no change to ...
Can a class be configured with a factory object?
This makes a class independent of how its objects are created (which concrete classes are instantiated). A class can be configured with a factory object, which it uses to create objects, and even more, the factory object can be exchanged at run-time. See also: § UML diagram.
What is Abstract Factory Pattern?
Abstract Factory patterns act a super-factory which creates other factories. This pattern is also called a Factory of factories. In Abstract Factory pattern an interface is responsible for creating a set of related objects, or dependent objects without specifying their concrete classes.
What pattern does Abstract Factory use?
Internally, Abstract Factory use Factory design pattern for creating objects. But it can also use Builder design pattern and prototype design pattern for creating objects. It completely depends upon your implementation for creating objects.
How does a prototype use the factory?
Prototype use the factory for building an object by copying an existing object. Read More Articles Related to Design Pattern.
What is a factory design pattern?
As per the definition of Factory Design Pattern, the Factory Design Pattern create an object without exposing the object creation logic to the client and the client refers to the newly created object using a common interface.
What is a factory in Lehman's perspective?
From Lehman’s point of view, we can say that a factory is a place where products are created. In order words, we can say that it is a centralized place for creating products. Later, based on the order received, the appropriate product is delivered by the factory. For example, a car factory can produce different types of cars. If you are ordering a car to the car factory, then based on your requirements or specifications, the factory will create the appropriate car and then delivered that car to you.
What is a factory in Gang of Four?
According to Gang of Four, the Factory Design Pattern states that “A factory is an object which is used for creating other objects”. In technical terms, we can say that a factory is a class with a method. That method will create and return different types of objects based on the input parameter, it received.
Can a car factory produce a car?
For example, a car factory can produce different types of cars. If you are ordering a car to the car factory, then based on your requirements or specifications, the factory will create the appropriate car and then delivered that car to you. The same thing also happens in the factory design pattern.
Can you specify the exact class name in C#?
It would not be a good programming approach to specify the exact class name while creating the objects by the client which leads to tight coupling between the client and the product. To overcome this problem, we need to use the Factory Design Pattern in C#. This design pattern provides the client with a simple mechanism to create the object. So, we need to use the Factory Design Pattern in C# when
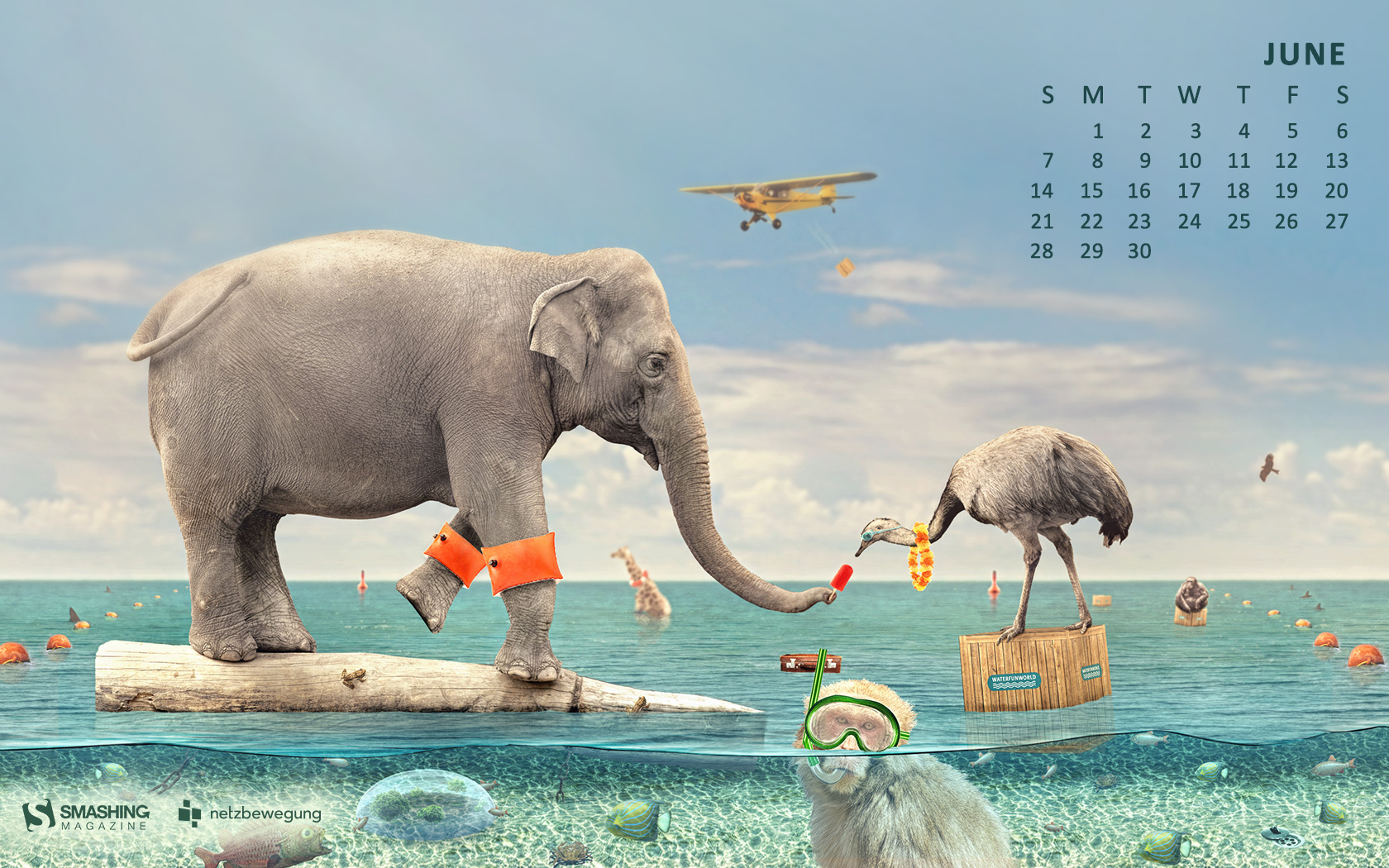