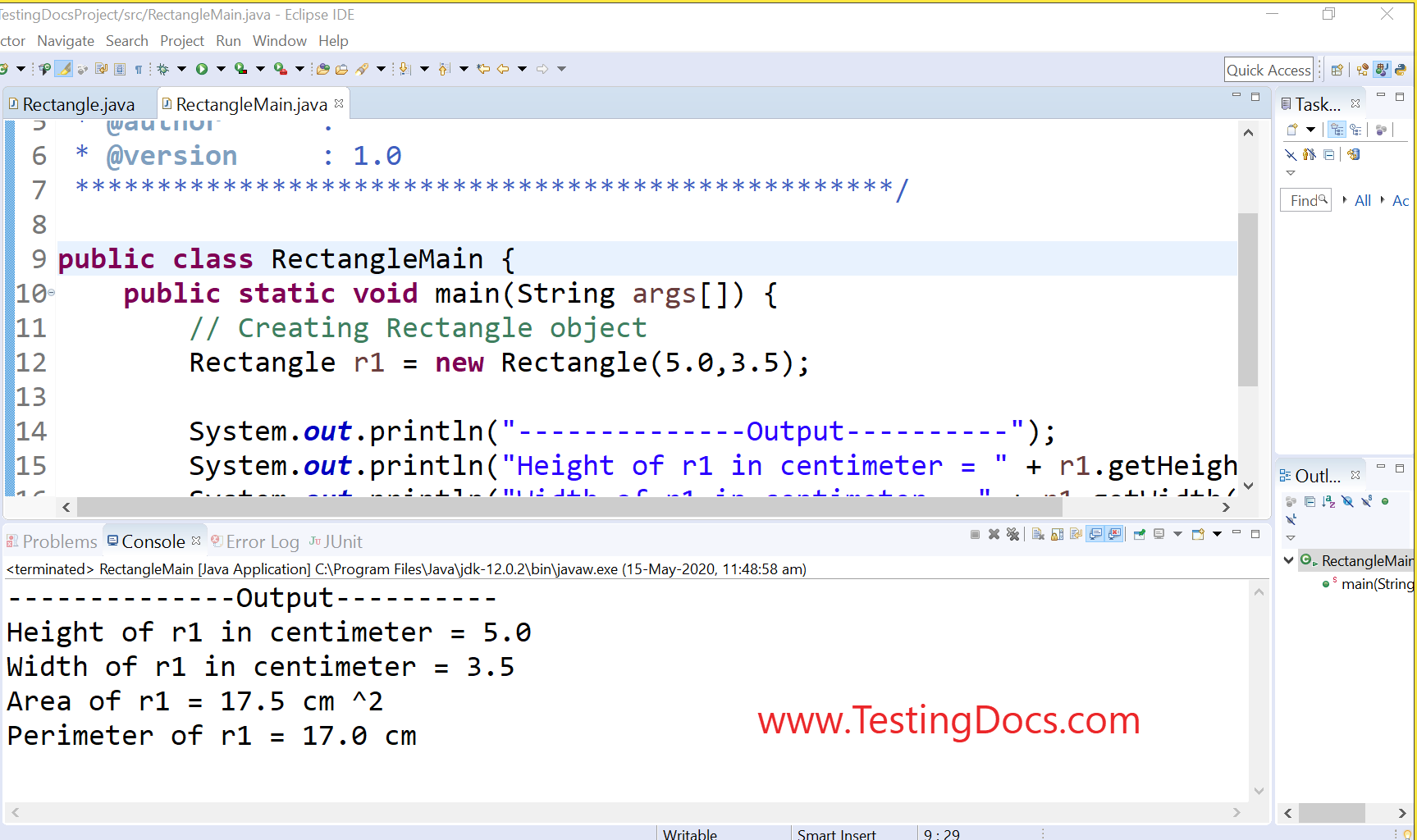
How do I format a number in Java?
Number Formatting in Java
- Overview. In this tutorial, we'll look at different approaches to number formatting in Java and how to implement them.
- Basic Number Formatting with String#format. The String#format method is very useful for formatting numbers. ...
- Decimal Formatting by Rounding. ...
- Formatting Different Types of Numbers. ...
- Advanced Formatting Use-Cases. ...
- Conclusion. ...
How to format decimal number in Java?
Using different Decimal Format Symbols to format data
- setDecimalSeperator () : This function sets any symbol to represent a decimal point.
- setGroupingSeperator () : This functions sets any symbol to represent a grouping symbol.
- setCurrency () : This function sets the number to any desired currency.
- setDigit () : This function is used to represent the digit in a format.
How to avoid number format exception in Java?
how to avoid NumberFormatException. As we know, while parsing a string in to int/floal by using the method Integer.parseInt (String s)/Float.parseFloat (String s), it will return int/float value, if the String contains any numeric value like "101" or "101.11". but if it is not numeric like "a12", then it will throw NumberFormatException.
How to convert ByteBuffer to string in Java?
There are three ways to convert a ByteBuffer to String:
- creating a new String from bytebuffer.array ()
- creating a new String from bytebuffer.get (bytes)
- using charset.decode ()

In which package is the class NumberFormat?
java.text packageNumberFormat class is present in java. text package, and it is an abstract class.
Is NumberFormat thread safe?
NumberFormat is not thread safe.
What is getCurrencyInstance in Java?
The getCurrencyInstance() method is a built-in method of the java.text.NumberFormat returns a currency format for the current default FORMAT locale. Syntax: public static final NumberFormat getCurrencyInstance()
What is decimal formatting in Java?
