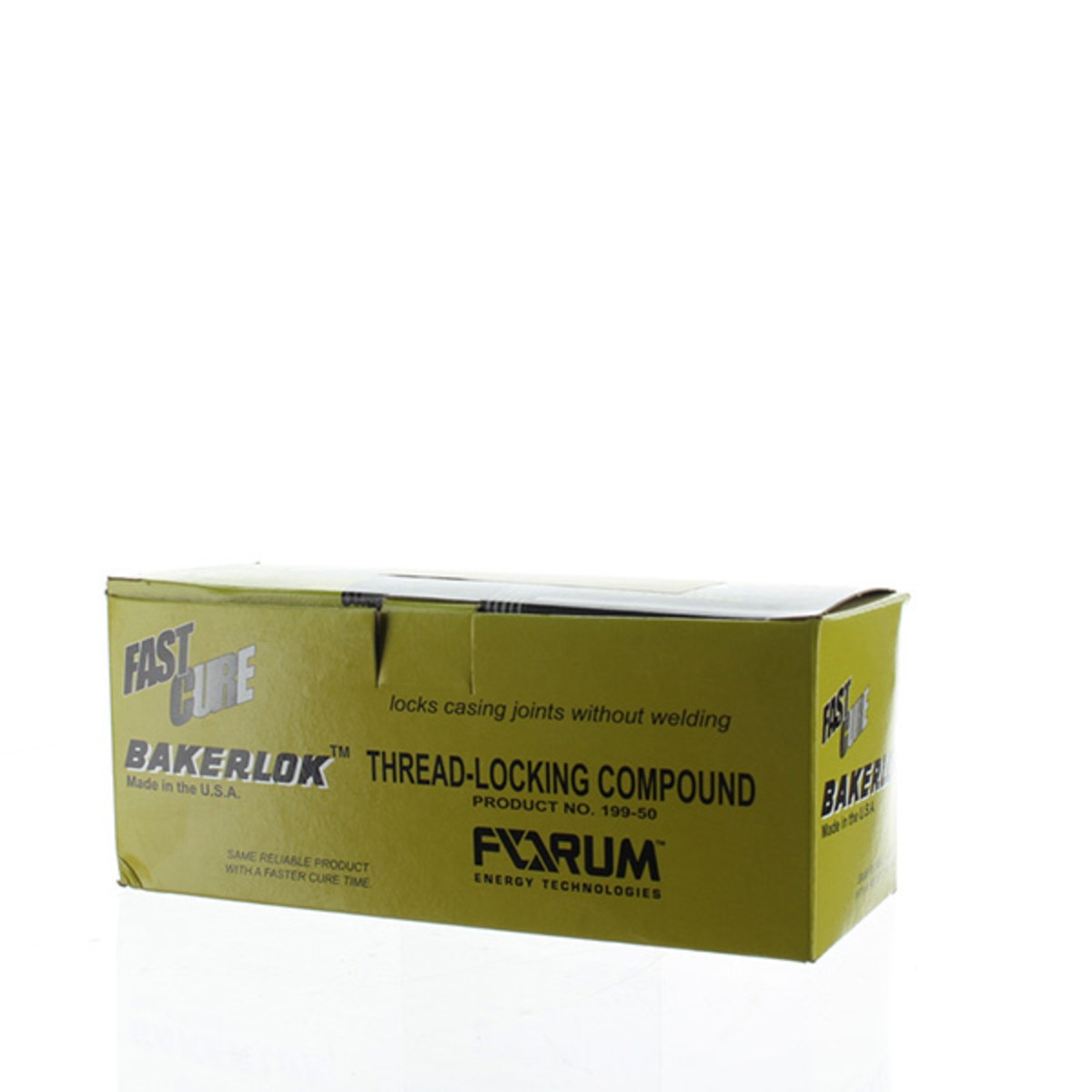
What happens when you detach a thread?
The answer basically states "you detach when you detach". Separates the thread of execution from the thread object, allowing execution to continue independently. Any allocated resources will be freed once the thread exits. After calling detach *this no longer owns any thread.
