
JavaScript supports different kinds of loops:
- for - loops through a block of code a number of times
- for/in - loops through the properties of an object
- for/of - loops through the values of an iterable object
- while - loops through a block of code while a specified condition is true
- do/while - also loops through a block of code while a specified condition is true
How to break out of a for loop in JavaScript?
The good thing: break works for all JavaScript loops:
- for..of
- for..in
- for (i; i<length; i++)
- while
How to do for loops in JavaScript?
- Initialization condition: Here, we initialize the variable in use. ...
- Testing Condition: It is used for testing the exit condition for a loop. ...
- Statement execution: Once the condition is evaluated to true, the statements in the loop body are executed.
- Increment/ Decrement: It is used for updating the variable for next iteration.
How do I create an infinite loop in JavaScript?
Infinite Loop. In a while loop, you need to write a condition for the loop to continue to run. However, if you don't handle the condition correctly, it's possible to create an infinite loop. Look at this example, which tries to print out the numbers 0 to 9:
How to use setTimeout inside a for loop in JavaScript?
Using setTimeout in the example loop will not behave as expected, if you expect that there will be a one second interval between each task. What happens instead is the whole loop is executed in under a millisecond, and each of the 5 tasks is scheduled to be placed on the synchronous JS event queue one second later.
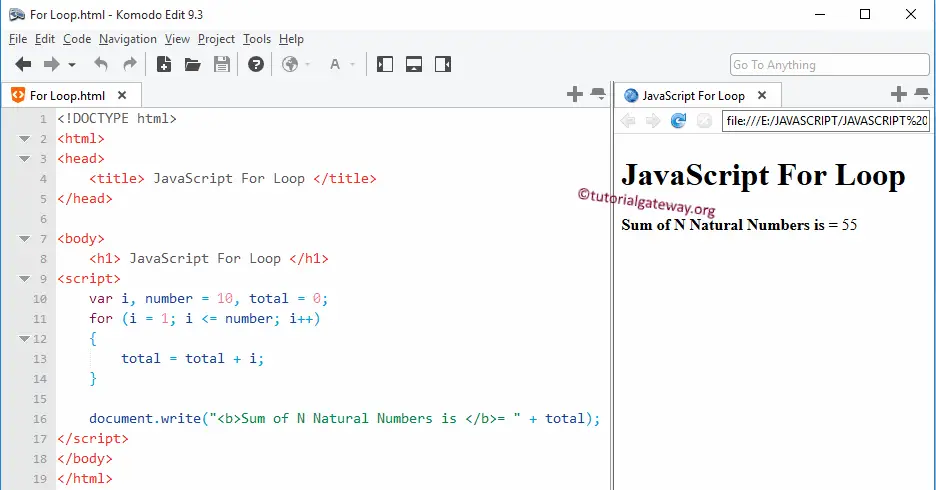
What are the loops in JavaScript with example?
Example 1: Display a Text Five TimesIterationVariableAction1sti = 1 n = 5I love JavaScript. is printed. i is increased to 2.2ndi = 2 n = 5I love JavaScript. is printed. i is increased to 3.3rdi = 3 n = 5I love JavaScript. is printed. i is increased to 4.4thi = 4 n = 5I love JavaScript. is printed. i is increased to 5.2 more rows
What is loops in JavaScript and explain its type?
Different Kinds of Loops for/in - loops through the properties of an object. for/of - loops through the values of an iterable object. while - loops through a block of code while a specified condition is true. do/while - also loops through a block of code while a specified condition is true.
What is loop and example?
A "For" Loop is used to repeat a specific block of code a known number of times. For example, if we want to check the grade of every student in the class, we loop from 1 to that number. When the number of times is not known before hand, we use a "While" loop.
How many loops are in JS?
There are 7 kind of loops you will find in JavaScript.
What are the 5 types of loops?
Types of Loops.While Loop.Do-While loop.For loop.Nested loop.Break Statement.Continue Statement.
What is loop syntax?
The syntax of a for loop in C programming language is − for ( init; condition; increment ) { statement(s); } Here is the flow of control in a 'for' loop − The init step is executed first, and only once.
What are the 3 types of loops?
In Java, there are three kinds of loops which are – the for loop, the while loop, and the do-while loop. All these three loop constructs of Java executes a set of repeated statements as long as a specified condition remains true. This particular condition is generally known as loop control.
What is called loop?
In computer programming, a loop is a sequence of instruction s that is continually repeated until a certain condition is reached. Typically, a certain process is done, such as getting an item of data and changing it, and then some condition is checked such as whether a counter has reached a prescribed number.
What are the 4 types of loops?
Types of Loops in CSr. No.Loop Type1.While Loop2.Do-While Loop3.For LoopJun 4, 2022
What is array in JavaScript?
Arrays are Objects Arrays are a special type of objects. The typeof operator in JavaScript returns "object" for arrays. But, JavaScript arrays are best described as arrays. Arrays use numbers to access its "elements".
What are JavaScript data types?
JavaScript typesBoolean type.Null type.Undefined type.Number type.BigInt type.String type.Symbol type.
What is loop in web programming?
In computer programming, a loop is a sequence of instructions that is repeated until a certain condition is reached. An operation is done, such as getting an item of data and changing it, and then some condition is checked such as whether a counter has reached a prescribed number.