DecimalFormat is a concrete subclass of NumberFormat that formats decimal numbers. It has a variety of features designed to make it possible to parse and format numbers in any locale, including support for Western, Arabic, and Indic digits.
Is JDBC Driver thread-safe?
The PostgreSQL JDBC driver is thread safe. Consequently, if your application uses multiple threads then you do not have to worry about complex algorithms to ensure that only one thread uses the database at a time.
Is clone thread-safe?
No it is not thread safe if two threads are trying to execute this method over the same instance of Foo. You should create a mutex using this instance . For example place the code which executes this clone method in synchronized(fooInstance) block.
What does '%' mean in Java?
modulusmodulus: An operator that works on integers and yields the remainder when one number is divided by another. In Java it is denoted with a percent sign(%).
What is getMax in Java?
The getMax() method of LongSummaryStatistics class in Java is used to get the maximum of records in this LongSummaryStatistics. Syntax: public int getMax() Parameter: This method do not accept any value as parameter. Return Value: This method returns the maximum of the records in this LongSummaryStatistics.
What does '*' mean in Java?
* (Multiplication) Multiplies values on either side of the operator. A * B will give 200. / (Division) Divides left-hand operand by right-hand operand.
What is %d called in Java?
Format decimalFormat specifiersSpecifierExplanation%dFormat decimal (integer) numbers (base 10)%eFormat exponential floating-point numbers%fFormat floating-point numbers%iFormat integers (base 10)6 more rows•Mar 23, 2022
What is %s and %D in Java?
%d means number. %0nd means zero-padded number with a length. You build n by subtraction in your example. %s is a string. Your format string ends up being this: "%03d%s", 0, "Apple"
What are the 3 types of decimals?
What are the 3 types of decimals? Three types of decimals are non-recurring (non-repeating or terminating, like ); recurring (repeating or non-terminating, like ); and decimal fractions (a.k.a. converting a decimal into a fraction whose denominator is a power of ten).
Is simple data format thread-safe?
It is recommended to create separate format instances for each thread. If multiple threads access a format concurrently, it must be synchronized externally. So SimpleDateFormat instances are not thread-safe, and we should use them carefully in concurrent environments.
Is Boost Filesystem thread-safe?
Almost all classes provided by Boost. Signals2 are thread safe and can be used in multithreaded applications.
Is CSV writer thread-safe?
This class implements multi-threading on writing more than one bean, so there should be no need to use it across threads in an application. As such, it is not thread-safe.
What is NumberFormat?
NumberFormat helps you to format and parse numbers for any locale. Your code can be completely independent of the locale conventions for decimal points, thousands-separators, or even the particular decimal digits used, or whether the number format is even decimal.
What is number format?
NumberFormat helps you to format and parse numbers for any locale. Your code can be completely independent of the locale conventions for decimal points, thousands-separators, or even the particular decimal digits used, or whether the number format is even decimal.
How to get a normal number format?
Use getInstance or getNumberInstance to get the normal number format. Use getIntegerInstance to get an integer number format. Use getCurrencyInstance to get the currency number format. And use getPercentInstance to get a format for displaying percentages. With this format, a fraction like 0.53 is displayed as 53%.
Why do you format multiple numbers?
If you are formatting multiple numbers, it is more efficient to get the format and use it multiple times so that the system doesn't have to fetch the information about the local language and country conventions multiple times.
Is number format synchronized?
Number formats are generally not synchronized. It is recommended to create separate format instances for each thread. If multiple threads access a format concurrently, it must be synchronized externally.
NumberFormat Class in Java
The format of currencies in different countries around the world varies widely. They also follow different number systems to represent the numbers. In Java, NumberFormat is the abstract base class present in java.text package that is used to represent numbers in all number formats.
Summary
The knowledge of NumberFormat class of java.text package is very important for a Java developer to know while developing an application that will be used worldwide. In this tutorial, we covered the methods of NumberFormat class that can be used to format the data according to their locale.
What is number format?
NumberFormat is a Java class for formatting and parsing numbers. With NumberFormat, we can format and parse numbers for any locale.
What is the number format.getPercentInstance?
The NumberFormat.getPercentInstance () is used to format percentages.
How to control number of fraction digits?
We can control the number of fraction digits with the setMinimumFractionDigits () and setMaximumFractionDigits (). If there are fewer digits than the minimum number of fraction digits, zeros are added to the value. If there are more digits than the maximum number of fraction digits, the number is rounded.
What happens if there are more fraction digits than the maximum number of allowed digits?
As we have already stated above, if there are more fraction digits than the maximum number of allowed digits, the value is rounded. There are several rounding techniques available.
What is setgroupingused in math?
For ease of reading, numbers with many digits may be divided into groups using a delimiter. The setGroupingUsed () sets whether grouping is used in the format.
What is the most complex task when working with numbers?
One of the most complex tasks when working with numbers is to format currencies. We use the NumberFormat.getCurrencyInstance () to get the number format for the currencies.
Can you use underscores in Java?
Since Java 7, it is possible to use underscore characters in numeric literals.
Why does numberformat exception occur?
Since NumberFormatException occurs due to the inappropriate format of string for the corresponding argument of the method which is throwing the exception , there can be various ways of it. A few of them are mentioned as follows-
How to avoid NumberFormatException?
So, to avoid this exception, the input string provided has to be well formatted.
How to make a string valid?
To have a valid and well-formatted string, first of all , check whether the input string is not null. Then, check for unnecessary spaces and trim out all of them after that put several checks to verify that argument string matches the type of the method which we are using for parsing the string. If the method is ParseInt (), check that the string is has an integer value and likewise for all other methods do the required checks.
