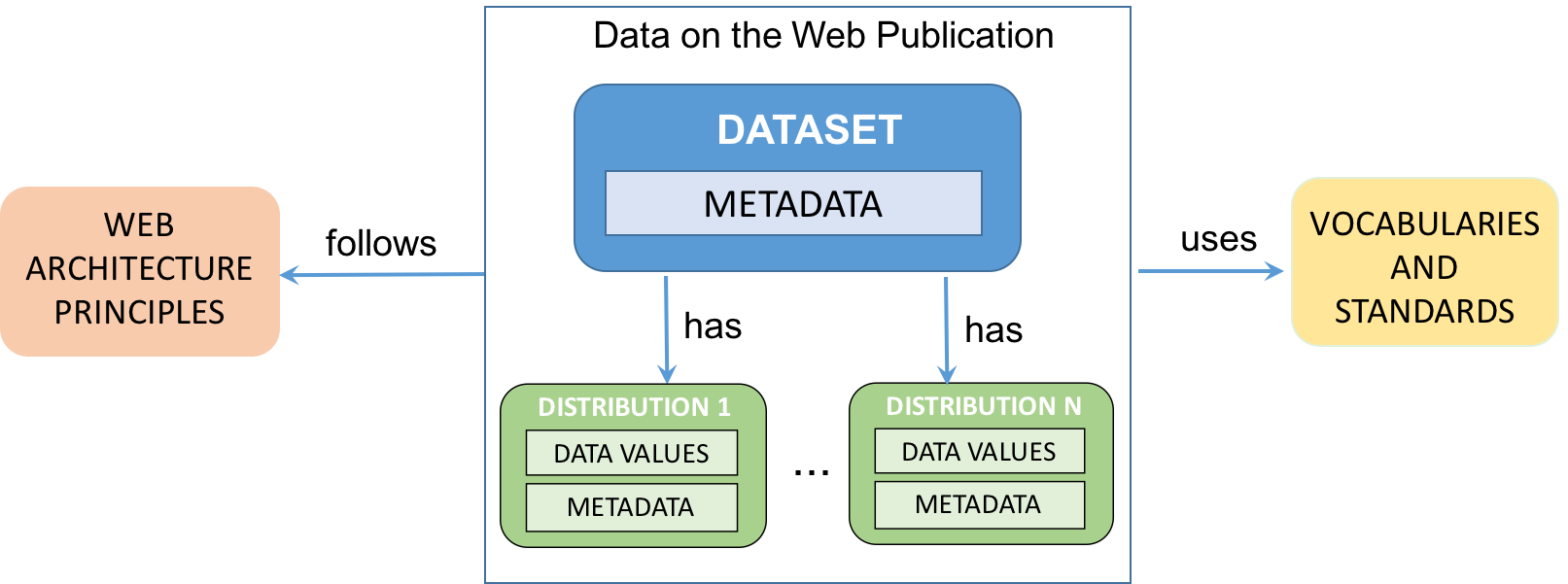
Full Answer
What is context manager in Python?
Python provides an easy way to manage resources: Context Managers. The with keyword is used. When it gets evaluated it should result in an object that performs context management. Context managers can be written using classes or functions(with decorators).
What are context managers used for?
A context manager usually takes care of setting up some resource, e.g. opening a connection, and automatically handles the clean up when we are done with it. Probably, the most common use case is opening a file. The code above will open the file and will keep it open until we are out of the with statement.
What is Context Manager service?
Access Context Manager allows Google Cloud organization administrators to define fine-grained, attribute based access control for projects and resources in Google Cloud. Administrators first define an access policy, which is an organization-wide container for access levels and service perimeters.
How do I create a context manager?
How To Create a Custom Context ManagerDefine a function.(optional) Write any setup code your context needs.Use the yield keyword.(optional) Write any teardown code your context needs.Add the @contextlib. contextmanager decorator.
What is context in programming?
A programming context can be defined as all the relevant information that a developer needs to complete a task. Context comprises information from different sources and programmers interpret the same information differently based on their programming goal.
What is context in Python function?
Context variable objects in Python is an interesting type of variable which returns the value of variable according to the context. It may have multiple values according to context in single thread or execution.
What is context manager Android?
Overview. Access Context Manager supports using Google basic mobile management and advanced mobile management to create access levels that check for certain characteristics of mobile devices. For iOS and Android devices, requests can be accepted or rejected based on: Whether screen lock is enabled.
What is async context manager?