What are JavaScript data types?
JavaScript typesBoolean type.Null type.Undefined type.Number type.BigInt type.String type.Symbol type.
How many types of loops are there in Java?
three typesIn Java, there are three types of loops.
How many types of looping structure present in JavaScript mention them?
JavaScript mainly provides three ways for executing the loops. While all the ways provide similar basic functionality, they differ in their syntax and condition checking time.
What is variable typing in JavaScript?
Like PHP, JavaScript is a very loosely typed language; the type of a variable is determined only when a value is assigned and can change as the variable appears in different contexts. Usually, you don't have to worry about the type; JavaScript figures out what you want and just does it.
What are the different types of loops in JavaScript?
JavaScript supports different kinds of loops: 1 for - loops through a block of code a number of times 2 for/in - loops through the properties of an object 3 for/of - loops through the values of an iterable object 4 while - loops through a block of code while a specified condition is true 5 do/while - also loops through a block of code while a specified condition is true
What is statement 2 in JavaScript?
Statement 2. Often statement 2 is used to evaluate the condition of the initial variable. This is not always the case, JavaScript doesn't care. Statement 2 is also optional. If statement 2 returns true, the loop will start over again, if it returns false, the loop will end.
Is statement 3 optional in JavaScript?
This is not always the case, JavaScript doesn't care, and statement 3 is optional. Statement 3 can do anything like negative increment (i--), positive increment (i = i + 15), or anything else. Statement 3 can also be omitted (like when you increment your values inside the loop):
Does JavaScript care about statement 1?
This is not always the case, JavaScript doesn't care. Statement 1 is optional.
What is a JavaScript loop?
The JavaScript loopsare used to iterate the piece of codeusing for, while, do while or for-in loops. It makes the code compact. It is mostly used in array. There are four types of loops in JavaScript. for loop.
What is looping in JavaScript?
Looping in JavaScript are used to repeatedly run a set of instruction while a given condition is true or false. JavaScript programming have four type of Loops.
How many types of loops are there in JavaScript?
There are four types of loops in JavaScript.
When to use while loop in JavaScript?
The JavaScript while loopiterates the elements for the infinite number of times. It should be used if number of iteration is not known. The syntax of while loop is given below.
Example
Try the following example to learn how a for loop works in JavaScript.
Output
Starting Loop Current Count : 0 Current Count : 1 Current Count : 2 Current Count : 3 Current Count : 4 Current Count : 5 Current Count : 6 Current Count : 7 Current Count : 8 Current Count : 9 Loop stopped! Set the variable to different value and then try...
What is an event loop?
Event loop: An event loop is something that pulls stuff out of the queue and places it onto the function execution stack whenever the function stack becomes empty.
What is the cycle called in JavaScript?
This cycle is called the event loop and this how JavaScript manages its events.
What does parallel run mean in JavaScript?
It means that the main thread where JavaScript code is run, runs in one line at a time manner and there is no possibility of running code in parallel.
How long can a loop execute?
Loops can execute a block of code as long as a specified condition is true.
Is a while loop the same as a for loop?
If you have read the previous chapter, about the for loop, you will discover that a while loop is much the same as a for loop, with statement 1 and statement 3 omitted.
Do while loops always execute?
The example below uses a do while loop. The loop will always be executed at least once, even if the condition is false, because the code block is executed before the condition is tested:
What happens to the message in an event loop?
At some point during the event loop, the runtime starts handling the messages on the queue, starting with the oldest one . To do so, the message is removed from the queue and its corresponding function is called with the message as an input parameter. As always, calling a function creates a new stack frame for that function's use.
What is a JavaScript message queue?
A JavaScript runtime uses a message queue, which is a list of messages to be processed. Each message has an associated function which gets called in order to handle the message.
How do two runtimes communicate?
Two distinct runtimes can only communicate through sending messages via the postMessage method. This method adds a message to the other runtime if the latter listens to message events.
What is concurrency model in JavaScript?
JavaScript has a concurrency model based on an event loop, which is responsible for executing the code, collecting and processing events, and executing queued sub-tasks. This model is quite different from models in other languages like C and Java.
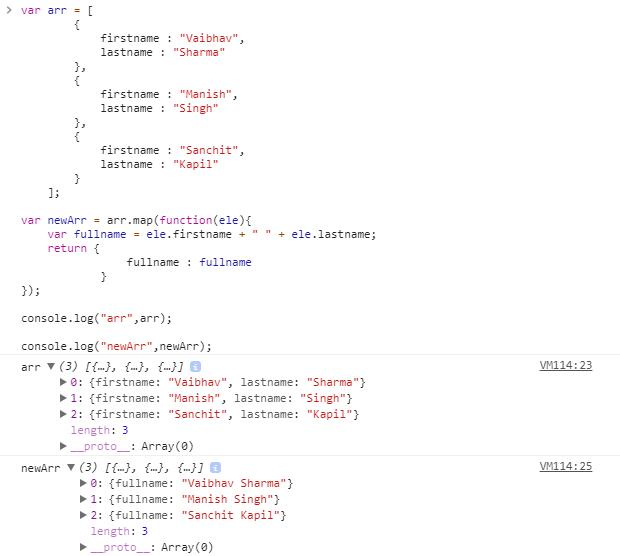