What is asyncstorage and why should you use it?
Asynchronous means that each of its methods returns an object that could be a Promise or an Error. It’s unencrypted, so beware of using it to store sensitive data. Being persistent means it saves the data, even when the user has closed the API or even the device. Finally, key-value pairs are the way data is stored. Why should you use Asyncstorage?
What is async storage in React Native?
Data storage system for React Native. Async Storage is asynchronous, unencrypted, persistent, key-value storage solution for your React Native application. Data storage solution for Android, iOS, Web, MacOS and Windows.
What is the difference between asyncstorage and JavaScript?
On iOS, AsyncStorage is backed by native code that stores small values in a serialized dictionary and larger values in separate files. On Android, AsyncStorage will use either RocksDB or SQLite based on what is available. The AsyncStorage JavaScript code is a facade that provides a clear JavaScript API, real Error objects, and non-multi functions.
What database does asyncstorage use on Android?
On Android, AsyncStorage will use either RocksDB or SQLite based on what is available. The AsyncStorage JavaScript code is a facade that provides a clear JavaScript API, real Error objects, and non-multi functions.
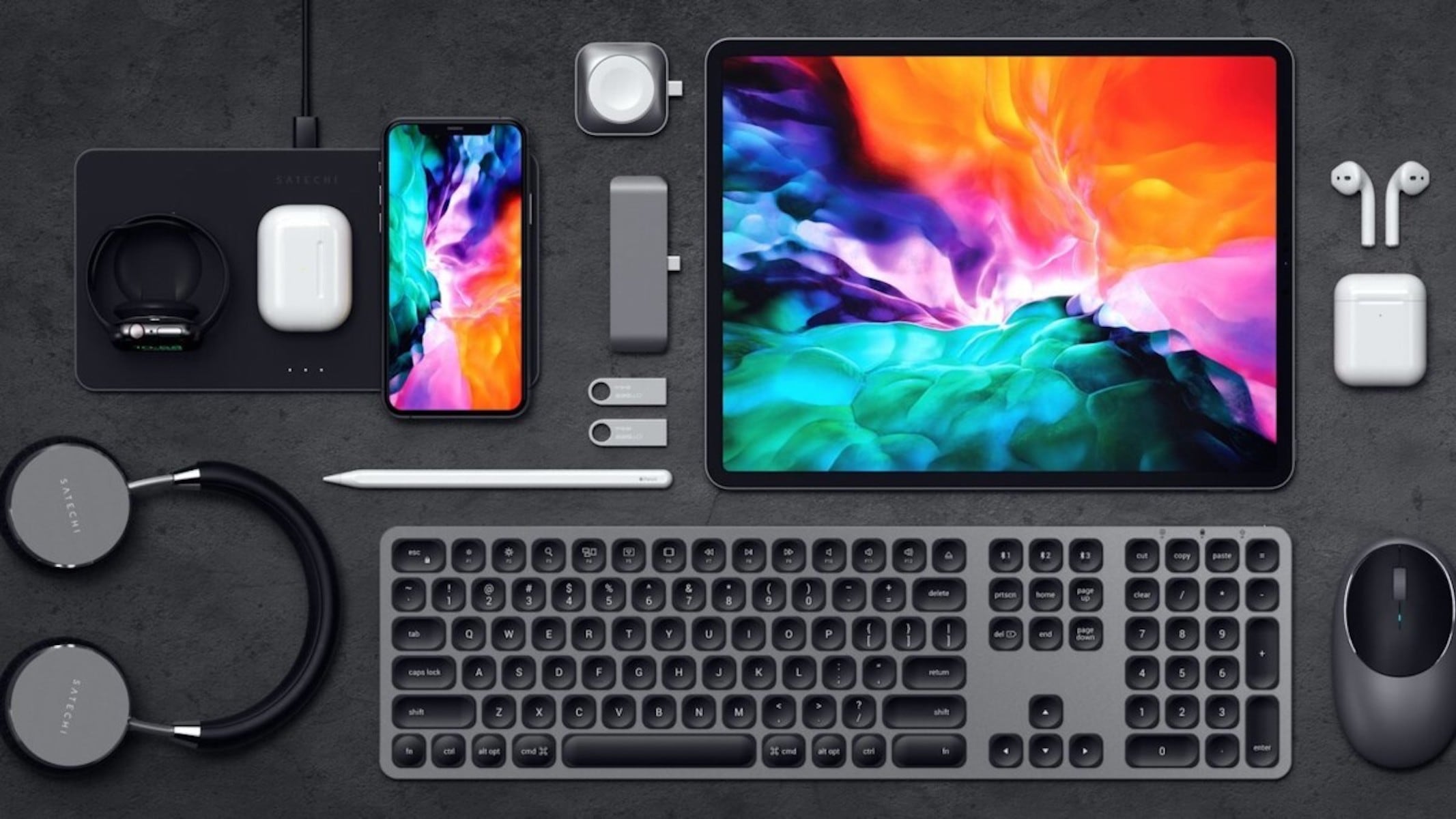
What is async storage used for?