Asynchronous context managers are, fairly logically, an extension of the concept of context managers to work in an asynchronous environment, and you will find that they are used a lot in asyncio-based library interfaces. An asynchronous context manager is an object which can be used in an async with statement.
What should __ exit __ return?
__exit__() documentation: If an exception is supplied, and the method wishes to suppress the exception (i.e., prevent it from being propagated), it should return a true value. Otherwise, the exception will be processed normally upon exit from this method.
What is __ str __ in Python?
Python __str__() This method returns the string representation of the object. This method is called when print() or str() function is invoked on an object. This method must return the String object.
Which methods are required to implement context management protocol?
They just return objects that implement the context management protocol....Creating Custom Context ManagersOpen and close.Lock and release.Change and reset.Create and delete.Enter and exit.Start and stop.Setup and teardown.
What is Python with statement?
In Python, the with statement replaces a try-catch block with a concise shorthand. More importantly, it ensures closing resources right after processing them. A common example of using the with statement is reading or writing to a file. A function or class that supports the with statement is known as a context manager.
What is a Context Manager?
The best way to describe context managers is to show an example almost every Python programmer has encountered at some point, unknowing they were using one! The bellow code snippet opens a .txt file and stores the lines in a python list. Let’s see what this looks like:
Is context lib bad?
This syntax is not too bad, but there is an easier way. The context lib provides a decorator that takes care of the boiler plate and replaces the class with function. Lets see how the above example would look using the decorator:
What is context manager?
Context managers allow you to allocate and release resources precisely when you want to. The most widely used example of context managers is the with statement. Suppose you have two related operations which you’d like to execute as a pair, with a block of code in between. Context managers allow you to do specifically that. For example:
What does contextmanager decorator do?
The contextmanager decorator returns the generator wrapped by the GeneratorContextManager object.
What happens if something other than True is returned by the __exit__ method?
If anything other than True is returned by the __exit__ method then the exception is raised by the with statement.
How many arguments does exit__ accept?
Our __exit__ method accepts three arguments. They are required by every __exit__ method which is a part of a Context Manager class. Let’s talk about what happens under-the-hood.
Does exit__ return true?
Our __exit__ method returned True, therefore no exception was raised by the with statement.
Can you use decorators in context manager?
We can also implement Context Managers using decorators and generators. Python has a contextlib module for this very purpose. Instead of a class, we can implement a Context Manager using a generator function. Let’s see a basic, useless example:
Working of Context Manager with Examples
As to what exactly is context manager and how it is used, we need to understand it. Context managers are the managers to manage resources. Say when a program wants to access the computer resources, it will first ask OS, and OS will provide it back to the program that requested that resource. E.g.
Implementing a Context Manager as a Class
It has two methods _enter_ () and _exit_ () in the class. The _enter_ () method is called when the execution of the program enters the context, and the _exit_ () method is called when execution leaves the context again to release the resources. Let us take an example:
Implement Context Manager as a Generator
In this way, the Python provides you in-built library known as contextlib for this purpose, so instead of constructing class, we can use this module to implement a context manager.
Conclusion
The context managers are used to manage the resources. In any other languages, file management can be done using try, finally, block but it might give an exception, and again even these are handled, and it might be a practice to say after opening a file it must be closed as there can be resource leakage, but most of the time we forget to do this.
Recommended Articles
This is a guide to Python Context Manager. Here we discuss the introduction, working of Context Manager along with the examples. You can also go through our other related articles to learn more–
What is context manager?
A context manager is an object that defines a runtime context executing within the with statement.
How does a context manager work?
Context managers can work with change and reset scenario. For example, your application needs to connect to multiple data sources. And it has a default connection. To connect to another data source: First, use a context manager to change the default connection to a new one. Second, work with the new connection.
When you use ContextManager class with the with statement, what does it do?
When you use ContextManager class with the with statement, Python implicitly creates an instance of the ContextManager class ( instance) and automatically call __enter__ () method on that instance.
Does Python always execute the exit__ method?
Python always executes the __exit__ () method even if an exception occurs in the with block.
Can context manager start timer?
For example, you can use a context manager to start a timer and stop it automatically.

Working of Context Manager with Examples
Implementing A Context Manager as A Class
Implement Context Manager as A Generator
Conclusion
Recommended Articles
- It has two methods _enter_() and _exit_() in the class. The _enter_() method is called when the execution of the program enters the context, and the _exit_() method is called when execution leaves the context again to release the resources. Let us take an example: Output: In this above program, the demo_file object is created of the class demo_file and then assigned to a variable …