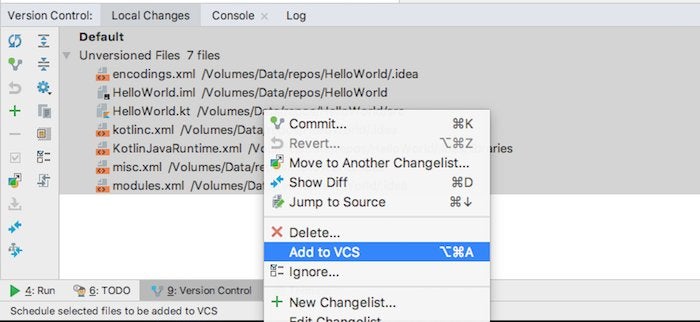
What is apply function in Kotlin?
In kotlin language, it has a lot of functions, classes, and other keywords for utilising the kotlin logic in the application. Like that, apply is one of the scope functions that can be used to configure the object once initialized and returns the object itself.
What is the difference between also and let in Kotlin?
The also expression returns the data class object whereas the let expression returns nothing (Unit) as we didn’t specify anything explicitly. Kotlin apply is an extension function on a type. It runs on the object reference (also known as receiver) into the expression and returns the object reference on completion.
What are scope functions in Kotlin?
One special collection of relevant functions can be described as "scope functions" and they are part of the Kotlin standard library: let, run, also, apply and with. You probably already heard about them and it's also likely that you even used some of them yet.
What is Kotlin good for?
Kotlin brings a touch of succinctness in places, where even programmers new to Java, may find code unnecessarily over-bloated. How many times have you had to do the same thing over and over again: Having an object, you want to modify some of its properties and simply return the same object.
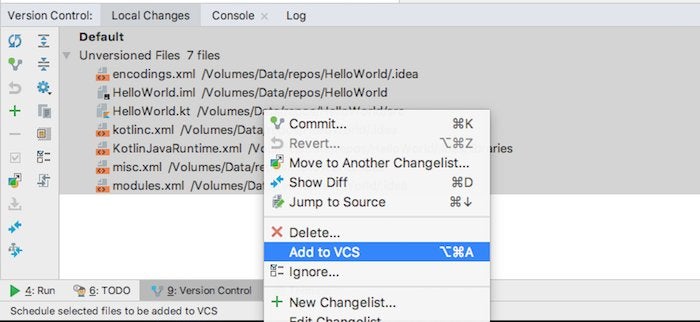
Why apply is used in Kotlin?
apply accepts an instance as the receiver while with requires an instance to be passed as an argument. In both cases, the instance will become this within a block. apply returns the receiver and with returns a result of the last expression within its block.
What does .also do in Kotlin?
also is an extension function to Template class which takes a lambda as a parameter, apply contract on it, execute the lambda function within the scope of calling object and ultimately return the same calling object of Template class itself.
What does ?: Mean in Kotlin?
In certain computer programming languages, the Elvis operator ?: is a binary operator that returns its first operand if that operand is true , and otherwise evaluates and returns its second operand.
What is run block in Kotlin?
run(block: T.() -> R): R. Calls the specified function block with this value as its receiver and returns its result. For detailed usage information see the documentation for scope functions.
What is difference between apply and run in Kotlin?
Kotlin apply vs with with runs without an object(receiver) whereas apply needs one. apply runs on the object reference, whereas with just passes it as an argument. The last expression of with function returns a result.
What is by lazy in Kotlin?
Lazy is mainly used when you want to access some read-only property because the same object is accessed throughout. That's it for this blog.
What is safe call in Kotlin?
Safe Call Operator (?.) Kotlin has a safe call operator (?.) to handle null references. This operator executes any action only when the reference has a non-null value. Otherwise, it returns a null value. The safe call operator combines a null check along with a method call in a single expression.
What is null in Kotlin?
Kotlin type system has distinguish two types of references that can hold null (nullable references) and those that can not (non-null references). A variable of type String can not hold null. If we try to assign null to the variable, it gives compiler error.
What are coroutines in Kotlin?
A coroutine is a concurrency design pattern that you can use on Android to simplify code that executes asynchronously. Coroutines were added to Kotlin in version 1.3 and are based on established concepts from other languages.
What is lambda in Kotlin?
Lambda expression is a simplified representation of a function. It can be passed as a parameter, stored in a variable or even returned as a value. Note: If you are new to Android app development or just getting started, you should get a head start from Kotlin for Android: An Introduction.
What is sealed class in Kotlin?
Here, we have a data class success object which contains the success Data and a data class Error object which contains the state of the error. This way, Sealed classes help us to write clean and concise code! That's all the information about Sealed classes and their usage in Kotlin.
What is inline fun in Kotlin?
An Inline function is a kind of function that is declared with the keyword "inline" just before the function declaration. Once a function is declared inline, the compiler does not allocate any memory for this function, instead the compiler copies the piece of code virtually at the calling place at runtime.
What is a Kotlin companion object?
Answer: Companion object is not a Singleton object or Pattern in Kotlin – It's primarily used to define class level variables and methods called static variables. This is common across all instances of the class.
How do you use Elvis operator Kotlin?