AsyncStorage is a simple, asynchronous, unencrypted by default module that allows you to persist data offline in React Native apps. The persistence of data is done in a key-value storage system. There are numerous scenarios where this module can be beneficial.
Is Async storage safe?
According to official RN docs, AsyncStorage is an asynchronous and unencrypted key-value store. Because it is unencrypted, nothing persisted in AsyncStorage should be considered as secured.
Where does async storage store data?
React Native on Android actually uses SQLite to back up the AsyncStorage API. The database file is /data/data/
What is the difference between async storage and local storage?
AsyncStorage is very similar to localStorage, but built for React Native. It was built to integrate with both iOS and Android to utilize their storage management efficiently.
Is Async storage slow?
AsyncStorage takes about 12x more time to read/write on average compared to localStorage.
What is the safest online storage?
Top 10 Safest Cloud Storage of 2021Microsoft OneDrive.Google Drive.Egnyte Connect.MEGA.Tresorit.SpiderOak.Koofr.Conclusion.More items...•
Is localStorage sync or async?
synchronousLocalStorage is synchronous, each local storage operation you run will be one-at-a-time. For complex applications this is a big no-no as it'll slow down your app's runtime. AsyncLocalStorage is asynchronous, each local async storage operation you run will be multi-at-a-time. It'll speed up your app's runtime.
How does async storage store data?
Async Storage can only store string data, so in order to store object data you need to serialize it first. For data that can be serialized to JSON you can use JSON. stringify() when saving the data and JSON. parse() when loading the data.
How much data we can store in async storage?
6MBMotivation Current Async Storage's size is set to 6MB. Going over this limit causes database or disk is full error. This 6MB limit is a sane limit to protect the user from the app storing too much data in the database.
What is the benefit of async?
Benefits of Asynchronous Programming Improve your application's performance and responsiveness, especially if you have long-running operations that don't require blocking the execution. In this case, you can do other things while you wait for the long-running Task to finish.
Should I use sync or async?
The differences between asynchronous and synchronous include: Async is multi-thread, which means operations or programs can run in parallel. Sync is single-thread, so only one operation or program will run at a time. Async is non-blocking, which means it will send multiple requests to a server.
Why is async important?
Asynchronous programming is a technique that enables your program to start a potentially long-running task and still be able to be responsive to other events while that task runs, rather than having to wait until that task has finished.
Does async storage persist?
AsyncStorage is also asynchronous, meaning that its methods run concurrently with the rest of your code, and it's persistent, meaning that the stored data will always be available globally even if you log out or restart the application.
Should localStorage be async?
LocalStorage is synchronous, each local storage operation you run will be one-at-a-time. For complex applications this is a big no-no as it'll slow down your app's runtime. AsyncLocalStorage is asynchronous, each local async storage operation you run will be multi-at-a-time. It'll speed up your app's runtime.
Is it safe to use session storage?