What is the difference between join and detach in threading?
detach basically will release the resources needed to be able to implement join. It is a fatal error if a thread object ends its life and neither join nor detach has been called; in this case terminate is invoked. This answer is aimed at answering question in the title, rather than explaining the difference between join and detach.
What is joining in threading with example?
“Joining” is one way to accomplish synchronization between threads. For example: The pthread_join () subroutine blocks the calling thread until the specified threadid thread terminates. The programmer is able to obtain the target thread’s termination return status if it was specified in the target thread’s call to pthread_exit ().
What happens when a thread ends without blocking or synchronizing?
Both threads continue without blocking nor synchronizing in any way. Note that when either one ends execution, its resources are released. After a call to this function, the thread object becomes non- joinable and can be destroyed safely.
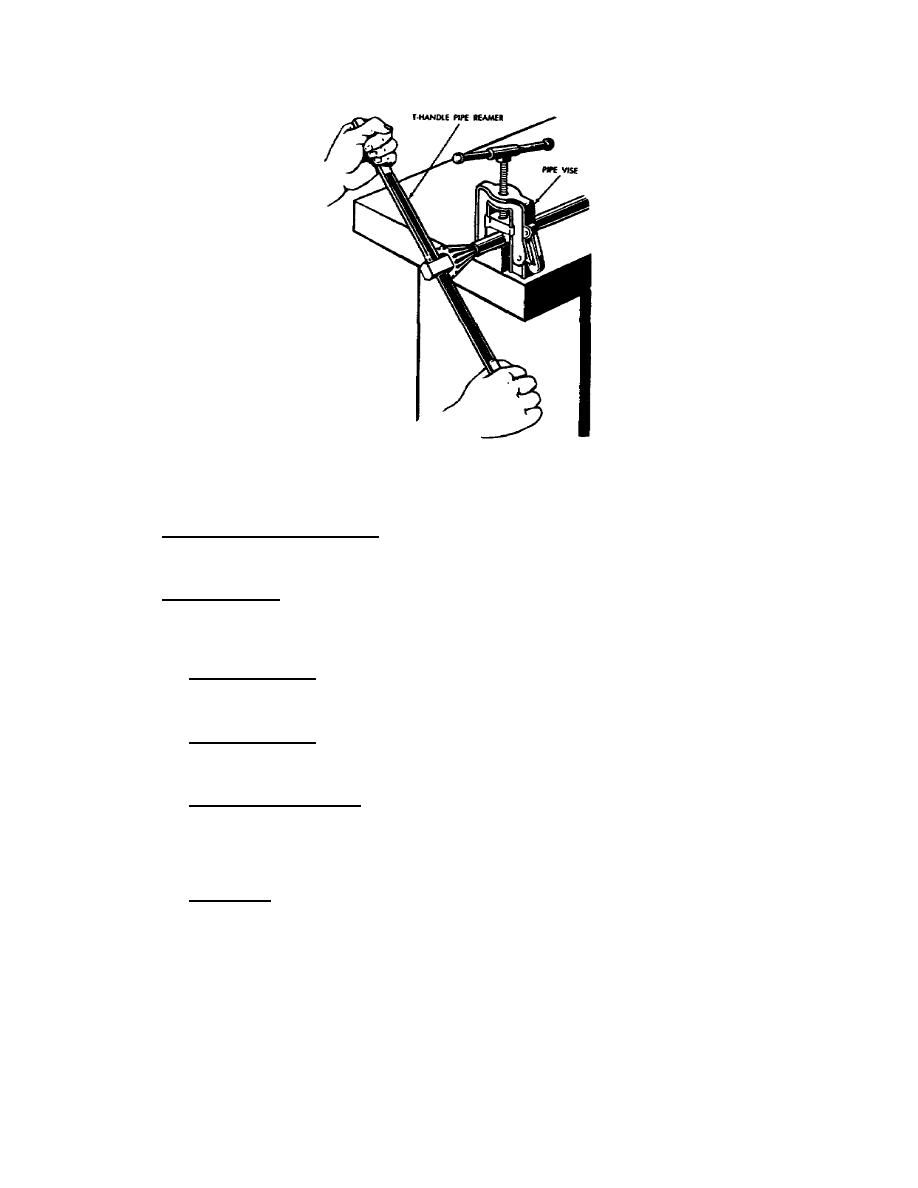
What happens when you detach a thread?