Elvis operator (?:) is used to return the not null value even the conditional expression is null....This can be used for checking functional arguments:funfunctionName(node: Node): String? {val parent = node. getParent() ?: return null.val name = node. getName() ?: throw IllegalArgumentException("name expected")// ...}
How do I create a data class in Kotlin?
There are following conditions for a Kotlin class to be defined as a Data Class: The primary constructor needs to have at least one parameter. All primary constructor parameters need to be marked as val or var. Data classes cannot be abstract, open, sealed, or inner.
Kotlin :: apply
In Kotlin, apply is an extension function on a particular type and sets its scope to object on which apply is invoked. Apply runs on the object reference into the expression and also returns the object reference on completion.
Kotlin :: with
Like apply, with is also used to change properties of an instance. But here we don’t require a object reference to run, i.e. : we don’t need a dot operator for reference.
What is apply in lambdas?
apply is recommended for lambdas that mainly operate on the object members or call its functions or assign properties. In short, it is mostly used for object configurations.
Can a template be called on any object?
Since its an extension function to the Template class, it can be called on any object.
Can you use a named parameter in apply?
Please note that we can even point to the calling object by this but we can't use a named parameter in apply .
What is a scope function in Kotlin?
The Kotlin standard library contains several functions whose sole purpose is to execute a block of code within the context of an object. When you call such a function on an object with a lambda expression provided, it forms a temporary scope. In this scope, you can access the object without its name. Such functions are called scope functions. There are five of them: let, run, with, apply, and also.
What is "also" good for?
also is good for performing some actions that take the context object as an argument. Use also for actions that need a reference rather to the object than to its properties and functions, or when you don't want to shadow this reference from an outer scope.
What is the return value of a context object?
The context object is available as an argument ( it ). The return value is the lambda result.
What is let used for?
let is often used for executing a code block only with non-null values. To perform actions on a non-null object, use the safe call operator ?. on it and call let with the actions in its lambda.
When you pass a context object as an argument, can you provide a custom name for the context object inside the?
Additionally, when you pass the context object as an argument, you can provide a custom name for the context object inside the scope.
Can you continue a call chain on the same object after the return value of apply?
The return value of apply and also is the context object itself. Hence, they can be included into call chains as side steps: you can continue chaining function calls on the same object after them.
What does apply do in code?
By definition, apply accepts a function, and sets its scope to that of the object on which apply has been invoked. This means that no explicit reference to the object is needed. Apply () can do much more than simply setting properties of course. It is a transformation function, capable of evaluating complex logic before returning. At the end, the function simply returns the same object (with the added changes), so one can keep using it on the same line of code.
Can you chain an object in Java?
Having an object, you want to modify some of its properties and simply return the same object. In Java, you can't do this in a single chained fashion. In Kotlin, you can squeeze the above method to the following single line of code:
Does Kotlin support Python?
Kotlin supports named parameters, known from other languages, such as Python and Scala. In this case, they make life much easier, as all you need to do, is specify the name of the particular property (or properties) you want to modify by copying. At the end you can combine the power and succinctness of both apply () and copy () ...
Is applying an object thread safe?
Please, remember that using apply on an object is NOT a thread-safe operation, and mutates the state of the object. If you want to retain the original object and return an immutable copy of it, you can use the function copy (), provided in all instances of data classes.
Is it bad to use getters and setters in Kotlin?
Note: Please, note as well the direct use of properties instead of explicitly calling getters and setters. This is not a bad practice, since Kotlin implicitly invokes provided getters and setters of a property, when working with the property (similar to C#).
What is the common case for apply?
The common case for apply is the object configuration.
What is also function?
The also function takes a lambda in which you refer to the object you called the function on (receiver T) with either it (implicit name) or a custom name.
Can you use one or the other code?
So, depending on how well you code should be readable you can always use one or the other.
When to use apply or run?
I'm not sure there can be some strict rules on which function to choose. Usually you use apply when you need to do something with an object and return it. And when you need to perform some operations on an object and return some other object you can use either with or run. I prefer run because it's more readable in my opinion but it's a matter of taste.
What does apply accept?
apply accepts an instance as the receiver while with requires an instance to be passed as an argument. In both cases the instance will become this within a block.
What does "with" and "apply" mean?
With and Apply both accept an object as a receiver in whatever manner they are passed.
What is the application function?
The apply function is another scope function that was added because the community asked for it. Its main use case is the initialization of objects, similar to what also does. The difference will be shown next. Let's inspect its signature first:
What is the ultimate use case for apply?
The ultimate use case for apply is object initialization. The community actually asked for this function in relatively late stage of the language. You can find the corresponding feature request here.
What is inline fun repeat?
inline fun repeat(times: Int, action: (Int) -> Unit ) As you can see, repeat takes two arguments: An ordinary integer times and also another function of type (Int) -> Unit. According to the previously depicted definition, repeat is a higher-order function since it "takes one or more functions as arguments".
Is a function a class in Kotlin?
In Kotlin, functions are as important as integers or strings. Functions can exist on the same level as classes, may be assigned to variables and can also be passed to/returned from other functions. Kotlin makes functions "first-class citizens" of the language, which Wikipedia describes as follows:
