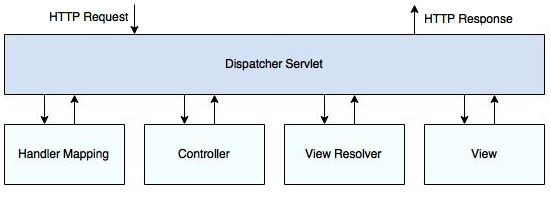
Spring @Configuration annotation is part of the spring core framework. Spring Configuration annotation indicates that the class has @Bean definition methods. So Spring container can process the class and generate Spring Beans to be used in the application.
What is @configuration annotation in Spring Boot?
One of the most important annotations in spring is @Configuration annotation which indicates that the class has @Bean definition methods. So Spring container can process the class and generate Spring Beans to be used in the application. This annotation is part of the spring core framework.
How to enable spring annotation wiring in Spring Framework?
By default, Spring annotation wiring is not turned on in Spring Framework. Therefore, you need to enable it before you can use the Spring annotation-based wiring in the Spring Configuration file. Below is the configuration file in case you want to use annotation in your application:
What is @configuration annotation in Java?
This annotation is used on classes which define beans. @Configuration is an analog for XML configuration file – it is configuration using Java class. Java class annotated with @Configuration is a configuration by itself and will have methods to instantiate and configure the dependencies.
How do I annotate a method in spring?
So consider the following configuration file in case you want to use any annotation in your Spring application. Once <context:annotation-config/> is configured, you can start annotating your code to indicate that Spring should automatically wire values into properties, methods, and constructors.

What is @configuration and @bean in Spring?
Annotating a class with the @Configuration indicates that the class can be used by the Spring IoC container as a source of bean definitions. The @Bean annotation tells Spring that a method annotated with @Bean will return an object that should be registered as a bean in the Spring application context.
What is @configuration annotation used for?
@Configuration annotation indicates that a class declares one or more @Bean methods and may be processed by the Spring container to generate bean definitions and service requests for those beans at runtime.
What is configuration Spring boot?
Spring Boot lets you externalize your configuration so that you can work with the same application code in different environments. You can use a variety of external configuration sources, include Java properties files, YAML files, environment variables, and command-line arguments.
What is difference between @component and @configuration?
The main difference between these annotations is that @ComponentScan scans for Spring components while @EnableAutoConfiguration is used for auto-configuring beans present in the classpath in Spring Boot applications.
Can we use @bean without @configuration?
@Bean methods may also be declared within classes that are not annotated with @Configuration. For example, bean methods may be declared in a @Component class or even in a plain old class. In such cases, a @Bean method will get processed in a so-called 'lite' mode.
Does @configuration create a bean?
It is a method-level annotation. During Java configuration ( @Configuration ), the method is executed and its return value is registered as a bean within a BeanFactory .
What is the difference between @configuration and autoconfiguration?
You can use @EnableAutoConfiguration annotation along with @Configuration annotation. It has two optional elements, exclude : if you want to exclude the auto-configuration of a class. excludeName : if you want to exclude the auto-configuration of a class using fully qualified name of class.
What is difference between @controller and @RestController?
@Controller is used to mark classes as Spring MVC Controller. @RestController annotation is a special controller used in RESTful Web services, and it's the combination of @Controller and @ResponseBody annotation. It is a specialized version of @Component annotation.
What is the difference between @bean and @component?
@Component is a class-level annotation, but @Bean is at the method level, so @Component is only an option when a class's source code is editable. @Bean can always be used, but it's more verbose. @Component is compatible with Spring's auto-detection, but @Bean requires manual class instantiation.
Can we have @bean in @component?
@Willa yes, @Bean can be used in inside a class annotiated with @Component . "You can use the @Bean annotation in a @Configuration-annotated or in a @Component-annotated class." docs.spring.io/spring-framework/docs/current/reference/html/…
Can we use @bean inside @component?
No. It is used to explicitly declare a single bean, rather than letting Spring do it automatically. If any class is annotated with @Component it will be automatically detect by using classpath scan. We should use @bean, if you want specific implementation based on dynamic condition.
What is difference between @bean and @autowired?
@Bean is just for the metadata definition to create the bean(equivalent to tag). @Autowired is to inject the dependancy into a bean(equivalent to ref XML tag/attribute).
Why do we use @configuration annotation in Spring?
One of the most important annotations in spring is @Configuration annotation which indicates that the class has @Bean definition methods. So Spring container can process the class and generate Spring Beans to be used in the application. This annotation is part of the spring core framework.
Do we need @configuration in Spring boot?
@Configuration is: not required, if you already pass the annotated class in the sources parameter when calling the SpringApplication. run() method; required, when you don't pass the annotated class explicitly, but it's in the package that's specified in the @ComponentScan annotation of your main configuration class.
What is the difference between @SpringBootApplication and @EnableAutoConfiguration annotation?