Both SessionStorage and LocalStorage are vulnerable to XSS attacks. Therefore avoid storing sensitive data in browser storage. It's recommended to use the browser storage when there is, No sensitive data.
Can async storage be used to securely store data locally?
As described on React Native's website: “AsyncStorage is an unencrypted, asynchronous, persistent, key-value storage system that is global to the app.” It's a mouthful. But simply put, it allows you to save data locally on the user's device.
What is AsyncStorage
AsyncStorage is React Native’s API for storing data persistently over the device. It’s a storage system that developers can use and save data in the form of key-value pairs. If you are coming from a web developer background, it resembles a lot like localStorage browser API.
Why use it?
AsyncStorage can prove very helpful when we want to save data that the application would need to use, even when the user has closed it or has even closed the device. It is not a replacement for state data and it should not be confused with that; besides, state data will be erased from memory when the app closes.
Simple usage
It is recommended that you use an abstraction on top of AsyncStorage instead of AsyncStorage directly
Usage with state manager
When we use a state manager within our apps (i.e. Redux — or new React’s context API), it is a really good idea to abstract the related AsyncStorage code within the state manager code, instead of “overloading” the screen’s component code.
What do you think?
What do you think about this article? How and when do you use AsyncStorage API within you apps? Offer your perspective and ideas in the comments section below.
Persistent storage
Async Storage is asynchronous, unencrypted, persistent, key-value storage solution for your React Native application.
Simple API
A handful of tools to simplify your storage flow. Easily save, read, merge and delete data at will!
Installing the community module
React Native version 60+ has lots of its API modules available as separate packages that you can easily install with a package manager such as npm. These packages are managed by community members as well as open-source developers.
Using the AsyncStorage API
Let us start creating the demo application which is going to save a value from the user's input in the storage and fetch the value from the storage. This reading and writing of data are going to be done with the help of AsyncStorage API functions. Open the App.js file and start by importing the following components.
Saving the data
To save the data for the app to read, let us define an asynchronous helper method called saveData. This method is going to be promise-based, thus, you can use the async-await syntax with try-catch block.
Reading the data
When the app restarts, it should be able to read the data persisted in the previous section. For this, create another helper function called readData that is going to be asynchronous.
Clearing all storage
The last API method that the current demo app is going to utilize from the AsyncStorage API is called clear (). This method deletes everything that was previously saved.
Controlling the input
The last two helper functions this demo app requires are onChangeText and onSubmitEditing. These methods are going to be responsible for reading the user input and updating the state variable as well as storing the user's input.
Completing the app
We have defined all the helper functions that are required by the app. Let us complete the return statement by defining the JSX as below:
Where does AsyncStorage store data?
On iOS, AsyncStorage is backed by native code that stores small values in a serialized dictionary and larger values in separate files. On Android, AsyncStorage will use either RocksDB or SQLite based on what is available.
How much data can be store in AsyncStorage in react native?
Current Async Storage’s size is set to 6MB. Going over this limit causes database or disk is full error.