Detaching Threads Separates the thread of execution from the thread object, allowing execution to continue independently. Any allocated resources will be freed once the thread exits.
Can you join a detached thread?
5.6 [ISO/IEC 9899:2011], states that a thread shall not be joined once it was previously joined or detached. Similarly, subclause 7.26. 5.3 states that a thread shall not be detached once it was previously joined or detached.
How do you stop a thread from detaching?
In C++, once the thread is detached or uses the detach() function, then we cannot stop such threads, and still, if there is a need for stopping such threads, then only one way is to return the thread from the initial thread function by instantiating it in main() function, adding the Boolean value but before exiting ...
What does .join do in C++?
Syntax of C++ thread join The C++ thread join is used to blocks the threads until the first thread execution process is completed on which particular join() method is called to avoid the misconceptions or errors in the code. If suppose we are not using any join() method in the C++ code.
Why would you detach a thread?
You should call detach if you're not going to wait for the thread to complete with join but the thread instead will just keep running until it's done and then terminate without having the spawner thread waiting for it specifically; e.g. detach basically will release the resources needed to be able to implement join .
What happens if thread join is not called?
Yes, it is safe insofar as the thread will continue until completion by itself, you do not need to have a reference to the Thread object to keep it alive, nor will the behavior of the thread change in any way.
What is Native_handle?
thread::native_handle Returns the implementation defined underlying thread handle.
What is Jthread?
jthread fixes this; it joins on destruction by default (hence the name: "joining thread"). It also supports a mechanism to ask a thread to halt execution, though there is no enforcement of this (aka: you can't make another thread stop executing). At present, there is no plan to deprecate std::thread .
What is STD thread?
std::jthread The class jthread represents a single thread of execution. It has the same general behavior as std::thread, except that jthread automatically rejoins on destruction, and can be cancelled/stopped in certain situations.
Why We Use join in thread?
Join is a synchronization method that blocks the calling thread (that is, the thread that calls the method) until the thread whose Join method is called has completed. Use this method to ensure that a thread has been terminated. The caller will block indefinitely if the thread does not terminate.
Does join destroy a thread C++?
join() cannot be called on that thread object any more, since it is no longer associated with a thread of execution. It is considered an error to destroy a C++ thread object while it is still "joinable".
Do you always need to join threads?
no, you can detach one thread if you want it to leave it alone. If you start a thread, either you detach it or you join it before the program ends, otherwise this is undefined behaviour.
How do you make a detached thread?
Detached Thread & pthread_detach() No other thread needs to join it. But by default all threads are joinable, so to make a thread detached we need to call pthread_detach() with thread id i.e. int pthread_detach(pthread_t thread); #include
1:282:04Joinable and Detached Threads - YouTubeYouTubeStart of suggested clipEnd of suggested clipInstead. They stick around until another thread joins them this other thread makes a call to aMoreInstead. They stick around until another thread joins them this other thread makes a call to a thread join specifying.
A thread may either be joinable or detached. If a thread is joinable, then another thread can call pthread_join(3) to wait for the thread to terminate and fetch its exit status. Only when a terminated joinable thread has been joined are the last of its resources released back to the system.
A thread object is said to be joinable if it identifies/represent an active thread of execution. A thread is not joinable if: It was default-constructed. If either of its member join or detach has been called. It has been moved elsewhere.
Spawning and detaching 3 threads... Done spawning threads. (the main thread will now pause for 5 seconds) pause of 1 seconds ended pause of 2 seconds ended pause of 3 seconds ended pause of 5 seconds ended
Basic guarantee: if an exception is thrown by this member function, the thread object is left in a valid state.