The @EnableAutoConfiguration annotation is based on @Conditional annotation of Spring 4.0 which enables conditional configuration. 7. The @SpringBootApplication annotation also provides aliases to customize the attributes of @EnableAutoConfiguration and@ComponentScan annotations.
What is @ComponentScan annotation in Spring boot?
Using @ComponentScan in a Spring Application. With Spring, we use the @ComponentScan annotation along with the @Configuration annotation to specify the packages that we want to be scanned. @ComponentScan without arguments tells Spring to scan the current package and all of its sub-packages.
What is @Qualifier annotation?
The @Qualifier annotation along with @Autowired can be used to remove the confusion by specifiying which exact bean will be wired.
Can you use annotation wiring in Spring?
Annotation wiring is not turned on in the Spring container by default. So, before we can use annotation-based wiring, we will need to enable it in our Spring configuration file. So consider the following configuration file in case you want to use any annotation in your Spring application.
What annotations are supported by Spring Framework?
JSR-250 Annotation s: These Spring annotations are supported by Spring Framework including @Resource, @PreDestroy and @PostConstruct annotations. These annotations are not really required as you already have the alternatives.
Can you use Spring annotation wiring in Spring Framework?
By default, Spring annotation wiring is not turned on in Spring Framework. Therefore, you need to enable it before you can use the Spring annotation-based wiring in the Spring Configuration file. Below is the configuration file in case you want to use annotation in your application:
What is the annotation in SQL?
The#N#@SqlConfig#N#@SqlConfig#N#annotation defines the metadata that is used to determine how to parse and execute SQL scripts configured via the#N#@Sql#N#@Sql#N#annotation. When used at the class-level, this annotation serves as global configuration for all SQL scripts within the test class. But when used directly with the config attribute of#N#@Sql#N#@Sql#N#,#N#@SqlConfig#N#@SqlConfig#N#serves as a local configuration for SQL scripts declared.
What is the annotation used for in Java?
This annotation is used on classes to indicate a Spring component. The#N#@Component#N#@Component#N#annotation marks the Java class as a bean or say component so that the component-scanning mechanism of Spring can add into the application context.
What is request mapping N#?
The#N#@RequestMapping#N#@RequestMapping#N#annotation is used to map web requests onto specific handler classes and handler methods. When#N#@RequestMapping#N#@RequestMapping#N#is used on class level it creates a base URI for which the controller will be used. When this annotation is used on methods it will give you the URI on which the handler methods will be executed. From this you can infer that the class level request mapping will remain the same whereas each handler method will have their own request mapping.
What is Spring Framework 4.3?
Spring framework 4.3 introduced the following method-level variants of#N#@RequestMapping#N#@RequestMapping#N#annotation to better express the semantics of the annotated methods. Using these annotations have become the standard ways of defining the endpoints. They act as wrapper to#N#@RequestMapping.#N#@RequestMapping.
What is class level annotation?
Class level annotation used to specify property sources for the test class.
What is @DataJpaTest annotation?
The @DataJpaTest annotation will only provide the autoconfiguration required to test Spring Data JPA using an in-memory database such as H2.
What language supports annotations?
The Java Programming language provided support for Annotations from Java 5.0. Leading Java frameworks were quick to adopt annotations and the Spring Framework started using annotations from the release 2.5. Due to the way they are defined, annotations provide a lot of context in their declaration.
What does @configuration annotation mean?
It clearly states that a class having @Configuration annotation tells Spring container that there is one or more beans that needs to be dealt with on runtime. Depending upon your type of configuration i.e. Web or Non-Web, Spring bootstrap your @Configuration classes with the context.
What is configurationmarks in Java?
I'm relatively new to Java and struggling with some of the language, but my understanding so far is: @Configurationmarks a class that contains methods that generate beans (which are really just classes getters/setters and at least a no-arg constructor). These methods should be annotated @Bean. This is important for Spring because this is how it manages IoC - it gathers methods that can create objects, and hunts around for @Autowireddefinitions (using reflection?). When it finds one, it executes the appropriate function that creates the object.

Objective
What Is Spring Annotation Based configuration?
- In Spring Framework annotation-based configuration instead of using XML for describing the bean wiring, you have the choice to move the bean configuration into component class. It is done by using annotations on the relevant class, method or the field declaration. Before performing XML, injection annotation injection is performed. Therefore, the la...
Spring Annotation List
- Here, we discussed some of the important Spring annotations: 1. @Required:It is applicable to bean property setter methods. 2. @Autowired:It is only applied to the bean property setter methods, constructors, non-setter methods and properties. 3. @Qualifier:This Spring Framework annotation along with the @Autowired is used for removing the confusion by specifying the exa…
Conclusion
- Hence, in this Spring Annotation Tutorial, we discussed what is an annotation in Spring Framework. In addition, we saw the types of Spring annotation configurations and how to configure them. Along with that you saw the working examples with Eclipse IDE in place for each of the annotations. Furthermore, if you have any query regarding Spring Annotation, feel free to a…