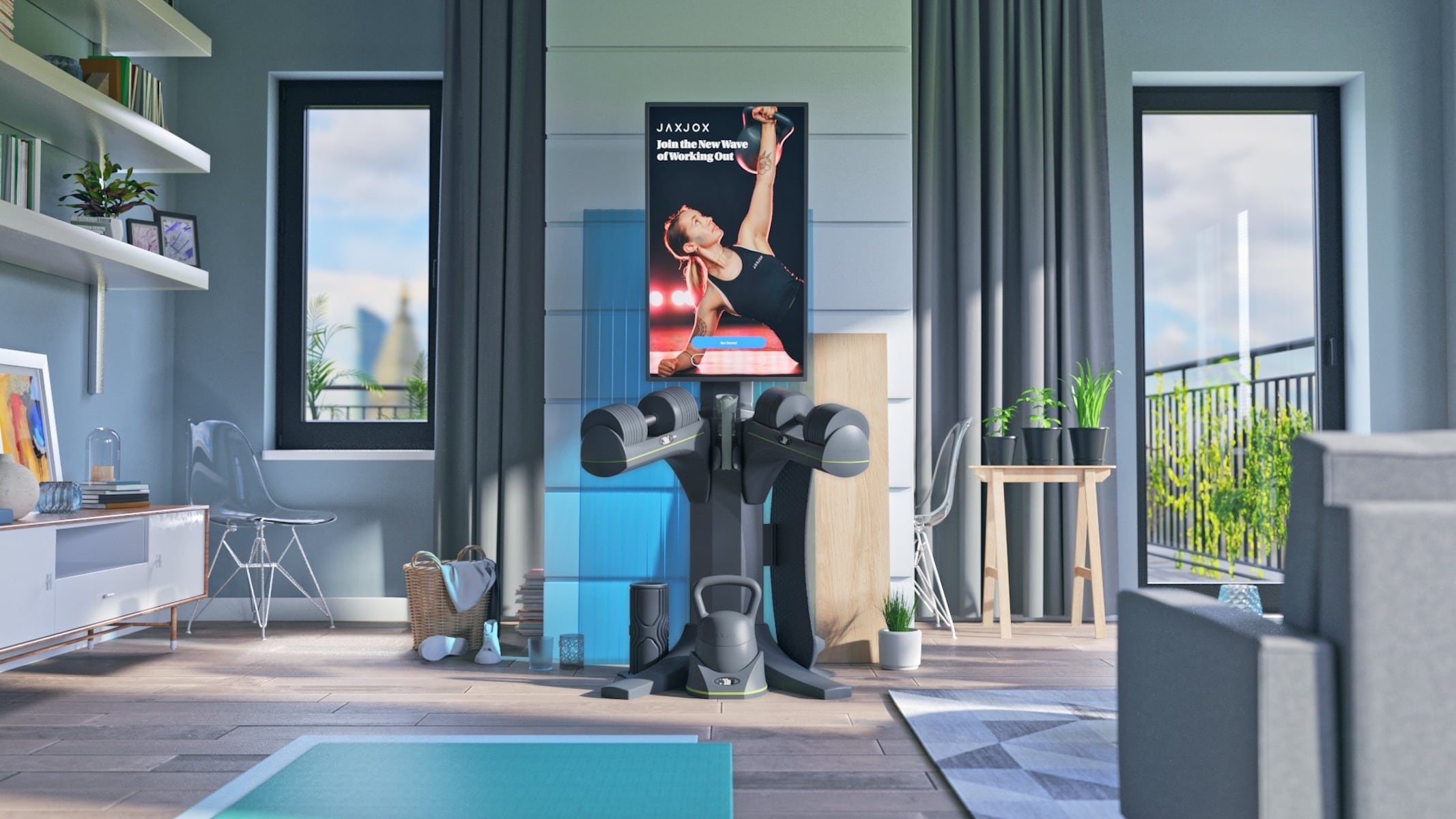
What Is AsyncStorage
Why Use It?
- AsyncStorage can prove very helpful when we want to save data that the application would need to use, even when the user has closed it or has even closed the device. It is not a replacement for state data and it should not be confused with that; besides, state data will be erased from memory when the app closes. A typical example of that, is the data that the app will need to login the use…
Simple Usage
- As per the documentationstates: Here, it suggests not to use AsyncStorage object directly, but instead use the API methods that are designed for this purpose — exactly as we are supposed to do with React’s class components and the state object / methods. The basic actions to perform in AsyncStorage are: 1. set an item, along with its value 2. retrieve an item’s value 3. remove an item
Usage with State Manager
- When we use a state manager within our apps (i.e. Redux — or new React’s context API), it is a really good idea to abstract the related AsyncStorage code within the state manager code, instead of “overloading” the screen’s component code. To understand what this means and how to achieve that, let’s assume our example from before; saving user’s id along with user’s session id …
What Do You think?
- What do you think about this article? How and when do you use AsyncStorage API within you apps? Offer your perspective and ideas in the comments section below.