After detaching, if the called thread completes after the calling thread, there should be no problem. Detaching means breaking the join (link).
When a thread ends its execution, the implementation releases all the resources that it owned. When a thread is joined, the body of the calling thread waits at that point until the called thread completes its execution, then the body of the calling thread continues its own execution.
A thread detaches from what? – A thread detaches from a join. The next question is “what is a join?” – Instead of having a program of statements running from the beginning to the end, sequentially, the program can be grouped into special sections of statements. The special sections are called threads, which can then run in parallel or concurrently. To convert a set of statements into a thread, special coding is needed. Unfortunately, threads in C++ would run independently if they are not joined. In such a situation, a second thread may end after the main thread has ended. This is usually not desirable.
For there to be any joining, two threads are needed. One thread calls the other thread. Joining a thread means that, while the calling thread is running, it would halt at a position and wait for the called thread to complete its execution (to its end), before it continues its own execution. At the position where the thread halts, there is a join expression. Such halting is called blocking.
A thread can be instantiated at global scope. The following program illustrates this:
And so execution continues with the main function after the called thread has exited successfully, as anticipated (with all its resources released). That is why,
A thread can only be detached after it has been joined; otherwise, the thread is already in the detached state.
The pthread_detach () routine can be used to explicitly detach a thread even though it was created as joinable.
If you know in advance that a thread will never need to join with another thread, consider creating it in a detached state. Some system resources may be able to be freed.
A joining thread can match one pthread_join () call. It is a logical error to attempt multiple joins on the same thread.
When a thread is created, one of its attributes defines whether it is joinable or detached. Only threads that are created as joinable can be joined. If a thread is created as detached, it can never be joined.
While Pthread detached behavior is different (1) The detached thread cannot be joined back once detached (2) The detached attribute merely determines the behavior of the system when the thread terminates; it does not prevent the thread from being terminated if the process terminates using exit (or equivalently, if the main thread returns). The pthread_detach () function marks the thread identified by thread as detached. When a detached thread terminates, its resources are automatically released back to the system without the need for another thread to join with the terminated thread.
The pthread_detach () function marks the thread identified by thread as detached. When a detached thread terminates, its resources are automatically released back to the system without the need for another thread to join with the terminated thread.
I think in terms of memory model, it's possible that thread A can exit while thread B can continues running. Moreover, program will exit if there are no user threads executing... or you can think only the daemon threads remain so the application will end in different way
Detached threads are background threads sometimes also called daemon threads. To detach a thread we need to call std::detach () function on std::thread object. ie., Once you call the detach (), then the std::thread object is no longer connected with the actual thread of execution.
In this case, if neither join nor detach is called with a ‘std::thread’ object that has associated executing thread then during that object’s destruct-or it will terminate the program.
When a join () function called on a thread object, join returns 0 then that ‘td::thread’ object has no associated thread with it. If the join () function again called on such an object then it will cause the program to Terminate. Similarly, detach () function will work.
How do I create a joinable thread?
Is thread join mandatory?
What does it mean for a thread to be joinable?
Example
Exception safety
What does it mean when a thread is detaching?
What happens when a thread ends its execution?
What is a thread in C++?
How many threads are needed for joining?
Can threads be instantiated at global scope?
Does execution continue after the thread exits?
Can a thread be detached?
What is pthread_detach?
What to do if you know a thread will never join with another thread?
Can a joining thread match a pthread_join call?
Can a thread be joined?
What does detached thread do?
What does pthread_detach do?
Can thread A exit while thread B continues running?
What is a detach thread?
What happens if neither join nor detach is called with a ‘std::thread’?
What happens when you call join on a thread?
Recall
Article Content
See more on educba.com
detach() Syntax
Ambiguity of Detaching in The Calling Thread’S Body
Thread Name at Global Scope
Detaching Within The called Thread
Conclusion